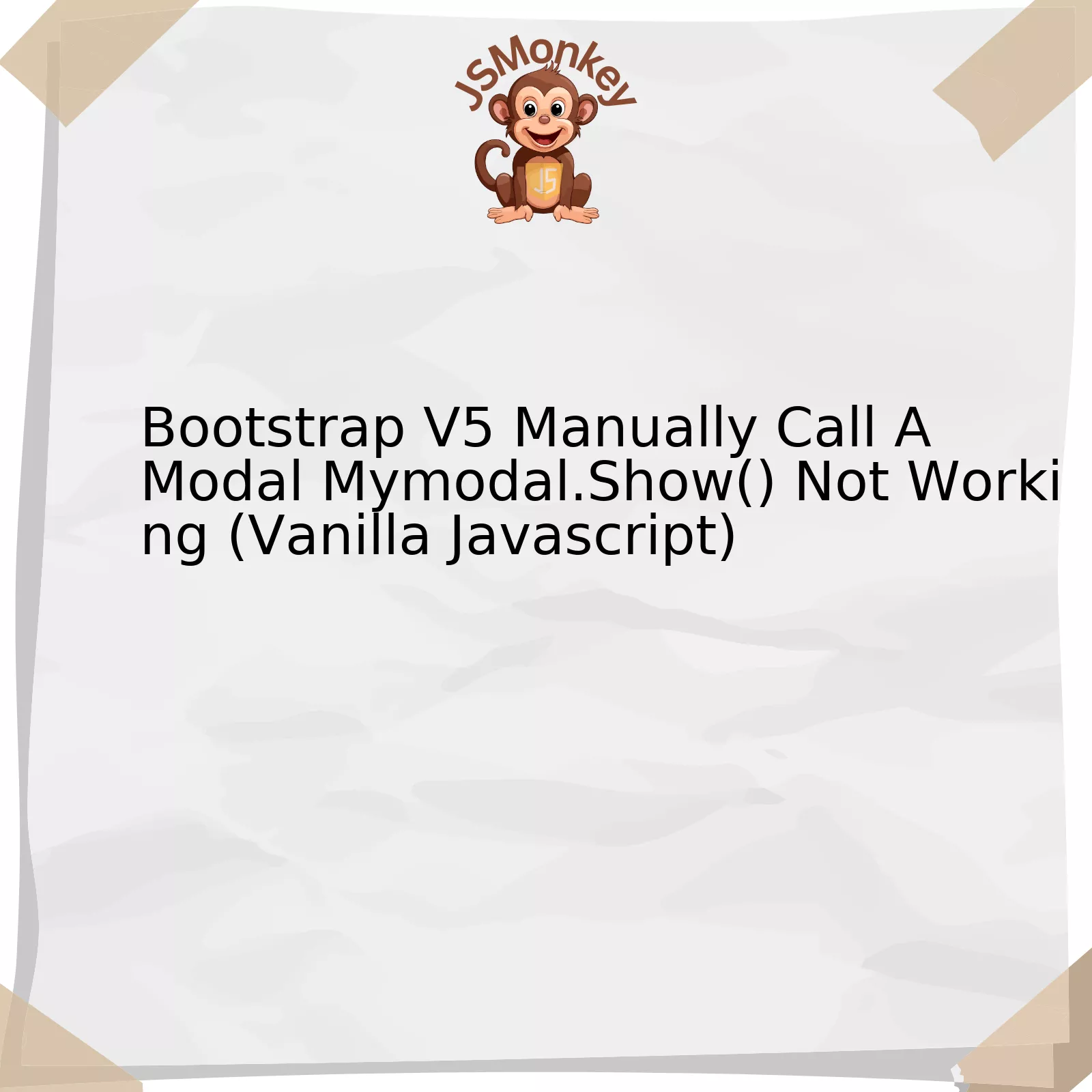
When working with Bootstrap V5 and Vanilla JavaScript, often developers experience the issue of not being able to manually call a modal using
mymodal.show()
. Before dissecting this further, it’s crucial to understand the structure of a typical modal in Bootstrap V5.
Modal Part | Description |
---|---|
Modal Dialog | This outermost container holds everything concerning the modal dialog. |
Modal Content | The place where we add the contents of the modal. It wraps the header, body, and footer sections. |
Modal Header | Contains the title section for the modal, along with the close button. |
Modal Body | This is where you place the main content that you want to display to the users when the modal pops up. |
Modal Footer | Usually contains actions related to the modal, like a close or save button. |
One potential reason that you may be unable to call a Bootstrap V5 modal manually might be if your project does not correctly import or use the required Bootstrap JavaScript files. Remember that Bootstrap has migrated away from JQuery starting with version 5, so we need to leverage vanilla JavaScript moving forward. Thus, ensure the correct JavaScript bundle file is linked in your script tag.
A right way to call a modal programmatically in Vanilla JavaScript in Bootstrap V5 looks as follows:
var myModal = document.getElementById('myModal') var myInput = document.getElementById('myInput') myModal.addEventListener('shown.bs.modal', function () { myInput.focus() })
This event listener is triggered when the modal has been made visible to the user (will wait for CSS transitions to complete). It then makes sure to set the focus on the input field within that modal.
Another potential problem could be if the HTML structure of the modal is not properly set up following Bootstrap’s guidelines. Incorrect addition or removal of required classes, such as ‘modal-dialog’, ‘modal-content’, and others, could lead to failures in functionality.
As Herbert A. Simon once said, “The programmer, like the poet, works only slightly removed from pure thought-stuff. He builds his castles in the air, from air, creating by exertion of the imagination.”. Debugging cases like this involves understanding the detailed structure and features of the library you’re working with. It requires a mix of creativity and logic, much like crafting an architectural masterpiece out of thin air.
Troubleshooting Modal Issues in Bootstrap V5
When you’re troubleshooting modal issues in Bootstrap v5, specifically for the use case where manually calling a modal with `mymodal.show()` doesn’t work as expected, there are several factors to consider. This discussion will focus primarily on possible reasons for this issue and solutions using Vanilla Javascript.
– First, it’s essential to confirm that the Bootstrap JavaScript library has been correctly imported. Without this key resource, the functionality underpinning modal behavior simply won’t be available, and any attempts to instantiate or show modals will lead to errors. Reference the official documentation for guidance on including the library.
– In accordance with the instructions provided in the Bootstrap v5 documentation, you have to create an instance of the modal first before calling the `show()` method:
var myModalEl = document.getElementById('myModal') var myModal = new bootstrap.Modal(myModalEl) myModal.show();
– Pay close attention to how the `bootstrap.Modal` object gets instantiated. The HTML element chosen must indeed relate to the modal’s ID. If the incorrect ID is used, or if the proper modal does not exist within the DOM, the resultant error will prevent the modal from being displayed.
– Check your browser console for any arising errors. Errors can indicate uncaught exceptions or syntax problems, which can thwart the successful execution of your script, including the display of modals.
It’s critical to understand that debugging software issues is often a process of elimination. “The best debugger ever made is a good night’s sleep.”—Bob Metcalfe
Working through these points systematically should shed light on your modal issue and ultimately facilitate its resolution.
Understanding the Manually Call Method: Mymodal.Show()
Understanding the intricacies of invoking a modal in Bootstrap V5 manually through
Mymodal.show()
using Vanilla JavaScript can be critical. The manual method calling allows explicit control, finely tuning when and how the modal dialog appears.
First off, keep in mind that Bootstrap V5 has moved away from jQuery and embraces Vanilla JavaScript, unlike its predecessors. Consequently, one frequent malfunction occurs if jQuery continues to be employed.
So, how does this manual method call work? A new modal instance must be created for every modal on a page. Subsequently, applying the
show()
command will open the dialog:
Code |
---|
var myModal = new bootstrap.Modal(document.getElementById('myModal'), options); myModal.show(); |
The `myModal` represents the id of your modal, while `options` refers to an object containing any required configurations. If the `
myModal.show()
` is not working, it often owes to:
1. An incorrect id was used when initializing the new
bootstrap.Modal()
instance, so the modal that should be displayed is not properly identified.
2. The modal has not been initialized properly with
new bootstrap.Modal()
.
3. Not instantiating a new modal for each id on the page, leading to conflicts – particularly pertinent for dynamic web pages with multiple modals.
4. Not loading the proper Bootstrap scripts or loading them in an incorrect order – noteworthy is that BootStrap’s JavaScript relies on Popper, which must be imported before Bootstrap’s JavaScript.
As Paul Irish, a Google Chrome developer famously said, “In JavaScript, there is a beautiful, elegant, highly expressive language that is buried under a steaming pile of good intentions and blunders.” Understanding these nuances, such as the proper utilization of bootstrap’s
show()
, can bring one step closer to unearthing this elegant language.
Common Mistakes when Using Vanilla Javascript with Bootstrap Modals
When utilizing Bootstrap modals with vanilla JavaScript, a range of common mistakes can potentially impede functionality. Most prominent among these issues is the inability to manually implement your Modal. A consequence of this is that when attempting to use `MyModal.show()`, it may not perform as intended. Delving deeper into this topic, we shall discuss why this issue might occur, possible solutions, and best practises.
While working with bootstrap v5 and trying to manually call a modal using the command `MyModal.show()`, one common mistake developers often make resides in the sequence they link their JavaScript files. If you place the Bootstrap’s scripts links before your custom script, it can result in an error or unexpected results because the custom script might run before Bootstrap’s components are fully loaded.
Incorrect way: |
---|
<script src="custom.js"></script> <script src="bootstrap.js"></script> |
Correct way: |
<script src="bootstrap.js"></script> <script src="custom.js"></script> |
Another common error arises from incorrectly initializing the modal. The init function may be missing or improperly placed. Here’s the correct way to do it:
var myModal = new bootstrap.Modal(document.getElementById('myModal'), options);
The modal works via an instance object and by neglecting the `new` keyword, you compromise the functionality of the modal’s `.show()` method. Your modal instance should strictly follow object-oriented programming practices to avoid common pitfalls.
Moving forward, understanding that initializing the modal before DOM is fully loaded can lead to unexpected behaviour is paramount. Thus, delaying the script until after all HTML is parsed becomes key. The impact of this practice was expressed by Brendan Eich, creator of JavaScript, who stated: “Always bet on JavaScript.” This reinforces the importance of JavaScript patterns and anticipating nuances within its operational domain.
Another frequent error stems from referencing a non-existent modal or using an incorrect ID. Your scripts should always reference existing elements on the page; if they don’t, troubleshooting such errors can prove challenging. E.g., the element (`myModal`) needs to exist for `MyModal.show()` to work correctly under normal circumstances.
Therefore, as you integrate Bootstrap modals with your vanilla JavaScript, remaining mindful of these common mistakes will support in creating harmonious functionality across your web applications. Standardizing correct scripting practices and methodically laying out your code’s sequence encourages a disciplined approach towards programming – culminating in resilient, efficient code!
Reference:
Bootstrap Modal Documentation
Effective Use of Bootstrap V5 and Vanilla JavaScript for Modal-Handling
Harnessing the power of Bootstrap V5 for manual modal handling in conjunction with Vanilla JavaScript can prove to be immensely advantageous in generating interactive web elements. It’s crucial, however, to consider certain factors that could potentially interfere with the functionality of a method like `show()`. In particular, the issue of `MyModal.show()` not functioning properly could arise from a variety of reasons.
To begin with, it is important to ascertain that we are initializing our modal correctly. It involves referencing the correct modal ID and ensuring that our Vanilla JavaScript code syncs perfectly with the HTML structure. Here’s an example:
html
In the JavaScript code, make sure to initialize your modal using the correct ID as follows:
javascript
var myModal = new bootstrap.Modal(document.getElementById(‘myModal’));
Also, always keep in mind the order in which script tags are placed on your webpage. The script tag that contains the Bootstrap JS library should precede the one that holds your Vanilla JavaScript code. This is due to the fact that JavaScript executes synchronous code in a sequential manner. Hence, placing the bootstrap library after your script might cause failure since it won’t yet recognize the bootstrap objects and methods at runtime.
Regarding this matter, Bootstrap’s official documentation provides useful tips and examples.
While troubleshooting `Mymodal.show()`, it also pays to scrutinize the javascript console for any errors. A common situation is when the code doesn’t recognize `bootstrap` as an object. However, it could be rectified by adding Popper.js before Bootstrap.js.
Here’s how you could ideally structure it:
html
Software engineer, Rasmus Lerdorf, succinctly sums up our approach to such issues when involved in coding: “Solving big problems is easier than solving little problems.” In this context, we need to dive deeper into understanding how Bootstrap 5 and Vanilla JavaScript interact and operate together, rather than merely grappling with smaller individual errors.
By giving careful attention to the initialization of your modal, structure of your scripts, and debugging errors from the console, it is possible to successfully handle any glitches that occur while manually triggering modals using Bootstrap V5 and Vanilla JavaScript.
The issue with manually calling a modal using
mymodal.show()
in Bootstrap v5, particularly when using Vanilla JavaScript, is an occurrence that developers often face. It’s critically important to understand the nuances within this common scenario and how it can be resolved.
Bootstrap V5 introduced some significant changes from its previous versions. Notably, jQuery was removed, and all its functionalities were translated into pure JavaScript or Vanilla JS. Such a significant change has affected several features, including how modals function.
When you encounter the problem of
mymodal.show()
not working, ensure that your syntax aligns with the modifications included in Bootstrap v5. Unlike the previous versions where you could call a modal by using jQuery, with Bootstrap v5, you need to create a new instance of the Modal class for each modal you want to interact with.
Here is an example of how you should correctly call a modal in Bootstrap v5:
var myModal = document.getElementById('myModal') var myModalInstance = bootstrap.Modal.getInstance(myModal)
After creating the modal instance (myModal), we can now call the show method:
myModalInstance.show()
If the usual vanilla JavaScript initialization and manipulation methods don’t solve the issue, consider checking if all necessary scripts and dependencies are loaded correctly, ensuring there’s no JavaScript conflict from other sources or scripts.
It’s also worth exploring the official Bootstrap documentation from time to time, which proves itself an invaluable resource to stay current with the updates or changes.(source)
As Brendan Eich, the creator of JavaScript, once said, “Always bet on JavaScript.” Adapting to changes in versions and understanding nuances are part of growing as a developer. Mastering these facets, such as getting Bootstrap’s
mymodal.show()
to function as expected, will surely aid in that growth.