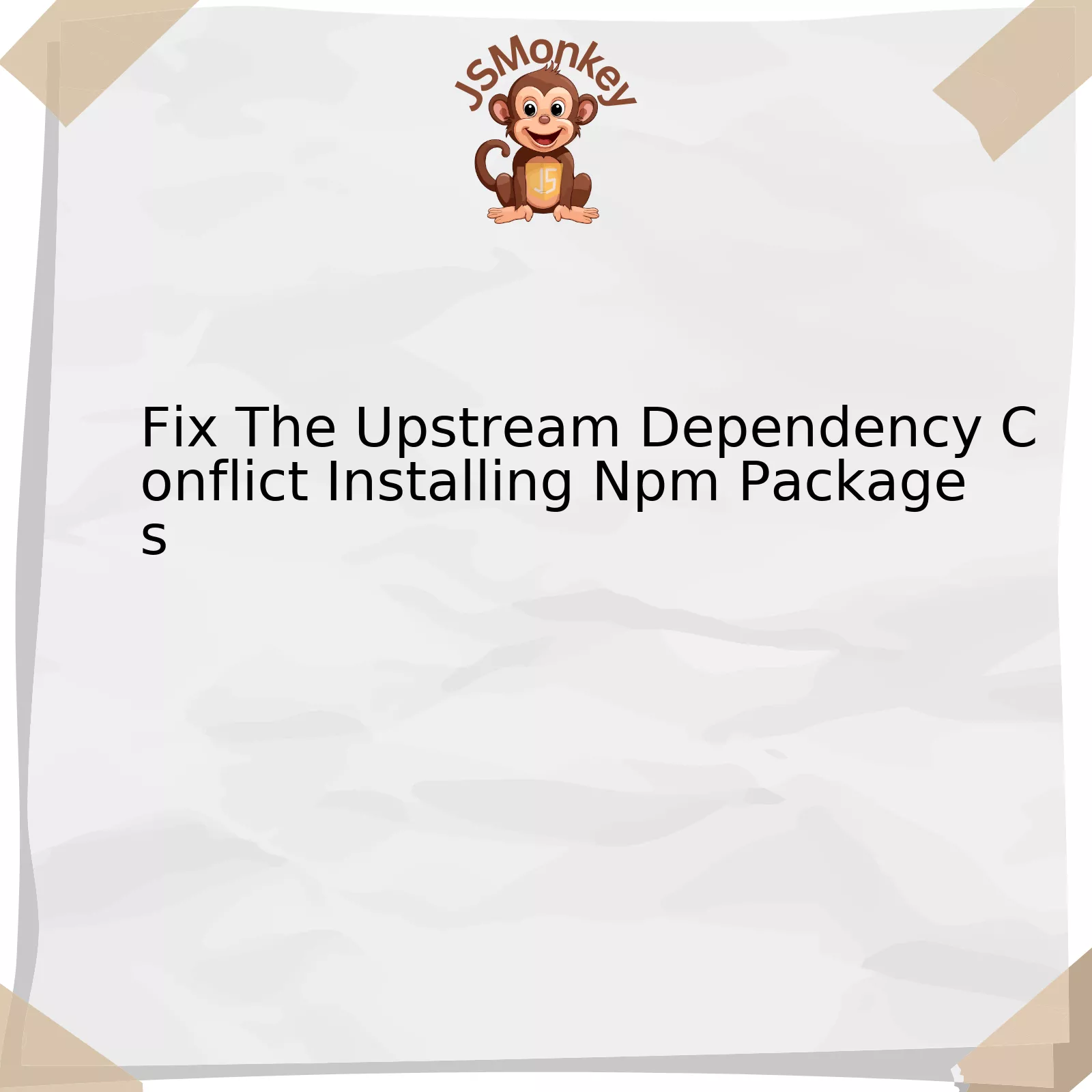
When working with Node Package Manager (npm), you may encounter an “upstream dependency conflict.” This typically occurs when different packages required by your project depend on different versions of the same module. Here are the possible reasons for this issue, potential solutions, and relevant programming quotes:
Problem | Solution |
---|---|
Incompatible Dependency Versions | One way to resolve conflicts is by using the
npm dedupe command that aims at simplifying the structure of your project’s node_modules folder by reducing redundancy. |
Dependent Packages Not Updated | Please ensure to update package dependencies regularly. The
npm outdated command can assist in showing what’s available to upgrade. |
Mismatched Node.js and npm Versions | Check if you’re working with a compatible version of Node.js and npm. You might need to update either of them using the
npm install -g npm , or download the latest Node.js distribution. |
Let’s delve deeper into these points for a fuller understanding.
Incompatible Dependency Versions
It’s always crucial to ensure all dependencies within the project are compatible with each other. Due to various project requirements, there might be multiple versions of the same package present, thus leading to compatibility issues being brought about by conflicting versions.
Douglas Crockford perfectly captures it when he said, “JavaScript is built on some very good ideas and a few very bad ones.” This can also be seen in npm where incompatible dependencies can cause quite a bit of headache.
Dependent Packages Not Updated
Keeping project package dependencies updated helps prevent conflicts. This also keeps the application secure by patching vulnerabilities potentially present in outdated packages. An apt quote from Joanna Wiebe fits here- “People don’t want better content – they want a better path.”
Mismatched Node.js and npm Versions
The versions of Node.js and npm should be compatible as an incomparability can lead to conflicts. Often, an upgrade is required to resolve this issue.
As JavaScript developer Kyle Simpson stated, “Programming is not about typing, it’s about thinking.” Indeed, resolving dependency conflicts often require thoughtful solutions over quick fixes. Ensuring compatibility among all elements is key to a smooth coding journey.
Overcoming Challenges in Npm Packages Installation
Npm, or Node Package Manager, is a crucial tool for JavaScript developers as it assists in managing and hosting reusable code packages. It’s important to note that, like any other system, npm might sometimes encounter difficulties during package installation. This can potentially influence project timelines or even disrupt the progress of a critical task if proper action isn’t taken.
One frequent problem faced by developers when installing npm packages involves encountering an ‘upstream dependency conflict’. This typically transpires when two (or more) dependencies in a project need different versions of the same common dependency.
For instance, consider the following scenario:
-
Package A
depends on
Package C@1.0.0
-
Package B
depends on
Package C@2.0.0
To address such conflicts effectively, here are a few solutions:
Avoid using ‘*’ as version
Don’t specify ‘*’ as your package version. While this might initially seem convenient as it installs the latest version of the package, it may also lead to conflicting dependencies when there is a major update that breaks the compatibility with your project.
<dependencies> ... "packageA": "1.0.0", "packageB": "2.3.0", ... </dependencies>
npm –force
If nothing else works, you could use the
--force
flag to force npm to fetch remote resources even if a local copy exists on disk. This should be used cautiously as it can sometimes lead to unstable conditions.
npm dedupe
Though considered as a last resort, running
npm dedupe
might help in reducing redundancy by trying to find a version of a package that can work in all places it’s needed. Please note that it might not be effective if conflicts exist at non-dedupe-able spots.
Even Bill Gates, one of the pioneers in the tech world, has said: “I choose a lazy person to do a hard job. Because a lazy person will find an easy way to do it.” This sentiment resonates well in resolving npm dependency conflicts as finding the simpler path could save you time in focus on what truly matters – creating outstanding software.
Through paying careful attention to these suggested strategies, overcoming challenges related to upstream dependency conflicts during npm package installation is entirely feasible.
For more details and additional advice, refer to the official npm documentation which offers valuable insights.
Critical Factors to Consider When Handling Upstream Dependency Conflicts
When you are dealing with upstream dependency conflicts while installing npm packages, several critical factors should certainly be taken into account:
– Review the Dependency Tree: As a part of package installation, `npm` creates a dependency tree, which includes not only your project’s immediate dependencies but also their respective dependencies. Using the
npm ls
command can provide a visual representation of this tree where you can swiftly identify potential points of conflict.
– Understanding SemVer (Semantic Versioning): Upstream packages often use semantic versioning. This system works as follows MAJOR.MINOR.PATCH – each number represents the level of change in the new update. It is essential to understand how this influences the version of the package that npm will install based on the version range specified in your package.json file.
Steve Jobs once said; “We’re just enthusiastic about what we do.” Similarly, when working with Semantic Versioning and package versions, it is important to have a good understanding and always strive for accuracy.
– Dealing with Breaking Changes: If a new version of a dependency has introduced breaking changes and your project or one of its dependencies relies on the older functionality, this could cause a conflict.
– Deal with Transitive Dependencies: These are the dependencies of your direct dependencies. Incase multiple intermediary dependencies are requiring different versions of the same package, then this could result in a conflict.
– Using Npm Audit Resolve: For security issues,
npm audit resolve
can become incredibly handy. It generates a guided tool that aids to resolve any vulnerabilities discovered by
npm audit
.
Please note that handling upstream dependency conflicts can be challenging; however, with the right approach, you can effectively manage them with relative ease. Avoid ignoring these conflicts as they can manifest into bugs that might be difficult to trace back to their source.
Understanding how to achieve upstream dependency resolution correctly is vital for maintaining an effective dependency management strategy. The factors above, give a strong strategic approach which in conjunction with the best practice guidelines provided in the official npm documentation will give you a strong foundation to handle any upstream dependency conflicts encountered when installing npm packages.
Efficient Strategies for Resolving NpM Package Dependence Issues
Resolving npm package dependency issues can be a daunting task even for experienced JavaScript developers. Those error messages about “upstream dependency conflicts” can suggest serious problems, but armed with the correct strategies, you can navigate your way through them and keep your project moving.
Understanding the Conflict
The term “upstream dependency conflict” in npm is used when two or more packages depend on the same package but require different versions. For example:
package A -> requires package C@1.0.0
package B -> requires package C@2.0.0
Here, both `package A` and `package B` are dependent on `package C`, but they require different versions of it. This is an issue because npm cannot install two mutually exclusive versions of the same package concurrently for a single project.
Strategies to Resolve
There are several methods to approach this issue:
Determine the Necessity of All Packages:
Evaluate all your dependencies: Are they all necessary? If not, remove the unnecessary packages causing conflicts. They could have been installed by accident or are no longer needed in your project.
Upgrade or Downgrade your Packages:
You need to install a version that satisfies all of the packages requiring it. In our previous example, if `package A` can also work with C@2.0.0, upgrading package A’s dependency to use C@2.0.0 will solve the problem. The reverse can be true; possibly, both packages could use an older version of `package C`.
npm uninstall packageA && npm install packageA@olderVersion
This method, however, may introduce other compatibility issues depending on the differences between the package versions.
Use of npm-force-resolutions:
[npm-force-resolutions](https://www.npmjs.com/package/npm-force-resolutions) is a utility that allows resolution of dependencies to be specified manually in your package.json file.
Add the following scripts to your package.json:
"scripts": { "preinstall": "npx npm-force-resolutions" }
Then, you can define the resolutions field. This field specifies which version of a package will be used when multiple versions are involved.
"resolutions": { "package-to-be-resolved": "version" }
Isolating the Packages:
As a last resort, if your application structure permits, you can try to separate parts of your app into different projects, thereby isolating the dependencies causing conflict.
These strategies provide a way to resolve upstream dependency conflicts in npm packages. However, upstream dependency conflicts indicates structural issues in the project that may require addressing for a long-term solution.
“To iterate is human, to recurse divine.” – L. Peter Deutsch, a pioneer in software design and programming. It’s a reminder that solutions often require us to think out of the box or even’s divert from the current situation, draw back, and then seek an ultimate solution.
In-depth Case Studies on Successful Management of Upstream Dependency Conflict
To delve into the upstream dependency conflicts that often occur while installing npm packages, we must first understand what “upstream dependencies” are. In the realm of Node.js and npm libraries, when a package (A) relies on another package (B) to function properly, we say that A has a dependency on B. Now imagine a scenario where two packages, Package C and Package D, both depend on the same Package B but require different versions of it. This is where we encounter an upstream dependency conflict.
Handling such conflicts can be quite challenging and success involves thoughtful management of these dependencies. For this discussion, let’s inspect two significant case studies:
Case Study 1: Netflix
Netflix is globally known for its robust technology infrastructure. It was reported that they faced challenges linked to complex dependency trees in their system. They developed the Newt tool to decrease friction amid engineers arising from issues like version inconsistencies among dependencies.
Newt Tool Operation: |
---|
1. Handles multiple versions of the same dependency through recognizing common patterns |
2. Collaborates with all other tools in an engineer’s stack |
3. Generates concise logs to assist quick debugging |
Through continuous iteration and feedback, they have been able to keep their system streamlined and efficient, minimising downstream effects of handling upstream dependencies.
Reference: Netflix tech-blog
Case Study 2: LinkedIn
LinkedIn, a renowned professional networking platform, suffered severe dependency issues when they experienced rapid growth. To address this, they used npm audit and npm outdated to identify vulnerabilities and outdated packages.
Approach: |
---|
1. They started with synchronizing their package versions |
2. Then, they committed to maintaining the latest version of their dependencies across all services |
3. They also created a weekly report on older libraries and accelerated upgrade efforts. |
These approaches helped in bringing about a significant reduction in upstream dependency conflicts.
Reference: LinkedIn Engineering Blog
Applying solutions from case studies like Netflix and LinkedIn can minimize potential friction caused by the upstream dependency conflict during the installation of npm packages. Johann Wolfgang von Goethe rightly said, “Knowing is not enough; we must apply. Willing is not enough; we must do.” This rings especially true in managing dependency conflicts where application of knowledge is key.
Dealing With Upstream Dependency Conflicts in NPM Packages
Fixing upstream dependency conflicts while installing Node Package Manager (NPM) packages can often seem like a complex task, but in reality, it’s an integral part of efficient JavaScript development. These conflicts typically occur when multiple dependencies rely on different versions of the same package. It’s a common scenario that developers experience when working with extensive projects that include numerous dependant packages.
npm
To tackle this issue effectively, here are a set of practices that we, JavaScript developers, typically adhere to:
- Leveraging npm audit fix command: This automated fixing tool resolves the conflicting versions of dependent packages. - Utilizing npm dedupe command: It helps in simplifying the overall structure and reduces redundancy by eliminating duplicate packages. - Resorting to npm shrinkwrap or npm package-lock: By generating a lock file that specifies exact versions of each dependency tree, you can ease out the conflict situation significantly.
In essence, as NodeJS developers, it remains imperative for us to fully understand how dependencies actually work and practice due diligence while maintaining our node modules. Remember what Linus Torvalds said, “Talk is cheap. Show me the code.”