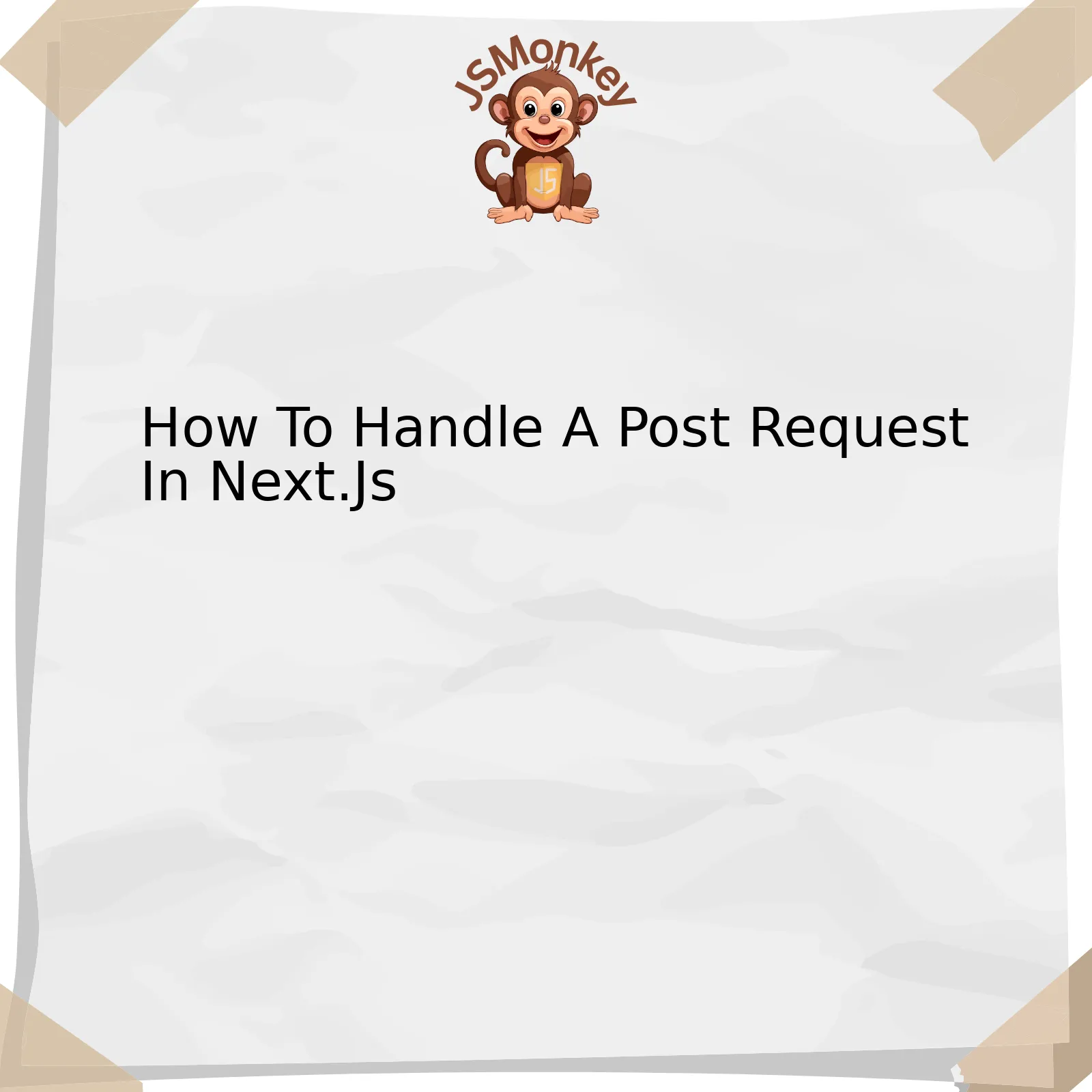
To handle a POST request in Next.js, the first thing you need to do is set up an API endpoint. This is where your application will receive and process POST requests.
The following table explains the general steps:
Step | Action |
---|---|
Endpoint Creation | Create a new file inside the ‘pages/api’ directory. The file’s path relative to ‘pages/api’ becomes the API route. |
Request Handler | The file should export a default function, which serves as the request handler. This function takes in two arguments: request (req) and response (res), both of which are objects that contain information about incoming and outgoing HTTP requests. |
Method Verification | To handle a POST request specifically, validate the HTTP method on the ‘req’ object using ‘req.method’ property. If the method is not ‘POST’, send an appropriate response back to the client. |
Data Extraction | If it’s a POST request, the payload or body of the request can be parsed using ‘await req.json()’ and then any required logic can be performed with the extracted data. |
Response Send | After processing, the server can respond back to the client by calling ‘res.status().json()’, setting the status code and sending a JSON response. |
// Inside pages/api/your-end-point.js export default async function handler(req, res) { if(req.method !== 'POST') { return res.status(405).end('Method not allowed'); } const { key, value } = await req.json(); // Do something with the key and value res.status(200).json({ message: 'Success' }); }
In this code snippet, we first check if the HTTP method associated with the request is ‘POST’. If it’s not, we send back a status of 405 (Method Not Allowed). If it is a POST request, we parse the incoming data using `await req.json()`.
This creates an object containing the payload (in this case, `key` and `value`), which then can be manipulated as per the business logic. Finally, a success message is sent back to the client using `res.status(200).json()`.
As Ryan Florence once said about modern web development techniques, “Whether you realize it or not, as developers, we’re life-long learners who thrive on stretching our capability.” Adapting to utilize Next.js API functionality enables us to enhance the interactivity of our applications by effectively handling various types of HTTP requests, thereby further honing our skills in creating robust and dynamic server-side rendered applications. [Nextjs Official Post Requests Documentation](https://nextjs.org/docs/api-routes/introduction)
Understanding the Basics of POST Request in Next.Js
Understanding and handling POST requests in Next.js is fundamental for developers working with server-side rendering and APIs. The essentials of managing POST requests include comprehension of HTTP methods, the structure of POST requests, and the appropriate coding approach for handling these events.
Comprehending HTTP Methods
HTTP verbs such as GET, POST, HEAD, PUT, DELETE, TRACE, OPTIONS, CONNECT and PATCH communicate intended actions to be performed on the specified resource. A POST request is utilized to send data to the server to create a new resource. The server processes the data passed in the body of the request and creates a new object as per the demand.
“Simplicity is prerequisite for reliability.”
Edsger W. Dijkstra
Data Transportation via Body in a POST Request
Unlike GET requests, which append data to the URL, POST requests send data through the body of the HTTP request. This feature of POST requests offers two significant merits:
- Data size: There are no size limitations; hence substantial amount of data can be transported.
- Data type: Apart from ASCII characters, binary data can also be sent which allows files and images to be transmitted.
Handling POST Requests in Next.js
In Next.js, we use API Routes for handling incoming HTTP requests including POST. The process entails writing an async function in a file under the /pages/api directory in your project root. This function then receives the incoming POST request and processes it.
To illustrate, here’s a simple example:
export default async function handler(request, response) { if (request.method === 'POST') { // Code here to process POST request } else { // For other request methods, return 405 - Method Not Allowed response.status(405).end(); } }
In this example, we are checking if the HTTP request method matches ‘POST’. If true, coding logic to process the POST request is inserted. But when the request method isn’t ‘POST’, our API function returns a status code of 405 indicating “Method Not Allowed”.
Using Body Parsing Middleware
Next.js automatically integrates middleware for body parsing. This means it parses incoming requests with JSON payloads and is based on body-parser – allowing you to access the payload via `request.body`.
Let’s review a simple scenario where data received through a POST request is processed:
export default async function handler(request, response) { if (request.method === 'POST') { const { username, password } = request.body; // destructuring data from request body // Process data... } else { response.status(405).end(); } }
In this snippet, properties `username` and `password` have been destructured from the request body, which can then be used as required.
Handling POST requests in Next.js requires understanding of how server-side processing works, but once you get the hang of it, you can build robust APIs that handle complex data transactions. Keep operability, simplicity, and reliability at the forefront while designing your APIs – just like Dijkstra suggested.
Implementing API Routes for Handling POST Requests
API Routes in Next.js provides a solution for creating API endpoints within your Next.js application. This feature gives you the ability to add server-side functionalities, like handling POST requests. To implement an API Route that handles a POST request, you create a file inside the `pages/api` directory of your Next.js application which will respond to HTTP POST methods instead of the default GET method.
When carrying a POST operation, you generally send data from the client side to the server side. In order to fetch this data on the server side, we utilize the `req.body` object in Next.js. Here is an illustrative example:
HTML
export default function handler(req, res) { if (req.method === 'POST') { // Process a POST request let { title, body } = req.body; // Process the data... res.status(200).json({ message: 'Success' }); } else { // Handle any other HTTP method res.status(405).end(); // Method Not Allowed } }
In this code snippet, we are exporting a default function called `handler`. This function checks if the incoming HTTP request is a POST request using `req.method === ‘POST’`. If it’s a POST request, we pick out the data attached with the request in the `req.body` and process it. Once done, we send a response back to the client with a `200 OK` status and a JSON object `{ message: ‘Success’ }`.
If the HTTP request method isn’t POST, we returning a 405 status code indicating that requested HTTP method is not supported by the server.
This is how you implement an API Route for handling POST requests in a Next.js application. Remember to cater for all other HTTP Methods to ensure a robust, and error-free application.
As Node.js creator Ryan Dahl once stated: “Doing things the right way matters. It isn’t simply about getting a functional product out, it’s about building one that can grow and evolve”. Applying this to our current topic, handling each type of request appropriately contributes to building a maintainable and scalable API within your Next.js app.
Error Handling and Validation Techniques for POST Requests in Next.js
The act of handling POST requests effectively in Next.js involves a series of explicit steps executed to ensure that all data coming into your application through these requests are valid and expected. Consequently, error handling is an integral part of this entire process, serving as both a proactive and reactive measure against negatives outcomes resulting from invalid or malicious data.
Targeted towards developers familiar with JavaScript and the Next.js framework, here’s a comprehensive rundown on tackling POST requests, with a specific concentration on error handling and validation techniques:
Integrated Middlewares:
Middlewares offer amazing capabilities for performing operations such as parsing incoming request bodies, facilitating session management, and most importantly, validating request data before it even reaches your route handlers. Specifically, in Next.js, you can utilize custom middlewares or choose from a vast selection offered by libraries like express-validator, which come pre-packaged with functionalities for checking data types, sanitizing inputs, etc.
Error Handlers:
Next.js allows you to implement global or local error handlers tailored to cushion the potential impact of unexpected scenarios related to processing POST requests. For example, if a runtime exception happens during the execution of your request handler, Next.js automatically sends a status code 500 response back to the client, allowing you to handle this scenario manually with a custom error page.
Body-Parsing:
Parsing the body of the POST request is a standard procedure in HTTP request handling. Invalid or malformed requests can be immediately flagged, using the built-in JSON middleware in Next.js. This way, you get to analyze the payload as a JSON object inherently bundled with the request object, raising errors when required.
Here, consider a general pattern of capturing errors in a Next.js application:
export default function handler(req, res) { try { // hypothetical validation checks if (req.method !== 'POST') throw new Error('Invalid request method') if (!req.body.name) throw new Error('Missing name field') res.status(200).json({ message: 'Successful POST request' }) } catch (error) { res.status(400).json({ error: error.message }) } }
The above function illustrates a Next.js API route handling function, where different validations occur prior to the core business logic. This approach emphasizes dependency on JavaScript’s try/catch blocks for exception handling and manual error throwing where there might be validation issues.
Also worth noting is a quote from Steve McConnell, a well-known software engineer, emphasizing that “Good code is its own best documentation,” hence, strive for creating robust yet understandable validation and error handling structures in your Next.js apps. The long-term maintainability of your app will surely appreciate this effort.
Client-Side Validations:
While server-side validations are crucial, adding client-side checks can greatly optimize request processing by preventing invalid or unnecessary requests from being sent in the first place. Libraries such as react-hook-form or formik provide control components to accomplish intricate checks before the form data journeys towards the server.
Implementing these structures described above, developers get to avoid an extensive range of problems surrounding HTTP POST requests. Nonetheless, remember to employ suitable strategies aligning with project specifications, standards, and complexities.
Relevant resources to look into include the [Next.js API Routes documentation](https://nextjs.org/docs/api-routes/introduction) for understanding the fundamental underlying concepts involved.
Advanced Concepts: Enhancing Functionality with Middleware in Post Requests
Middleware is a powerful and flexible mechanism that you can employ to add functionality to your HTTP POST requests processing within Next.js. They provide excellent features to handle important aspects of applications, such as cookies manipulation, body parsing for form submissions, logging, and more.
At its core, middleware stands between the client application making the request and the Next.js server handling the response. The middleware effectively acts as an intermediary, having the ability to process the request before it hits your main server logic or even modify the response on its way back to the client.
To better understand, let’s break down the introduction of middleware’s role in handling a POST request in Next.js:
– **POST Request Handling:** When a client sends a POST request, the server is expected to receive and process the data being sent. This may include form data, JSON, or even files. Conventionally, this would be carried out directly by the main server function in Next.js.
However, with the introduction of middleware, you can offload some of these responsibilities. For example, instead of writing repetitive code to parse form data or JSON in various parts of your application, you can use a middleware to take care of this.
Here is a simple example of how to incorporate middleware in handling a POST request in Next.js:
html
import nextConnect from ‘next-connect’;
const handler = nextConnect();
handler.use(express.json())
.post((req, res) => {
const { name, email } = req.body;
// Do something with name and email
res.json({ status: ‘success’ });
});
In the above code,
express.json()
is added as a middleware using the .use method of the handler returned by nextConnect(). This middleware takes care of parsing the body of incoming POST requests if they have a JSON payload.
– **Middleware Enhancement:** It offers opportunities to enhance functionalities beyond just parsing requests. For instance, you can collect metrics on the request/response cycle times, control access by checking authentication status or user roles, handle cookies, log the incoming requests for debugging purposes, and more.
So, as essence:
Middleware offers capabilities to extend and customize server-side processing in Next.js. It empowers developers to modularize their code, making it easier to reason about and maintain over time. By delegating responsibilities to middleware, one creates a more efficient and effective structure of handling POST requests.
Also noteworthy is this quote from Eric Elliot, a renowned JavaScript expert:
“Any application that can be written in JavaScript, will eventually be written in JavaScript.”
This suggests the strength and growing popularity of JavaScript (and Next.js), which further emphasizes the crucial role middleware plays in maximizing your application’s functionality and versatility.
Writing efficient, reliable server-side applications with Next.Js often involves dealing with POST requests. This can be achieved in various ways within Next.js that ensure your application receives and handles data effortlessly and effectively.
Incorporating
Next.js API routes
, you have a built-in feature to simplify managing POST requests. Constructing an API endpoint in your Next.js application, you easily interact with POST requests by defining a function within the request handler. The
req.method
is utilized to determine the HTTP method – whether it’s GET, POST, or any other HTTP verb.
Dealing with POST request data comes next. Reaching for libraries like
body-parser
might seem like a standard way to parse data from a request body. However, with Next.js, it’s less complicated using
req.body; a parsed version of the incoming request data is readily available. To handle forms, Front-end components will make use of thefetchmethod to send a POST request, passing on user form data to your API endpoint.
Here at Next.js, it's clear that focusing on developer experience has resulted in features that provide seamless handling of tasks such as setting status codes, easy parsing of JSON content among others - making the job of dealing with POST requests more enjoyable than ever before.
As Tim O'Reilly, founder of O'Reilly Media, once remarked, "We're entering a new world in which data may be more important than software." . In this regard, efficiently handling POST requests and managing the associated data becomes immensely crucial in modern web development practices, and Next.js proves to be a pertinent tool in achieving this feat.
You can find more about handling Post Requests In Next.Js in its official documentation.
Remember, the handling of POST requests in Next.Js is straightforward and efficient, largely due to the design intentions of the Framework. So, delve deep into this practice, constantly refine your skills, and ensure that you employ the best practices as often as possible.