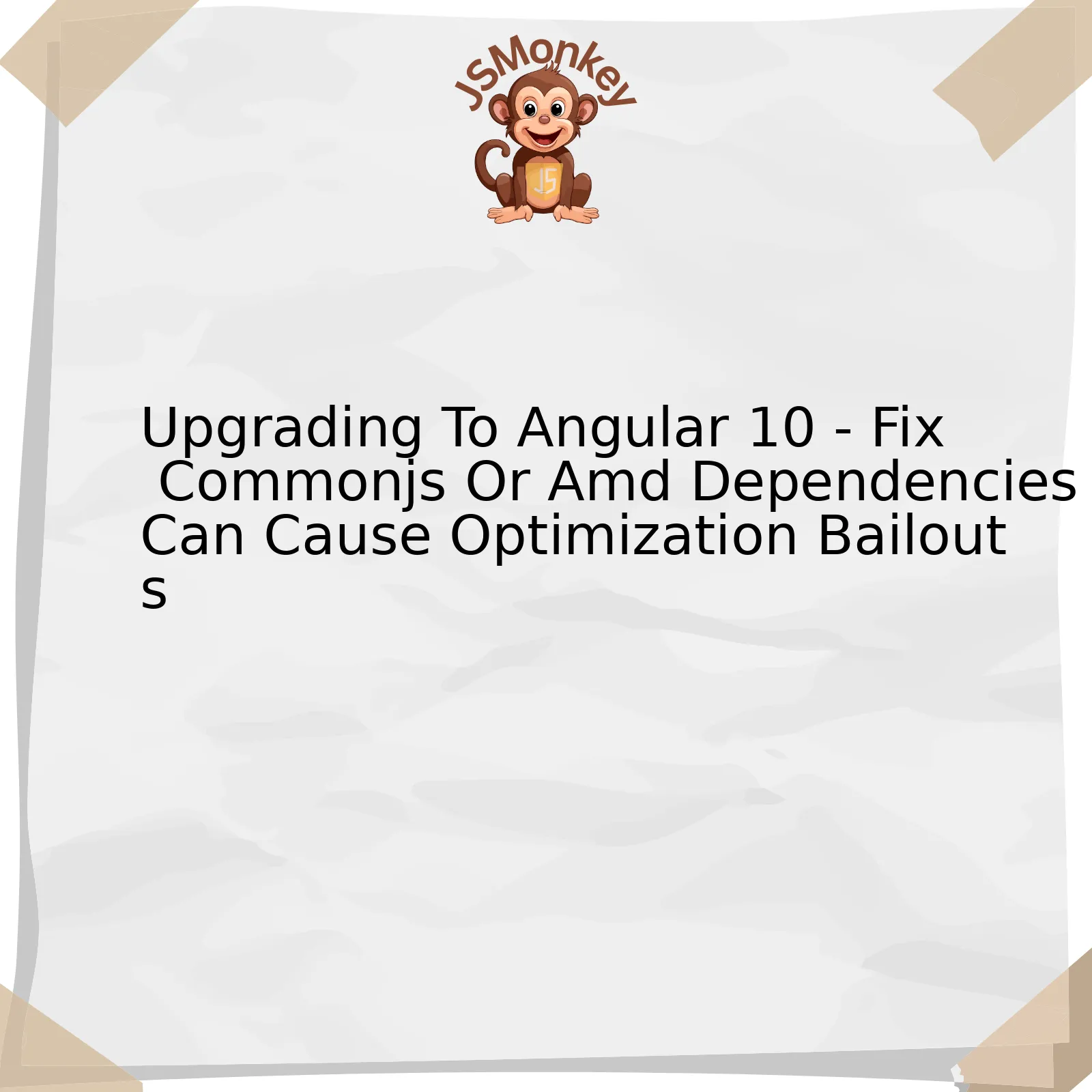
Issue |
Solution |
---|---|
Dependencies in CommonJS or AMD format cause optimization bailouts |
|
When you migrate to Angular 10, you may encounter a warning related to dependencies that use the Commonjs or AMD formats causing an optimization bailout in the development console. This is because Angular now has an improved check for CommonJS or AMD dependencies, a change that was instituted to inform developers about the potential hazards linked with using these dependencies.
While CommonJS and AMD are widely used module systems in JavaScript, they may not be suitable for client-side web applications as they have a challenge with static code analysis which is critical for tree-shaking and creating smaller bundles. ES2015 modules, on the other hand, are statically analyzable, allowing better tree shaking and smaller application bundles.
The table illustrates this phenomenon distinguishing between the issue at hand and the proposed solutions. It stipulates that when faced with the issue, key solutions include replacing these libraries with ES2015 (also known as ES6) modules, making changes in the tsconfig.json files to use ES2015 module configuration, and enabling Ahead-of-Time (AOT) Compilation.
As John Papa, a well-known figure on Angular technology, once said: “The process is not about making code perfect, it’s about enhancing its readability and maintainability”. Indeed, by adopting ES2015 modules instead of CommonJS or AMD ones, we fortify our application’s performance, readability, and maintainability.
For further reading on this topic Angular official guide provides an extensive approach. It’s vital to dive deeper into the intricacies of optimizing Angular applications for better performance with smaller bundles.
Understanding Optimization Bailouts in Angular 10
When delving into the intricacies of Angular 10, a key area of interest for many developers revolve around understanding Optimization Bailouts. These are extremely pertinent when one is upgrading from an older version to Angular 10 and confronting issues regarding CommonJS or AMD dependencies.
Angular offers a remarkably high performance by complementing JavaScript engine optimization through tree-shaking, a technique that eliminates unused segments of code. However, certain programming practices can hamper this process, thereby prompting optimization bailouts.
Concisely, an optimization bailout happens when Angular concludes that a particular segment of your application’s code cannot be adequately optimized. As a result, it forgoes tree-shaking for that part, leading to possibly larger bundles. Elements like CommonJS or AMD modules play a role in sometimes triggering these bailouts.
import { something } from 'commonjs-package'; // Warning: CommonJS or AMD dependencies can cause optimization bailouts...
When you upgrade to Angular 10 with a warning about CommonJS or AMD dependencies causing optimization bailouts, it’s crucial to comprehend that Angular recommends using ECMAScript modules over CommonJS or AMD. It’s because ECMAScript supports static imports which aligns better with tree-shaking.
Troubleshooting such issues involves two steps:
The first step involves locating dependencies containing CommonJS or AMD modules within your codebase. This task can be made easier by leveraging tools like are-you-es5.
After identifying the problematic dependencies:
- Consider whether they are essential and could be replaced with alternatives packaged as ECMAScript modules.
- If replacement isn’t a viable option, reach out to the package maintainer and inquire about an ESM version.
Obstacles such as optimization bailouts can initially seem intimidating. Nonetheless, with a proper understanding of their causes, primarily the use of CommonJS or AMD dependencies, you can confidently navigate through these challenges when upgrading to Angular 10.
“The function of good software is to make the complex appear to be simple.” – Grady Booch
Remember that each challenge encountered in coding is an opportunity for growth and mastering your craft. Let this guide you as you tackle Optimization Bailouts in Angular 10.
Addressing Commonjs/AMD Dependencies: An In-Depth Look
In the process of upgrading to Angular 10, developers often face a seemingly challenging task – dealing with CommonJS/AMD dependencies. As detailed by the official Angular guide, using such dependencies can cause optimization bailouts, resulting in compromised performance for your Angular application.
Understanding the Nature of the Issue
CommonJS and AMD are popular module systems that were extensively used prior to the introduction of ES6 modules. The issue at hand stems from Angular’s ability to perform ahead-of-time (AOT) compilation, which includes full tree-shaking processes to eliminate unused code and effectively reduce bundle sizes.
Should the Angular CLI detect CommonJS or AMD dependencies during build, it will trigger an optimization bailout as these older module systems do not support tree-shaking optimally. This generally results in larger bundle sizes leading to a slower initial loading time.
The Solution Path
To handle this situation, developers are advised to proceed with the following steps:
1. Identify the Dependencies
The first step towards fixing the problem is recognizing the offending dependencies. Thankfully, Angular’s alert mechanism flags these upon detected during the building process. The console warning message outlines which dependencies are triggering the optimization bailouts.
2. Opt For ESM-Compatible Versions Or Alternatives
Once identified, check if the package highlighted has an ECMAScript Module (ESM) format counterpart. Using ESM versions allows for more effective tree shaking thereby mitigating the optimization bailout.
If ESM versions aren’t available, consider seeking alternate libraries that support ESM formats. This change could have some development impact, thus risk assessment before making this switch is crucial.
3. Supplier Relationship Management (SRM)
In some cases, migration might be infeasible due to the absence of alternate ESM-compatible libraries or operational constraints. Here, reaching out to the offending package’s maintainer or contributing to the package could potentially solve the issue.
To illustrate, let’s consider a pseudo Angular 9 application with the following dependency:
import * as _ from 'lodash';
During build, this raises a warning “Using lodash in CommonJS format can cause optimization bailouts”. In an effort to mitigate this, opting for lodash-es, the library’s ESM version can help:
import _ from 'lodash-es';
Although the process seems straightforward, changing dependencies carries certain risks and should only be done after careful consideration. However, by executing such changes, developers will greatly enhance their application’s performance and ensure effective adherence to Angular 10’s recommended practices.
As Jeff Atwood, co-founder of Stack Overflow, once said: “There are few sources of energy so powerful as a procrastinating college student.” Similar is the case with developers and delayed code optimization – embracing the challenge now saves much time and effort down the line.
Strategies for Upgrading to Angular 10 and Avoiding Optimization Bailout
For many JavaScript developers, upgrading to Angular 10 can be a challenging endeavor; but with strategic insights and understanding the common pitfalls, the optimization bailouts due to CommonJS or AMD dependencies can be effectively managed.
The first step in your upgrade strategy should focus on analyzing your current Angular application and its dependencies. This can be accomplished by studying how dependencies are imported and used throughout your application. Direct import statements from CommonJS modules can cause significant performance problems due to their inability to utilize Tree-Shaking—an optimization technique offered by modern libraries.(Webpack)
// Avoid doing this. import { map } from 'rxjs/operators';
Instead of importing directly, switching to ECMAScript (ES) Modules would be beneficial. They have built-in static structures that effectively support Tree-Shaking.
// Do this instead. import { map } from 'rxjs/operator/map';
To assist in uncovering these issues, use the command ng build — prod while paying close attention to warning messages about your imports causing optimization bailouts. These warning messages serve as an invaluable tool to pinpoint where you need to change your code.
Employing code splitting strategies is another method by which to reduce the optimization bailout impact. As opposed to loading the entire application at once, you can commit to using Angular’s lazy-loading feature to load components only when needed, resulting in improved performance.(Angular.io)
Additionally, the Angular team provides a ngcc configuration option, which can be added to “package.json”. In scenarios wherein phylogenetic packages throw optimization bailout warnings, it’s especially helpful.(Angular.io)
"ngccOptions": { "propertiesToConsider": ["es2015", "browser", "module", "main"] }
As Brad Green, Engineering Director at Google stated, “Angular gives power back to the developers. It gently guides you towards best practices and offers powerful tools to get work done.” By considering these strategies when migrating applications towards Angular 10, developers can improve overall application performance and make efficient use of the plethora of features available within Angular framework.
Exploring the Effects of CommonJS or AMD Dependencies on Angular 10 Optimization
As we dive deeper into understanding the effects of CommonJS or AMD dependencies on Angular 10 Optimization, it’s imperative that we establish the relevance of this concept to our discourse – “Upgrading To Angular 10 – Fix Commonjs Or Amd Dependencies Can Cause Optimization Bailouts”.
With the advent of Angular 10, developers started receiving a warning during the build time whenever they imported a dependency packaged using CommonJS or AMD. This is due to the fact that these non-ECMAScript (ES Module) dependencies can lead to sub-optimal bundling, potentially increasing the size of payload that ends up being sent to the client.
To illustrate this, consider an instance where you have imported a library packed with CommonJS in your Angular project. While modern JavaScript module systems allow for tree-shaking to remove unused code to optimize the final bundle, dependencies packaged with CommonJS aren’t fully tree-shakable. As a result, even if you use only one function from a huge library, the entire library will end up in your production bundle, leading to poor optimization and performance issues.
// import from a CommonJS packaged library // The full library will add to the bundle regardless of the used parts. import * as _ from 'lodash';
Angular 10 now warns against this potential risk ahead of time allowing you to switch to an ES Modules alternative as such:
// import only necessary function from lodash // optimized bundling due to the ES modules import cloneDeep from 'lodash/cloneDeep';
Finding an ES Module alternative might not always be feasible or available. In such scenarios, you could consider these two broad approaches to better handle the situation:
* Use Webpack’s IgnorePlugin to completely exclude bulky CommonJS modules from your bundles.
* Consider using Babel Plugin Transform Runtime to optimize CommonJS dependencies.
Robin Wieruch, a German Software Engineer, perfectly summed it up when he said, “In an era where performance is key, tree-shaking is a necessary step towards optimizing web applications.” While Angular’s optimizations are undoubtedly striving to ensure peak application performance, it’s the responsibility of developers to align their practices with these enhancements. As we aspire to upgrade to Angular 10 and beyond, let’s continue embracing ES Modules over AMD or CommonJS dependencies for improved bundling and better performing end-products.
Optimizing your Angular applications is paramount in ensuring efficient performance and a seamless user interface; hence the heightened attention on overcoming the CommonJS or AMD dependencies issue that might lead to optimization bailouts during an upgrade to Angular 10.
Comprehending that encountering the warning notifications during the build process is indicative of potential likelihoods that might discourage achieving an optimally bundled package, it’s necessary to underline the influence pertaining to the size of your application bundle and, correspondingly, your application’s performance.
To mitigate this, there exists a series of approaches:
– Strive for ESM2015 (or higher) equivalents of dependencies by using
import ... from 'dependency';
– Refactoring the project into Angular Package Format (APF), which requires native ECMAScript modules.
– Setting aside development modes leverages tree-shaking, consequently allowing you to impede these dependencies from being a part of your final bundle.
All these procedures factor into arming you with the requisite tools to combat such underlying issues succinctly while invariably enhancing your Angular application’s efficiency post-upgrade.
Bill Gates once said, “I choose a lazy person to do a hard job. Because a lazy person will find an easy way to do it.” Cleverly resolving the CommonJS or AMD dependencies’ challenge harmonizes with that sentiment as it necessitates streamlining builds for optimal efficiency.
The peripheral landscape revolving around upgrading to Angular 10 has its fair share of twists and turns, yet surmounting the CommonJS or AMD dependencies renders palpable enhancement in terms of critical components including application speed, size, and overall performance.
For further insight on ambitiously embracing the upgrade to Angular 10, one can always refer to documents like the Angular Ivy guide. It provides a comprehensive understanding of the Angular architecture, thereby underscoring its inherent capabilities to construct highly efficient applications while shedding light on the premise for such warnings in your build process.