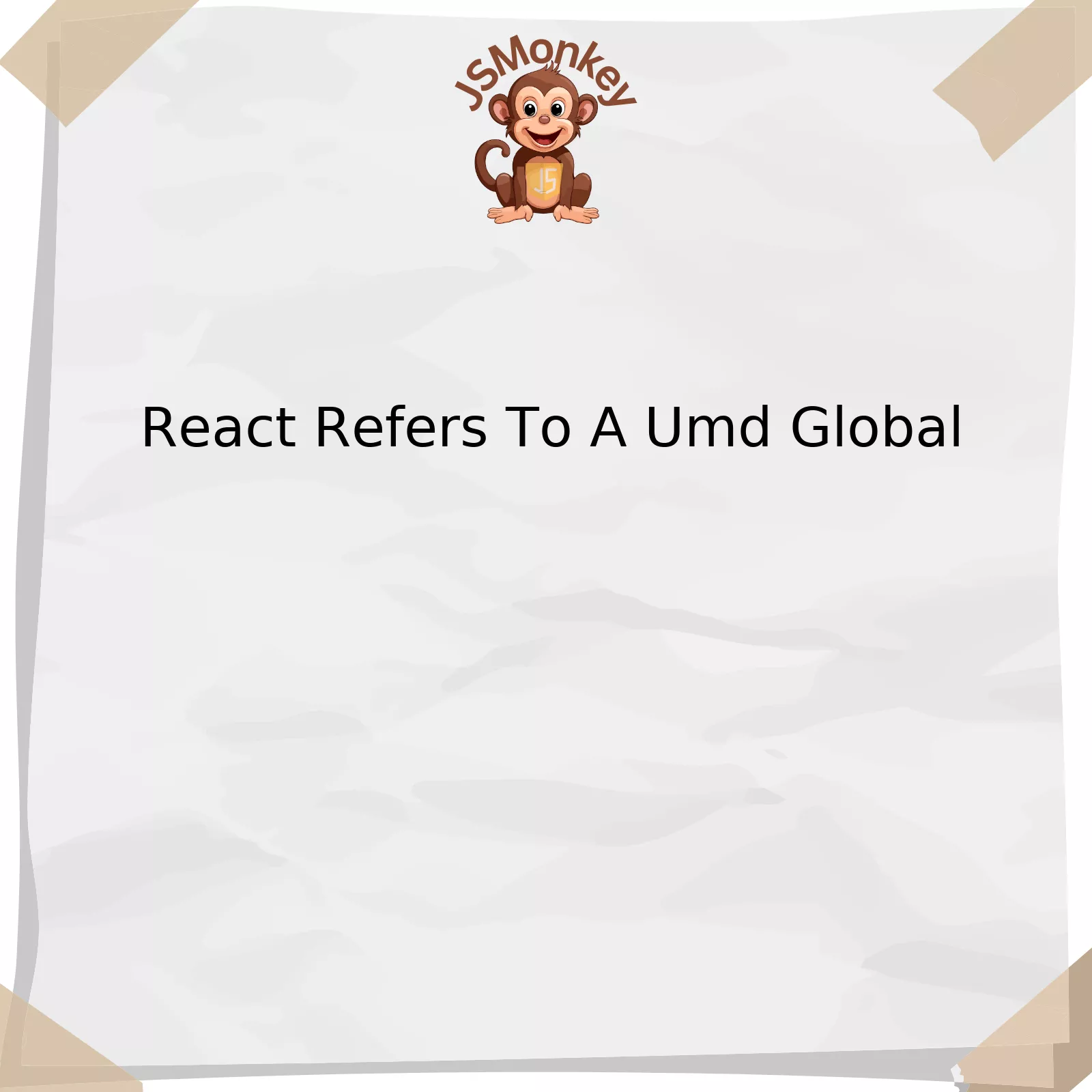
| Particulars | Description |
| ———– | ———– |
| Name | React refers to a UMD global |
| Definition | UMD (Universal Module Definition) pattern allows JavaScript modules to work in multiple environments like browser global variables, AMD (Asynchronous Module Definition), and CommonJS (used in Node.js). The UMD pattern enables these modules, such as React, to be accessible globally. |
| Purpose | The main aim is to make the library usable in different application environments, considering the JavaScript module systems aren’t universally supported yet. |
| In Context of React | When one loads React from a script tag, it refers to the UMD global since React makes itself compatible with UMD and allows itself to be consumed using different module patterns. |
| Importance | It ensures that JavaScript libraries are widely compatible and maintain proper functionality across various module systems.
To elaborate further on this principle; ‘React refers to a UMD global’ implies that when you load React via a script tag, rather than using a module bundler like Webpack or Babel, React operates under the UMD pattern. This means that whenever React is loaded through a script tag, whether in a client-side or server-side environment, it will function correctly because it has been designed to recognize and adapt to the way each environment handles and interfaces with JavaScript modules.
UMD globally refers to how JavaScript Libraries, such as React, are made universally available regardless of the module system employed by the host environment. This becomes crucial for library maintainability and usability, allowing it to function seamlessly in all its implementations. Ensuring this broad compatibility is integral to the development of libraries intended for general use within the JavaScript universe.
Thus, the procedural phrase ‘React refers to a UMD global’, encapsulates the methodology employed by libraries to maintain universal functionality and accessibility, irrespective of the host environment or the specific module handling syntax employed therein.
Understanding the Functionality of React as a UMD Global
React, when referred to as a UMD global, is implying the Universal Module Definition (UMD) pattern which is commonly used in JavaScript programming. The UMD pattern enables JavaScript modules to be consumed or loaded in several different ways such as with AMD, CommonJS module systems, or as a global variable.
When we talk about “React as a UMD Global,” it means we are referencing React from a script that’s included globally within our HTML file instead of installing and importing it as a module in our project. This global reference makes React available on the window object as window.React.
This setup is beneficial in script-included React environments and is an alternative way to use React. Yet, this approach is more common in legacy systems or small projects where you likely may not have the overhead of larger build processes or JavaScript bundles. It provides you the freedom to still use React’s features without having to integrate it as part of an imported module-based structure in your application sources.
However, this should not be confused with the best practice of modern JavaScript development. Using local imports allows for better scope management, bundling optimizations, and tree shaking which most of the time leads to better performance and cleaner code.
Implementing React in Universal Module Definition Projects
Universal Module Definition (UMD) is a type of Javascript module system that supports both traditional global variable linking and newer CommonJS or AMD-based modular systems. React itself can also be added to any project as a UMD module.
If you want to use React in UMD modules, first, you will have to include React into your project. It can be done by including links to the React and ReactDOM UMD libraries in your HTML file:
html
After this, React becomes available as a global object on the window namespace, i.e., `window.React` and `window.ReactDOM`.
You can now utilize React and ReactDOM in your Universal Module Definition Projects just like you would do with other global variables:
javascript
(function(global, factory) {
// Choose the correct global object based on environment (ex.browser or server)
let root = ((typeof self == ‘object’ && self.self == self && self) || (typeof global == ‘object’ && global.global == global && global));
// If both exports and module are present, define the UMD
if(typeof exports === ‘object’ && typeof module === ‘object’)
module.exports = factory(root[“React”], root[“ReactDOM”]); // Node Module System
else if(typeof define === ‘function’ && define.amd)
define([“React”, “ReactDOM”], factory); // Asynchronous Module Definition
else{
var a = typeof exports===’object’ ? factory(root[“React”], root[“ReactDOM”], root[“YourModule”]) :
factory(root[“React”], root[“ReactDOM”]);
root[“YourModule”] = a; } // Set to global variable
}(this, function(React, ReactDOM) {
return YourModule = (props) => {
return React.createElement(‘h1’, null, `Hello, ${props.name}`);
};
}));
In the above code, we are creating a UMD that depends on React and ReactDOM. These dependencies will be automatically resolved regardless of the environment — global variables in a browser, CommonJS modules in Node.js, or AMD modules with RequireJS.
Please remember to replace “YourModule” with the actual name for your module.
This would allow you to support various module loading scenarios, ensuring greater compatibility across different environments, all while leveraging the power of React.
Key practices for Utilizing React within UMD Globals
React, as a UMD (Universal Module Definition) Global, seems to be a bit confusing concept for beginners. Key practices for utilizing React within UMD Globals focus on the manner of using ReactJS in various environments including the browser, Node.JS, or other JavaScript environments due to its portable design.
Firstly, whenever one refers React as a UMD Global, it means that they are treating React as a module that can work on any JavaScript-supported platform, thanks to the Universal Module Definition pattern. It means that the same piece of code can be seamlessly executed on different client/server environments with minimal or no changes at all.
Secondly, React UMD builds make React available as a standalone.js file. They’re useful if you aren’t using a bundler (like Webpack, Parcel, or Rollup). To use a React UMD build, just script tag it into an HTML page along with the desired ReactDOM UMD build. The UMD builds make React accessible as a window.React and window.ReactDOM globals.
Thirdly, in order to make full use of this environment agnosticism offered by React UMD globals, one needs to fully understand how to import/export modules using both AMD (Asynchronous Module Definition) or CommonJS system. While AMD is more suitable for browsers due to its support for asynchronous behavior, CommonJS works well with server-side execution due to its synchronous loading method.
Lastly, keeping scope limited to single component files, defining less stateful components & splitting components into smaller sub-components can make the task of maintaining and updating components easier. This will ensure better enforceability and manageability of your React application when utilized with UMD Globals.
Remember, React’s ease of use doesn’t come from the fact that it’s tied to UMD (or AMD, or CommonJS), but rather from its inherent design principles i.e., creating complex UIs from simpler components, unidirectional data flow, and explicit state management.
Exploring Troubleshooting Tips for React and Universal Module Definitions
Universal Module Definition (UMD) is a common way of making JavaScript code compatible with various module systems, like AMD or CommonJS. It plays a crucial role in deploying React components that need to be accessible on different platforms or environments.
Now, when working with React and UMDs, you might sometimes run into troubleshooting issues related to the UMD Global. React refers to a UMD global when you include React in your project through a