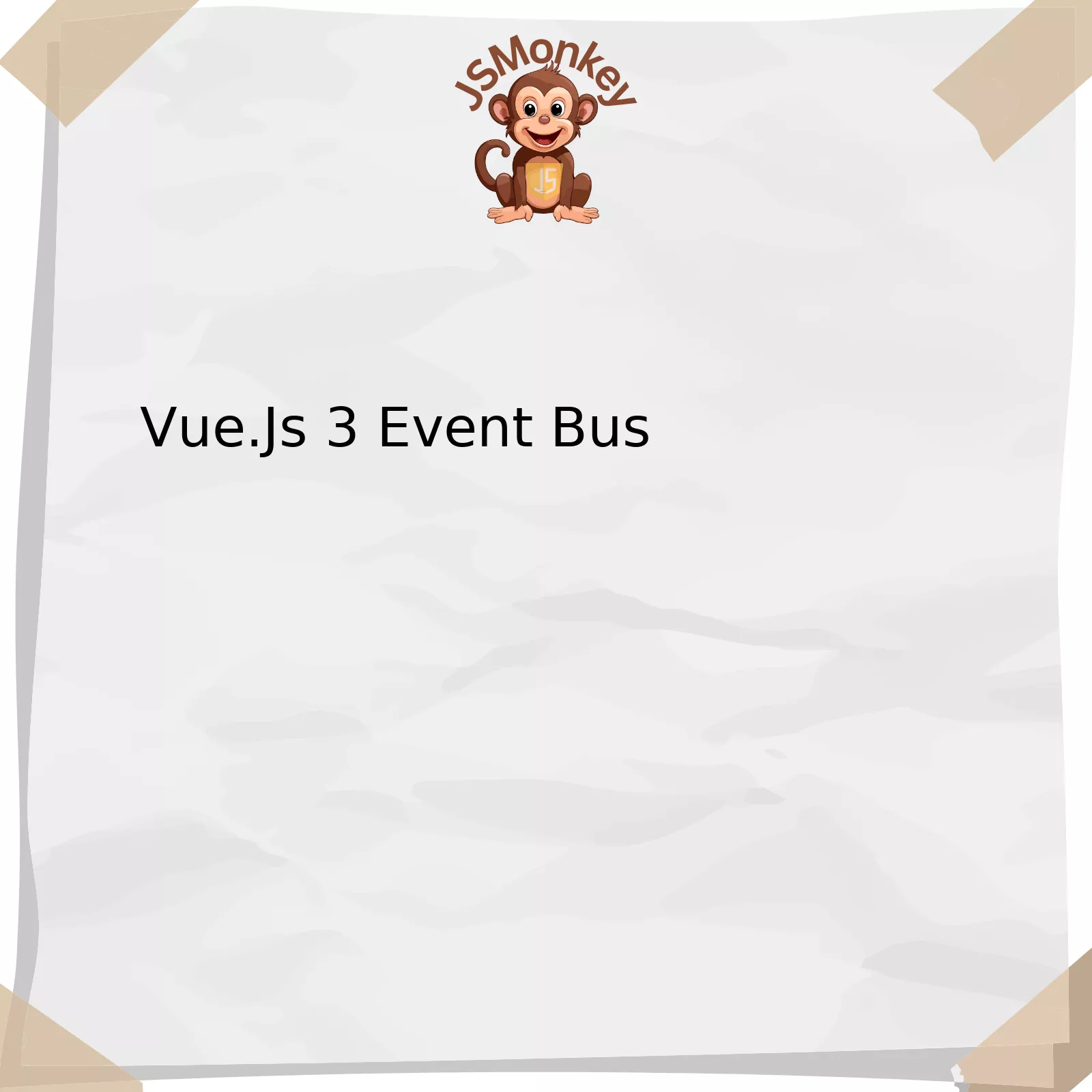
Vue.js is a progressive framework used for building user interfaces. One of its distinct features is the implementation of an event bus, which is an avenue for components in Vue.js to communicate with each other.
Feature | Description |
---|---|
Creation of Event Bus |
This involves creating a new instance of Vue specifically dedicated to handling events Vue.js Documentation. |
Emitting Events |
The process by which an event is broadcast on the event bus. This uses the
this.$bus.$emit('event') method and usually occurs within the component that generated the event. |
Listening to Events |
The act of catching or receiving transmitted events. This process employs the
this.$bus.$on('event') method, done in the component interested in the event. |
Removing Event Listeners |
Deleting registered event listeners from the event bus as they may lead to memory leaks if not properly handled. The method for this is
this.$bus.$off('event') . |
The use of an event bus in Vue.js 3 allows for decoupling of components, thus promoting code reusability and maintainability. It gives you better control over your application state and events, by ensuring every part is notified of relevant changes.
However, just like any other tool, the event bus can be destructive if not used properly. Broadcasting too many events can lead to a crowding of the bus, making debugging more difficult. Also, if components are overly reliant on the event bus for communication, it might create a complex web of dependencies. This intricacy may make updating individual components more difficult.
“Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” – Martin Fowler
This quote captures the essence of how to properly employ an event bus in Vue.js 3 for building interactive user interfaces. It should be used cautiously and efficiently, with an emphasis on creating understandable, clean, and modular code.
It is also advised to couple the use of Vue.js’s event bus system with its robust reactive data handling capabilities. This way you can adhere to the principle of ‘single source of truth’, where your application’s state is kept consistent across all components.
Use of Vuex, a state management pattern + library for Vue.js applications, is recommended for larger scale applications Vuex Documentation. It serves as a centralized store for all the components in an application, ensuring that the state can only be mutated predictably.
By leveraging Vuex with the event bus, successfully programming large-scale applications using Vue.js becomes an easier feat. This ties back into Fowler’s concept of writing code that not only machines can understand but humans too. You’ll enable better collaboration among developers and ensure that new team members can effectively understand and contribute to the project.
Utilizing Vue.js 3 Event Bus for Effective Communication
Vue.js 3 Event Bus encapsulates a unique approach for component communication in Vue.js applications. Primarily, it replaces the necessity of prop drilling or managing global state, thereby enhancing the ease of code management and overall programming efficiency.
Understanding Vue.js 3 Event Bus
The fundamental concept of Vue.js 3 Event Bus lies in creating an independent Vue instance, primarily used to emit events from one component and listening to them on another. It follows a publish-subscribe pattern(NOT an observable pattern), where various components within an application can broadcast (publish) events, and other components can receive (subscribe) and act on those events.
// Instance of Event Bus const EventBus = new Vue() // Component that emits event EventBus.$emit('eventEmitted', data) // Component that listens and acts upon the emitted event EventBus.$on('eventEmitted', processDataFunction)
The code snippet above reflects the basic representation of how the Event Bus mechanism would be implemented across different components. Here, ‘eventEmitted’ is the custom event being dispatched with some ‘data’. Additionally, the ‘processDataFunction’ is a method defined within the listener component, engaging in some action based on the received ‘data’.
Accessibility of Vue.js 3 Event Bus
Being a globally accessible instance, Vue.js 3 Event Bus can effectively handle communication between disconnected or nested Vue.js components without relying exclusively on Vuex state management library. This makes it an efficient toolset for developers when dealing with localized inter-component communication, which doesn’t justify the use of a centralized store.
Caveats of Vue.js 3 Event Bus
While the Event Bus method provides ease and structure into managing state across disparate components, it does come with certain caveats. Majorly, debugging could become a challenge as an application scales because the flow of data is harder to track compared to local state or via Vuex.
Pros | Cons |
---|---|
Eliminate prop-drilling complexity between nested/disconnected components | Debugging could be challenging in larger applications due to non-linear data flow |
Avoids over-reliance on Vuex for simple inter-component interaction | Lack of automated cleanup can lead to memory leaks |
Indeed, as Alligator.io appropriately states – “A properly placed event bus can save you a ton of headaches and keep your code clean.”
EPSILON: Adopting efficient coding practices and leveraging compact solutions like Vue.js 3 Event Bus is imperative to keep our programs maintainable and performance-centered. As quoted by Robert Glass, a renowned software engineer, “Measuring programming progress by lines of code is like measuring aircraft building progress by weight.”
Pros and Cons: A Closer Look at Vue.Js 3 Event Bus
When we analyze the Vue.js 3 Event Bus system, different advantages and disadvantages emerge related to its functionality, adaptability, and usability. Let’s take a detailed look to better understand the system’s pros and cons in order to gain a more complete perspective.
Pros of Vue.js 3 Event Bus
– Decoupled Communication: The event bus system allows components to communicate without direct parent-child relations. This promotes less coupled components, thereby enhancing the flexibility of your applications.
<br/>
– Data Transfer: Events can carry data when they are emitted. Data sent through an event bus is available to all listening parties, making it a convenient method for transferring data between non-parent-child components.
<br/>
– Clear Structure: With the event bus, the code structure becomes clearer and easier to maintain. It is possible to recognize at one glance where events are coming from and where they are going to.
<br/>
The pros mentioned above speak volumes about Vue.js 3 Event Bus system’s potential. However, like any other system, it also has its share of downsides.
Cons of Vue.js 3 Event Bus
– Increased Complexity: Using an event bus can increase the complexity of simple tasks as it’s tougher to track which component triggered an event and which ones responded to it.
<br/>
– Debugging Difficulty: Debugging can be much harder with the usage of an event bus, since issues aren’t always predictable. It may become increasingly difficult to figure out what’s going wrong in which part of the code.
<br/>
– Potential for Chaotic Situations: Without disciplined usage, an event bus can quickly contribute to a chaotic situation. It might create troubles, especially in larger applications where events could accidentally be emitted and caught by unsuspecting components.
<br/>
The disadvantages listed above make it clear that there is room for improvement.
To highlight the essence of tech evolvement, here’s a fitting quote by Mark Zuckerberg: “Our philosophy is that we care about people first.”
Despite its challenges, Vue.js 3 Event Bus remains a powerful tool for managing cross-component communication efficiently if employed with care and discipline. The pros and cons vary depending on your project’s size, complexity, and specific requirements. Ultimately, it’s the delicate balance between these elements that will determine whether using Vue.js 3 Event Bus is the optimal choice for your project. For further information and deeper understanding, you may refer here.
Best Practices When Implementing Vue.Js 3 Event Bus
The Vue.js 3 Event Bus is a robust tool developers use to handle communications between components in a Vue.js application. Using the tool right demands some best practices, and we shall delve into these while remaining relevant to the Vue.js 3 Event Bus:
Avoid Overuse:
The beauty of the Vue.js Event Bus lies in its power and simplicity, but like all efficient tools, overuse could lead to complications. Therefore, use this feature sparingly to avoid confusion and cluttering your codebase. While the machine may not notice this complexity, human developers maintaining or working with your system will appreciate it.
User-Defined Events:
Custom events are crucial when using the Event Bus, as they simplify complex processes and improve readability significantly. However, you need to watch out to prevent overlaps and redefine standard event names inadvertently. Custom events should be clear enough to explain what they do self-evidently.
We can define our custom event like so:
bus.$emit('user-defined-event', payload)
.(vue.js docs)
Emit and Listen:
When you emit an event (bus.$emit), there must be a corresponding listener (bus.$on). Ensure you’ve always got matching emitters and listeners for every action on your bus.
The following example shows how to emit an event and how to listen to it:
Emitting:
bus.$emit('user-event')
Listening:
bus.$on('user-event', function () { // Execute action })
Cleanup Listeners:
Something often overlooked is the cleanup needed after a component has stopped listening to an event. Removing event listeners that no longer serve any purpose is crucial to maintain the application’s performance and prevent memory leaks. Use bus.$off to clean up listeners.
Example of removing an event listener:
bus.$off('user-event')
Use Middleware:
Not always, but in some cases, you may want to use middleware for your Event Bus logic. Middleware can allow you to execute pieces of code before passing the emitted event to its intended destination. This can be extremely useful for analytics, debugging, or other applications where additional logic might be necessary.
In the words of Douglas Crockford, a well-recognized figure in the world of JavaScript and JSON, “Programmers should take their design cues from the data, selecting the algorithm that most straightforwardly expresses that structure.” Understanding the data flow in your Vue.js 3 Event Bus system can significantly impact your choices when designing the event-based communication structure for your application. Making it easier for others to understand your system is one of the keys to successfully using complex tools such as the Vue.js 3 Event Bus.
Exploring Real World Applications of Vue.js 3 Event Bus
Vue.js 3 Event Bus is an interesting mechanism that allows different Vue components to communicate with each other, irrespective of their parent-child relationship. This broadly opens up practical and real-world applications, making the overall development process more streamlined, thereby enhancing performance and scalability. Let’s delve deeper into its applications in response to your query.
1. Building Complex Applications:
In real-world applications that comprise several nested components, prop drilling often leads to complex structures, resulting in a cumbersome codebase. Vue.js 3 Event Bus comes to the rescue by providing easy component communication. No longer are you required to pass on props from parent to child or raise events through each nested layer. A bus permits direct communication between non-parent/child components, lending greater performance and maintainability.
Take this simple example:
// Creating an EventBus instance const EventBus = new Vue();
This instance can then be used for firing events:
// Emitting event with data EventBus.$emit('event', data);
The corresponding action would look like this:
// Listening for the event EventBus.$on('event', function(data) { // Handle 'event' });
2. Micro-frontends Architecture:
Micro-frontends architecture has become the buzzword, promising developers the flexibility to introduce changes without causing disruption site-wide. Precisely, you can have multiple teams managing different parts of a web app independently. Here, Vue.js 3 Event Bus shines out brilliantly, facilitating seamless integration between the micro-frontends, essentially acting as a backbone for information transfer.
3. Enhancing User Experience:
The efficient dispatching and handling of events via the Event Bus enable you to deliver an enriched UX/UI. You get equipped to manage state transitions and handle UI updates effectively, thus serving up a smooth user interaction flow.
One of the architects of the modern web, Brendan Eich once quoted “Always bet on JavaScript”. With the rich capabilities that Vue.js 3 Event Bus brings to the table, it seems JavaScript and its libraries won’t cease to surprise us anytime soon.
For more in-depth knowledge of event bus system, I recommend checking the official Vue.js Guide [source].
4. Greater Testability:
Application testing becomes rather convenient with Event Buses, where one could simply listen for events fired and assert that components are behaving as expected. This significantly reduces complexities that may have otherwise spawned due to prop drilling or context passing, enhancing overall testability.
While these were some of the standout uses, the real-world potential for Vue.js 3 Event Bus is tremendous. By providing enhanced component communication, simplified state management, superior application architecture, and improved testability – Vue.js 3 Event Bus undeniably takes your web development efforts to the next level.
Vue.js 3 Event Bus is undeniably a significant advancement in modern web development. It offers a myriad of features that enhance the ability of developers to build highly reactive and dynamic applications. From application-level state management, decoupled communication between components, to even asynchrony handling, Vue.js 3 Event Bus streamlines the complexity of data flow in Vue applications.
A few key points worth noting about Vue.js 3 Event Bus include:
• Maintains Application State: The Event Bus provides an elegant way to maintain state across your Vue.js application, especially when prop drilling becomes strenuous. It eradicates the need to pass state via child components by providing a central repository.
// Creation of an event bus const eventBus = new Vue()
• Decouples Component Communication: In scenarios where non-parent-child components need to communicate, Vue.js 3 Event Bus serves as an intermediary, enabling such communication without tight coupling.
// Emitting an event eventBus.$emit('eventName') // Listening to an event eventBus.$on('eventName', functionToExecute)
• Handles Asynchrony: Unlike prop-passing, the Event Bus handles asynchronous data, meaning events can be listened for and emitted at any time, aligning with JavaScript’s non-blocking nature.
The Event Bus in Vue.js shifts paradigms on component interaction, establishing more fluid and adaptable means of state management and component communication. Its proper utilization contributes significantly to building robust, maintainable, and reactive applications, thus making Vue.js 3 a potent tool in the domain of front-end frameworks.
As Martin Fowler, a British software developer, once said,
> “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.”
In essence, Vue.js 3 Event Bus makes your code easier to understand, raising the quality of your programming.
For more technical insights on Vue.js 3 Event Bus, refer to the official Vue.js documentation. Remember, mastering a tool is different than just knowing it. Keep practicing and happy coding!