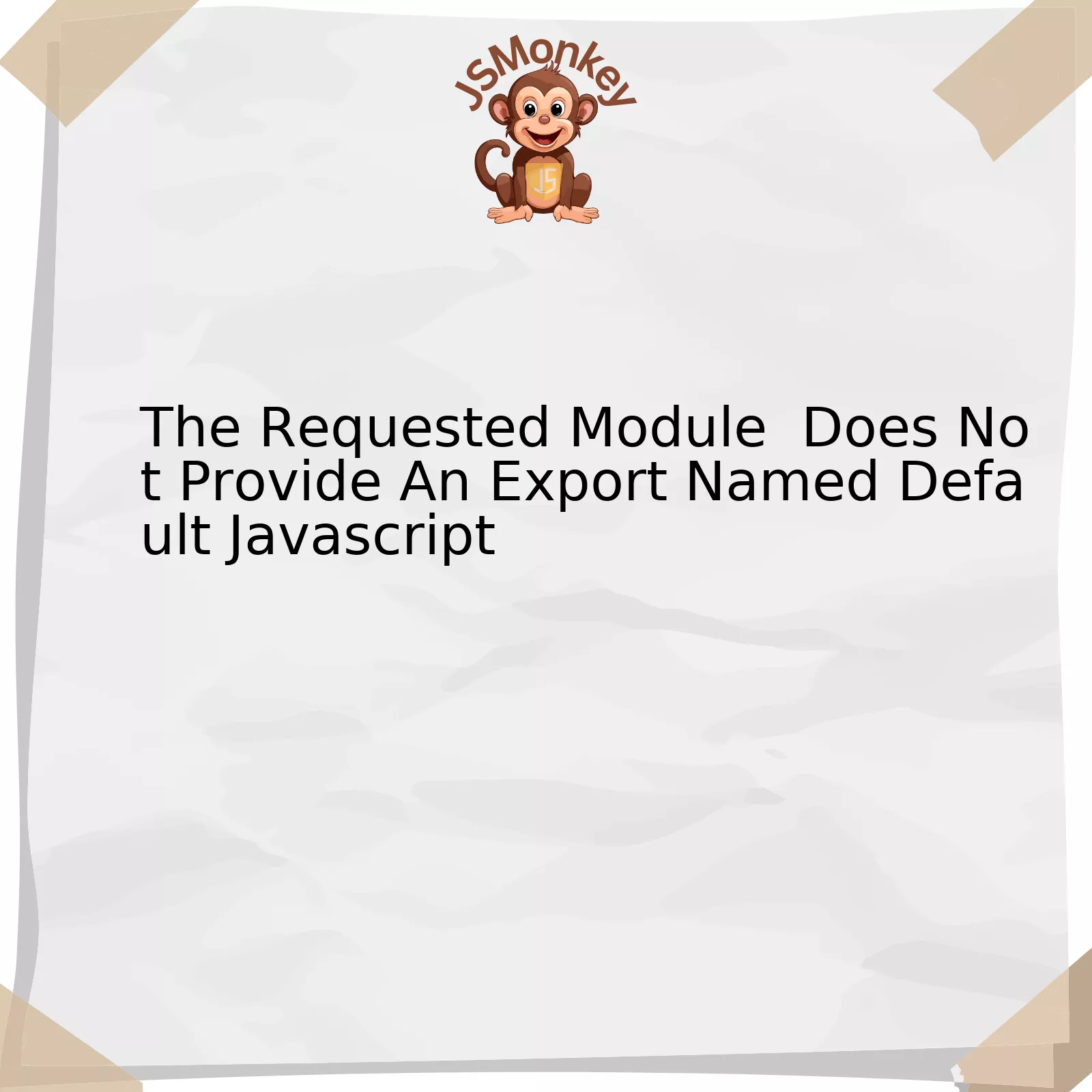
Oh, absolutely. The error “The Requested Module Does Not Provide An Export Named Default” in JavaScript is encountered when we attempt to import a default module from another file using the
import
statement but that module has not been exported as such.
Let’s provide you with an illustration in tabular format:
Case | Code snippet | Description |
---|---|---|
Export of default module |
// general.js let val = "Hello world!"; export default val; |
In the example here, the value
"Hello world!" is being exported as a default from the module. |
Correct import of default module |
// app.js import greeting from './general.js'; console.log(greeting); // logs "Hello world!" |
The module that is set as default in the source file can be imported into another JavaScript file by merely specifying the import pathname. Like this example wherein the string
"Hello world!" is successfully imported and used. |
Erroneous handling leading to the issue |
// general.js let val = "Hello there!"; export {val}; // app.js import val from './general.js'; //will throw error 'The Requested Module Does Not Provide An Export Named Default' |
Here, the value is not exported as a default. Consequently, when attempting to import it as such, JavaScript throws an error because no default export can be found, manifesting as “The Requested Module Does Not Provide An Export Named Default”. |
As you observe, the way JavaScript’s module system works necessitates that anything we wish to utilize elsewhere in our codebase must explicitly be made accessible, or “exported”. A “default” export signifies a fallback or “main exported value” for a module that makes imports tidier if only one thing is being exported.
For avoiding such errors, ensure your
import
/
export
statements align correctly with each other in your codebase and that you’re exporting as default if you’re importing a default.
This issue underlines the importance of getting well acquainted not just with a language’s syntax but also its norms of use, as robustly expressed by Steve McConnell in his renowned book Code Complete: “Managing complexity is the most important technical topic in software development. In my view, it’s so important that Software’s Primary Technical Imperative has to be managing complexity.”
Understanding the Concept of Module Export in JavaScript
When diving into JavaScript modules, a significant concept to grasp is the export functionality. Before reaching that comprehension, one should recognize foundationally what JavaScript modules are. Modules in JavaScript can be seen as self-contained “pieces” of code that can be utilized and exported to different parts of a project. It’s like composing your favorite melodies separately and then putting them together to create an enthralling symphony. Notably, JavaScript modules help keep your code organized and efficient by splitting it into separate files with specific purposes, thus maintaining the clean-architecture approach.
Now on to ‘Module Export’. The export statement in JavaScript is used when creating JavaScript modules to export functions, objects, or primitive values from the module so they can be used by other programs with the import statement.
The phrase
The Requested Module Does Not Provide An Export Named 'Default'
essentially informs us that there is an attempted import from a module without a default export specified within that module file. In JavaScript, there are two ways to export modules: named and default exports.
1. Named Exports (Zero or more per module)
Under this method, you can have multiple named exports per module. The users of the module will employ the same name to import these functions.
Here is how we usually define a named export:
// functions.js export function add(a, b) { return a + b; }
And here is how you would import a named export:
// app.js import { add } from './functions.js'; console.log(add(1, 2));
2. Default Exports (Only one per module)
A default export can be thought of as the fallback export for the module, also used when only a single result value should be exported. There can only be one default export per module. When importing a default export, you don’t need to use curly braces `{}`.
Here is how we define a default export:
// functions.js export default function add(a, b) { return a + b; }
And here’s how you would import a default export:
// app.js import add from './functions.js'; console.log(add(1, 2));
Hence, addressing the issue in question
"The Requested Module Does Not Provide An Export Named 'Default'"
, it generally arises due to attempting to import a module using the default import syntax when it has not been exported as such. To avoid this error message, one should ensure that the syntax used for exporting and importing JavaScript modules matches appropriately.
At last, in the words of Edsger W. Dijkstra, “If debugging is the process of removing software bugs, then programming must be the process of putting them in”. So remember to match your exports and imports correctly and you’ll be just fine.
Exploring Errors: The Requested Module does not Provide an Export Named Default
Working with Javascript modules is a seamless process most of the time, but there are those moments when we encounter errors that can be puzzling for even experienced developers. One such error is “The Requested Module does not Provide an Export Named Default.” Understanding this error and knowing how to resolve it will significantly streamline our development workflow.
This error typically occurs when we try to import from a module using syntax for importing a default value, yet the exported item doesn’t have a `default` flag expressly set. Not all values exported from a module have a `default` status, thus they must be gripped differently.
Let’s navigate through the specific mechanisms leading to this error and potential solutions.
Consider an example of an array export from a module named `example.js:
export const arr = [1, 2, 3];
If this array is imported in another file using:
import arr from './example.js';
It will throw ‘The Requested Module does not Provide an Export Named Default’ error. This happens because ‘arr’ is not a default export.
A solution is to change your import statement and use destructuring to access ‘arr’:
import { arr } from './example.js';
This approach will help avoid the aforementioned error as it correctly imports ‘arr,’ utilizing its actual name rather than the `default`.
Note further that ES6 allows both default and named exports in a module. For example:
// inside example.js const arr = [1, 2, 3]; export default arr; export const str = 'Hello World!';
Both `arr` and `str` get exported, but `arr` is the default export while `str` is a named export. To import these, you would use:
// importing in another file import arr, { str } from './example.js';
Implementing these changes provides a viable solution to the error “The Requested Module Does not Provide an Export Named Default.”
As Anthony Burchell, a renowned software engineer, once said, “Coding is a puzzle waiting to be solved. Each problem helps us build a better solution next time.” Thus, understanding each error we encounter in our development journey strengthens our skills and enlarges our coding repository.
This resource provides additional information on JavaScript modules and exports/imports.
Decoding and Resolving JavaScript Export-Import Issues
Understanding and resolving JavaScript export-import issues hinge primarily on getting to grips with one of the linchpins of modular JavaScript, which is undoubtedly the ‘require’ and ‘import’ statement. The error message “The requested module does not provide an export named default” frequently surfaces due to a misunderstanding between the interplay of default and named exports.
Default Exports vs. Named Exports
Default Exports: Every module can have just one default export. Default export can be either object, function or even a primitive value. It doesn’t always need a name because it is assumed to be the main exported value of the module. For example:
export default myFunction;
Named Exports: A module can have multiple named exports. Each named export reflects a unique property that you would like to expose from the module for usage in other modules. For instance:
export { myFunction };
The key difference here is in how they are imported.
Importing Default Exports: When importing functions or values exported as default, we don’t have to specify their names.
import myFunction from './myModule';
Importing Named Exports: While importing named exports, the exact name of the exported function or variable has to be used within curly braces.
import { myFunction } from './myModule';
The error “The requested module does not provide an export named default” typically implies there’s a mismatch between the type of export (default or named) in your file, and the type of import being expected.
Resolving the Error
There are a few ways to preemptively sidestep this issue:
• If you’re facing this error, it likely means you tried to use a default import statement on a module that doesn’t have a default export. You could switch your approach to named exports instead. This means using curly braces for imports and supplementing your file with ‘export’ before the constants, classes or functions you’d like to use elsewhere.
• Alternatively, you may add a default export to the requested module if it is within your control.
• Lastly, checking the documentation of the library you are importing from can make it abundantly clear whether you should be using default or named imports. Don’t assume that every library supports both. For instance, certain popular libraries like “lodash” only support named imports, while some like “express” prefer default imports.
John Resig, the creator of jQuery once said, “Write code that’s easy to delete, not easy to extend.” Although he wasn’t specifically referring to JavaScript modules and the import/export syntax, the philosophy translates well into this circumstance. If you’re constantly finding yourself in a bind due to tangled imports and exports, it might be time to audit the structure of your modules, strive for simplicity and potentially discard complicated patterns.
In essence, understanding the differences between default and named exports and being aware of which one to use when requiring a module ensures smooth and efficient transference of code components across files. It keeps the codebase readable and maintainable by letting each part of your application live in its own dedicated module. Remember, gathering clarity on your import/export needs is fundamental to building clear and concise JavaScript projects.
Practical Tips to Avoid ‘No default export found’ in your JavaScript Code
Before we unravel the “No default export found” problem in JavaScript, it’s essential to grasp a core understanding of the concept of ‘exporting modules’. JavaScript modules are distinct scripts that can import functions from one another by declaring them as `export` or `import`. To-a-point, exporting makes your code re-usable, maintainable and clean.
Let’s break down the error message: The Requested Module Does Not Provide an Export Named Default. This typically implies the module which is being imported either doesn’t have a `default` export or is not using the correct syntax for exporting a module.
To counter this issue, let’s dive into some practical tips:
– Use named exports: Named export allows you to export multiple values, where each value has its name. When importing, use curly braces `{}` to encompass the function or variable name. Here’s an example:
`
export const exampleFunction = () => {...}
`
You could then import the module with:
`
import { exampleFunction } from './exampleModule'
`
– Implement default exports: A module can only contain one `default` export. It’s useful when the module exports just a single thing, for instance, a function. The `import` statement can be made without curly braces `{}` in this case.
An exemplary `default` function export looks like this:
`
export default myFunction;
`
And you simply import it without any curly braces:
`
import myFunction from './myModule';
`
– Correct Syntax: Sometimes the ‘No default export found’ error arises due to an incorrect or mismatched syntax. Double check your syntax, make sure the export match up with their respective imports and vice versa.
– Treeshaking: If your setup includes bundlers such as Webpack or Rollup, they offer a process called ‘treeshaking’ which helps to remove unused exports to reduce the impact of fat modules^[1]^.
Reflecting on these tips, remember that “Programs must be written for people to read, and only incidentally for machines to execute.” – Harold Abelson. Functionality and readability should go hand in hand when writing JavaScript codes; constantly practice the art of making it understandable to yourself and others who might look at your code in the future.
The topic at hand is an error message ‘The Requested Module Does Not Provide An Export Named Default’ that JavaScript developers may encounter when they are working with modules in their project. This error typically happens when trying to import a default export from a module that does not have one.
Every JavaScript module generally has its own unique scope, where local variables, functions or classes declared do not affect the outer or global scope. However, there are situations where you might want to share your code across several files and for this purpose, we use exports and imports.
Let’s say we have two files, file1.js and file2.js. If we’re handling an object or function in file1.js that we desire to utilize in file2.js, we’d need to export it from file1.js and then import it into file2.js.
A common practice is using the
export default
instruction in a module, which makes a single value or entity available for importing into other files. The subsequent syntax would look like so:
// file1.js export default myFunction; . . .
Here,
myFunction
can now be imported from another module using:
// file2.js import myFunction from './file1.js'; . . .
In some scenarios however, a developer might run into “The Requested Module Does Not Provide An Export Named Default” error. The error arises because the script from which we’re trying to import doesn’t have any default exports. In simpler terms, the item you’re referencing in your import statement doesn’t exist in the exported items list of your referenced script/module.
The solution is simple, check the exporting script/module. You’ll probably find something like:
// file1.js export { myFunction }; . . .
Here, the developer isn’t declaring a default export but rather an “named export”. It could very well be the case that multiple items are being exported in this manner.
To avoid the error, ensure you’re importing named exports correctly like so:
// file2.js import { myFunction } from './file1.js'; . . .
As Linus Torvalds said, “Talk is cheap. Show me the code.” So keep your coding practices consistent and remember to match your import syntax with the type of export declaration done in the referenced module.
The proper use of modules can result in maintainable code, better productivity and less prone to errors since they provide a way of deploying encapsulation, a core principle of object-oriented programming. Moreover, exploring JavaScript’s modules features further can help in understanding and solving common JS errors such as ‘The Requested Module Does Not Provide An Export Named Default’ promptly.
Reference:
JavaScript export statement – MDN Web Docs