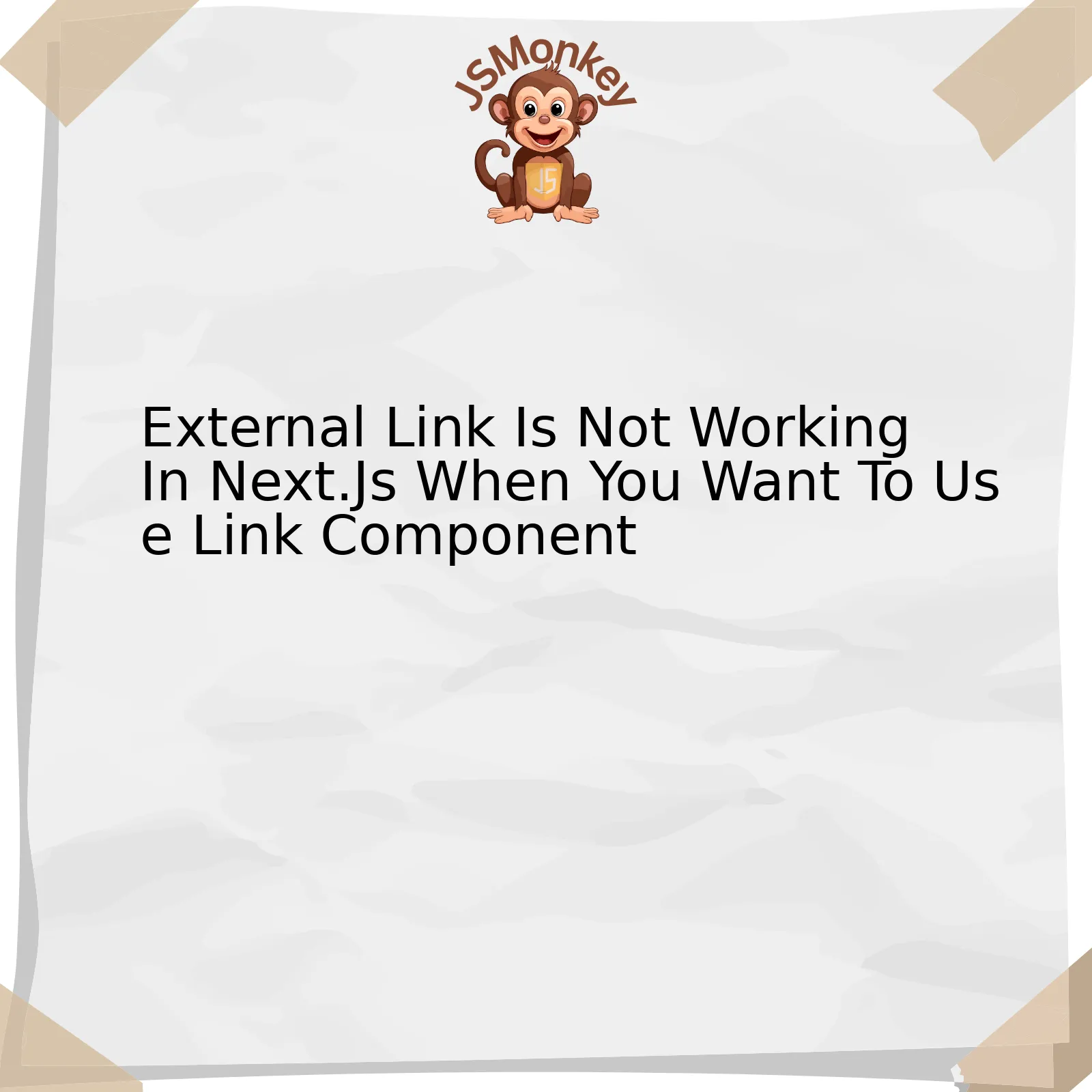
The issue of “External Link Not Working in Next.js when using Link Component” can be explored by comparing the behavior of native anchor (
<a>
) tags and Next.js’s Link component. Often, developers utilize the
Link
component from the ‘next/link’ package for internal routing within a Next.js app, but the
Link
component is not designed to handle external links, hence you may experience issues with external linking while using it.
Breaking it down and analyzing:
Element/Component | Usage | Pros | Cons |
---|---|---|---|
<a> (anchor tag) |
Suitable for creating both internal and external links. | Familiar syntax for most developers. Works consistently across many browsers. | Does not benefit from the preloading capabilities or route prefetching provided by Next.js’s Link component. |
Link from ‘next/link’ |
Designed for internal navigation within a Next.js application. | Offers enhanced performance features such as automatic preloading and route prefetching. | Not built to handle external links. |
By understanding these differences, it becomes apparent why using the Next.js’s
Link
component may not work as expected for external links. If you want to link to an external page, use the native anchor tag, as exemplified below:
<a href="https://externalwebsite.com">Visit External Website</a>
Meanwhile, for internal routes, you can use the
Link
component:
import Link from 'next/link'; // then in render <Link href="/internal-page"><a>Go to Internal Page</a></Link>
As Paul Irish, a Google Chromium developer, once said, “The best products do not focus on features, they focus on clarity.” Therefore, while the enhanced performance features of Next.js’s
Link
component are appealing, it’s important to understand its limitations regarding external links. Clear and appropriate usage will provide the most stable results for your web application.
Understanding Next.Js and the Link Component Functionality
Next.js is a powerful React framework, developed by Vercel, for creating user interfaces. An essential aspect of this framework is the Link component that allows efficient client-side transitions between routes in your application.
The
Link
component in Next.js is often employed for wrapping around other components, such as buttons or text, to provide click-through functionality that navigates to different pages within a Next.js application. By default, it achieves this through client-side navigation where the browser doesn’t need to fetch a new HTML document from the server.
However, if you’re encountering an issue with the external link not functioning when using the Link component, it’s typically due to a misunderstanding of what the Link component is designed for. The Link component is intended exclusively for internal navigation within your app. Understandably, it can be confusing since traditional HTML anchors are often used for both internal and external links.
If you’re looking to navigate outside of your Next.js app – for example, linking to another website, then the standard HTML
tag would be more suitable. Here’s an illustration:
In the above snippet, “noopener noreferrer” are added in rel attribute, which are recommended for safety reasons when using target=”_blank”. “noopener” prevents the new page from being able to access the window that opened it, and “noreferrer” masks the origin of the request.
For situations in which one needs to use a component or prop based approach like Next.js Link offers, consider devising a helper function that renders a Link for internal urls (paths that start with “/”) and an
a
tag for external ones.
Remember to distinguish between when to use the Link component and when to use the standard anchor tag. Like Marc Andreessen, co-founder of Netscape and a leading venture capitalist in Silicon Valley once said, “In technology, it’s about the people – getting the best people, retaining them, nurturing a creative environment & helping to find a way to innovate.”
You may refer to the Next.js documentation here for a more detailed understanding of using Link component in Next.js.
Addressing Issues with Non-Functioning External Links in Next.js
Next.js, a powerful JavaScript library, fosters the development of robust web applications and offers numerous features — one being the handling of internal links through the
Link
component. However, this isn’t the case when dealing with external links. The Next.js `` component doesn’t handle external URLs, as it’s specifically designed for internal routing to different paths in your application.
Using the `` component for an external link can lead to issues such as the link not functioning as expected or even breaking the application. Here’s how you should avoid doing:
<Link href="https://www.externalwebsite.com"> <a>External Website</a> </Link>
The correct way to address external links in Next.js is to use the standard HTML `` tag with the `href` attribute pointing to the external URL.
<a href="https://www.externalwebsite.com">External Website</a>
You might wonder, ‘Why design a `` tag in Next.js if we still need to use the `` tag?’ It’s important to understand that the `` component does add significant value, but for internal routing. When used correctly within the realm of Next.js pages, it provides pre-fetching functionality which is a core aspect of the superior performance of Next.js applications.
Apart from simply using the anchor `` tag, you also have the option to leverage custom hooks in Next.js to handle external links. For instance, you can set up a hook which ensures all URLs that do not belong to your site’s domain are opened in a new tab, providing additional user experience control.
Additionally, be aware that managing internal and external links correctly is crucial not only for functional reasons but also in the context of SEO (Search Engine Optimization). Search engines distinguish between internal and external links, applying different evaluation metrics in their ranking algorithms.
“External links are more important than internal links. So far as boosting your domain’s authority and reputation.” – Neil Patel, co-founder of NP Digital
Understanding these perspectives in Next.js link management makes it extremely important to correctly handle both types of links. By doing so, your application will not only run smoothly but also improve in terms of SEO.
Incorporating Effective Methods to Fix Broken External Links in Next.js
Fixing and managing non-operating external links within the Next.js framework can be accomplished by implementing the
Link
component correctly. In this context, the Link component from Next.js is a vital element which allows for internal navigation between pages in your Next.js application. However, while working with external links, a different approach needs to be adopted.
To troubleshoot problems with external links not functioning properly in Next.js, utilize the standard HTML anchor tag
<a>
instead of the `Link` component when dealing with external URLs.
Here is an example:
html
External Link
The above code snippet helps facilitate effective redirection to external sources. Nonetheless, it’s essential to understand that the HTML
<a>
“link tag” will cause the page to refresh, unlike the Link Component that doesn’t.
The complexity of addressing broken links goes beyond mere incorporation of the
a
HTML marker. To ensure an optimal user experience, you might want to:
* Monitor Your Site Regularly – Automated tools provide solutions to monitor website’s hyperlinks and report if any link breaks. Services like [Dead Link Checker](https://www.deadlinkchecker.com/), [Broken Link Check](https://www.brokenlinkcheck.com/), or [Dr. Link Check](https://www.drlinkcheck.com/) can be effectively used for this purpose.
* Get Notified When A Link Breaks – Services such as Google Search Console send notifications via email if such anomalies surface.
In the words of Jaime Levy, an esteemed UX strategy author and professor, “bad first impressions caused by faulty links or poorly directing them are hurdle to companies’ credibility.”1 Therefore, to maintain site credibility and ensure a smooth journey for the users, it is important to fix faulty links.
Finally, note that while Next.js excels at routing for internal pages, when managing external links in a Next.js application, HTML’s native capabilities can often provide the most effective solution.
1 [Jaime Levy, UX Strategy: How to Devise Innovative Digital Products that People Want](https://www.goodreads.com/book/show/24743127-ux-strategy)
Examining Best Practices for Creating Reliable Working Links in Next.js
Next.js is a popular JavaScript framework built on React that is used heavily for server-side rendering. One key feature of the Next.js is its routing system, which includes the Link component to facilitate navigation in your application. However, there are reported instances where users struggle with using the Link component for external links. The best practices for creating reliable working links in Next.js becomes quite crucial in this regard.
Why Using Next.js Link Component for External Links May Not Work
When it comes to the
Link
component in Next.js, developers might run into issues with linking to external URLs. This happens because the
Link
component in Next.js is primarily made for internal navigation i.e., link redirection within pages in your app and not designed for external linking (linking to different websites). Using
Link
for an external URL, therefore, may lead to unpredictable behaviors.
Making External Links Work in Next.js
While you can’t use the
Link
component directly for external links in Next.js, there is still a workaround. You can essentially use the native HTML anchor tag (
<a>
) as a child of the Next.js
Link
component. However, this may still include unnecessary processing for external links in your Next.js app. Therefore, in the actual scenario, if you need to create a secure, reliable and stable method for external linking in Next.js, simply rely on the standard HTML
<a>
tag.
Code Example:
The following example demonstrates how to use an anchor tag for consistent and reliable external linking within your Next.js application:
<a href="https://external-website.com">Visit External Website</a>
Considerations:
- If the link is external, employ a plain HTML anchor tag instead of Next.js Link component.
- Always specify the full URL (including ‘http://’, or ‘https://’) for external links.
As Bill Joy, co-founder of Sun Microsystems, once said: “Innovation happens elsewhere.” This sentiment resonates with us in the field of software development where we are constantly learning and adopting best practices from others. While the
Link
component may not be the solution for external links in Next.js, turning to the traditional HTML
<a>
tag can certainly prove to be a reliable solution.
You can find more details about Next.js routing and linking in the official Next.js documentation.
Troubleshooting the issue of the external link not functioning properly when utilizing the Link component in Next.js can be linked to multiple potential culprits, often found within the intricacies and specifics of your coding strategy. Here is some insight into the problem:
Firstly, the Next.js
Link
component was inherently designed to only function with internal routing systems. Its main objective revolves around supporting the navigation through pages in a Next.js application. When you attempt to use this feature to create an external link – for instance, directing to a Google page or another domain entirely – you are straying from its intended usage. In these scenarios, it’s likely that Next.js will report errors or peculiar behaviors due to improper usage.
The solution is fairly straightforward: Consider reverting back to utilizing the traditional HTML
<a>
tag for defining hyperlinks that lead to external destinations. The following illustrative snippet emphasizes this:
<a href="https://www.google.com" target="_blank"> Visit Google </a>
This enables you to retain the expected behavior of linking to an external source without facing the issues Next.js presents with using their
Link
component for this purpose.
Following this direction should ensure smoother transitions and interactions with your links. It’s essential to understand the role and limitation each tool plays in building and managing your projects—an integral and non-negotiable aspect of web development that eliminates numerous unpredictable scenarios beforehand.
Remember, as Marshal McLuhan once said, “We become what we behold. We shape our tools and afterwards our tools shape us.” Embracing the unique peculiarities and strengths of different components like Next.js’s
Link
and HTML’s hyperlink system is critical to crafting optimally functioning web applications.