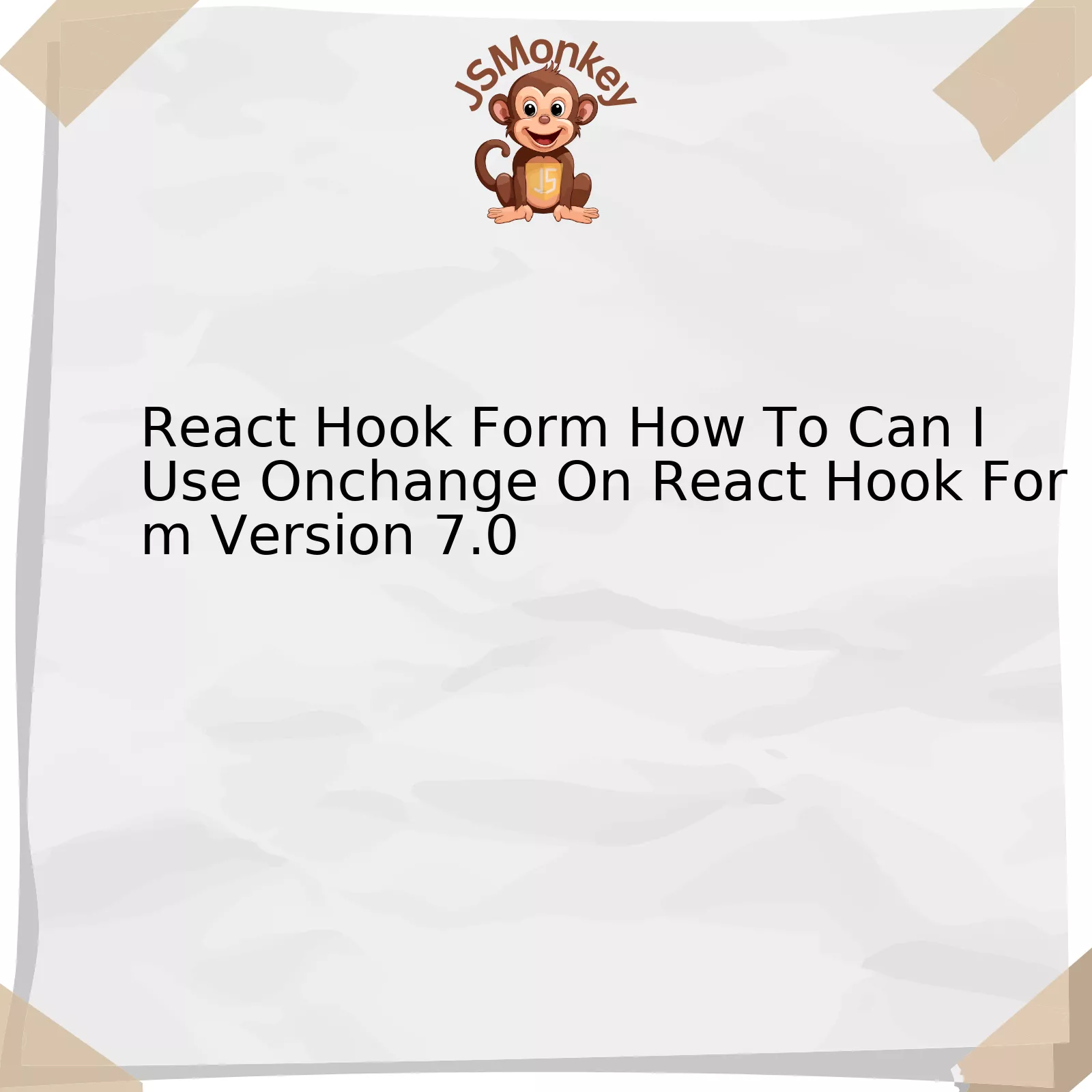
React Hook Form is a highly efficient, intuitive, and simple to use library that supports various APIs for form validation in React applications. With the Version 7.0 release, many improvements have been made, introducing increased functionality and versatility. For intricate conversations concerning particular properties such as ‘onChange’, it’s paramount to fathom the particulars of their employment.
In handling ‘onChange’ with React Hook Form version 7.0, you may consider the following tabulated fundamental elements:
Element | Description |
---|---|
register |
This function enables you to register input/select Ref at the custom hook. It attaches necessary event listeners for the component. |
useForm |
An essential custom hook provided by React Hook Form. A series of functions are returned that aid in managing the form; one of these functions is the ‘register’ function. |
Controller |
In case you choose not to use the ‘register’ function to hook up inputs, a ‘Controller’ would be ideal. It will monitor and test the input for you while integrating with other UI libraries smoothly. |
Upon delineating the table contents above, an important aspect to highlight is the manner of utilizing ‘onChange’ handlers on registered components. Since the primary role of handlers such as ‘onChange’ is catching particular events occurring in DOM elements, they play a critical role within the React framework.
Explicitly mapping ‘onChange’ onto form fields can sometimes lead to needless re-renders and overall code bloat within your forms. To bypass this, React Hook Form utilizes the `register` function provided from the `useForm` hook. This allows you to gather the required information without using ‘onChange’.
Here’s an example of how to achieve this:
html
The crucial point here is that React Hook Form’s register function automatically attaches ‘onChange’, ‘onBlur’, and many other event listeners for you. Therefore, no explicit declaration is needed.
Another noteworthy facet is handling external or custom components with libraries such as MUI or AntD which hide ‘ref’ within their implementation, thereby making it challenging to ‘register’. For these scenarios, React Hook form introduces the `Controller` component:
html
/>
In hindsight, instead of directly registering the ‘ref’, Controller provides a smoother way of maintaining form states by bridging the communication between React Hook Form with other UI libraries.
As Brian Kernighan once said, “Controlling complexity is the essence of computer programming.” Understanding subtle frameworks such as React Hook Form and utilizing them effectively is indeed a fundamental part of managing this complexity. It does not only provide a simple approach to crisp HTML forms but also improves performance by minimizing unnecessary re-renders.
Utilizing OnChange in React Hook Form Version 7.0
React Hook Form (RHF) provides a more efficient and granular approach to form management in React applications, as it leverages the power of uncontrolled components and utilizes the native HTML5 form validations. In version 7.0, RHF introduced improvements and breaking changes that developers need to consider, especially concerning form handling via the onChange event.
Hook | Description |
---|---|
register() |
This method allows the tracking of input element(s) and extraction of its value(s). |
handleSubmit() |
This function will execute form validation on submission and provide form data if successful. |
watch() |
A function enabling you to watch selected inputs and render based on those input values. |
setError() |
Allows user to manually set an input field error. |
In React Hook Form Version 7.0, handling the `onChange` event in forms has some subtle differences compared to traditional controlled components. Instead of incorporating `onChange` for every field, one could combine the use of `register()` within `ref` attribute in the input fields which would automatically update and collect user input data. With this approach, the component doesn’t need to re-render each time the state updates, thus enhancing performance.
An example on how to achieve that:
jsx
import { useForm } from ‘react-hook-form’;
function UserForm() {
const { register, handleSubmit } = useForm();
const onSubmit = data => console.log(data);
return (
);
}
In the code snippet above, React Hook Form’s `register` function is used to register an input field into the custom form hook. Whenever a user inputs data, the `onChange` event occurs under RHF’s hood, effectively keeping track of the input field’s value.
register |
An application programming interface (API) that allows you to register a field within the custom useForm hook. This eliminates the need for manual management of onChange, onBlur events and local state within each form component. |
Remember that forms created with RHF will not re-render upon changes by default. If one wants to listen for specific changes in input values, using the watch method would be ideal. This method returns the value of the specified input or inputs, triggering a re-render when the input(s) change.
Ken Thompson, co-inventor of UNIX, once said, “One of my most productive days was throwing away 1000 lines of code.” While upgrading to React Hook Form Version 7.0 might prompt you to modify some lines of code related to your form validation, it’s likely that the net outcome will be a cleaner, more efficient approach to form management.
For further information about React Hook Forms, consider exploring the official API documentation where detailed explanations and examples about these powerful features can be found.
In-Depth Look: How to Use React Hook Forms
React Hook Form (RHF) version 7.0 provides a seamless way to deal with form validations in React applications, thereby improving the performance and user experience of your web application. Rather than focusing on the typical `onChange` events that most forms libraries use, RHF leverages `onSubmit` events which gets triggered when the form is validated successfully. However, if there’s a desire or need to capture `onChange` events, one could still accomplish this.
To employ `onChange` with React Hook Form, here are several strategies you can consider:
Strategy | Description |
---|---|
Use the
watch function |
RHF’s
watch function allows for monitoring input value changes. This can effectively simulate `onChange`. |
Make use of the Controller component | The Controller component from RHF wraps around your inputs and allows you to control the rendering, as well as providing callbacks such as `onChange` |
### Use the watch function
The
watch
function supplied by RHF can be used to track changes in the form field values. It observes form state and re-renders your component upon any changes.
So, the assigned variable always re-represents the latest value from the designated form field. Here is an example of how it can be used:
html
import { useForm } from ‘react-hook-form’;
function YourComponent() {
const { register, watch } = useForm();
const watchAllFields = watch();
console.log(watchAllFields);
return (
);
}
“Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” – Martin Fowler, 2008
### Use the Controller Component
The `Controller` component is another versatile element provided by RHF. It abstracts away the registration process and provides an interface to easily integrate with other UI libraries whilst offering a simple way to integrate and overwrite `onChange` events.
An example implementation where `onChange` is used could look something like this:
html
import { useForm, Controller } from ‘react-hook-form’;
function YourComponent() {
const { control } = useForm();
return (
/>
);
}
In conclusion, although React Hook Form focuses primarily on `onSubmit` events, it has flexibility for use cases where `onChange` events need to be captured. This can be achieved via features like the `watch` function and `Controller` component.
For deeper insights on these concepts in React Hook Form, you might want to check out [React Hook Form’s official documentation](http://react-hook-form.com/).
Optimizing Performance with React Hook Form
Optimizing performance in a React application is key to providing an engaging user experience, and React Hook Form (RHF) is a humming engine under the hood, powering form processing at high speeds. Introduced in version 7.0, RHF brought new capabilities for using
onChange
events.
Firstly, it’s crucial to know that the
useForm()
hook in RHF v7.0 has an `mode` option which governs when form validation should occur. By setting the mode to
'onChange'
, the form validation will run on each input change. This offers you granular control over form validation – each keystroke allows feedback to be provided instantly to the user if their input doesn’t meet the necessary criteria.
HTML
import { useForm } from ‘react-hook-form’;
const { register, handleSubmit } = useForm({ mode: ‘onChange’ });
…
However, while using
onChange
provided by RHF can enhance real-time validation, care must taken to avoid unnecessary re-renders thus causing potential performance bottleneck. As JavaScript engineer Addy Osmani quoted “Less JavaScript means less parse/compile/render time”. Your aim should always be to strike a solid balance between real-time feedback to the user and overall performance efficiency.
In relation to this, one of the handy aspects of RHF v7.0 is its refactored
Controller
component. It enables integration of controlled components, like Material UI or Antd components, inside your forms. Inside
Controller
, you can make use of the
render
prop method which receives
field
. Here you will find
field.onChange
that you can wire up with your controlled component’s
onChange
.
HTML
import { Controller } from “react-hook-form”;
…
/>
…
In the grand scheme of things, your optimal React application performance involves judicious use of form validation, leveraging the benefits of RHF v7.0, without overtaxing browser resources. Increasingly complex web applications necessitate sharp focus on crafting efficient JavaScript for an ever-demanding mobile-first world.
For a deeper dive into best practices and performance with React Hook Form, I recommend exploring their official [documentation]. The demo section provides enlightening examples that allow you to see the hooks in action while the API documentation lets you delve deep into each hook’s capabilities.
As web guru Steve Souders once said, “Performance is a feature.” Maximizing that feature through intelligent form management and utilization of React Hook Form can go a long way toward enhancing your web application performance. Approach every coding decision as an optimization opportunity and you’ll inevitably build web applications that meet user expectations for both functionality and speed.
Mastering Validation Techniques in React Hook Form
React Hook Form, an efficient and versatile library in React for managing form states, provides exceptional validation techniques that can be mastered with a little effort. The current version 7.0 of React Hook Form supports the use of `onChange` events which is a significant shift as updates are no longer triggered automatically.
React Hook Form and Validation
The key advantage of using React Hook Form for validation is its extensive customization potential. It relies on uncontrolled components, maximizing performance by minimizing the number of re-renders. Furthermore, it also offers built-in mechanisms to handle errors effectively. You can validate each input individually, or all of them together for multi-step forms.
Usage of OnChange in Version 7.0
In version 7.0 of the React Hook Form, the usage of `onChange` has been made more explicit. Instead of triggering re-renders automatically after each keystroke or change, it is more streamlined and performance-orientated now. Here’s how you can implement this:
You can register your input with the help of the `useForm` hook, for instance, like this:
const { register } = useForm(); <input {...register("example")} onChange={handleChange} />
This code registers an input field and also attaches a `handleChange` function to it, that will execute whenever there’s an ‘OnChange’ event.
In the `handleChange` function, you can add your own custom logic and validation rules, validating the data exactly as you want. This level of control makes handling complex validations simpler and easier.
Supported Validation Methods
Amongst several supported methods for validation, one of the influential methods includes leveraging `register`–a method exposed by `useForm`. With this, each field can be individually registered for validation with custom rules.
Also, you can make good use of `watch` and `getValues`, to observe changes and retrieve input values respectively. These hooks enable you to write more complex validations that depend on multiple fields or change over time.
With mastery in these efficient validation techniques, React Hook Form’s expressive power can boost the overall quality and performance of your web forms.
As Edsger W. Dijkstra famously said, “Program testing can be used to show the presence of bugs, but never to show their absence.” Use these validation techniques effectively as they are essential safety nets for maintaining the integrity of data inputs.
The interaction with forms in React has evolved significantly with the incorporation of React Hook Form, particularly in its version 7.0. Renowned for its performance optimization and smoother user interactions, it has become a popular choice among developers.
One common question that arises is how to utilize the ‘onChange’ event with this library. Gracefully, the version 7.0 accounts for this need by replacing the traditional means used in class components with a more sophisticated approach.
To illustrate, let’s presume we need to capture and handle data entry into an input field. In typical scenarios, this would be accomplished with the ‘onChange’ event. However, in the case of React Hook Form version 7.0, applying `register` on your input field and destructuring `handleChange` from `useForm` are the main steps:
html
import { useForm } from “react-hook-form”;
function MyComponent() {
const { register, watch } = useForm();
return (
)
}
export default MyComponent;
It’s vital to note, though, that in React Hook Form, `register` encompasses all attribute integrations such as ‘onChange’, ‘onBlur’, and ‘ref’. Watch function is instrumental in viewing form input value updates delicately.
Moreover, as IT leader Chris Wanstrath rightly said, “_Code is not just code. It’s your thoughts encapsulated into an executable form._” Harnessing superior libraries like React Hook Form aids us in producing such profoundly expressive code outputs.
Lastly, I highly recommend diving deeper into the official documentation of React Hook Form for more comprehensive insights. Remember, keeping up-to-date with changes in libraries and frameworks forms an integral part of enhancing your skills as a JavaScript developer.