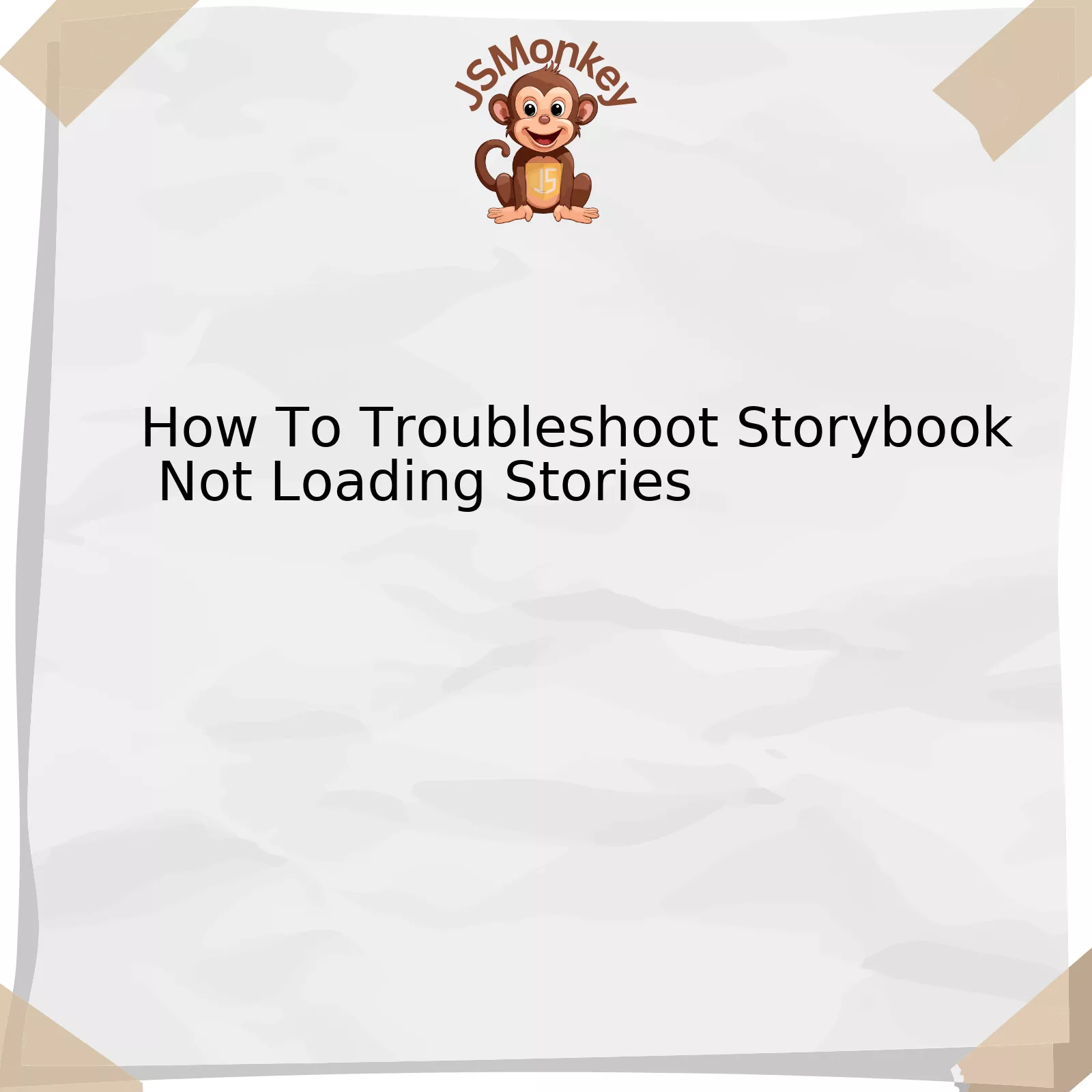
Problem | Potential Cause | Solution |
---|---|---|
Storybook does not load stories | Inappropriate story file path | Check and confirm the accuracy of the directory paths in the
.storybook/main.js configuration file. |
Stories missing from Storybook’s UI | Error in console indicating a specific issue with the story structure | Carefully inspect the console log, seek for error messages and accordingly adjust your code to fix it. For instance, wrong export or syntax can be fixed. |
Blank screen after installing a new addon | Misconfiguration or compatibility issues | Inspect the documentation for the addon and ensure proper integration. Consider checking for version compatibility as well. |
Troubleshooting Storybook not loading stories necessitates a systematic approach. When Storybook is not loading stories, it could be due to a variety of reasons, the most common of which are highlighted in the table provided.
The first cell in the table corresponds to the problem where Storybook fails to load any stories. This issue might arise if you’ve specified an incorrect path for your story files. To solve this, make sure to verify the correctness of the directory paths indicated in your `.storybook/main.js` file.
The second-row deals with the issue when story files don’t appear within the Storybook user-interface. Often, this type of problem is accompanied by a specific error message displayed on your console that indicates a particular issue with the structure of your story. The best course of action for this issue is to thoroughly inspect the console log, locate the error messages, and make the necessary code adjustments to resolve them.
Lastly, the table also addresses the problem when you encounter a blank screen after installing a new addon which might be due to incorrect configuration or compatibility concerns with existing packages or versions. Documentation specific to addons should serve as a reliable reference that can guide you towards the correct integration and configuration steps. Remember to check for potential compatibility issues between different package versions.
As Martin Fowler, a renowned software engineer, once said, “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” It reminds us here that while we solve the technical issues our code faces when loading stories on Storybook, it’s equally important to maintain code readability and properly document the steps so it becomes easier for others (or even ourselves) to debug in the future.
Understanding the Basics of Storybook’s Operation
Under the hood, Storybook primarily operates as a user interface development environment and playground for components. It allows developers to design and test components separately and in isolation from the rest of the application. The fundamental aspect of Storybook lies in its distinct structure: it creates an isolated space for each component – or ‘story’ – thereby helping in visualizing how components appear in different states.
However, if you find that Storybook is not loading your stories, it might be due to an array of factors. Here are some potential solutions to troubleshoot this issue:
Check Configuration File
First and foremost, verify the configuration settings in your
.storybook/main.js
file. Ensure that the path referenced in the
stories
array accurately points towards the location of your story files.
Ensure Correct Naming Convention
Storybook typically identifies stories through filenames ending in
.stories.js
. Make sure your component story adheres to this naming convention, and if your project utilizes TypeScript, the extension should be
.stories.tsx
.
Inspect Component Imports
In every story file, the respective component has to be imported correctly. A mistake in this import statement might lead to Storybook being unable to load the specific story.
Use a Clean Cache
There is a possibility that the cache could have old or conflicting information retained. Try cleaning the cache and running Storybook once again. This operation can be executed with command
yarn cache clean
followed by
yarn install
.
To delve deeper into the working process of Storybook and its troubleshooting, you may visit their official documentation.
As famous computer scientist Donald Knuth said, “Debugging is twice as hard as writing the code in the first place. Therefore, if you write the code as cleverly as possible, you are, by definition, not smart enough to debug it.” This stands true while working with tools like Storybook. Understanding its intricacies will facilitate identifying and rectifying any problems encountered.
Resolving Common Issues Perdaining to Loading Stories in Storybook
Storybook is a popular open-source JavaScript library that allows developers to construct component-based applications, gained traction in modern JavaScript frameworks. However, instances occur when it doesn’t load stories as expected. When Storybook can’t load stories, the difficulties are usually related to either misconfigurations or conflicts with other extensions or environments.
Firstly, let’s consider file loading issues. These may occur due to problems in the filesystem, specifically files not being named appropriately or placed in the correct directories. To counteract this:
– Make sure to place your story files alongside your component files.
– Include a `*.stories.js` suffix on all Storybook story filenames. This wildcard (*) shows that any file that ends with “.stories.js” will be treated as a Storybook file.
// Correct naming MyComponent.stories.js
Another common issue is the misconfiguration of `.storybook/main.js`, which acts as the configuration file for defining how Storybook loads and bundles files.
– Ensure you’ve defined your configurations correctly within `.storybook/main.js`.
module.exports = { stories: ['../src/**/*.stories.mdx', '../src/**/*.stories.@(js|jsx|ts|tsx)'], addons: ['@storybook/addon-links', '@storybook/addon-essentials'], };
Issues might also arise from incompatible versions. At times, the inability for stories to load could be a symptom of differing or incompatible versions between Storybook and its plugins/dependencies. Consider:
– Checking if all dependencies and plug-ins are up to date and that their versions are in sync.
Then, the environment settings like Babel, Webpack, etc., could conflict with Storybook’s functionality, impairing its ability to load stories.
– Check if your project’s babel or webpack configuration is causing an issue. If need arises, set up a custom webpack configuration for Storybook to use.
Aspect of syntax errors cannot be ignored. Syntax errors in your story definition files can lead to them not being loaded correctly.
– Review your story definition syntax carefully against the Storybook documentation to rule this out.
The ever-changing landscape of JavaScript frameworks and libraries makes it significant to incorporate dynamic debugging mechanisms. As Daniel Alghisi noted, “Debugging is the process of identifying, isolating and fixing problems. Everyone will do it differently, but the better you get at this process, the faster you’ll be able to fix issues.”
By investigating all these, one could effectively troubleshoot why Storybook might not load and render stories as required and take appropriate measures to resolve the issue thereby ensuring a smooth workflow in component development.
Delving into Advanced Troubleshooting for Persistent Storybook Errors
The diagnosis of chronic Storybook errors when it’s not loading stories can be robustly performed by dissecting and comprehending several corners which could potentially house the issue.
When faced with Storybook refusing to load stories, one might consider two broad categories of solutions:
– One sphere is encircling adjustments within the Storybook configuration files themselves—the `.storybook` directory.
– The other segment contains a dive into dependencies and environment that your actual project is running on.
Firstly, a dig into the Storybook configuration files: these include `main.js`, `manager.js`, and `preview.js` files housed in the `.storybook` directory.
The primary file among these is `main.js` where you define the list of stories to be loaded via the `stories` key. A typical error here is simply misconfiguration.
Check for invalid path specifications or erroneous glob patterns. The `stories` array should contain valid paths to directories enclosing your stories.
Here is an example illustrating clearly delineated valid path:
html
module.exports = {
stories: [‘../src/components/**/*.stories.@(js|jsx|ts|tsx)’]
};
Additionally, any addons listed in `addons` array within `main.js` should have corresponding packages installed in your project.
Let us pivot towards exploration within the project dependencies and environment plane.
Node version inconsistencies between local development environment and deployment or build environments stand as notorious sources of Storybook refusing to load stories. Frequent practice validating version consistency across different environments can minimize such scenario.
Moreover, ensure your Babel and webpack configurations are congruent with Storybook’s requirements.
In alignment with our comprehension of advanced troubleshooting of persistent Storybook Errors, a reminder from renowned software engineer Robert C. Martin( Uncle Bob), “Debugging is like being the detective in a crime movie where you are also the murderer”. Thus, thoroughly probing our codebase, configs and making precise alignments can lead us to the successful loading of stories in Storybook.
For a thorough and efficient approach to troubleshooting Storybook, consider leveraging official Storybook documentation. It’s a comprehensive resource periodically updated by Storybook creators geared towards smooth navigation through the most common hurdles users might encounter. They cover an abundant ground on debugging techniques and tools tailored for Storybook implementation.
Implementing Best Practices for Preventive Maintenance of Storybook
You can encounter issues with Storybook not loading stories, which can be a common hurdle in development. To eliminate this problem, you should consider implementing preventive maintenance best practices that will keep your Storybook application up and running smoothly. These practices can streamline the debugging process, helping you quickly pinpoint and patch any issues impeding your workflow.
Every developer wishes to avoid situations where Storybook refuses to load stories. Proven preventive measures can help alleviate such concerns. I’ve found that the four most effective methods include:
- Regular Code Reviews
- Frequent Updates
- Implementing Unit Tests
- Paying attention to Error messages
Regular Code Reviews
Regular code reviews are an excellent way to prevent technical issues and bugs. They promote clean and efficient code, minimizing the risk of Storybook failing to function properly. Use a linting tool like ESLint for automatic detection and fixing potential problems within your codes. Additionally, you can utilize Prettier with ESLint to automatically format your code, making it cleaner and more readable.
npm install --save-dev eslint prettier
Frequent Updates
Keeping your Storybook package up to date is crucial. Software updates often come with fixes and improvements that address existing bugs and vulnerabilities. To update your Storybook version, you can use the following command:
npx sb upgrade
. Stay updated with the latest Storybook releases through their official website .
Implementing Unit Tests
Unit testing is an effective practice for catching errors early in the development cycle, making bug hunting a much easier task. Testing libraries such as Jest can be helpful tools for adding unit tests to your Storybook stories. With Jest, you can automate testing, further strengthening your preventive maintenance provisions. Already written unit tests will also aid you in discerning why Storybook may not be loading stories.
Paying attention to Error messages
Error messages are a developer’s clues to what might be wrong. Ignoring console warnings or error messages might cause Storybook not to load your stories. It’s advisable to address these promptly to avoid potential hitches.
As Donald Knuth, a computer science visionary, once remarked, “Let us change our traditional attitude to the construction of programs: Instead of imagining that our main task is to instruct a computer what to do, let us concentrate rather on explaining to humans what we want the computer to do.”
By observing these practices and finding more ways to maintain your code, you’ll significantly reduce the instances of Storybook failing to load stories. It’s all about cultivating an attitude of constant improvement and refinement. Here’s to writing better, more efficient, and problem-free code!
While exploring Storybook, it’s not uncommon to encounter challenges such as the app failing to load stories. Identifying the reason behind this glitch could involve several steps.
First and foremost, take note of any error messages that might be appearing in your console. Key insights to correct functional faults can often be extracted from these error details.
Additonally, scrutinize the structure of your project setup. Analyze if all the prerequisites for Storybook have been correctly put into place – including but not limited to: installation of dependencies and correct directory configuration.
Another strategy would be to revisit the version compatibility. While continually upgrading versions might seem like a good practice, sometimes compatibility issues could emerge due to multiple factors. It wouldn’t hurt to cross verify the compatibility of your Storybook version with associated tools and libraries.
Lastly, dividing your stories into smaller units can also come in handy. Adding too many stories into a single setting can sometimes overwhelm the application.
Sampling a quote, Ada Lovelace once said “Imagination is the Discovering Faculty, pre-eminently. It is that which penetrates into the unseen worlds around us, the worlds of Science”. Following her wisdom, let’s not gag our troubleshooting capabilities, instead approach Storybook with an open mind leveraging skills along with vast development resources available online.
If Your Storybook Fails to Load | Probable Causes |
Error Messages in Console | Error details can provide first clues |
Project Setup | Ensure necessary dependencies are installed & configured correctly |
Version Compatibility | Check if current storybook version works well with other tools/libraries |
Story Size | Large stories may cause loading problems |
In learning to troubleshoot, remember there’s no one-size-fits-all solution. Persistence and curiosity are key in navigating these issues successfully. Also, ensure frequent use of platforms like Storybook official docs or community forums for additional help when required.
No matter the hiccups, let them not deter your journey but fuel your quest instead, moments of struggle often lead to the greatest breakthroughs!