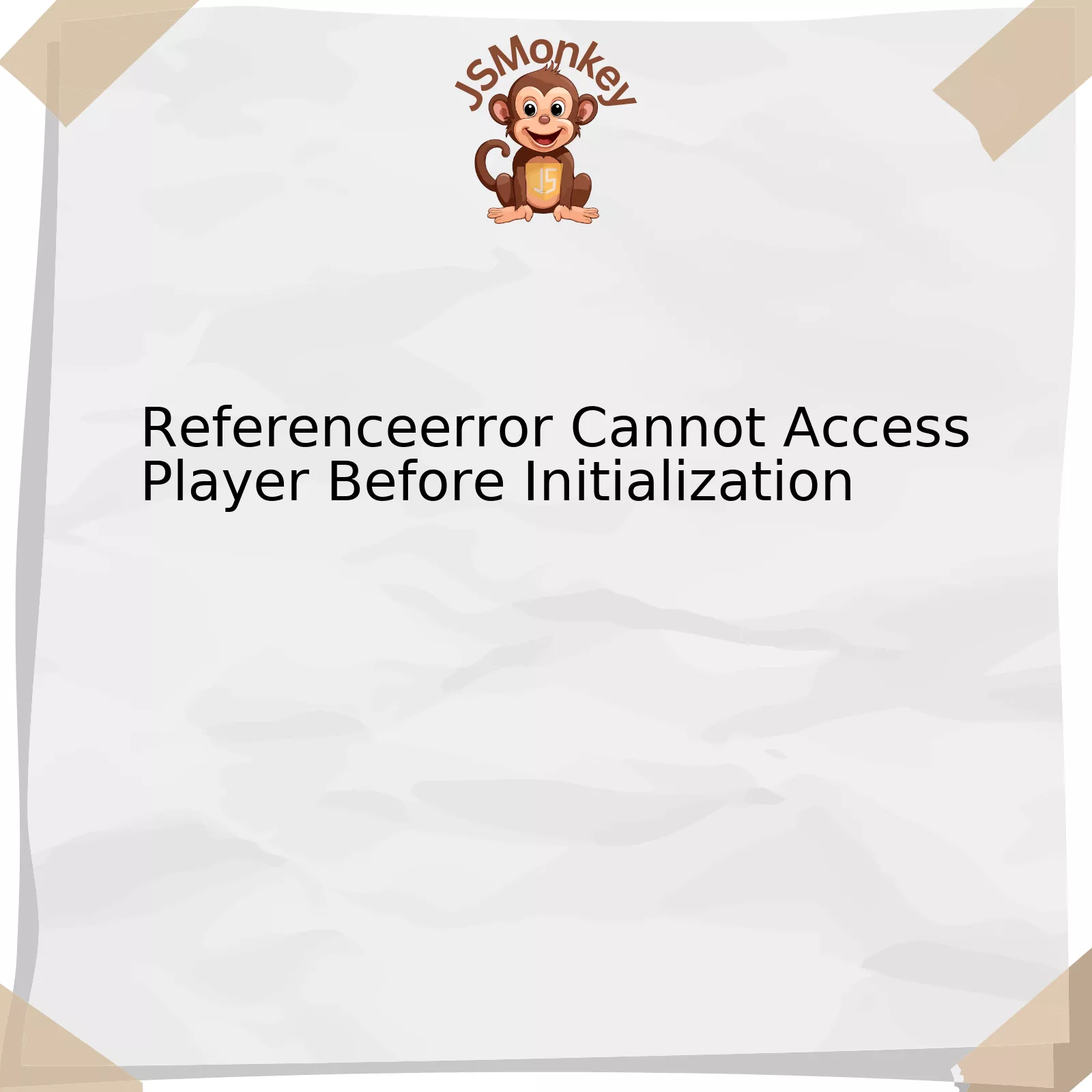
When discussing the “Referenceerror: Cannot Access Player Before Initialization” error, it usually occurs in JavaScript when we try to reference a variable that has been declared but not yet initialized. This error can be better understood by analyzing it in detail using a conceptual representation.
Error Component | Details |
---|---|
Type of Error | ReferenceError |
Error Message | Cannot Access ‘Player’ before initialization |
Common Cause | Accessing a variable before it is defined |
Possible Solution | Ensure the variable is defined before it is used |
Now let’s dive into the details represented in the above structural formation:
– Type of Error – ReferenceError: It’s part of the standard set of core JavaScript errors. It indicates that an invalid reference value has been detected.
– Error Message – Cannot Access ‘Player’ before initialization: It’s explicative, telling us that some code is trying to access the ‘Player’ variable before it’s been completely set up.
– Common cause – Accessing a variable before it’s defined: The JavaScript interpreter reads code from top to bottom. If a piece of code tries to interact with a variable before it’s declared or initialized (i.e., assigned a value), you’ll encounter this error.
– Possible solution – Ensure the variable is defined before it is used: To solve this issue, you need to ensure that variables are properly initialized before they are referenced in the code. This often means rearranging your code so that variable declarations and initializations happen before the variables are used.
As Douglas Crockford, a prominent figure in the JavaScript community, once said, “Programming is not about typing, it’s about thinking.” Thus, understanding errors such as these can help shape our problem-solving skills as developers, ultimately aiding us in writing efficient and error-free code. For more information on JavaScript errors and debugging, you can check Mozilla Developer Network’s guide on the same.
Understanding the ‘ReferenceError: Cannot Access Player Before Initialization’ Issue
The `ReferenceError: Cannot access ‘Player’ before initialization` is a common JavaScript error that occurs when you attempt to use a variable before it has been declared or initialized. This message details how and why the problem can occur, techniques for avoiding the issue, and solutions for handling this type of error.
Whenever we write JavaScript code, each variable has its specific scope from where it can be accessed. Using a variable before it’s declared or initialized results in a `ReferenceError`.
An example scenario where the above error occurs is as follows:
html
This results in the error: `ReferenceError: Cannot access ‘Player’ before initialization`, because JavaScript’s temporal dead zone (TDZ) gets triggered. The TDZ is a behavior of `let` and `const` variables, which are not hoisted. In contrast, variables declared with `var` wouldn’t throw an error, as they’re hoisted and automatically initialized with `undefined` if not assigned any value.
Understanding and following the concept of “variable hoisting” could help avoid errors related to accessing variables before initialization.
– Variable hoisting: JavaScript’s default behavior of moving declarations to the top. But only the declaration gets hoisted, not initialization.
– Hence, always declare and initialize your variables at the top of your script or function to ensure they exist before you try to use them.
To address the issue in the example, simply place the `Player` variable definition before it’s used:
html
However, sometimes it’s not always feasible to rearrange your code to avoid such issues. In those cases, consider utilizing JavaScript’s `typeof` operator:
html
The `typeof` operator checks whether the variable exists before trying to access it, which helps avoid unintended ReferenceErrors.
Avoiding this problem requires a solid understanding of how JavaScript handles variable declaration, initialization and hoisting. As Douglas Crockford, a renowned JavaScript expert, once said: “JavaScript is the only language that people feel they don’t need to learn before they start using.”
Strategies to Avoid ReferenceErrors in Code Execution
When dealing with JavaScript and encountering an error like
ReferenceError: Cannot access 'player' before initialization
, it can interrupt the workflow and cause confusion. It’s a common error that arises when variable declarations are hoisted to the top of their enclosing function, while their initializations stay where they are. This misalignment triggers the ReferenceError; however, there are strategies to avoid this.
Understanding Hoisting:
Hoisting is a behavior in JavaScript where variable and function declarations are moved to the top of their containing scope during the compilation phase. However, only declarations are hoisted, not initializations. Therefore a variable that’s used before declaration will result in
ReferenceError: Cannot access 'player' before initialization
.“One of the most common error with hoisting is using a variable before it’s declared” – Kyle Simpson, author of the “You Don’t Know JS” book series.
Strategies to Avoid the Error:
Strategy | Description |
---|---|
Declare Before Use | Avoid using any variables before declaring them. Ensure that any variable you’re going to use is declared at the beginning of your code block or function. |
Let and Const | Use
let or const for variable declaration instead of var . Unlike var , these keywords do not hoist the variable to the top, which reinforces the practice of declaring variables before use and thus helps in avoiding this error. |
Strict Mode | Enable strict mode by adding
'use strict' at the top of your JavaScript file or function. This forces you to declare all variables, which keeps coding practices clean and prevents errors from hoisting. |
Real-life Scenario:
Let’s consider a real-life example where ‘player’ is used before it’s initialized:
function game() { console.log(player); let player = "John"; } game() //ReferenceError: cannot access 'player' before initialization
In the above code snippet, ‘player’ is referenced before it is initialized with “John” causing the ReferenceError. To avoid this error, move the player declaration and initialization before its first usage as follows:
function game() { let player = "John"; console.log(player); } game() //John
This now works without triggering any ReferenceErrors, demonstrating the effectiveness of declaring variables before use.
Utilizing these strategies can drastically reduce the likelihood of encountering the error:
ReferenceError: Cannot access 'player' before initialization
. Ensuring to always adhere to good programming standards will not only improve the quality of your code but also eliminate potential headaches caused due to unpredictable JavaScript behaviors such as hoisting.
You can read more about JavaScript hoisting from the Mozilla Developer Network documents (MDN).
Exploring Common Triggers for ‘Cannot Access Player Before Initialization’ Error
The ‘Cannot Access Player Before Initialization’ error, also known as the ‘ReferenceError: Cannot access ‘variable’ before initialization’, is a common predicament among developers dealing with JavaScript. This error often transpires when developers try to work with variables that are yet to be initialized. However, rectifying this specific issue is straightforward when we understand its root cause.
Root Cause of The Error
Essentially, the problem behind this is the concept of ‘Temporal dead zone.’ It is a period between entering scope (when the variable gets created) and getting to the line where declaration happens. Within this period, if we attempt to access or interact with the variable, we encounter the ‘ReferenceError – Cannot access player before initialization’ error. Here’s a rudimentary example of this:
let players = ['John', 'Alex']; for(let player of players){ console.log(player); let order = "PlayerNotFound"; if(order === 'PlayerNotFound'){ console.log(player); } }
In this case, JavaScript enters the block with orders not initialized and encounters a `let` order while trying to access it before the initialization. Hence, it triggers an error.
Solving the Issue
Overcoming this issue involves two main approaches:
1. Switching to use of `var` instead of `let` and `const`. Although using `var` might circumvent the problem, it can potentially create issues with variable hoisting and confuse the code’s overall meaning in a broader context.
1. Ensuring that every instance of a variable is initialized before it is called upon, thereby avoiding the ‘temporal dead zone’. Regularly initializing each variable first is typically the best practice, especially when you’re working with teams.
Consider this adjusted version of the previous code snippet:
let players = ['John', 'Alex']; for(let player of players){ let order = 'player'; console.log(player); if(order === 'PlayerNotFound'){ console.log(player); } }
In this revised example, we’ve initialized `order` before using it in a `console.log()`, thus circumventing the error.
Remember, as Douglas Crockford, the creator of JSON pointed out: “JavaScript is the world’s most misunderstood language.” But understanding its quirks and features just make us better developers. Adopting disciplined coding practices will help you to minimize bugs and efficiently handle exceptions like ‘ReferenceError: Cannot access player before initialization’.
For additional insights regarding ‘Temporal Dead Zone,’ refer to this online resource.
Taking into account these considerations can prevent your JavaScript from throwing ‘Cannot Access Player Before Initialization’ error, thereby making your code more robust and less susceptible to potential issues in future applications or during maintenance.
Troubleshooting Steps To Resolve ‘Cannot Access Player Before Initialization’ Error
The ‘Cannot Access Player Before Initialization’ Error is a common issue encountered by many JavaScript developers. This error typically occurs when you try to reference or use a player object before it has been fully initialized in your code. This issue falls under the JavaScript ReferenceError category, which signifies that an attempt is being made to access a variable that does not exist.
Properly troubleshooting this error involves several major steps:
– **Understand the Timing of Initialization**: JavaScript is an asynchronous language, which means that not all code runs sequentially. As a result, you may run into situations where you’re trying to access a player object before it’s completely loaded. The solution is to ensure that your code referencing the player only runs after the player has indeed been created and initialized. You can often manage these dependencies using callbacks or Promises.
HTML
// Call the function only after the Player is finished loading.
Player.onload = function() {
playSong();
};
– **Look at the Scope**: If the player object exists but is defined within the scope of a different function or block, then trying to access it from outside that scope will result in this error. It’s essential to pay attention to where your variables are declared and where they are accessed.
HTML
{
let Player = new PlayerInstance();
// The player is accessible here.
}
// Outside the block, the player is uninitialized.
playSong(Player);
– **Correct Syntax and Spelling Errors**: Among the most basic causes for the ‘Cannot Access Player Before Initialization’ Error are syntax errors or spelling mistakes in your code. Always double-check the names you use for your variables against their actual definitions. This measure helps to avoid any typographical errors causing such initialization issues.
– **Debug Your Code**: Use a debugging tool to inspect your code and identify where exactly the error is originating. Most modern web browsers come with built-in developer tools that allow you to step through your code line by line, watching how variables and values change.
“Talk is cheap. Show me the code.” – Linus Torvalds
– **Referencing Objects Properly**: When referencing objects or properties, ensure that they exist before you attempt to access them. If an object is not yet defined, attempting to call a property on it results in a ReferenceError.
HTML
let Player;
if(Player){
// The player exists, safe to use.
Player.play();
}else{
// Player is not initialized. Do something else.
}
In summary, resolving the ‘Cannot Access Player Before Initialization’ error requires understanding the nuances of JavaScript scope, timing issues with asynchronous loading, correct syntax, and debugging practices. By keeping these factors in mind, you can smoothly code while minimizing such initialization issues.
The “ReferenceError: Cannot access ‘player’ before initialization” is a common error encountered by JavaScript developers. This occurs when you are attempting to use a variable, in this case ‘player’, before it has been adequately defined or initialized within your code block.
This error primarily occurs for two reasons:
* The variable ‘player’ has been declared, but not yet assigned any value.
* The variable is being used before the declaration and initialization, likely due to hoisting. The concept of “hoisting” in JavaScript implies that variable and function declarations are moved to the top of their containing scope during the compile phase, making it seem as if they are accessible anywhere in the code.
An easy way to overcome this issue would be to ensure that every variable you plan on using is declared and initialized before any operations are conducted with it.
Take a look at this incorrect usage example:
html
console.log(player); let player = 'Player Initialized';
In the above example, the ‘console.log()’ statement comes before the variable ‘player’ has been defined, causing the Reference Error.
Here’s how you can correct this scenario:
html
let player = 'Player Initialized'; console.log(player);
In the improved code snippet, the variable ‘player’ is properly initialized before the ‘console.log()’ statement is executed, preventing the reference error.
It’s noteworthy that `Jeff Atwood, co-founder of Stack Overflow`, once mentioned _”…almost all good programmers do coding by making mistakes, learning from those mistakes, and moving on”_(source). Errors such as “ReferenceError: Cannot access ‘player’ before initialization” are part and parcel of the learning curve in the dynamic world of JavaScript programming. Embracing these error messages and learning to navigate them will strengthen your coding skills over time.
The practice of careful variable declaration and initialization aligns well with this approach, ensuring more robust code and fewer run-time errors such as the one discussed.