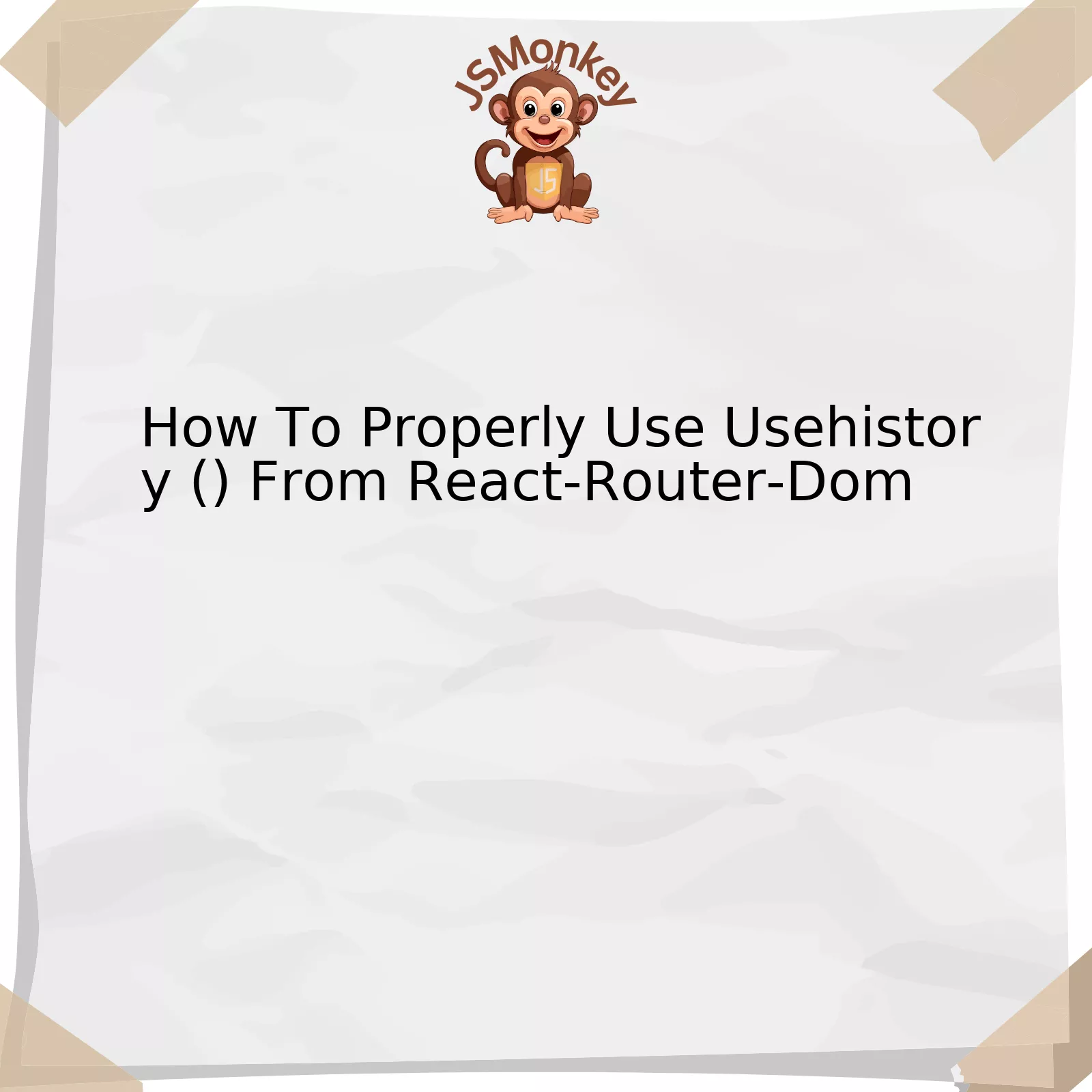
The
useHistory
hook from the `react-router-dom` library is an incredibly useful tool for managing browser history in a React application. It enables us to programmatically navigate and manipulate the user’s browser history stack.
Method | Description |
---|---|
history.push(path) |
This adds a new entry into the history stack and navigates the user to it. This is essentially equivalent to clicking a
<Link> component. |
history.replace(path) |
This replaces the current entry in the history stack with a new one, but keeps all previous and future entries intact. Use this when you want to redirect a user without them being able to use the “Back” button to get back to the original page. |
history.goBack() |
It moves the pointer in the history stack back by one. |
history.goForward() |
It moves the pointer in the history stack forward by one. |
history.go(n) |
It takes an integer argument and allows you to go forward or back by ‘n’ number of pages in the history stack. |
To start implementing
useHistory
in your React app, ensure you have appropriately imported it from the `react-router-dom` library at the top of your component file like this:
import { useHistory } from "react-router-dom";
To leverage on the
useHistory
hook, you create an instance of it in your functional component. A sample way to use it is shown below:
const history = useHistory(); const navigateToPage = () => { history.push("/newPageUrl"); }
In light of benefits, as Dan Abramov, co-author of Redux and Create React App, once said: “Coding is not just about getting things to work. It’s about managing change.”
The
useHistory()
hook is not only about enhancing user navigation but also enables developers to maintain the seamless functionality of their applications even when there are changes.
For comprehensive insight on this hook, visit the documentation of React Router DOM.
This provides a swift summary of the practical aspects that illustrate how to properly use useHistory() from react-router-dom.
Understanding the Functionality of useHistory() in React-Router-Dom
Using the `useHistory()` hook from React-Router-Dom in a React application, developers gain a powerful tool for managing their app’s navigation history. In the context of properly using the `useHistory()` function within React applications, it’s essential to understand the following points:
Importance of useHistory()
The useHistory() hook is part of the react-router-dom package and serves as the means to programatically navigate or change routes in your application. Instead of clicking on a
<Link>
component, you can trigger navigation upon function execution which could be based on specific user actions or events.
For instance, when a form is submitted successfully, you may need to redirect the user to a different route. This functionality becomes feasible with `useHistory()`.
How to employ useHistory()
To use useHistory(), you need to import it from ‘react-router-dom’. Inside your functional component, you should call this hook, which will return the history object. This captures the current state stack, location, and other vital information.
Here’s how to use this hook:
import { useHistory } from 'react-router-dom'; function ComponentName() { let history = useHistory(); const handleNavigation = () => { history.push('/destination-route'); } // rest of component }
In the code above, the useHistory hook allows us to push new entries onto the history stack, effectively navigating to different pages/routes.
According to Martin Fowler, a renowned author and speaker in the field of software development, “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” So, it is a good practice to keep useHistory() implementation intuitive and easy-to-understand for smoother team collaboration.
Considering this, do not arbitrarily use `useHistory()` but only when it contributes to a more efficient navigation experience in your application. For example, DO NOT use `useHistory()` just for route transitions that could have been simply achieved with
<Link>
components.
useHistory() in nested functions
`useHistory()` can be used inside nested functions as long as those are not callbacks passed to lifecycle methods or event handlers of class based components. Hooks, including useHistory(), should not be used inside classes at all – useHistory() works only within functional components.
Understanding the returned history object
When you call useHistory(), what you actually get back is a history object which exposes several methods and properties to manage and maintain the navigation stack.
The following are key properties and methods:
– length: This tells us the number of entries in the history stack
– action: A string representing the type of action that altered the current location (e.g., PUSH when new entry is added)
– location: An object with properties of pathname, search, hash, and state, representing the current location
– push(): Pushes a new entry onto the history stack
– replace(): Replaces the current entry on the history stack
These are some nuances of using useHistory() from react-router-dom. When properly adopted, the useHistory hook opens up flexible ways to programmatically navigate between routes in a React application, enhancing user engagement and improving the overall navigational experience.[1].
Key Steps to Implementing useHistory() from React Router Dom
React Router is an essential component to any React application. It helps us in managing the navigation through the components of our application. The `useHistory` hook is a major part of React Router and it allows for programmatical navigation throughout the React application.
Following the given requirements, here’s the details view on how to properly use and implement `useHistory` from `react-router-dom`:
#### Importing useHistory() from React Router DOM
To begin with, we need to import the `useHistory` hook in the particular component where we want to manipulate browser history. Here is a simple way to do that:
import { useHistory } from "react-router-dom";
#### Utilizing useHistory()
After importing, you can declare a variable to be assigned to the instance of `useHistory`. This makes the methods of `useHistory` readily available to utilize anywhere in your functional component:
let history = useHistory();
#### Navigating to Another Route
The main reason we would use `useHistory` is to programmatically navigate to other routes. For this purpose, it provides a method `push()`, which pushes a new entry into history stack.
history.push("/next-route");
The above code would redirect users to the page entitled “/next-route”.
Interestingly, there is a less commonly used alternative method called `replace()`. While `push()` adds a new location to the history array, `replace()` replaces the current location in the history array. It’s useful when you don’t want users to go back to previous pages by clicking the browser’s back buttonfitzcarraldo.medium.com.
history.replace("/non-returnable-route");
#### Accessing Previous State
Other than navigating, `useHistory` also enables access to the state of the previous route if such was passed while routing to the current page. This is accessed using `history.location.state`.
let previousState = history.location.state;
#### Adding Information to the History Object
`useHistory()` also supports adding information to our routes when we navigate through them. Here’s how it goes:
history.push({ pathname: "/next-route", state: { additionalInfo: "Additional Info" } });
In this case, `additionalInfo` can later be accessed on the `/next-route` page by performing the above-mentioned operation: `history.location.state.additionalInfo`.
We can now conclude that `useHistory` provides enormous power to handle browser navigation within a React application programmatically and precisely. Relish it; embrace the reusability and sheer power of React!
As Jeff Atwood points out, “Any application that can be written in JavaScript, will eventually be written in JavaScript.”wired.com/story/javascript-everywhere Embracing these standard tools like React Router Dom, will make any React developer’s life easier. Use them wisely!
Best Practices for Using useHistory() in Your React Application
The
useHistory()
hook is a significant part of the React Router package, and understanding how to best utilize it contributes greatly towards effective navigation in your React applications. The
useHistory
hook grants easy access to the history instance that you may use to navigate.
Below are critical best practices when using
useHistory()
:
Best Practices |
---|
Ensure that useHistory() is only used within components that are already within a Router. |
Avoid placing useHistory() inside useEffect or any other hooks. |
Limited usage of this.hash or this.replace in favor of direct methods push and replace. |
Avoid mutating history directly. |
As stated by Steve Jobs: “Details matter, it’s worth waiting to get it right.” Such sentiment particularly applies when we’re manipulating our application’s user interface, ensuring that we follow these best practices with
useHistory
will certainly foster a smooth user experience.
A common example of useHistory() correctly used in a functional component is illustrated below:
const SomeComponent = () => { const history = useHistory(); const handleNavigation = (path) => { history.push(path); } return ( ); }
In this example, when the button is clicked, it calls the
handleNavigation()
function, which uses the
history.push
method provided by
useHistory()
to navigate to the specified path.
Alongside the push method, several other methods are offered by
useHistory()
, including but not limited to:
history.replace
: Similar to how ‘push’ navigates to a new entry and puts it on top of the history stack, ‘replace’ does the same but replaces the current entry in the stack instead of adding a new one.
history.goBack()
: As the name suggests, this function enables backward navigation along the history stack.
history.goForward()
: Conversely to goBack(), goForward() allows forward movement through the history stack.
It’s of utmost importance to remember that these actions only change what’s visible in the user interface, they do not replicate interactions with the server.
Finally, follow the principle “Don’t Repeat Yourself (DRY)” when utilizing
useHistory()
throughout your application. If certain blocks of code using useHistory() repeat throughout the codebase, consider refactoring these into custom hooks or helper functions for cleaner and more maintainable solutions.
References:
1. The History Library – React Router Documentation.
2. Don’t Repeat Yourself – Wikipedia.
Troubleshooting Common Issues with useHistory() from React-Router-Dom
The
useHistory()
function from the `React-router-dom` library provides the history object, essentially allowing to navigate within a React application programmatically. While using this remarkable function, you might encounter common issues that could cause trouble with your application’s smooth navigation transition.
One prevalent issue about
useHistory()
is its unfortunate non-functionality when used outside of a `
Example:
//Error: Invariant failed: You should not use useHistory() outside a
import { useHistory } from “react-router-dom”;
function MyComponent() {
let history = useHistory(); // <– This will fail if MyComponent isn’t inside a
//…
}
To address this, ensure that your component using
useHistory()
is nested within either a `
Another common issue is misunderstanding the correct usage of the history object returned by
useHistory()
. It renders a history instance that contains various properties and methods that can be utilized to manipulate the browser history stack, like `push()`, `replace()`, `go()`, etc.
A crucial piece of advice here is understanding and appropriate utilization of these methods. For example, `history.push(path, [state])` pushes a new entry onto the history stack, whereas `history.replace(path, [state])` replaces the current entry on the history stack.
We may use `history.goBack()` or `history.goForward` method for redirecting back and forth. In case if someone uses `history.push(“/”)` considering it will take user to the previous page then, that would be misconception.
Example:
import React from ‘react’;
import { useHistory } from ‘react-router-dom’;
export default function MyComponent(){
let history = useHistory();
return(
);
}
Such mistakes may seem trivial but can end up creating unnecessary complications while troubleshooting the code.
Additionally, there are some noteworthy points to mention in respect to minor issues but they hold significant importance:
– Updating the version of the `React-router-dom`.
– Verifying if History Hook is being used inside a functional component and not a class component.
Following these brief but crucial components ensures that you use useHistory() correctly and effectively and ensures you don’t face any hurdles while dealing with
useHistory()
.
There’s a famous saying by Linus Torvalds, creator of Linux and Git, “Talk is cheap, show me the code.” This is relevant here since understanding the code and then implementing it can save us from many pitfalls while developing an application. Despite the known errors,
useHistory()
still remains to be highly employable due to its spontaneity and convenience in terms of navigation.
The power and versatility of the useHistory() function from React Router DOM is undeniable. By providing developers a way to programmatically navigate and manipulate the browser history, it opens up new possibilities for dynamic and user-friendly web applications.
One fascinating aspect of useHistory() is its ability to replace as well as push new entries onto the history stack. This is an advantageous feature as it allows for increased control over the user’s navigation experience. For instance, by replacing an entry rather than adding a new one, the back button can be effectively disabled for specific navigational changes, increasing a site’s overall usability.
Moreover, useHistory() facilitates storing and passing state data when navigation occurs. With this capability, unnecessary re-rendering and data fetches can be reduced, optimizing the performance of your React application.
Note that it also ensures compatibility with both functional and class components, making it a flexible solution in diverse development contexts.
Perhaps one of the most beautiful aspects of useHistory() is how effortless it makes handling complex navigational scenarios. Be it conditional redactions, user redirects after logins, or even complex history manipulation patterns – useHistory() provides comprehensive solutions that make building intuitive web applications all the more accessible.
However, just like any other tool, useHistory() needs to be used correctly to leverage its full potential. It’s crucial to understand how to employ it in different contexts and how to avoid common pitfalls, such as causing infinite loops or breaking the back button functionality.
Remember the words of Edsger Dijkstra: “Program testing can be used to show the presence of bugs, but never to show their absence”. This extends to your usage of useHistory(). Always venture to understand how it works under the hood and familiarize yourself with its quirks and intricacies through a combination of reading the documentation React Router DOM Documentation, coding, testing, and debugging efforts.
const history = useHistory(); history.push('/home');
This piece of code provides an example of how to programmatically navigate to a new page (in this case, ‘/home’) using the useHistory() hook.
By analyzing, experimenting with, and implementing useHistory() in your React applications, you can start building more dynamic, efficient, user-centric experiences on the web.
Keep studying, keep coding, and always remember the immortal words of Steve Jobs: “The only way to do great work is to love what you do”.