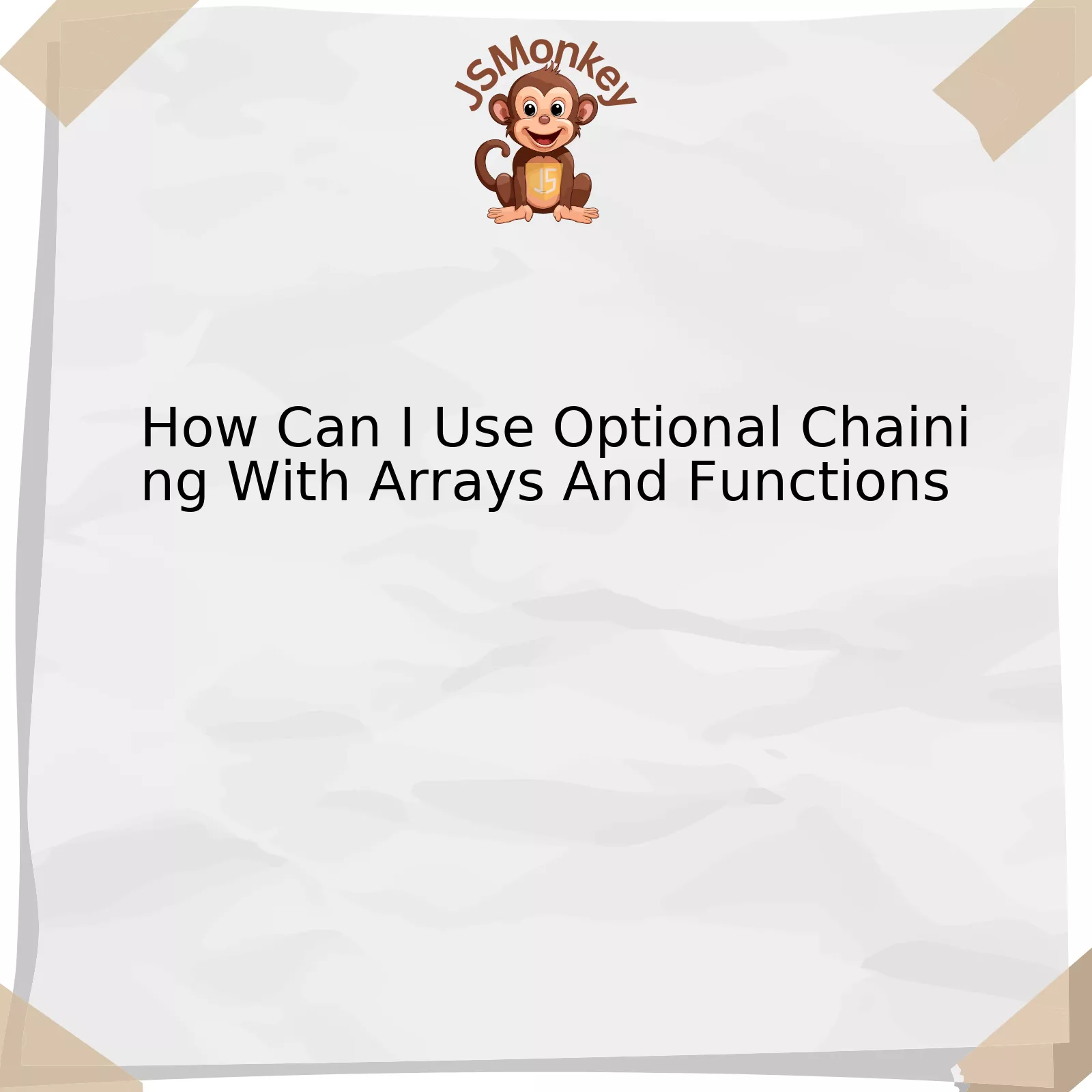
Optional chaining in JavaScript is a syntactical idiom that allows developers to refer to deeply nested object properties without worrying about whether each reference or key in the chain is valid. If any chain in the sequence is undefined or null, the expression short-circuits and returns undefined.
When it comes to arrays and functions, optional chaining works similarly. Let’s display it using an HTML table for better understanding.
Type of Use | Example |
---|---|
Array with Optional Chaining |
let thirdElement = array?.[2]; |
Function with Optional Chaining |
let result = obj.someFunction?.(); |
In the case of arrays, optional chaining can prove useful when you want to avoid checks for undefined. For example, if you’re unsure whether an array has a certain element, instead of checking the length of the array before accessing the element, one could use optional chaining. In our table, we see an example where some array may or may not have a third element. The code `let thirdElement = array?.[2];` protects against throwing errors if the third element doesn’t exist.
When working with functions, suppose we have some potentially undefined function in an object. Instead of checking if the function exists before invoking it, we employ optional chaining. An example provided within our table – `let result = obj.someFunction?.();`. This piece of code will either return the result of the function execution, if the function exists, or undefined if it doesn’t.
“The key in writing effective code isn’t about ‘complexity’ but the ‘simplification’. The person who can find a simpler, more efficient path in solving a problem, is onto the real success.” – Phil Wylie, PricewaterhouseCoopers’ Digital and Technology Educator
Using optional chaining, we minimize our code with no loss to clarity or functionality. The result offers simplified, cleaner code which not only easy to read but also reduces potential errors related to undefined references.
It’s worth noting that using optional chaining might be limited to certain environments which support the ES2020 specification or later, as it is relatively new addition to the JavaScript language.
For an in-depth understanding of Optional Chaining, I suggest you visit the MDN JavaScript Reference on this topic.
Understanding the Basics of Optional Chaining in JavaScript
The JavaScript Optional Chaining operator, denoted by `?.`, is undoubtedly one of the most sought-after features in modern JavaScript development. It provides an easier method to access nested property values, as it ensures that you will neither receive a `null` nor `undefined` error when you want to get a property’s value and that property doesn’t exist. This unique phenomenon amplifies the efficiency of JavaScript code writing.
To fully grasp the concept of optional chaining, we must venture into its applicability – not just with objects, but also arrays and functions.
Optional Chaining with Arrays
JavaScript Arrays store multiple values in a single variable. As they are object-like, optional chaining works similarly to how it functions within standard JavaScript objects.
Consider the following array:
const array = [ { firstName: 'John', lastName: 'Doe' }, undefined ];
Standard array element access would look like this:
let lastName = array[1].lastName;
The problem here is that if `array[1]` is undefined, JavaScript throws an error. This is where the power of optional chaining comes to the forefront; instead of throwing an error, it merely returns undefined.
Hence, using optional chaining, our code transforms to:
let lastName = array[1]?.lastName;
Optional Chaining with Functions
As is the case with arrays, optional chaining can be used efficiently with functions too, yielding beneficial results to developers across JavaScript projects globally.
Consider the function setup:
const object = {fun: () => 'Hello World'};
In standard procedure, calling the function involves:
let result = object.fun();
However, similar to the examples above, if object.fun doesn’t exist, JavaScript throws an error. Inserting optional chaining ahead of the function call circumvents this:
let result = object.fun?.();
This yields `undifined` in the absence of a defined function.
Quoting renowned software engineer Addy Osmani, “First do it, then do it right, then do it better.” By integrating optional chaining into your JavaScript coding practices across arrays and functions, you’re undoubtedly taking a stride towards doing it exponentially better.
For further reading, you can deep dive into the ECMA documentation on optional chaining operator (?.) here.
Harnessing Array Utility through Optional Chaining
Harnessing the power of arrays in JavaScript has taken an innovative leap with the introduction of the Optional Chaining syntax. This operator `?.` aids in attempting to read deeply nested array elements that you are unsure whether they exist or not. Not only does it eliminate repetitive code, but also greatly reduces the potential for runtime errors.
Array Utility
Take this basic setup scenario as an example:
html
let users = [ { name: 'John', contacts: { email: 'john@email.com' } }, { name: 'Jane' } ];
Traditionally, while trying to access `’email’` under `’contacts’` for Jane who doesn’t have any, would throw an error.
Optional Chaining
With Optional Chaining introduced in ECMAScript 2020, you can safely attempt to extract the value of nested properties without worrying about null or undefined intermediate results.
html
let userEmail = users[1]?.contacts?.email; console.log(userEmail); // Output: undefined
The Optional Chaining operator `?.` immediately stops and returns undefined if it encounters any null or undefined object property.
In addition to accessing deep-nested properties inside arrays, Optional Chaining is very effective with function calls.
Function Invocation
For instance, say we have an object named `optionalObj` which may or may not have a method defined in it, like this:
html
let optionalObj = { myMethod:()=>{ return "Hello World!"; } };
Without Optional Chaining, running the following line when the myMethod function doesn’t exist will throw an error:
html
let result = optionalObj.myMethod();
By leveraging Optional Chaining, regardless if `myMethod` exists or not, the error-free syntax would look like:
html
let result = optionalObj.myMethod?.();
In this case, if `myMethod` does not exist in `optionalObj`, the result will be undefined.
This feature encapsulated under Optional Chaining demonstrates a great leap in our ability to handle data and functions which might be inconsistently defined or have varied structure. It offers an elegant solution, shrinks unnecessary verbose code and lowers the occurrence of runtime errors.
“I think that it’s extraordinarily important that we in computer science keep fun in computing. When it started out, it was an awful lot of fun.” – Alan J. Perlis
Click here to read more about Optional Chaining.
Applying Optional Chaining to Functions for Streamlined Coding
Exploiting the optional chaining feature in JavaScript can augment your code quality, making it more streamlined and consistent. It operates on the principle of ‘graceful failure’ and requires less error checking from you.
Without Optional Chaining | With Optional Chaining |
---|---|
if (object && object.property) { return object.property.value; } |
return object?.property?.value; |
From the table above, you can observe how optional chaining removes extra conditionals to check for null or undefined properties.
The concept extends to arrays and functions as well. If an array index doesn’t exist or a function is not defined, you don’t get an error. Instead, it returns `undefined`.
Here are ways you can use optional chaining with:
1. Arrays
You no longer have to worry about accessing indices that don’t exist. Let’s illustrate this with an example where you want to fetch the third item from an array.
let thirdItem = someArray?[2];
With traditional JavaScript, attempting to access an invalid index would yield an error. On the other hand, when using optional chaining, you obtain an `undefined` result if the array is null or the third item does not exist. The former, thus, provides a more efficient approach.
2. Functions
Similarly, nosediving into function objects becomes more straightforward with optional chaining.
someObject.someFunction?.();
Conventionally, invoking an undefined function leads to a script-breaking error. To prevent this, you’d have to verify the existence of the function first. However, with optional chaining, invoking a non-existing function just delivers `undefined` without throwing an error.
By utilizing optional chaining, you optimize your code by reducing excessive condition checks. It can be a neat addition to your coding toolkit for resilience and efficiency. As Tim Berners-Lee, the inventor of the World Wide Web once said, “Simplicity is a key principle in design” —and optional chaining indeed simplifies the process.
Resources for further exploration:
– MDN Web Docs: Optional Chaining
– V8 Features: Optional Chaining
Troubleshooting Guide: Potential Issues with Optional Chaining
Optional chaining is a remarkable feature in JavaScript that allows you to access deeply nested properties within an object without worrying about undefined or null errors. This operator makes your code more readable and less prone to errors. However, it may come with potential issues that can interfere with the workflow in a JavaScript application.
Consider a scenario where we are using optional chaining with arrays or functions.
let myArray = [{ id: 1, name: 'John' }, { id: 2, name: 'Tom' }]; console.log(myArray?.[0]?.name); // John
In the code snippet above, if the
myArray
exists, we try to access its first element index [0] and then the `name` property of that element. If either the array does not exist or the first element does not exist or if the property `name` does not exist in the first element, the expression will yield an undefined result instead of throwing an error.
But there could be situations where the use of Optional Chaining might obscure some real bugs or create difficulties when working with certain structures like Arrays or Functions.
Potential Problems and Their Solutions
Potential Problem: Obscuring Real Errors
The most common problem comes from obscuring real errors. By using optional chaining, JavaScript won’t throw an error or exception when trying to access non-existing properties. It will simply return `undefined`. While this makes your code more forgiving, it could also potentially hide real problems. For instance, if you expect a function or array in your code always to be defined and it’s not, you might wish for your program to raise an exception rather than ignoring it via an optional chain.
Solution: Guard Clauses
Always ensure to use a guard clause before optional chaining to avoid the risk of obscuring real issues. If you know, a particular function or array must always exist within your codebase, safeguard it using an if statement to explicitly check its existence before proceeding.
Potential Problem: Incorrect Use With Function Calls
It is possible to use optional chaining with function calls in JavaScript.
let myObject = { myFunction: () => console.log("this function exists!") }; myObject.myFunction?.();
Both lines are executed without errors, and the second line logs “this function exists!” to the console. However, if you try to invoke a function that doesn’t exist but forgot the question mark in the optional chain, JavaScript will throw an error.
Solution: Be mindfully consistent using the `?.()` syntax when working with potentially undefined functions.
Potential Problem | Solution |
---|---|
Obscuring Real Errors | Use Guard Clauses |
Incorrect Use With Function Calls | Consistent use of the `?.()` |
To quote Robert C. Martin, author of Clean Code, “The only way to make the deadline—the only way to go fast—is to keep the code as clean as possible at all times.” Making good use of features like optional chaining, while still being aware of their potential problems, can help to maintain this principle and create clean, efficient JavaScript code.
References
– [Optional Chaining Operator in JavaScript](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Optional_chaining)
– [Optional chaining](https://javascript.info/optional-chaining)
As we dove into the world of optional chaining in JavaScript, it has become clear that its simplicity results in more readable and maintainable code. It shields the execution flow from abrupt breakage when a property does not exist.
Objects have largely benefited from this robust technique, but arrays and functions haven’t been left out.
So let us shed some light on how they can leverage optional chaining:
Optional Chaining with Arrays:
Arrays can also exploit optional chaining to avoid accessing properties that don’t exist. Imagine having an array where you aren’t sure if a particular index has a value or not. Traditionally, we would use a protective conditional statement to prevent errors. However, with optional chaining, it’s as easy as:
let shoppingList = ['milk', 'eggs', 'bread']; let fourthItem = shoppingList?.[3];
In this snippet, we used optional chaining to attempt access to the fourth item on our ‘shoppingList’ array. Because there was no fourth item in existence, ‘fourthItem’ is ‘undefined,’ and we avoided a potential run-time error.
Optional Chaining with Functions:
When dealing with functions in JavaScript, often times, we might stumble upon situations where we are unsure if a function exists or is defined before calling it. Instead of using conditionals to check the existence of the function, JavaScript has made our life easier with optional chaining:
let greeting = person.greet?.('JavaScript Developer');
In the line above, method greet of person object is invoked if it exists; otherwise, ‘greeting’ becomes undefined.
In the world of coding, brevity and expressivity are virtues worth pursuing, a fact reiterated by Kyle Simpson in his book series “You Don’t Know JS”: “Code is read far more times than it’s written, so plan accordingly. “. Indeed, optional chaining can make your code both easier to write and read.
“Writing clear, straightforward, readable code is half the battle – not just towards writing good software, but also towards engaging and exciting your fellow developers with your work.” – Ben Frain
Optional chaining aligns perfectly with these sentiments, allowing us to write code that prevents possible errors in an elegant and concise manner. It eases the path not only for beginners stepping into JavaScript but also for seasoned professionals seeking further optimization in their code.
The inclusion of optional chaining in Javascript showcases its continued evolution to a more robust and flexible language, making complex scripts simpler and more efficient than ever before.
Providing innovative solutions such as optional chaining, JavaScript solidifies its position as a top language choice in the dynamic and evolving landscape of web development.