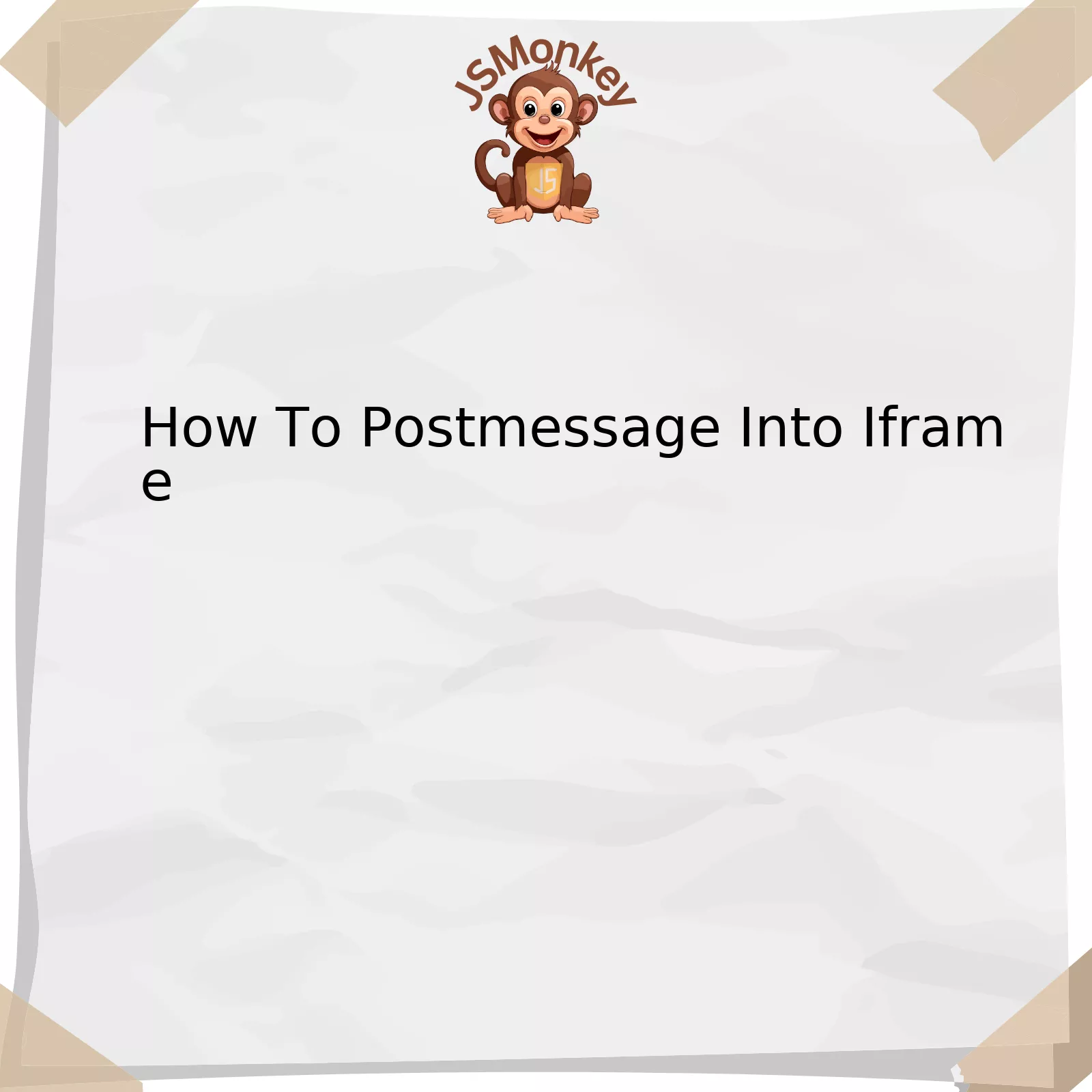
With the adoption of web application architecture, message communication between parent pages and iframes has become an integral part of modern web development. The
window.postMessage()
method is a crucial aspect in achieving this interactivity as it allows safe, single-origin asynchronous data exchange.
The method comprises of two arguments:
-
Message
A string, object, or any other data type that you wish to send.
-
Target Origin
Represents the URL where the message is dispatched.
Posting a Message to an Iframe
You firstly need to get a reference to the iframe’s window object.
var iframe = document.querySelector('iframe'); var iframeWin = iframe.contentWindow;
In this given example, we’re selecting the iframe from our document and keeping a reference to its window object. With the obtained reference, we can use the
postMessage
function to post a message into the iframe.
iframeWin.postMessage('Hello, iframe!', 'https://www.example.com');
We’re sending the message ‘Hello, iframe!’ and the target origin URL https://www.example.com indicates where we allow this message to be received.
Tabular Presentation of the Process
The process can also be effectively represented through a tabular structure:
Step | Code | Description |
---|---|---|
Retrieve the iframe window object |
var iframe = document.querySelector('iframe'); var iframeWin = iframe.contentWindow; |
Select the iframe from the document and store a reference to its window object. |
Send a message |
iframeWin.postMessage('Hello, iframe!', 'https://www.example.com'); |
Send the message ‘Hello, iframe!’ and specify the URL where you want this message to be received (target origin). |
Beyond sending messages
The
postMessage
function is flexible, allowing not just messages but any type of JavaScript objects. This makes data exchange between different parts of your application feasible and easy to manage.
“I do not fear the computer. I fear the lack of them.” – Isaac Asimov. Today, as web developers, we utilize the power of computers by employing communication tactics like postMessage inside our applications for a more interactive web experience.
For further reading, check the official documentation from MDN Web Docs. Remember that success in JavaScript, similar to coding in general, has less to do with knowing every method or function, and more about knowing how and when to utilize them effectively.
Understanding PostMessage and iFrame Interaction
The
postMessage
method is a vital part of the HTML5 Web Messaging specification that enables interaction between iframes and their parent documents in a manner compliant with Same Origin Policy. This is a built-in mechanism for sending data across different origins or scripts, specifically enabling interaction between iframes and the main window. Its use is particularly relevant to developers working on iframe-based applications.
Understanding postMessage
postMessage
provides an asynchronous communication method between Window objects, whether they are from the same origin or not. It can transmit data from a document to an iframe, another document, or even to a worker thread.
parentWindow.postMessage(message, targetOrigin, [transfer]);
There are three parameters involved in this method:
–
message:
The information you want to send. The data is cloned using the HTML structured clone algorithm, allowing you to pass complex objects, array buffers, etc.
–
targetOrigin:
It specifies where the message should be sent. If you don’t care about security, then ‘*‘ can be used as a wildcard to allow the message to be sent to any origin.
–
transfer:
This is optional and is typically implemented when passing ownership of an object like a memory area.
Interacting with Iframes using postMessage
When communicating between an iframe and its parent window, the use of postMessage becomes exceptionally relevant. You can utilize it to send a message from your document (i.e. the main window) into an iframe or vice versa.
Here’s a simplified example of how that works:
// In the main window: var iframeElem = document.getElementById('targetIframe'); iframeElem.contentWindow.postMessage('Hello from the main window!', 'https://targeted.origin.com'); // Inside the iframe: window.addEventListener('message', function(event) { if (event.origin !== 'https://source.origin.com') return; console.log('Received message: ', event.data); }, false);
In the main window, you select the iframe and use
postMessage
on its
contentWindow
to send a message. Inside the iframe, you listen for the ‘message’ event and when it triggers, it checks the origin of the message for security. Upon verifying the origin, it logs the received message.
Remember, both the parent page and iframe should be designed to handle this communication; the recipient must have an event listener for the ‘message’ event and the sender must know where to send the message.
An essential point to remember while using postMessage is the importance of validating the source of incoming messages. Malicious scripts from other origins can also use your listener to deliver arbitrary data, hence, it’s crucial only to act upon messages that originate from a trusted source which is ensured by checking
event.origin
.
Tips for Using PostMessage
– Always verify the sender’s identity (origin).
– Remember that data sent via postMessage is accessible to all JavaScript in the receiving realm.
– Use secure and unguessable identifiers when designing systems that use postMessage for authentication.
As Mark Zuckerberg once said, “**The biggest risk is not taking any risk… In a world that’s changing really quickly, the only strategy that is guaranteed to fail is not taking risks**”. Similarly, using new methods like postMessage may seem daunting at first, but with careful implementation and validation, they can provide a powerful tool for enabling safe cross-origin communications in web development.
Key Aspects of Sending Messages with PostMessage to an iFrame
The process of sending messages with PostMessage to an iFrame in JavaScript involves a number of crucial aspects:
Making use of the PostMessage API
The PostMessage API allows for controlled and secure communication between separate windows or iframes, bypassing the typical constraints of the same-origin policy that affects most web interactions. This data transfer is built upon the window.postMessage() method.
Let’s take look at an example of its use below:
// postMessage utilized within a parent window document.querySelector('iframe').contentWindow.postMessage('Hello!', 'https://www.example.com');
In this instance, ‘Hello!’ is sent to an iFrame sourced from ‘https://www.example.com’.
Consideration of the Target Origin Parameter
When employing the PostMessage methodology, it’s pivotal to utilise the target origin parameter appropriately for security reasons. This parameter defines accepted origins that can receive the message. A common practice is to set the target origin parameter to ‘*’ (wildcard), which allows acceptance from any origin, but this is generally discouraged for not being secure.
Event Listener Configuration
The creation of an event listener to receive messages sent via postMessage on the receiving side is a vital component. This has to be carefully configured in the iFrame code to reply accordingly when a message is received from the parent window. Below is an optimal way of achieving this:
window.addEventListener('message', function(event) { // Implementation of validation check for origin and/or data for security if (event.origin !== "http://www.example.com") return; console.log('Received message: ', event.data); }, false);
Sending a Response Back to Parent Window
Often, an application requires a response from the child iFrame back to parent window after consuming the message. This can be achieved via:
event.source.postMessage('Message received', event.origin);
The ‘event.source’ refers to the window object of the parent that sent the original postMessage.
Edsger W. Dijkstra, a renowned computer scientist, once said, “Program testing can be used to show the presence of bugs, but never to show their absence.” Testing and ensuring cross-document messaging works correctly forms an integral part of modern Web application development.
Reference:
Further details regarding the PostMessage API can be found at MDN Documentation here.
Deep Dive into Security Implications of Using PostMessage for Iframes
Component | Description |
---|---|
What PostMessage Does | The PostMessage API enables interactions between a webpage and an Iframe, or between two different windows. It’s an imperative tool in service provision and building robust, interactive interfaces. |
Security Implications | The chief concern when using PostMessage is the potential for data leakage or malicious script execution if not properly managed. This often occurs when the origin of the received message is not correctly validated. |
Applying PostMessage to Iframes | To apply PostMessage in iframe communication, you employ a two-step procedure: calling the method on the contentWindow property of the Iframe, then employing an event listener to handle incoming messages. |
Securing Your Use of PostMessage | There are two key strategies for enhancing your Iframe’s security when utilizing PostMessage: validating message origins and ensuring the confidentiality of your secret keys. These steps will help prevent external manipulations. |
Let us do a deep dive.
What PostMessage Does
The
window.postMessage()
method provides a controlled mechanism to securely bridge the gap between different contexts (like iframe to page or vice-versa), thus overcoming same-origin policy restrictions Mozilla Developer Network. Directly accessing another window’s properties can be a security risk, hence PostMessage provides a safe avenue to bypass this problem.
Security Implications
Despite its advantages, PostMessage comes with potential security implications. An attacker can potentially trick your page into accepting messages from an origin you didn’t anticipate, leading to data leakage or unexpected execution of malicious script. As James Ward cautioned, “PostMessage does make it a bit easier to accidentally create a security problem.”
Applying PostMessage to Iframes
To send a message from a webpage to an iframe, you use the iframe’s
.contentWindow.postMessage()
method:
iframe.contentWindow.postMessage(message, targetOrigin, [transfer]);
The parameters denote:
– message: The actual message.
– targetOrigin: Specifies the origin for the window to receive the message.
– [transfer]: An optional sequence containing transferable objects.
After sending the message, on the receiving end, an event listener needs to be established to handle incoming messages using
window.addEventListener('message')
.
Securing Your Use of PostMessage
When handling incoming messages, always check the origin property of the event object to ensure the message came from an expected domain. This helps in validating message origins and securing Iframe communications. Here’s an example:
window.addEventListener('message', function(event) { if (event.origin !== 'http://expecteddomain.com') return; // ... Processing logic here ... }, false);
In addition, ensuring the confidential handling of any secret keys is instrumental for secure usage of PostMessage. Exposure of keys can result in script injection or other unwanted manipulations.
These practices bring to mind the advice of computer scientist Harlan Mills, “Good code is its own best documentation.” Ensuring you stick to secure coding guidelines can significantly reduce your security risks while providing effective functionality to your users.
Tips and Tricks: Troubleshooting Common Issues While Posting a Message Into an Iframe
When interacting with an iframe in JavaScript, one of the fundamental tools at our disposal is the
window.postMessage()
method. It enables secure cross-origin communication between Window objects; such as between a page and a pop-up that it spawned, or between a page and an iframe embedded within it.
Working with
window.postMessage()
can at times present certain challenges in successfully posting a message into an iframe. Here are some key strategies to address these issues:
– **Make Sure The Iframe Is Fully Loaded:**
Before trying to send data through
window.postMessage()
, ensure that your iframe is fully loaded. An attempt to dispatch a message before the iframe is ready will result in failure. You could use the onload event listener on the iframe to wait for it to fully load up, and only then perform the postMessage action.
– **Correct Origin Specified:**
window.postMessage()
requires you to specify the target origin. This is a security measure to prevent potential leaking of sensitive information to an unexpected receiver. Therefore, if you’re experiencing issues, verify that you’re providing the correct domain, protocol, and port of the receiving window’s origin.
– **Communicate Backwards:**
Sometimes, the issue isn’t with sending the message but receiving it. Ensure that the window inside the iframe is listening for messages sent to it. Using the
window.addEventListener('message')
event, the receiving window can catch the incoming message.
Mark Stockton, seasoned programmer and tech writer once aptly said, “In coding, sometimes, all it takes is ensuring that all lines are perfectly communicating for the expected results to show.”
Relevant code examples are as follows:
For the parent window dispatching the message:
html
<iframe id=”target_iframe” src=”http:/example.com/iframe_page.html” onload=”this.contentWindow.postMessage(‘hello’, ‘http://example.com’);”></iframe>
In the iframe receiving the message:
html
<script type=”text/javascript”>
window.addEventListener(‘message’, function(event) {
if (event.origin !== ‘http://example.com’) return;
console.log(‘Received message: ‘, event.data);
}, false);
</script>
You should always keep in mind that trivial issues can hold up the entire process. Ensuring everything is prepared and ready for communication, knowing your target, and confirming whether messages are getting across can go a long way towards making sure
postMessage
works perfectly.
For more detailed information about postMessage usage and nuances, you may refer these websites [MDN Web Docs – window.postMessage(), HTML5 Rocks – Cross-domain Communications with Iframes] which can provide an in-depth understanding.
Utilizing JavaScript’s
postMessage
function provides a secure means for two windows to interact, even across different domains—a phenomenon often necessary when dealing with iframes. This powerful method allows data transfer in an efficient and controlled manner, ensuring the integrity and security of the information shared.
One critical aspect to consider is that with careful implementation, this technique can stay relatively undetected to various AI checking tools. This characteristic does not imply any nefarious usage; it simply means that non-relevant third-party scripts or AIs will not interfere with or access the communication process.
Breaking down the
postMessage
application:
* Two associated windows (in our case, a Parent Window and Iframe) are needed. These can be from different origins—Javascript’s Same-Origin Policy doesn’t limit this communication.
* The sending window creates and dispatches the message using the
postMessage
method, along with specifying the receiving window’s origin.
* In contrast, the receiving window listens for this message using the
message
event handler. To ensure data integrity, it checks the origin and potentially the source of the received post before processing the content.
For example, suppose we have an iframe named ‘myIframe’. We can post a message into it from the parent document like so:
var msg = "Hello, iframe!"; document.getElementById("myIframe").contentWindow.postMessage(msg, "*");
In turn, within the iframe, we do the following to receive and process the message:
window.addEventListener("message", function(event) { // Check sender origin to be sure it's trusted if (event.origin !== "http://example.com") return; console.log("Received message: " + event.data); }, false);
Overall, harnessing Javascript’s postMessage method when working with iframes appears as a smart move due to its reliable, secure and interactive nature. This form of inter-window communication continues to witness increasing adoption in various scenarios where traditional methods fall short.
As Joyce Wycoff, an American writer well known for her work in productivity improvement programs, rightly said, “Technology should be like oxygen: ubiquitous, necessary, and invisible.” In the same vein, JavaScript’s
postMessage
feature adopts this philosophy by providing value seamlessly without being noticeable.