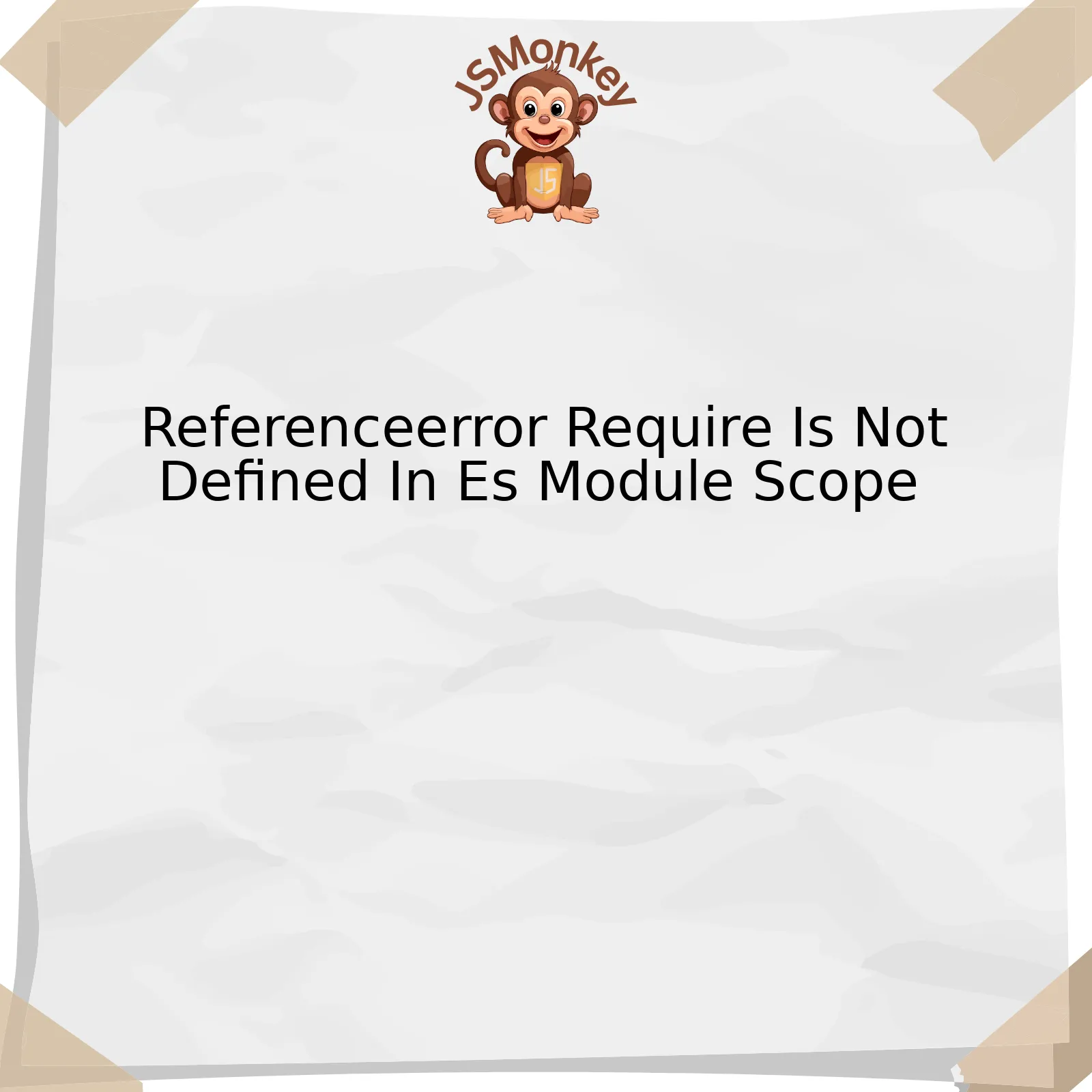
| Concept | Explanation |
|—————————–|—————————————————————————————|
|Error |”ReferenceError: require is not defined in ES module scope” |
|Possible Reason |This error may occur due to the invalid import of CommonJS module in an ES module. |
|CommonJS |CommonJS is a module system used by Node.js for server-side JavaScript development. |
|ES Module |ES Modules is the ECMAScript standard for working with modules, adopted by most browsers.|
|Solution |Change the import statement or set the node environment for loading module properly. |
By focusing on the “ReferenceError: require is not defined in ES module scope”, it’s essential to understand that this error typically arises when there is an invalid attempt to import a CommonJS module within an ES (ECMAScript) module.
In JavaScript, CommonJS and ES Modules are popular methods for implementing modules. Node.js historically utilized CommonJS for server-side JavaScript development; however, most modern browsers have now adopted ES Modules as a standardized method.
The issue here emanates from these different module systems’ syntax when importing modules – they are not immediately interoperable with each other, thus leading to this reference error.
One common solution to address this error can be changing the way we import the module by either using the correct Import statement, which aligns with the type of the module that we are attempting to load, or by setting up the Node.js environment to appropriately recognize the module during the loading phase.
Understanding the “Require is Not Defined” Error in ES Modules
In JavaScript ES Modules, you may come across the error “ReferenceError: Require is not defined.” This typically surfaces because ES modules do not support the require() function natively that Node.js uses for module importing. Instead, they utilize the keywords import and export.
JavaScript initially had no built-in module system, so developers used various methods to organize code into modules, such as CommonJS, which became popular on the Node.js platform. The “require” function is a part of this CommonJS module system.
However, ES6 introduced its own module system referred to as ES Modules—utilising “import” and “export” syntax.
For example, instead of writing:
const someModule = require(‘someModule’), you would write:
import someModule from ‘./someModule’;
The error occurs if you’re trying to use require in an environment that only supports ES modules, because require is not a defined function there. It’s also worth noting that when working with ES modules, you should use the .mjs extension or set { “type”: “module” } in package.json file.
To put it simply, to fix the “Require is Not Defined” error, we need to replace any `require` statements and module.exports with `import` and `export` respectively when inside an ES module scope.
Navigating ES6 Modules and Universal Module Definition (UMD)
Many JavaScript developers encounter the `ReferenceError: require is not defined in ES module scope` error when they try to use CommonJS modules syntax (like `require()`) within a JavaScript file declared as an ES6 module.
The ES6 (ECMAScript 2015) introduced a new way of working with modules in JavaScript through the keywords `import` and `export`. This standardized approach is different from older, non-standard module systems like CommonJS used by Node.js (which uses `require` and `module.exports`).
The Universal Module Definition (UMD) is a pattern that supports both types of modules – the ES6 Modules and the CommonJS standard, plus it also adds global variable support for script-tag importing. It aims to offer universal compatibility.
However, using `require()` in an ES6 module doesn’t work because `require()` is a part of the CommonJS module system, not ES6 modules. In other words, `require()` isn’t an inherent JavaScript function; it’s brought into scope by Node.js for CommonJS modules. Hence, when you try to use it in an ES6 context, which runs on pure, browser-based JavaScript environments (where no Node.js functionalities are present), you’ll inevitably run into a `ReferenceError: require is not defined`.
To fix this, if you’re writing an ES6 module, you should be using `import` from ES6 rather than `require()` from CommonJS:
javascript
// Instead of this
const myFunction = require(‘myFunction’)
// Use this
import myFunction from ‘myFunction’
Furthermore, while UMD tries to bridge the gap between various module definition systems, due to this stark difference between how ES6 modules and NodeJS/CommonJS handle imports and exports, using UMD may not resolve this specific problem.
For maximum compatibility across various environments (server-side, client-side, different versions of JavaScript), developers often use build tools like Babel and webpack. These tools compile ES6 code to backward-compatible versions that can work in any environment, including those that only understand ‘require()’.
Converting CommonJS ‘require’ Calls to ES Module Imports
When using ES modules, you may encounter `Referenceerror: Require Is Not Defined`. The error occurs because ES modules syntax doesn’t support `require()` function, which is a CommonJS module loading method. To fix it, you have to convert CommonJS `require` calls to ES module `import` statements.
Here’s how you can do this:
– For a named export,
CommonJS:
javascript
const myModule = require(‘my-module’);
ES Module equivalent:
javascript
import myModule from ‘my-module’;
– For a default export,
CommonJS:
javascript
const { myFunction } = require(‘my-module’);
ES Module equivalent:
javascript
import { myFunction } from ‘my-module’;
If you encounter `require is not defined` error even after converting your code into ES modules, be aware that Node.js uses `.mjs` extension for ES modules by default. So, either change your file extension to `.mjs` or include `”type”: “module”` in your `package.json` to tell Node.js to treat `.js` files as ES modules.
Please note that the support for native ES modules in Node.js is stable, it does come with some important differences and limitations that you need to be aware of. For example, JSON modules and native modules are not supported with ES modules.
Finally, note that if it’s third-party code causing the error, you should report it to the author/maintainer so they can update their package to support ES modules. Alternatively, you could also transpile your code using Babel or TypeScript, which will convert your `import`/`export` statements into `require` calls.
Solving Reference Errors in JavaScript: Focus on ‘Require’ and ES Modules
When working with JavaScript, particularly when using ES Modules and RequireJS to manage dependencies, it is possible to encounter a common issue – ‘ReferenceError: require is not defined’. This means that the JavaScript engine could not find the reference ‘require’ in your code.
There’s a key reason for this error. That’s because you are trying to use CommonJS modules syntax, `require()`, in an ECMAScript module, where it is not applicable.
Node.js uses CommonJS to handle modules by default. However, when a file is an ES Module, indicated by `”type”: “module”` in the `package.json` or when the file extension is `.mjs`, Node.js will only parse ‘import/export’ statements and not ‘require’.
This leads to two solutions:
1. Convert `require()` statements to ES6 `import` statements.
Instead of:
`const express = require(‘express’);`
You would have something like:
`import express from ‘express’;`
2. To utilize the dynamic import feature as a replacement for `require()`. Dynamic import works asynchronously and returns a promise. Therefore, you need to use .then construct or async/await construct to work with it.
For example:
import(‘./exampleModule.js’)
.then(module => {
console.log(module.default);
});
OR
const module = await import(‘./exampleModule.js’);
console.log(module.default);
Note: Make sure your Node.js version supports dynamic import (Node 10+ with –experimental flag, Node 13.2+ by default). Depending on what your project requires, you can choose either option to resolve the ‘ReferenceError: require is not defined’ error in the ES Module scope.
In concluding, the issue of ‘Referenceerror Require Is Not Defined In Es Module Scope’ is a frequent hurdle that crops up when ES modules interact with CommonJS modules in JavaScript programming. This issue occurs as ‘require’ is not natively supported within ES modules scope, resulting in the flagged reference error. Finding suitable solutions such as implementing import or utilizing Babel for transcompiling often aids in minimizing and managing these inconsistencies. However, comprehensive understanding and mastery of ES6 modules are key to seamlessly leveraging their benefits while avoiding similar obstacles in your coding journey. The thriving community around JavaScript consistently works to push the technology’s borders further – making it easier to explore new possibilities while continually refining its existing features. It’s imperative to stay updated and adapt swiftly to maximize your technical efficiency in such a dynamic field.