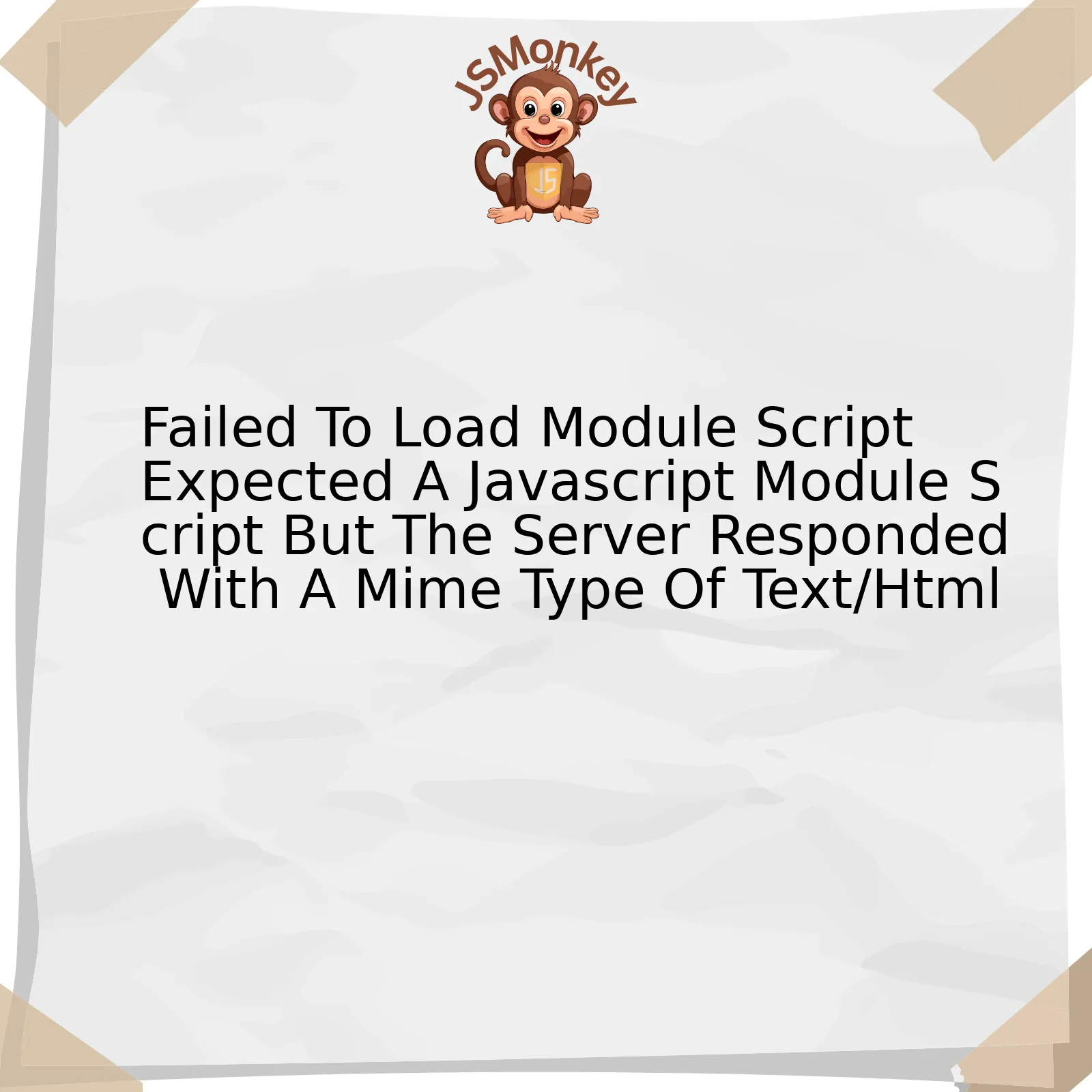
This issue, “Failed to Load Module Script: Expected a JavaScript Module Script but the Server Responded with a MIME Type of text/html,” arises when a browser fails to load a JavaScript module due to an incompatible media type. It is often the result of the server delivering a page with ‘text/html’ content type header rather than the one required for JavaScript modules, ‘application/javascript’.
Let’s look over the key aspects related to this error:
JavaScript Module Script | A modern feature introduced in ECMAScript standard which enables the use of import and export statements to incorporate additional scripts or functionalities within a main script. |
---|---|
MIME Type | A two-part identifier for file formats (like text/html and application/javascript), used by web browsers to understand how to process a received file. |
‘text/html’ MIME Type | The media type typically employed for HTML documents, not suitable for JavaScript modules. |
‘application/javascript’ MIME Type | A Mime type indicative of JavaScript files, which should be used when serving JavaScript module scripts to ensure correct processing by the browser. |
To resolve this error, it is necessary to configure the server settings so that it sends JavaScript module files with the proper ‘application/javascript’ content-type header. This can be done through the .htaccess file or by adjusting server configurations based on the type of server used.
For Instance:
<IfModule mod_headers.c> FilesMatch "\.(m?js)$" Header set Content-Type "application/javascript; charset=UTF-8" </IfModule>
A simple but commonly effective way to fix this issue is to make sure the file being requested exists in the first place. If the targeted JavaScript module file does not exist on the server, a 404 error page will be served, typically with ‘text/html’ MIME type, causing similar symptoms.
As reminded by Douglas Crockford, “Always code as if the person who ends up maintaining your code is a violent psychopath who knows where you live”, so it’s always good to ensure codes are bug-free and efficiently written for better maintainability.
Understanding the “Failed to Load Module Script” Error
The “Failed to Load Module Script: Expected a JavaScript Module script but the server responded with a MIME type of Text/HTML” error happens when a browser attempts to load and execute a JavaScript module, but gets a response from the server with an incorrect MIME type. While the expected MIME type is ‘application/javascript’ in this scenario, encountering a MIME type of ‘text/html’ leads to the error. This is part of the browser’s feature to ensure website security by scrutinizing potential security risks that incompatible or corrupt file types might introduce.
Understanding MIME Types:
MIME (Multipurpose Internet Mail Extensions) are file type identifiers that help the browser understand how to handle different files. The header of HTTP responses typically carries this information. For example, for HTML, it would be ‘text/html,’ while for JavaScript, it should ideally be ‘application/javascript.’
However, if there’s a mismatch between the actual and expected MIME type, it can lead to errors such as the one in question.
Below mentioned are the tips to rectify this problem:
– Double-check Server Settings: Ensure your server is set up to send the correct ‘Content-Type’ headers for .js files. For instance, it should send ‘application/javascript’ for JavaScript files, not ‘text/html’.
// in Apache (.htaccess) AddType application/javascript .js
– Verify File Paths: Make sure the JavaScript file you’re attempting to load exists at the given URL path. Misspelled words or incorrect paths can lead to 404 errors, where our server may fall back on responding with an HTML file, thus resulting in ‘text/html’ MIME type.
– Examine Browser Support: Some older browsers do not support module syntax and, as such, cannot correctly interpret the ‘type=module’ attribute in script tags. Hence, they end up misreading the JavaScript module as text/HTML.
<script type="module" src="/path/to/module.js"></script>
– Check AJAX Calls: If you’re making AJAX calls to fetch JavaScript, ensure that the ‘dataType’ property in your AJAX configuration is set to ‘script’, not ‘html.’
$.ajax({ url: "/path/to/script.js", dataType: "script" });
In the words of Brian Kernighan, a pioneer in computer science, “_Debugging is twice as hard as writing the code in the first place. Therefore, if you write the code as cleverly as possible, you are, by definition, not smart enough to debug it._” So keep your code simple and understandable while working through these potential fixes.
For further insights on this topic, consider referring to various online resources available on JavaScript modules and MIME types.
Resolving MIME Type Mismatch in JavaScript Modules
Resolving MIME type mismatch in JavaScript modules, particularly in instances where a Module script was expected, but the server responded with a MIME type of text/html, involves understanding both the problem and approaches to possible solutions. When this error occurs, it signifies that the client-side (JavaScript) is expecting a different content or file type than what is being supplied by the server.
MIME Type and JavaScript Modules
In the context of web development, MIME types (Multipurpose Internet Mail Extensions) are a standard used to indicate the nature and format of a document transmitted over the internet. These MIME types allow servers to understand how to handle this data effectively. Specifically, for JavaScript modules, the expected MIME type is
application/javascript
.
The Problem
When the server responds with a MIME type of text/html when a JavaScript module is requested, it could be because the web server is not correctly configured to serve JavaScript files. Therefore instead of rendering JavaScript as expected, they’re erroneously treated as HTML files.
Possible Solutions
Several strategies might help tackle this issue:
• Check your file paths: Incorrect file paths might lead to the server serving an HTML 404 error page instead of the expected JS file. This generates a MIME type conflict because this page uses the text/html MIME type.
• Correctly configure your server: The server may be improperly configured to serve .js files with the appropriate MIME type. Depending on the server you use. This configuration varies, but generally, it can be achieved by updating the server’s MIME types settings.
• Set the correct `type` attribute: While linking the JavaScript module, ensure it contains the accurate `type` attribute. For instance, ``.
As Tim Berners-Lee, inventor of the World Wide Web, said: “We need diversity of thought in the world to face new challenges.” The same pertains to error resolution in web development. It’s all about understanding the problem, finding the root cause, and applying diverse possible solutions.
Highly detailed resources on JavaScript modules and MIME types are available on Mozilla MDN Web Docs and the respective documentation pages for popular web servers such as NGINX or Apache httpd. Remember, it’s crucial to apply an approach that suits the specific configuration and constraints of your project.
Addressing Server-Side Issues
If you find that the server settings are the cause of the error, you’ll need access to its configuration to resolve the issue. Incorporating the necessary changes will involve a deep dive into your server’s documentation or reaching out to your hosting provider for guidance.
Each web server has its own way of recognizing and handling MIME types. For instance, Apache uses the `.htaccess` file, while NGINIX requires updating its configuration file. In both cases, you should meet the same goal: updating or adding to the file-type-to-MIME mappings to include `application/javascript` for .js files.
Thereby, by gaining insight into the problem domain and practical potential solutions can help rectify the ‘Failed To Load Module Script: Expected A Javascript Module Script But The Server Responded With A Mime Type Of Text/Html’ error, creating a gratifying user-experience through correct rendering of JavaScript elements on the targeted webpage.
Implications of Incorrect Server Response Types for JavaScript Modules
The implications of incorrect server response types for JavaScript Modules can be quite serious, particularly when the server responds with a MIME type of ‘text/html’ when a module script was expected.
Let’s first understand the context of this error; “Failed to load module script: Expected a JavaScript module script but the server responded with a MIME type of ‘text/html'”. Essentially, it means that the web server hosting the JavaScript module is misconfigured and not serving the files under the correct Media Type (also known as MIME type).
JavaScript modules should generally be served with the MIME type ‘application/javascript’, or more specifically ‘text/javascript’. However, if the server incorrectly serves the script using the ‘text/html’ MIME type – which typically happens when you request an HTML page that doesn’t exist, leading to a 404 error page being sent with ‘text/html’ MIME type instead – the browser will refuse to execute it for security reasons.
Here are a few critical implications of incorrect server response types for JavaScript Modules:
Error Messages: This could result in clients seeing errors such as ‘Failed to load module script’, potentially impacting their experience and confidence in your site.
Weakened Security: MIME type mismatches can cause security concerns. When an HTML document is loaded into a script context unaware, it opens risks to cross-site scripting attacks.
Broken Functionality: If the JavaScript module isn’t running correctly due to the mismatched MIME type, this could break functionality on your website, possibly leading to loss of business.
To avoid these issues, make sure to set up your web server correctly. Check the configuration files and make sure that JavaScript files are being served with the ‘application/javascript’ or ‘text/javascript’ MIME type. Once done, your JavaScript modules should correctly load without any errors regarding MIME type mismatches.
As Douglas Crockford, the creator of JSON said
"Programmers are dramatically more productive when they avoid writing code."
. Incorrect MIME type can complicate matters hence, the simplicity of serving files with correct Media Types should never be undervalued.
Here’s a table illustrating the difference between incorrect and correct server set up:
Incorrect Server Configuration | Correct Server Configuration | |
---|---|---|
MIME Type for Javascript Module | text/html | application/javascript or text/javascript |
User Experience | Potential error messages, broken functionality | Seamless operation, no errors |
Security Stance | Increased risk of scripting attacks | No unnecessary security risk |
NOTE: The HTTP specification mandates that every server response includes the ‘content-type’ HTTP header which tells the client about the MIME type of the returned content. For additional information on setting up your web server to serve the JavaScript files with the correct MIME type, you might find useful information in the Mozilla Developer Network documentation on MIME types.
For further reading on the specifics of implementing JavaScript modules, the article “ES modules in browsers” by Jake Archibald provides an in-depth look at the intricacies involved. It is highly recommended for understanding how JavaScript modules work and how they should be served.
Best Practices to Prevent MIME Type Errors in HTML/JavaScript
MIME (Multipurpose Internet Mail Extensions) type errors in HTML and JavaScript are common, especially when loading modules. This issue often arises due to improper configuration or misinterpretation of content types by different servers. When you encounter a ‘Failed To Load Module Script: The server responded with a non-JavaScript MIME type of “text/html”‘ error, it’s a clear sign that the MIME type expected by the JavaScript module does not match the one received from the server.
Here are several effective practices that can be utilized to prevent such errors:
1. Correct Server Configuration
The root cause of this error is often due to incorrect server configuration. It’s crucial that your server is set up to dispatch JavaScript files with the correct MIME types. Many popular servers such as Apache or Nginx require additional setting adjustments to serve the appropriate MIME types for .js files specifically. Check your specific server documentation for instructions on how to set MIME types.
2. File Extension Verification
Ensure your JavaScript files have the proper ‘.js’ extension. Sometimes, a simple file renaming to include the right extension can rectify the MIME type error.
3. Avoiding Inline JavaScript
Inline JavaScript can trigger MIME type errors when the browser cannot effectively distinguish between the HTML and JavaScript parts of a document. Best practice would be to store JavaScript in external
.js
files and link to them in the HTML using a script tag.
Incorrect: |
<script> |
Correct: |
<script src="example.js"></script> |
4. Avoiding HTML in JavaScript Files
Having HTML content present in a JavaScript file can provoke MIME type errors as the server may interpret it as HTML instead of JavaScript, and thus respond with a ‘text/html’ MIME type. By keeping JavaScript files purely JavaScript, you ensure the browser interprets the received content correctly.
As an eloquent reminder, Tim Berners-Lee, creator of the World Wide Web, once said, “Anyone who slaps a ‘this page is best viewed with Browser X’ label on a Web page appears to be yearning for the bad old days, before the Web when you had very little chance of reading a document written on another computer, another word processor, or another network.”
Applying this wisdom, ensuring proper communication between servers and browsers through accurate use of MIME types enables a positive and smooth web experience, showing professional prowess in your web application development.
References
- W3C – Setting charset information correctly.
- MDN Web Docs – Basics of HTTP / MIME types.
- Chrome Developers – Failed to load module script: The server responded with a non-JavaScript MIME type of “”text/html”.
The “Failed to Load Module Script: Expected a JavaScript Module script but the server responded with a MIME type of text/html” issue is typically encountered when your web application tries to import a JavaScript module but the server doesn’t produce the correct Content-Type header in its response. This leads to the browser rejecting the script, which eventually throws an error.
<script type="module">import {foo} from './bar.js';</script>
In the provided example, if our server delivers `bar.js` with a Content-Type of text/html instead of application/javascript or text/javascript, it could result in such an error.
Identifying and addressing this error involves maintaining a keen focus on some critical factors:
Validate MIME Types: Checking the MIME types for accuracy is essential, as the server must return scripts with the right MIME type (`application/javascript` or `text/javascript`). Applications comprise various modules, so it’s crucial each one corresponds to the correct type.
Server Configuration Changes: Adjustments may be required in server configuration settings to ensure that .js files (or the particular JavaScript module files) are served with the appropriate MIME type.
Cache Policies: In some instances, cache policies might affect the Fetch API, subsequently leading to this error. So, adjusting or disabling the service worker cache during development might rectify the problem.
If you’re curious about how MIME types work and their role in such errors, consult the Mozilla Developer Network guide.
As per Paul Irish, a developer advocate at Google, “Good habits formed at youth make all the difference.” And that’s very much applicable here in coding. An understanding of these foundational elements – including correctly specifying MIME types and other HTTP headers – is essential to create robust, maintainable, and future-proof web applications.