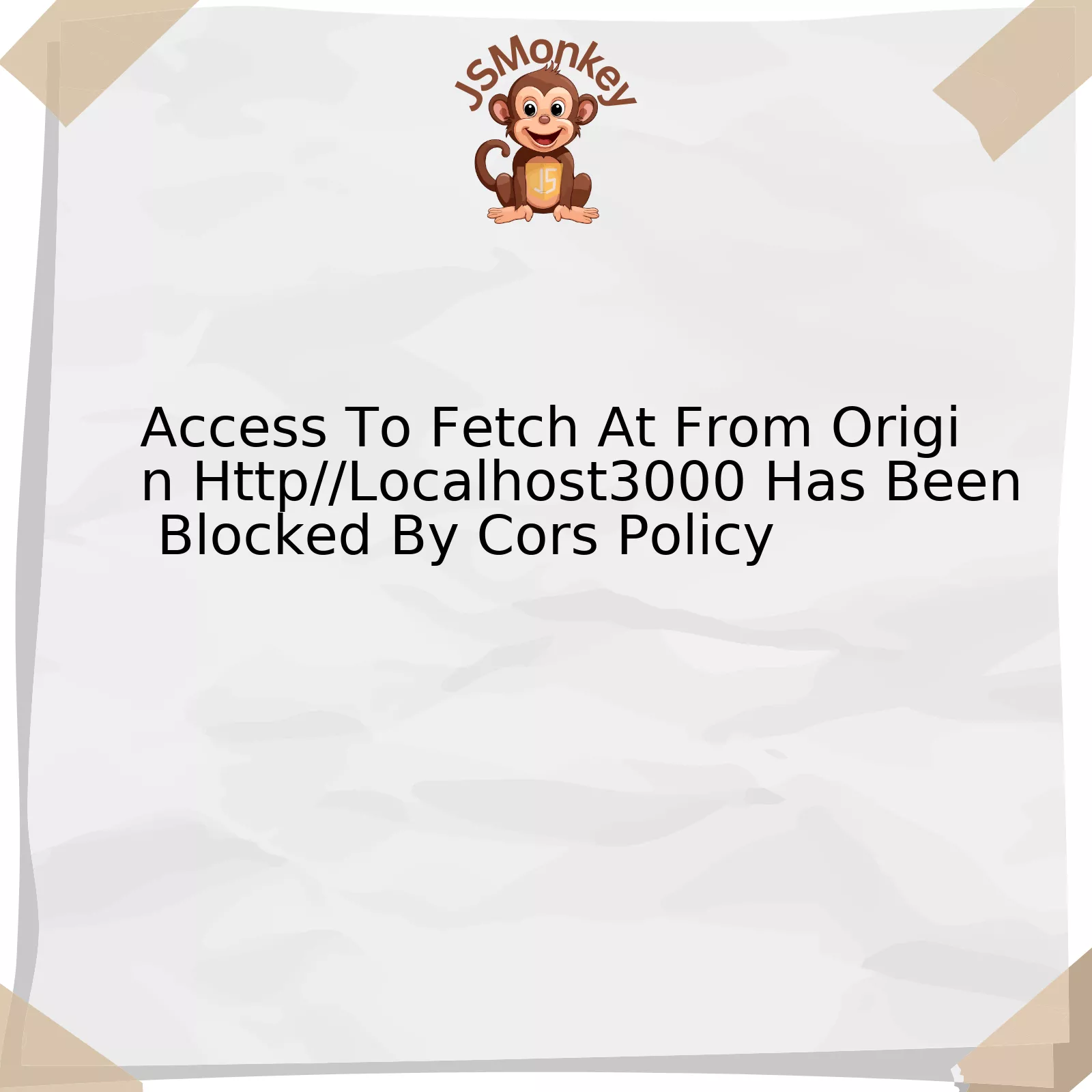
The phenomenon you’re encountering, “Access to fetch at from origin ‘http://localhost:3000’ has been blocked by CORS policy,” is a common issue developers face when working with fetching data and APIs while performing cross-origin requests. Let’s break this down in the following matrix:
Term | Explanation |
---|---|
Fetch | A JavaScript function used for accessing and manipulating HTTP(S) requests and responses. It’s used primarily to get or send data from/to a web server. Fetch requests are defined by the request method; GET, POST, DELETE etc. |
Origin http://localhost:3000 | Your local development environment where your current application resides. |
CORS Policy | Stands for Cross-Origin Resource Sharing. A mechanism that allows you to make a request from one website (origin) to another website (origin), provided certain headers are sent back within the response to the original requesting server. |
Cross-origin restrictions are security mechanisms implemented by browsers to restrict how resources on a page can be requested from different origins, to protect user’s data from malicious websites. This is why you see a `CORS` error when you attempt fetching data from different origins without appropriate allowance setup on the server side.
Typically, when encountering such barriers during development, it’s due to the local development server (in this case, http://localhost:3000) not being given explicit permission by the server holding the data to complete the fetch. The way to bypass this problem is to configure the server to send the correct headers allowing access.
Such headers instruct the browser that it’s safe to allow a web application running at one origin to access selected resources from a server at a different origin. A typical CORS header might look like this:
Access-Control-Allow-Origin: http://localhost:3000
When this is set, your application being served on ‘http://localhost:3000’ has permission to access this server.
“Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” – Martin Fowler. Therefore, it’s essential to fully understand both sides of a fetch request—the requesting client (browser) and responding server—to effectively resolve CORS issues. In your case, the server side would need the correct `CORS` policy configuration allowing access from `’http://localhost:3000’`.
Understanding CORS Policy: What, Why and How
Access to fetch at from origin http://localhost:3000 has been blocked by CORS policy is a common error message that developers encounter when working on web applications. This is because of a system implemented in web browsers for security purposes referred to as the CORS (Cross-Origin Resource Sharing) policy.
CORS Policy Explained
CORS is a mechanism that uses additional HTTP headers to tell browsers to give a web application running at one origin, access to selected resources from a different origin. For security reasons, web browsers prohibit web pages from making requests to a different domain than the one the website came from.
The Significance of CORS
The importance of having such policy can not be overemphasized considering the following points:
- It protects user data from being accessed by malicious scripts from unknown origins.
- Prevents inappropriate API use that could lead to unwanted side effects.
- API servers can control which origins are allowed, so they’re only used in the way they were intended.
Tackling Access Issues Caused By CORS
Dealing with the “Access to fetch at from origin http://localhost:3000 has been blocked by CORS policy” error comes down to two aspects i.e server-side or client-side adjustments.
Server Side modifications:
The error signifies that your server needs to include the appropriate CORS headers (Access-Control-Allow-Origin) in its response. Essentially, when a server receives a request from a different origin it must respond with this header specifying the requesting origin or ‘*’ to indicate all origins are acceptable.
Access-Control-Allow-Origin: * or Access-Control-Allow-Origin: http://localhost:3000
Client Side Modifications:
If you can’t modify your server-side, for instance when you’re working with third-party APIs, you’ll need to make use of a CORS proxy which adds the necessary headers to the response. Keep in mind though, that this isn’t recommended for production due to potential security risks.
As renowned computer scientist, Jack Ma, once said, “You should learn from your competitor but never copy. Copy and you die.” While working with technology, we must take proactive steps to understand its intricate details rather than resorting to quick fixes. If we simply try to circumvent policies such as CORS, we may open ourselves up to potential security vulnerabilities.
Approach | Method |
---|---|
Server Side | Add ‘Access-Control-Allow-Origin’ header in the server response |
Client Side | Use a CORS proxy to add required headers |
Indubitably, interpreting CORS is a fundamental part of understanding web development securities and rectifying related problems. Being oblivious of it drains ample time and effort during debugging. The more closely developers replicate the production, the sooner they catch issues like CORS, saving substantial efforts.
For further investigation on CORS policy, MDN Web Docs here, offers comprehensive resources.
Resolving Access Issues in CORS policy at http://localhost3000
Cross-Origin Resource Sharing(CORS) is a mechanism enacted by many web browsers to enhance the security of web applications. In connection with this context, HTTP requests are only permitted from the same domain due to a security concept known as the “same-origin policy”. The ‘Access to Fetch at from Origin http://localhost:3000 has been blocked by CORS policy’ error usually arises when you attempt to make a request from a different domain, port, or protocol. Fortunately, there are measures we can take to resolve this and still maintain the strength of your application’s security.
When addressing CORS issue, express.js on Node.js can be easily configured to allow specific or all domains for cross-origin access. Using the middleware function in Express.js, we can set certain headers to allow these requests:
const express = require('express'); const cors = require('cors'); const app = express(); app.use(cors()); app.get('/api', (req, res) => { res.json({ message: 'Hello from server!' }); }); app.listen(3000, () => console.log('Server started'));
Here, the CORS node package is leveraged to allow all origins by default. This is an easier approach than manually setting headers for allowing CORS.
Beyond allowing all domains, it’s possible to specify which domains should have access by configuring the CORS middleware:
app.use(cors({ origin: ['http://example1.com', 'http://example2.com'], methods: ['GET', 'POST'], credentials: true }));
Many developers opt for setting up a proxy to navigate around the issue. When creating React applications with create-react-app, a proxy can be set up inside the package.json file to redirect requests to the server:
{ "name": "client", "version": "0.1.0", “proxy”: “http://localhost:3000”, ... }
In this way, the server treats requests as coming from the same origin avoiding the CORS issue.
To reiterate, CORS is fundamentally a security measure and not an error. It prevents sensitive information from being exposed to malicious entities. The above solutions offer flexibility in configuring which domains can access your server’s resources while maintaining web application security. As telecommunication guru Tom Farley once said, “Like locks, websites should be secure enough to keep honest people honest.”
Remember, understanding how CORS works can help you troubleshoot access issues and will allow you to ensure the integrity of data interactions with your web applications.
Practical Tips for Troubleshooting ‘Blocked By Cors Policy’ Errors
The ‘blocked by CORS Policy’ error is a common issue faced by Web developers. This error occurs when a Web application tries to access resources from another domain without explicit permission. The problem in question is related to Access To Fetch At From Origin Http//Localhost3000 Has Been Blocked By CORS Policy.
CORS, or Cross-Origin Resource Sharing, is a security feature implemented by browsers to restrict Web applications from fetching resources from different origins, that is, different domain names, protocols, or ports. Mozilla Developer Network provides a comprehensive guide on how browsers use access control headers to ensure safe browsing experience for users.
When you see ‘Blocked By CORS Policy’ error for your localhost:3000 server, it signifies that the server is not configured properly to handle cross-origin requests from your Web application. Therefore, you may need to debug and fix issues in your server configuration. Here are some practical tips to troubleshoot this problem:
1. Enable CORS on Your Server:
Make sure that you have enabled CORS on your server. Check the server’s response headers to find out if ‘Access-Control-Allow-Origin’ is present. For Express.js servers in Node.js, you can enable CORS with the help of middleware like `cors`.
app.use(cors());
2. Specify the Correct Origin in Access Control Headers:
If you’ve enabled CORS but still encounter the error, check the value of ‘Access-Control-Allow-Origin’. If it’s set to a specific origin (say, http://example.com), make sure your web application’s actual origin matches this.
3. Check Access-Control-Allow-Headers and Access-Control-Allow-Methods:
Some cross-origin requests pass additional headers or use methods other than GET. In such cases, your server must specify these headers and methods in ‘Access-Control-Allow-Headers’ and ‘Access-Control-Allow-Methods’ respectively.
4. Investigate Preflight Options Requests:
Some requests trigger a “preflight” options request from the browser, including non-GET requests and ones with certain types of headers. Your server must respond correctly to this preliminary request in order for the browser to send the actual request.
While working on fixing the CORS issue, remember that “Debugging is like being the detective in a crime movie where you are also the murderer.” (Filipe Fortes, CTO at Clearbit). Just as in detective work, knowing what to look for and which tools to use can speed up your software diagnostics significantly.
Implementing Successful Solutions to Overcome Access Blocking by CORS
With regards to overcoming access blocking by Cross-Origin Resource Sharing (CORS), which is particularly relevant to the issue of Access to Fetch at from origin http://localhost:3000 has been blocked by CORS policy, there are several successful solutions to consider. These CORS principles largely focus on web security, based on the prevention of requests that might compromise user data.
Before we delve into solutions, understanding what triggers this CORS problem aids in effectively troubleshooting it. This particular error message occurs when an application residing on one website (http://localhost:3000, for instance) attempts to gain access or “fetch” resources from a different domain. A typical scenario may involve an API call where the server identifies cross-origin requests and restricts them due to potential security risks.
To mitigate such access barriers and enhance smooth cross-origin communication while ensuring optimal security, you might opt for strategies like:
– Modifying Server Configuration
– Utilizing Proxy Servers
– Implementing JSONP
– Leveraging CORS Browser Extensions
Modifying the server configuration allows us to endow browser-based applications with explicit permission for access. One typically does this by setting `Access-Control-Allow-Origin` headers:
app.use(cors({ origin: 'http://localhost:3000', optionsSuccessStatus: 200, }));
By specifying the origin value, the server permits incoming requests from http://localhost:3000.
Another tactical solution would be employing proxy servers, which essentially mimic a middleman – taking your request, sending it to the destination site, and returning the response back. Here’s an example using Express.js middleware:
const express = require('express'); const proxy = require('http-proxy-middleware'); const app = express(); app.use('/api', proxy({target: 'http://localhost:3000', changeOrigin: true})); app.listen(8000);
JSONP works around the CORS issue by using a method only applied to `GET` requests. It functions by adding a script tag to HTML, which fetches the JavaScript with JSON data embedded from the server.
Using browser extensions dedicated to handling CORS issues can also help bypass such problems. CORS Everywhere (for Firefox) and Allow-Control-Allow-Origin: * (for Chrome) are two popular options.
These solutions underscore the importance of refining CORS strategies—keeping in mind that security, transparency, and accessibility are essential to improve application performances and user experiences. As British computer scientist Sir Tim Berners-Lee once said, “The Web as I envisaged it, we have not seen it yet.” Inherent in this vision is a continuous call to programmers to seek improved and inclusive methods for accessing online resources while maintaining superior security norms.
Do note that while these solutions could mitigate CORS issues during development, they might not be ideal or secure for a live, production environment. It is advisable to develop comprehensive security policies outlining appropriate CORS practices for production environments along with diligent adherence to them.
Cross-origin resource sharing, commonly referred to as CORS, is a significant aspect of Javascript development, and it revolves around the security protocols that restrict which websites can access a server’s resources. Occasionally, developers may encounter a challenge known as ‘Access to Fetch at from Origin http//localhost:3000 has been blocked by CORS policy’. This issue typically occurs when a resource hosted on the localhost server attempts to fetch data from a different origin without permission from the host server.
Understanding CORS policy becomes crucial here. It is a mechanism that employs additional HTTP headers to inform browsers about which web applications running on other origins have permissions to access specific resources. In case of an error like ‘Access to Fetch at from Origin http//localhost:3000 has been blocked by CORS policy’, it means that the CORS policy of the requesting site hasn’t been set up to allow its requests.
To resolve this, modify your server configuration to return appropriate CORS headers. A simple solution would be returning a response header with
Access-Control-Allow-Origin: *
, which allows all domains to access the server’s resources. But it’s important to remember that setting an asterisk (`*`) can pose a potential security risk by granting access to potentially malicious actors. Hence, in any production environment, it’s better to specify individual domains.
While the above method solves the issue at the server level, it’s possible to resolve it on a developer level for testing purposes. Libraries such as ‘cors-anywhere’ can be used as a proxy server to add CORS headers to the proxied request.
As we continuously navigate our way through the evolving world of JavaScript Web Development, understanding and effectively implementing such measures can go a long way in ensuring safe and successful cross-origin communications. As renowned technologist Tim Berners-Lee once quoted, “The Web as I envisaged it, we have not seen it yet. The future is still so much bigger than the past.”
Beyond the complexities that CORS might present, lies the promise of a more secure and interconnected web environment. The capabilities of HTTP requests stretch far beyond fetching resources from a server – they encompass our ambition for an open yet secure web, paving the path for further innovations.