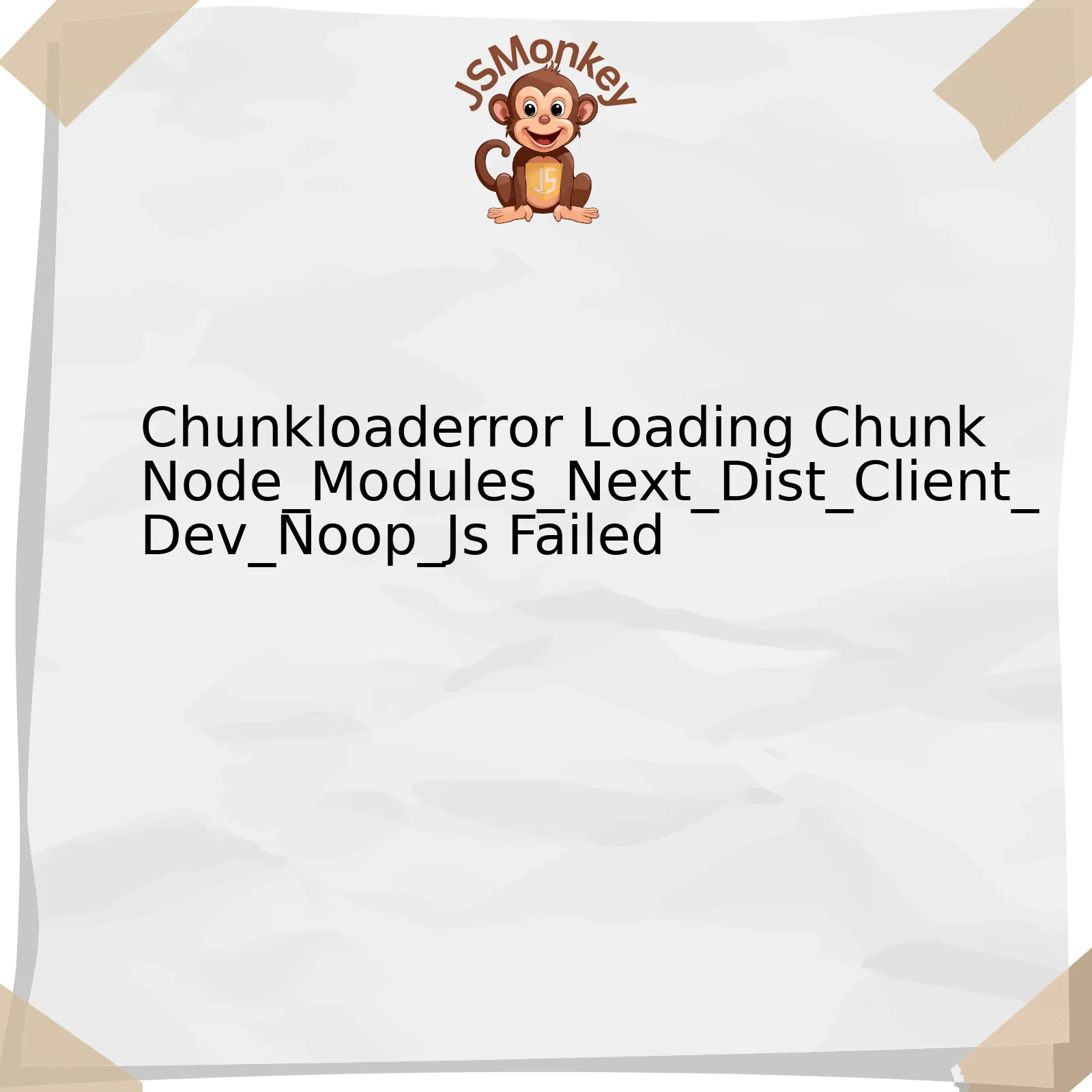
The
ChunkLoadError
is often encountered when operating with JavaScript modules, specifically while dealing with Next.js – a popular open-source development framework. It signifies difficulties in loading JavaScript chunks or parts, particularly the
node_modules/next/dist/client/dev/noop.js
module.
Let’s structure the issue in an easily understandable format:
html
Error Type | Affected Module | Possible Causes | Solutions |
---|---|---|---|
ChunkLoadError | node_modules/next/dist/client/dev/noop.js | Incomplete Dependency Installations, Network Errors | Reinstall Dependencies, Check Your Network |
A chunk load error can be attributed to several causes primarily ranging from issues surrounding incomplete dependency installations to errors happening on the network level.
As the table indicates, accidentally skipping certain dependency installations or experiencing disruptions during installation can trigger the `ChunkLoadError`. The affected module in question, the `node_modules/next/dist/client/dev/noop.js`, might not find the necessary dependencies to run successfully due to the incomplete installation process.
Possible resolutions include:
– **Checking your dependencies**: Sometimes, a corrupted or incomplete installation of dependencies leads to chunkload errors. In such a case, the course of action is reinstallation; make sure you have correctly installed all necessary dependencies.
– **Making Corrections in the Network**: Network errors sometimes hinder the proper loading of JavaScript chunks. Double-checking your network configuration can help rectify the problem. If the issue persists even on a stable network, it might indicate a deeper-rooted problem that needs professional attention.
As Bill Gates aptly put it, “Software innovation, like almost every other kind of innovation, requires the ability to collaborate and share ideas with other people, and to sit down and talk with customers and get their feedback and understand their needs.”
Hence, let’s remember that this error is not catastrophic; it’s an opportunity for learning and collaboration. We aim to embrace the challenge, tackle it strategically, and continue refining our approaches in robust software development.
ChunkLoadError
effectively serves as a diagnostic tool pointing us towards potential problems – we just need to comprehend its message and respond accordingly.
Understanding the Cause of ChunkLoadError in Node_Modules_Next_Dist_Client_Dev_Noop_Js
The `ChunkLoadError` in the context of `Node_Modules_Next_Dist_Client_Dev_Noop_Js`, inevitably, relates to JavaScript’s dynamic imports. The concept of chunk (splitting code for load optimizing) is an integral part of modern web development practices.
When you’re dealing with a large application, breaking down your code into manageable “chunks” leads to an improved application performance and user experience. However, as much as this technique is beneficial, it might cause some issues, especially in situations where chunks fail to load – which results in what we call the `ChunkLoadError`.
Error: Loading chunk {number} failed.
Here, `{number}` will vary based on the sequence number of the chunk experiencing the issue.
This error generally occurs due to:
- Network Connections: Unstable network conditions can hinder chunk loading. This could be because of poor internet quality or firewalls.
- Caching Issues: If there are changes made to your Next.js build, while a user still retains an old version of the site cached in their browser, there might be discrepancies trying to load the current chunks.
- Filename Changes: Any changes in filenames during runtime can lead to `ChunkLoadError` as it breaks the path required to load the specific file or module.
Having understood the common reasons behind `ChunkLoadError`, here are some tactics you could implement to prevent or solve these errors:
- Ensure Stable Network Connection: Run tests to ensure that the system has a stable internet connection before loading chunks.
- Invalidate Old Service Workers: To avoid conflicts with older caches, ensure to unregister any existing service workers and clear out all the caches with each update.
- Verify Filenames: Keep filenames consistent, especially when dealing with chunks. Avoid runtime name changes or alterations that could potentially result in chunk load failures.
“Debugging is twice as hard as writing the code in the first place. Therefore, if you write the code as cleverly as possible, you are, by definition, not smart enough to debug it.” – Brian Kernighan on tackling coding errors such as these.
Remember, debugging `ChunkLoadError` requires patience and thorough understanding of your application’s architecture and your user’s behavior. Persevere, learn from every error, and keep refining for better performance.
How to Troubleshoot ChunkLoadError in Next.js Applications
If you encounter a ChunkLoadError in Next.js applications, this usually means that some JavaScript chunks required for dynamic imports can’t be loaded. Notably, the reference to ‘chunk node_modules_next_dist_client_dev_noop_js’ indicates that this error comes from loading development-only code when not in development mode.
To troubleshoot such issues, consider these solutions:
1. Clean install dependencies:
Sometimes, deleted or moved files cause inconsistencies in the bundled JavaScript files and cache. To correct these inconsistencies:
- Remove your node_modules folder by running
rm -rf node_modules
- Delete package-lock.json or yarn.lock depending on what you use
- Reinstall dependencies using
yarn install
or
npm install
2. Update your Next.js version:
Along with other libraries like webpack, it’s possible that outdated versions could have bugs leading to ChunkLoadErrors. In many cases, updating to the latest version of Next.js should fix the issue. Run
npm update next
or
yarn upgrade next@latest
3. Check for correct server configuration:
Some server configurations may block loading of certain JavaScript files, causing a ChunkLoadError. You’ll need to ensure that your server allows all JavaScript chunk files to be accessed and loaded. This includes verifying that there are no conflicts in your .htaccess file or other firewalls that would prevent these files from being served correctly.
4. Review the build process:
Deeper issues may affect the bundling process, and in turn, impede chunks from loading. Perhaps you’re not properly defining some side-effect imports, or maybe the import() syntax is used incorrectly. Refine the build process, scrutinize the webpack output during the build, correct any errors or warnings seen here.
5. Inspect Network Tab in Developer Tools:
Examine your browser’s network tab to inspect failed network requests. This may reveal useful details about why a particular file failed to load, providing hints for solving the problem.
As Github co-founder Tom Preston-Werner once put, “Often, it’s not about making the right decision, it’s more about making some decision.”. When troubleshooting, isolating the problem is half the solution. With careful attention to error messages, strategic debugging methods and thorough examination of the conditions causing the issue, you can overcome this Challenge.
Sources:
Dynamic Import,
Code Splitting with Webpack,
Console.error()
Preventive Measures Against Node_Modules_Chunk Load Error Logging
One of the common problems you may encounter while working with Next.js or any other Node.js based frameworks is a load error related to certain chunks, such as
chunkloaderror loading chunk node_modules_next_dist_client_dev_noop_js failed
. Concerning this issue, primary cause often points to incorrect bundling or file paths.
Before proceeding to take preventive measures, it’s instrumental to understand that “chunks” in JavaScript techniques correspond to code splitting – an optimized packaging approach for JS modules, and Node Modules. Here, errors may emerge due to failure in loading these split parts of your application.
Let’s delve into the following approaches to circumvent this pitfall:
Ongoing Debugging
Utilize advanced debugging tools like Chrome DevTools in identifying sources of errors. Check whether the corresponding file is present and confirm the path being correct.
Optimal Bundle Configuration
Fine-tune your webpack configurations for precise and accurate chunk splitting. Ensure that all relevant node_modules are included appropriately in your build.
Use of Latest Packages
Employ the latest versions of key libraries/frameworks (like Next.js) which ensure substantial bug fixes, performance improvements, and enhanced features, thus reducing probability of encountering chunk load failures.
Adequate Tests
Craft critical end-to-end tests examining your apps behavior. Detect and mitigate risks associated with third-party packages or libraries including but not limiting to load errors.
Erroneous Service Workers
A service worker misconfiguration might be causing unnecessary issues. It would be worth checking if there are conflicts between service workers and your web pack configuration that are causing these load errors.
In the wise words of Mark Zuckerberg – “In a world that’s changing really quickly, the only strategy that is guaranteed to fail is not taking risks.” Therefore, while dealing with Node.js or similar tools, embrace the complexities. Persistence and tenacity will allow you to navigate through various challenges en route, including errors related to chunk loading.
Stackoverflow: Next.js Chunk Load Error could be a practical resource to find more discussions and fixes regarding this issue.
Comprehensive Solutions for Resolving ChunkLoadErrors
ChunkLoadErrors in JavaScript occur when a portion of your code, or a ‘chunk’, fails to load. In the context of “Chunkloaderror loading chunk node_modules_next_dist_client_dev_noop_js failed”, this indicates that the Next.js module is having trouble fetching a particular JS file from its server.
The error can arise due to a variety of factors such as:
– Network interruptions during the code download phase,
– Ad-blockers incorrectly blocking code files,
– Cache issues related to browser or service worker caches,
– Configuration errors during build time.
A comprehensive solution to these problems involves a multi-pronged approach:
1. Handle Network Interruptions
To counter network interruptions causing ChunkLoadErrors, script tags can be configured with additional attributes:
rel="preload"
and
as="script"
. These help preemptively start downloading scripts while concurrently fetching other resources, improving the chances of complete downloads:
<link rel="preload" href="/path/to/chunk.js" as="script">
2. Bypass Ad-blockers
Some Ad-blockers may falsely identify your chunks as adverts and block them. To prevent this, avoid naming conventions similar to those used by advertisements (like ‘ad.js’) for chunk files’ names. You also need to ensure your chunk isn’t hosted from URLs known to serve ads.
3. Managing Caching Issues
The strategy for resolving caching issues depends on the type of cache causing it – the browser or service worker cache.
Browser Cache:
For browser cache issues, set the HTTP Header ‘cache-control’ to ‘no-cache’ or use meta tags just after the head tag like shown below:
<meta http-equiv="Cache-Control" content="no-cache, no-store, must-revalidate">
Service Worker Cache:
For the service worker cache, try unregistering the service worker within your code like so:
navigator.serviceWorker.getRegistrations().then(function(registrations) { for(let registration of registrations) { registration.unregister() } })
Note that, although this resolves the ChunkLoadError, it requires a refresh to take effect and may disrupt caching strategies of PWAs (Progressive Web Apps).
4. Lastly, Correct Building Configurations
Appropriate build and deployment configuration is crucial in preventing ChunkLoadErrors. Most notably in applications using tools like Webpack or Next.js, meticulously ensure that the ‘publicPath’ field is correctly set.
Steve Jobs once said, “Technology is nothing. What’s important is that you have faith in people”. Similarly, while these technical solutions are valuable, do not be discouraged from investigating other potential issues or testing your solution across different environments.
Keep in mind, as technologies improve, there may be more precise ways of diagnosing and resolving issues like ChunkLoadErrors. Hence, keep updating your knowledge base and stay on top of new JavaScript developments.
Refer to the following links to further delve into this topic:
[Handling Network Interruptions](https://developer.mozilla.org/en-US/docs/Web/HTML/Preloading_content)
[Managing Browser Caching](https://developer.mozilla.org/en-US/docs/Web/HTTP/Headers/Cache-Control)
[Handling Ad-blockers](https://adblockplus.org/blog/how-we-decide-what-gets-added-to-the-defaultallowlist)
[Fixing Building Configuration Issues (Webpack)](https://webpack.js.org/guides/public-path/)
[Resolving Service Worker Issues](https://love2dev.com/pwa/service-workers/)
Addressing the issue of “ChunkLoadError loading chunk node_modules_next_dist_client_dev_noop_js failed”, it’s crucial to understand that this is a fairly common problem faced in JavaScript development, more specifically when using React and Next.js packages. Rather than a direct problem with the package `node_modules/next/dist/client/dev/noop.js`, such an error often arises from conflicts in different versions of Node.Js or corruption in installed dependencies.
It’s important to keep in mind that:
• This type of error originates because Next.js/Webpack is unable to load a specific module during runtime. This could be due to naming conflicts, broken links, or missing bundles.
• It may also result from incorrect caching. When the browser has cached older versions of the Javascript chunks while you’ve made changes to these chunks, it may lead to a ‘chunk loading error’.
To address this issue, several troubleshooting avenues can be explored for effective resolution:
•
npm cache clean --force
: This command forces Node Package Manager (NPM) to clear the cache.
•
delete node_modules
and
npm install
: Deleting the previous corrupted ‘node_modules’ directory and doing a fresh installation should rectify any internal mismanagement or corruption.
• Update Node.js to the current stable version: Using outdated versions of Node.js increases the likelihood of encountering such errors.
• Switch off “HardSourceWebpackPlugin”: If your Webpack has been configured with “HardSourceWebpackPlugin”, it causes trouble with hot reloading and leads to chunk loading issues.
To wrap up, fixing this error might involve examining your application’s setup closely, clearing Node.js or NPM caches, or even making alterations to your Webpack configuration. While it can be frustrating, understanding the root of cause allows you to gauge better measures towards resolution.
As Donald Knuth, a renowned computer scientist beautifully said: “Debugging is twice as hard as writing the code in the first place. Therefore, if you write the code as cleverly as possible, you are, by definition, not smart enough to debug it.” This situation serves as a perfect reminder of the importance of understanding and knowing how to fix bugs when dealing with complex packages such as Next.js.
For further details about `Next.js`, you can visit their Official Documentation.