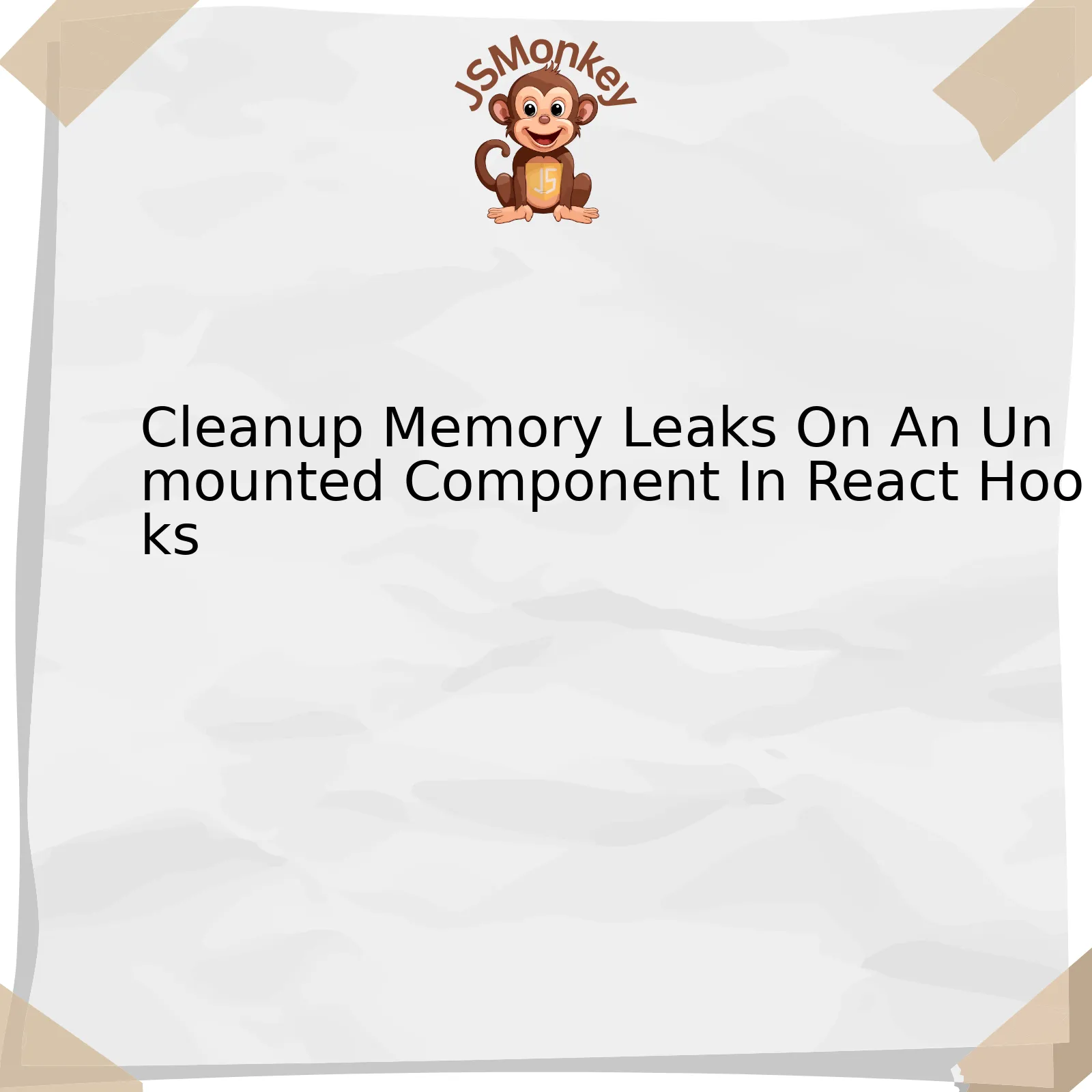
Component Lifecycle Stage | Action Taken | Possible Memory Leak | Cleanup Process |
---|---|---|---|
Mounting | Creation of component and initialization of React Hooks. | No memory leak occurs at this stage since the mounting is managed by React’s internal mechanisms. | No cleanup is needed in this phase. |
Updating | Component re-renders due to state or prop changes. Here, a new instance of function component or hook could be created. | Without proper cleanup, old instances might still hold references which can lead to memory leaks. | The cleanup function in the useEffect hook, returned by the effect itself, can be used for cleanup activities like timeout clearing or cancelling API calls etc. |
Unmounting | Destruction of component | If there are ongoing processes such as pending promises, subscriptions, or timers related to a component, their execution after unmounting could cause a memory leak. | In the body of the useEffect hook, return a cleanup function that cancels any ongoing async operations associated with the hook. This function runs when the component unmounts, preventing any potential memory leaks. |
When using Hooks in React, managing memory leaks becomes an essential aspect, particularly during the lifecycle of components: Mounting, Updating, and Unmounting. During the Mounting stage, there’s no risk of memory leaks as it’s managed by React’s frameworks.
However, the Updating- and Unmounting stages have potential risks. In the Updating stage, the component may re-render due to updated state or props. This rerendering generates a new instance of our Function Component or Hook. If we don’t manage this well, previous instances might maintain references leading to memory leaks.
To assist in managing this, React provides a cleanup function through the `useEffect()` hook. The returned function from useEffect hook becomes the cleanup function. We can use it to handle cleanup activities (like clearing timeouts or cancelling API calls).
javascript
useEffect(() => {
//component did mount logic here
return () => {
//cleanup logic goes here
}
}, [])
In the Unmounting stage, there are similar risks. Suppose a process such as a pending promise, subscription, or timer is associated with a component and continues execution after the component unmounts. This event leads to memory leaks. To remedy this, our useEffect hook’s body should return a cleanup function that cancels any ongoing async operations tied to itself. Executing this function before the component unmounts ensures the end of these processes, and therefore preventing memory leaks.
As Robert C. Martin famously stated: “The best way to make code robust is not by putting in lots of catch blocks; it’s by catching problems early” – a quote remarkably suitable for handling React memory leaks. By monitoring our component lifecycle stages appropriately and using the tools provided to us by the React hooks like `useEffect()`, we can avoid issues like memory leaks and develop more positive user experiences moving forward. Besides enhancing performance, this also adheres to the principles of clean and efficient coding.
Identifying Memory Leaks in Unmounted React Hooks Component
Identifying memory leaks in React Hooks components that are unmounted can be a task requiring keen observation and sound knowledge of how the flux of React components works.
However, before diving into the solution, it would be essential to understand the term ‘memory leak’ in the context of React Hooks. A memory leak is when out-of-scope references linger long after the component has been unmounted, leading to an unnecessary allocation of memory. These lingering references can cause devastating effects on the web application’s performance if not quickly identified and rectify.
When dealing with hooks, the function useEffect can help avoid memory leaks in your React app. The useEffect function provides a cleanup mechanism that runs when the component unmounts. This cleanup helps prevent side effects from running after the component has unmounted, essentially freeing up memory and therefore avoiding potential memory leaks. Below is an example:
useEffect(() => { //...Some Code... return () => { // clean up code here }; }, []);
An important thing to remember is that the array passed as the second argument to useEffect determines its behavior. An empty array ensures the useEffect hook only runs once (similar to componentDidMount), and cleanup is done when the component is unmounted.
Suppose you were, for instance, creating an event listener within the useEffect hook that needs to be cleaned up once the component is unmounted. In this case, the cleanup function becomes very vital, otherwise leading to a memory leak scenario. That might look something like below:
useEffect(() => { const handler = () => { /*Some event handling*/}; window.addEventListener('resize', handler); return () => { window.removeEventListener('resize', handler); }; }, []);
This way, the event listener gets removed when the component is unmounted, eliminating the possibility of a memory leak.
As Steve McConnell once said, “Good code is its own best documentation.” Therefore, comprehending and implementing proper code cleanup can help maintain high-performance applications by avoiding memory leaks.
Hence, React’s useEffect hook provides developers with an easy-to-utilize tool to track down and mitigate memory leak issues, which ultimately results in a more robust and efficient web application. (source). An essential factor with React Hooks and any JavaScript coding is to ensure that your setup and cleanup code are placed correctly to prevent memory leaks. The same principle applies whether you’re developing new software or maintaining existing systems.
Utilizing useEffect Hook for Error-free Unmounting
When dealing with asynchronous operations in React components such as timeouts, server requests, and subscriptions, it’s a common issue that those operations may complete after the component has already unmounted. If you try to update the state or access properties of an unmounted component, it leads to memory leaks and can cause crashes and undefined behavior in your application. To handle these situations effectively, we rely on the `useEffect` Hook which provides a built-in clean-up mechanism.
React `useEffect` Hook is a function that gets triggered at different stages of a component’s life cycle. It runs after every render including the first one and takes two parameters: A callback function where the side effect occurs, and a dependency array.
The cleanup process in `useEffect` is performed by returning a function from it. This returned function is executed when the component is going to unmount or before the next effect is run.
Here is an example of how `useEffect` can be used for error-free unmounting:
javascript
import React, { useState, useEffect } from ‘react’;
function ExampleComponent() {
const [data, setData] = useState(null);
useEffect(() => {
let isMounted = true;
fetchData().then(response => {
if (isMounted) {
setData(response.data);
}
});
return () => {
isMounted = false; // Component is no longer mounted. Stop updating state
};
}, []); // Empty array indicates this useEffect will run once when the component mounts.
return (
);
}
In the example above, we’re making use of a local variable (`isMounted`) within our `useEffect` hook to track whether the component is currently mounted. When the component unmounts, we change the value of `isMounted` to false via the cleanup function returned from `useEffect`.
This approach ensures that the state will only be updated if `isMounted` is true, preventing React from throwing an error about trying to update state on an unmounted component. Using this strategy helps clean up memory leaks effectively and ensure smoother application performance.
“To write readable and maintainable code, remember that functions should do one thing. They should do it well. They should do it only.”, Robert C. Martin articulates in his book Clean Code. The same principle can be applied when writing useEffect hooks. It’s important to keep a single concern in mind while writing these hooks for easier readability and debugging.
For further reading, you might want to consider referring to the official React Hooks documentation where useEffect hook and its cleanup mechanism are detailed thoroughly.
Applying Cleanup Functions to Manage Unmounted Components
React Hooks, introduced in v16.8, brought mature functional programming primitives to React. The adoption of hooks allows developers to express complex state manipulation logic in a lucid manner while preserving functional purity and avoiding bloated component trees.
A key aspect of maintaining clean, efficient applications is the proper use of cleanup functions, particularly in managing unmounted components within the purview of React Hooks. Managing these unmounted components improves app performance by preventing the support overload from “memory leaks”. These rogue procedures arise when a component is removed from the DOM before its associated processes are complete﹘whether because of data fetching or intensive computations. This results in dangling promises that point to nullified value, thereby causing memory leaks.
To illustrate cleanup function utilization for managing unmounted components via React Hooks, let’s look at this example:
JavaScript
import React, { useEffect, useState } from ‘react’;
function Example() {
const [data, setData] = useState(null);
useEffect(() => {
let mounted = true;
fetchData().then(response => {
if (mounted) {
setData(response);
}
});
return () => {
mounted = false;
};
}, []);
…
}
In this example code, we incorporated the `useEffect` hook which incorporates cleanup operations. In essence, everything returned by `useEffect()` simulates a deconstruction process that executes whenever the component dismounts﹘ what we typically recognize as `componentWillUnmount` in class components. By calling the cleanup function, we can ensure the `fetchData` process will no longer try setting state on an unmounted component—minimizing the chances of notorious memory leaks.
A similar tech perspective was also portrayed by Abby Carnelia, an experienced software engineer and ex-Stitchfix employee, who quoted, “By making memory leak prevention part of the developer’s toolkit, React Hooks improves upon the principles of both functional programming and good housekeeping within codebases.”
The key takeaway is this: proper use of cleanup functions in managing unmounted components within the context of React Hooks can effectively prevent memory leaks, leading to an overall preservation of performance. React as a framework encourages such practices by providing easy-to-understand life-cycle methods and safe data handling procedures.
Advanced Techniques for Solving Memory Leak Issues in React Hooks
Solving memory leak issues in React hooks, particularly when focusing on cleaning up memory leaks on unmounted components, often involves the utilization of advanced techniques tailored to ensure optimal code performance.
Memory leaks occur when no longer needed memory is not released back to the operating system.
In JavaScript
, this mainly occurs when the garbage collector can’t reach an object held in memory due to outdated references. This situation is more frequent in callback functions or event listeners that have been set but not cleared.
Component unmounting in React refers to the process of removing a component from the DOM, making it non-operational. Instances do occur where after setting up resources for a component — such as timers, network requests or subscription services — they are not properly cleaned up once the component is unmounted from the DOM.
Focusing on React Hooks, there are two prevalent methods utilized to combat memory leak issues associated with unmounted components:
1. Utilizing the
useEffect
Hook for Cleanup:
React’s
useEffect
provides a cleanup mechanism, which invokes a special function upon unmounting of the component. This handy feature enables developers to clear timers, cancel network requests or clean any subscriptions which were setup during the component life-cycle. A simplified example of this mechanism is shown below:
useEffect(() => { // Set up resources. return () => { // Clean up resources. }; });
This pattern ensures a lifecycle balance: every time a new effect is invoked (component updated), the previous effect will be cleaned up first.
2. Checking if a Component Is Mounted before State Update:
State variables defined using
React.useState
or
React.useReducer
should not be updated if the component has been unmounted as this commonly leads to memory leak issues. It’s beneficial to include a mechanism to check if the component is still mounted before proceeding with updates.
let isMounted = true; useEffect(() => { // Check if component is mounted and update state return () => isMounted = false; }, []);
In this scenario,
isMounted
is utilized to keep track of the component’s mount status and prevent state updates on unmounted components, with a boolean check built directly into the
useEffect
call.
The correct management of resources and effective cleanup plays an essential role in large-scale applications. It helps to reduce memory leaks that potentially degrade application performance over time. By leveraging React Hooks efficiently, developers can maintain better control over resource allocation and ensure smooth component lifecycle operations.
“Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” – Martin Fowler, renowned author, speaker and software development expert. While we may focus a lot on performance and efficiency, this quote underscores the importance of readability and clear logic when developing advanced solutions for complex issues such as memory leaks.
Additional information regarding memory leak prevention using React Hooks can be found in the official React documentationReact Docs-Hooks Effect.
Reflecting on the process of cleaning up memory leaks in unmounted components using React hooks, we delve into an essential feature of robust web application development. Memory leaks can drastically hamper an application’s performance and reliability. Employing React Hooks specifically has surfaced as a proficient method of mitigating these issues due to their lifecycle control offerings.
The utilization of
useEffect
hook, which triggers at different lifecycle stages of our component, is one such mechanism that proves advantageous.React’s official documentation provides a comprehensive understanding of this feature.
“Any application that can be written in JavaScript, will eventually be written in JavaScript.” – Jeff Atwood, Co-founder of StackOverflow.
It’s common practice, upon dispatching an asynchronous operation, to set the result state for the component. But anomalies arise if the component is unmounted before the operation completion, leading to an attempt of state update on an unmounted component — a quintessential genesis of memory leaks. We counter such a scenario by incorporating cleanup functions within our
useEffect
hook, which gets invoked automatically during the component’s unmount phase.
A classic example would be:
useEffect(() => { let isMounted = true; fetchStats().then(data => { if (isMounted) setState(data); }); return () => { isMounted = false }; }, []);
Here, the returned function acts as a cleanup mechanism setting the ‘isMounted’ flag to false when the component unmounts. This practice ensures thorough management of memory resources in your react application, and the prevention of undesirable memory leaks, leading to an enhanced user experience.
Enhancing memory management using React hooks underscores the effortless adaptability provided by modern JavaScript frameworks. These practices afford us the flexibility to build scalable, effective, and efficient applications that are both robust and provide optimal user experience.
Maintaining efficient memory use by cleaning up unmounted components should be fundamental in web application development, greatly impacting the app’s performance. By reducing memory leaks, these practices using React Hooks contribute to building a more seamless digital landscape, validating Jeff Atwood’s quote — a testament to JavaScript’s superiority and versatility in web application development.