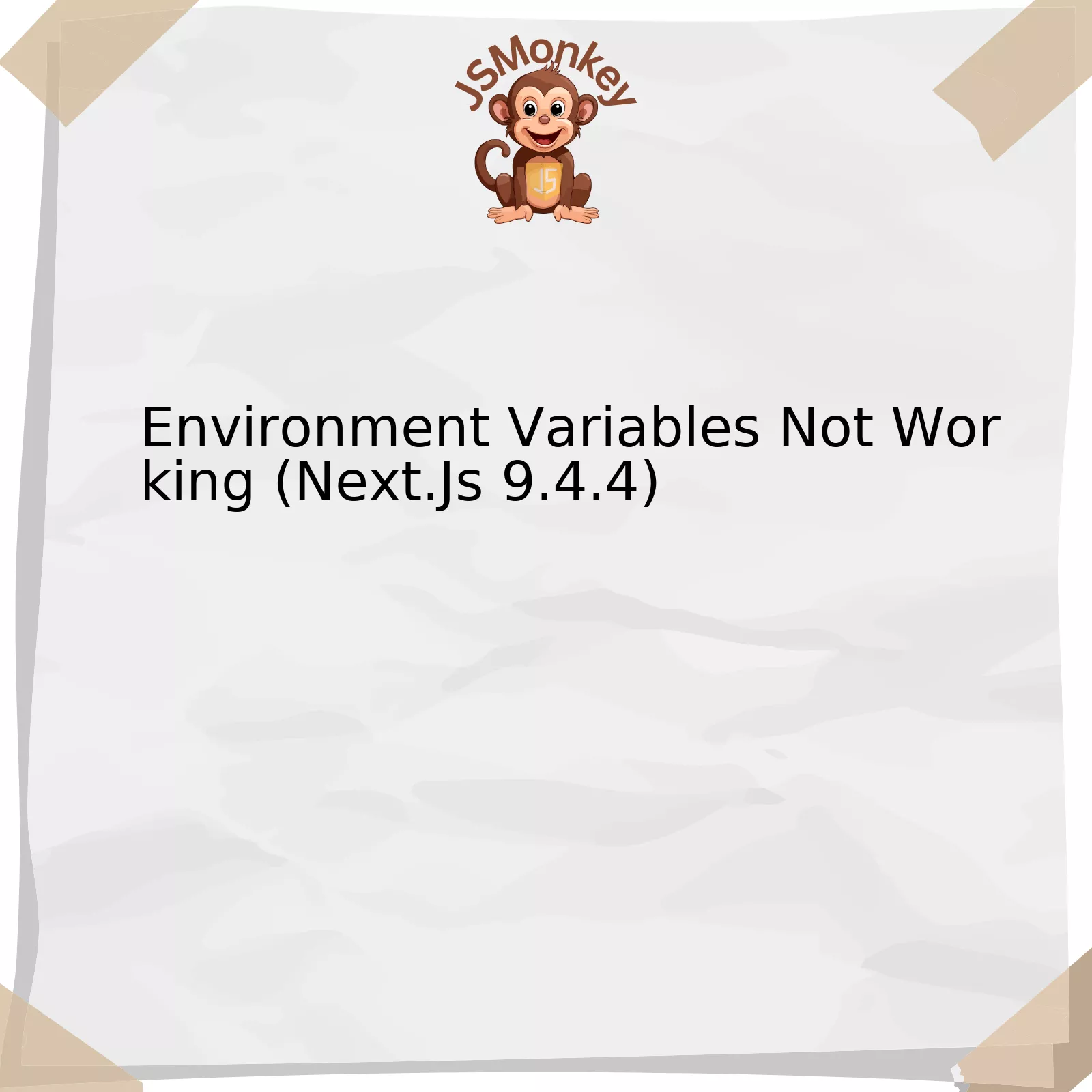
The following encapsulates the core precepts concerning Environment Variables not functioning in Next.Js 9.4.4:
Issue | Possible Cause | Proposed Solution |
---|---|---|
Environment variables not loading | Utilizing .env files not supported before Next.js version 9.4 | Upgrade to a later version that supports the .env methodology or use ‘next.config.js’ |
Environment variable values not reflecting on web application | Inefficient redeployment after altering environment variables | Make sure to redeploy the application after changing the values of environment variables |
Variables available at build time, but not in runtime | Prefixed variables without ‘NEXT_PUBLIC_’ term | Prefix all public runtime variables with ‘NEXT_PUBLIC_’ to make them available in browser code |
To delve into these issues more responsibly, the first potential problem could be arising from using an unsupporting Next.js version. Specifically, versions below 9.4 do not have support for using .env files to set up your environment variables. Therefore, if you’re working with version 9.4.4 exactly and experiencing this issue, upgrading to a later version is recommended.
Alternatively, you may consider setting up your environment variables using the ‘next.config.js’ file, instead. This configuration represents your solution if you intend to stick with version 9.4.4 or any earlier version that doesn’t support the .env file format.
Secondly, a probable cause for this dilemma may lie in redeployment. If you’re modifying environment variables and immediately checking changes in your web application without redeploying it, the problem persists. In Next.js, similar to various other modern JavaScript frameworks, changing environment variables calls for a fresh deployment of the application to observe the new variable values.
Lastly, the discrepancy in availability of environment variables between build time and runtime is explained as per the design ethos of Next.js. Since all environment variables are accessible during compile (build) time, public runtime variables need a prefix of ‘NEXT_PUBLIC_’ to become available in browser JavaScript code. Therefore, ensure adding this prefix to all runtime variable names.
As Sal Khan, the founder of Khan Academy, said: “With coding, you can create things that could be used by millions of other people.” Embarking on resolving your environmental variable issues will not only enhance your individual project but contribute towards a tool universally beneficial.
Determining Causes of Environment Variables Failure in Next.Js 9.4.4
Negating the appropriate functioning of environment variables in Next.js 9.4.4 could result from various determining factors. The typical developmental framework of Next.js is created to make use of environment variables relatively straightforward, rendering them an invaluable tool in the versatile JavaScript development cycle. Therefore, understanding the causes of their failure is a crucial aspect in troubleshooting and mitigating such issues.
Non-Compliance with Prefixed Variables
Firstly, one must ensure that the environment variables are prefixed with
NEXT_PUBLIC_
. This is a requirement necessitated by the way Next.js works. Anything not prefixed might cause the variables to stop functioning properly. For example:
// .env // Wrong approach DB_HOST=localhost DB_USER=root // Correct approach NEXT_PUBLIC_DB_HOST=localhost NEXT_PUBLIC_DB_USER=root
No Reload of Application after Defining The Variables
A common pitfall experienced by many developers is neglecting to reload their applications following the definition of environment variables. Remember, these variables will not be loaded into your application automatically. Outlined below is how it should be done:
// Define your environment variable then ... npm run dev // re-run the application.
No Manual Inclusion of the .Env File
In earlier versions of Next.js, excluding version 9.4 and later, the .env file doesn’t get loaded automatically as per the new updates. Developers would need to manually load it using the
dotenv
package. Here is an example of how it was commonly performed:
require('dotenv').config()
However, keep in mind that in Next.js 9.4.4, the .env file gets loaded automatically. Hence, if you have some code used to manually load the .env file, you should consider rechecking and remove it if not necessary.
Incorrect File Location
The placement of your .env file could also be the cause of failure. Ensure the file resides at the root of your project directory. Moreover, the file should not be pushed to your remote repository (GitHub, GitLab among others). Including .env files in your ‘.gitignore’ can prevent this.
As Tim Berners-Lee, the inventor of the World Wide Web says, “We need diversity of thought in the world to face the new challenges”. So, despite the outlined potential solutions, keep exploring new ways of troubleshooting and fixing issues related to environment variables not working in Next.js 9.4.4
Addressing Common Mishaps with Environment Variables in Next.Js 9.4.4
An interesting topic of note is the management of environment variables in Next.Js. Especially, challenges encountered in the version 9.4.4 where such variables may not work as intended. This scenario might come across as bothersome for developers working with this JavaScript framework.
Understanding Environment Variables and their purpose: Environment variables offer a way to affect the behaviour of software on your system without altering code base. They serve as external configuration that can be altered independently.
Failure of environment variables in Next.js primarily occur due to misinterpretation or misplacement of these variables. The issue usually surface when developers place sensitive data directly in their application code and attempt to utilize .env files for security purposes.
Here are potential root causes of Environment Variables failing in Next.Js 9.4.4:
1. Improper declaration: Names of environment variables must begin with
NEXT_PUBLIC_
. The prefix signifies that they will be embedded into the client-side javascript (React) at build time.
2. Inaccurate placement: You might have placed your environmental variables in a
.env.local
file but failed to include it in your gitignore. If you commit this file to a public repository you could expose sensitive information.
3. Lack of mention in server code: If environment variables aren’t mentioned within the server-side code, they will not function as expected.
Example: <code>process.env.MY_VAR</code> |
Incorrect | Correct |
---|---|
MY_ENV_VAR |
NEXT_PUBLIC_MY_ENV_VAR |
• Ensure that the naming convention for your .env files aligns with Next.js requirements. For instance,
Incorrect | Correct |
---|---|
.envMyApp |
.env or .env.local |
As software engineer Jonny Burger points out, “Programming is as much about understanding the toolset as it is about writing code.” In other words, you must understand Next.Js, its norms, and how to properly configure environment variables. By doing so, and by following the steps outlined in this answer, you can ensure that your environment variables function appropriately.
For additional information on environment variable configuration in Next.js 9.4.4., refer to the official documentation.
Improving Functionality: Best Practices for Using Environment Variables in Next.Js 9.4.4
Enhancing functionality by correctly harnessing the capabilities of environment variables in Next.js 9.4.4 is crucial for any proficient JavaScript developer. When applied accurately, they can prove to be a powerful tool. If you’re struggling because your environment variables are not working as expected in Next.js 9.4.4, there could be several causes and solutions.
Firstly, starting from version 9.4, Next.js has built-in support for loading environment variables from
.env.local
into the Node.js environment. However, this happens specifically during development. Before this update, developers relied heavily on third-party libraries like
dotenv-webpack
or manually loaded variables using custom server code.
Potential Issues | Solutions |
---|---|
Improper Naming | Prefix Variables with NEXT_PUBLIC_ if they’ll be used in the browser |
No Refresh after Addition/Modification | Restart your Next.js Development Server |
Checking Undefined Variabes | Use process.env.YOUR_VAR_NAME || defaultValue pattern |
Improper Naming: Be careful how you name your variables. In order to expose a variable to the browser, you would have to add a prefix:
NEXT_PUBLIC_
to your variable. So if your variable is named
API_KEY
, then it should be renamed to
NEXT_PUBLIC_API_KEY
.
No Refresh after Addition/Modification: Your Next.js development server needs to be restarted whenever you add or modify your environment variables. The updated values will be accessible only post a server restart.
Handling Potential Undefined Variables: During application runtime, some variables could potentially turns up being undefined. To defend against such possible scenarios, employ the
process.env.YOUR_VAR_NAME || defaultValue
pattern.
Ryan Dahl, the creator of Node.js, once said, “Most of the problems in systems software can be boiled down to resource allocation”. Understandably, environment variables serve as a way to allocate resources or configurations based on the specific environment they run in. The tricks shared above will ensure that you are maximizing resource allocation for your Next.js applications.
For more information about environment variables in Next.js 9.4.4., make sure to visit the [Next.js documentation](https://nextjs.org/docs/basic-features/environment-variables).
Environment variables in Next.JS 9.4.4 have irrefutably played a pivotal role in differentiating production and development settings, thus promoting codebase integrity and security. A challenge that has been reported frequently, however, is the non-functional aspect of these environment variables in specific scenarios. The reasons may differ and are determined by several influencing components.
One common stumbling block is incomplete updates on the ‘.env.local’ file. Reloading or restarting your local development server ensures that amendments to this file can take effect. Keep in mind that changes don’t dynamically update during an active session.
Additionally, reserved system keywords could transmit wrong values or cause variable malfunction, leading to silent errors that can be unnoticed for a considerable timeframe. Balancing the urge for familiar names and evading conflicts with reserved words is crucial.
Another potential originator of the non-functionality might be typographical errors occurring in the naming conventions of the variable both in the .env file and where it is being called at. This is often overlooked but maintains high relevance in debugging the problem.
.env File | Calling location |
---|---|
NEXT_PUBLIC_ENV_VAR=MyValue |
process.env.NEXT-PUBLIC-ENV-VAR |
Lastly, be wary of the misuse of prefix ‘NEXT_PUBLIC_’ for variables intended to be exposed to the browser. A failure to use this feature correctly will result in the variable not being embedded within the JavaScript bundle during build time.
As Bill Gates once remarked: “Measuring programming progress by lines of code is like measuring aircraft building progress by weight.” Your productivity as a developer, and the success of your projects, heavily rely on understanding tools and solving problems efficiently. By combatively debugging issues like inoperative environment variables in Next.JS 9.4.4, you gain more profound insight, pick up vital skills and, most importantly, grow stronger in your craft.
For further read: Visit Next.js documentation for comprehensive insight on environment variables.