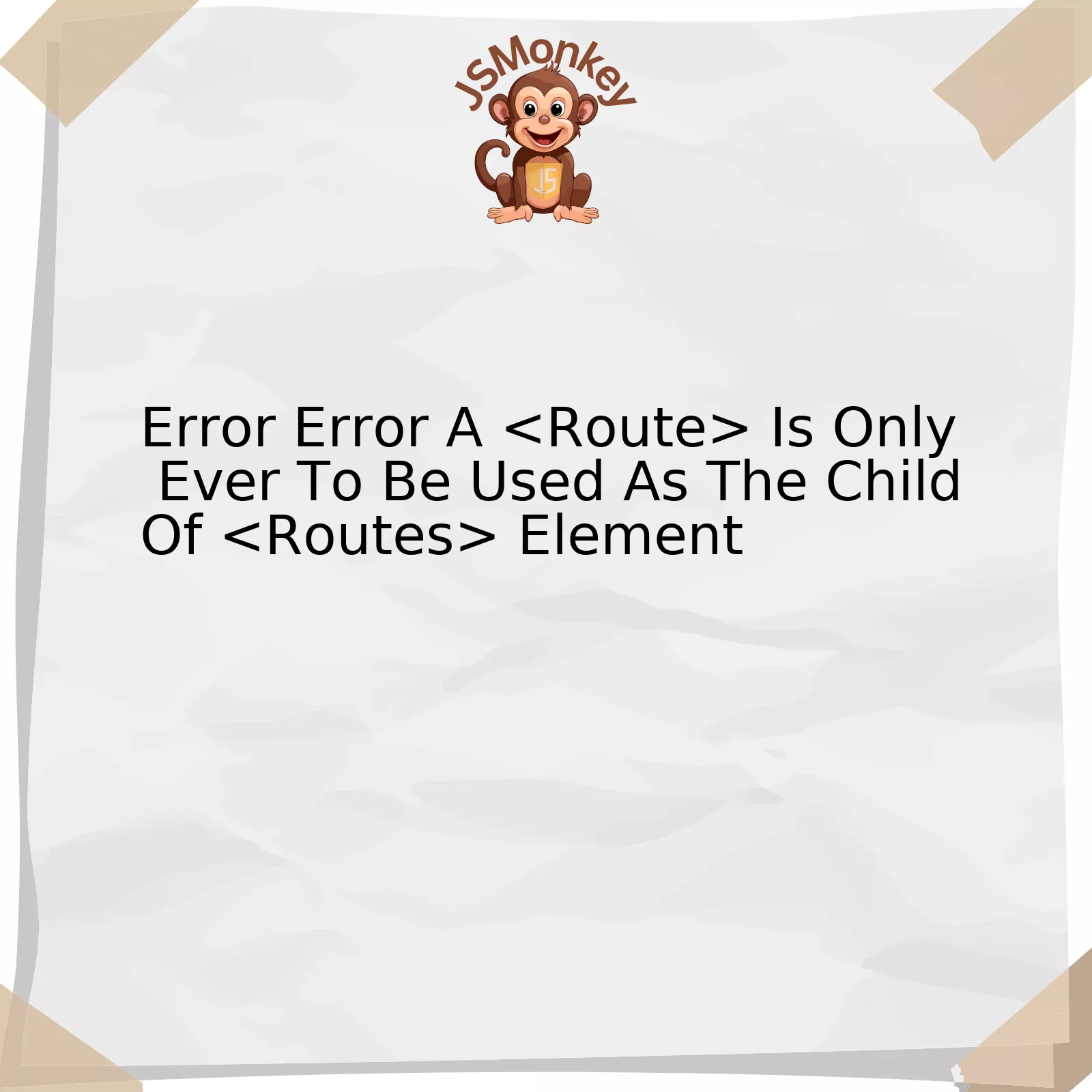
<BrowserRouter>
<Route path="/" element={<Home />} />
</BrowserRouter>
<BrowserRouter>
<Routes>
<Route path="/" element={<Home />} />
</Routes>
</BrowserRouter>
In the first code snippet (Incorrect Usage), the error would occur because the <Route> element is placed directly inside the <BrowserRouter> without being inside a <Routes> component. In contrast, the second block marked as ‘Correct Usage’ presents the right way of embedding <Route>, where it resides within the <Routes> element, thus avoiding the error message.
Assigning <Route> as a direct descendant of a <Routes> wrapper means that React Router can manage various routes across your application more effectively. It provides a centralized location to control route-related behaviors, such as rendering components based on the browser’s URL.
This understanding of structural positioning of routing elements is integral for exploiting the full functionality and dynamism of the React Router library in your React applications.
As Bill Gates once said, “Most people overestimate what they can do in one year and underestimate what they can do in ten years.” In the same way, understanding hierarchies and dependency relationships within your code – while sometimes seeming mundane or inconsequential – can dramatically bolster the effectiveness of your coding projects over time.
Understanding the Role of in React
Diving into React Router, a well-established and popular library among developers for controlling the flow of data and rendering components depending on the path, one can’t overlook the impact it achieves via its
component. However, you may encounter a common error message – “A
The
component serves various capacities. Notably, encapsulating and exhibiting particular interface elements or components when the application’s current location corresponds with the Route’s path. Furthermore, it designates the roadmap for your application in a declarative fashion.
<Routes> <Route path="/" element=<Home /> /> <Route path="/about" element=<About /> /> <Route path="/contact" element=<Contact /> /> </Routes>
Contemplating on the error “A
must exist as a direct child under the
An illustrative misconfiguration causing such an error might be:
<div> <Routes> <Route path="/" element=<Home /> /> </Routes> </div>
In this case, although
nests directly under the
As American computer scientist Donald Knuth beautifully said, “Programs are meant to be read by humans and only incidentally for computers to execute.” The essence underlying React Router’s design – including the described error associated with improper placement of
For more informative analysis and insights, refer to the official React [Router Documentation](https://reactrouter.com/).
Common Errors Encountered with Element
The error message “Error: A <Route> is only ever to be used as the child of <Routes> element” generally signifies a common and foreseeable issue faced by many JavaScript developers while utilizing React Router v6, one of the most commonly employed libraries for routing in React applications. This error typically arises due to violation of a critical routing rule within the application.
Using the <Route> component outside its expected context – that is, the <Routes> component is responsible for this specific error. The structural hierarchy of the routing components plays a pivotal role in understanding the root cause of this problem, as emphasized here:
Component | Description |
---|---|
<Routes> |
This component serves as the container for all <Route> elements in the application. In order to define various routes, each <Route> component must be nested within a single <Routes> parent. |
<Route> |
A <Route> component represents a singular route or path within the application and needs to be declared inside the <Routes> component. Every <Route> component should have two properties – ‘path’ (indicating the URL pattern) and ‘element’ (signifying the React component to render when the route matches). |
Contrary to this hierarchy, attempting to use the <Route> element independently and not as a child of <Routes> prompts the aforementioned error. To resolve this issue, it is vital to adhere strictly to the above-mentioned rule of hierarchy, ensuring each <Route> component falls directly under a <Routes> parent component in your application’s structure.
As elucidated aptly by Facebook’s software engineer Pete Hunt – “The best way to avoid bugs is to learn and understand the core concepts”. This stands true for handling such common routing errors in a React application as well. By comprehending the fundamental purpose and structural hierarchy behind the <Routes> and <Route> components of React Router v6, such mishaps can be effectively mitigated.
Here is an example showcasing correct usage of <Routes> and <Route>:
<Routes> <Route path="/home" element={<Home />} /> <Route path="/about" element={<About />} /> </Routes>
Note that in this snippet, all <Route> elements are correctly positioned as direct child components underneath a single <Routes> component, which complies with the desired hierarchy , therefore avoiding the error in discussion.
For further reading on React Router v6 fundamentals and hierarchy usage, I recommend the official React Router documentation, which provides comprehensive insights on these topics and more
Resolving Issues: Using as a Child of
The error message: “Error: A `
For better understanding, let’s delve into a brief overview of `
HTML:
<Routes> <Route path="/page" element={<Page />} /> <Route path="/about" element={<About />} /> </Routes>
In the above snippet, each `
– `
– `
Back to our error topic, if you encounter “Error: A `
Looking at the syntax, the error might occur if:
– `
– `
– There are multiple nested `
To counteract this, ensure `
Remember this advice from Mark Erikson, one of the maintainers of Redux:
“Just because libraries let you write code does not mean it’s idiomatic or correct.”
And do keep in mind that these issues can also be addressed by thorough reading of the official React Router documentation. Do reach out to the community forums and Stack Overflow for discussions related to niche problems.
In-depth Analysis: Unpacking the ‘ is only used as child of‘ Error
While working with the popular JavaScript library, React.js, and its routing library, React-Router v6, you may encounter a common error: “Error: A `
## Unpacking the Error – What does it mean?
In simple terms, the error message “A `
This error typically appears when a `
Here is a key guideline which is always vital to remember:
If they are not packaged correctly inside `
## Error Resolution
To resolve this error, ensure that all `
html
This hierarchy demonstrates how each `
In as much as coding can sometimes be challenging as Donald E. Knuth said, “We’ve lost sight of the goal that programming should be cheap and fun.” This mindset can help us understand and appreciate the importance of creating well-structured code bases.
To learn more about React-router v6 and its components, you can follow this helpful guide from the [official React-Router-Dom documentation](https://reactrouter.com/).
Wrapping up this discussion, having an understanding about the Error “A
is only ever to be used as the child of
element” can be a game-changer when developing web applications using JavaScript. It’s essential for Javascript developers to have a grasp on how routing works in libraries such as React Router v6 and others.
This particular error message is returned when you try to use a
component outside its intended environment: being a child of a
component. The updated API in version 6 of React Router has made it mandatory to maintain this hierarchical structure, to accommodate advanced features like nested routing and relative linking. If not adhered to, it can lead to undesired results or errors within your application.
Wrong | Right |
---|---|
<Router> <Route path="/" element={<Home />} /> </Router> |
<Routes> <Route path="/" element={<Home />} /> </Routes> |
Mitigating this error involves ensuring correct usage of
and
, keeping them tied together in a parent-child relationship respectively. This design pattern provides clear hierarchy, simplifies route definition and management, hence promoting easier debugging and code maintenance. Thus, fixing such a problem requires modifying your codebase to comply with the stipulated use of these components from the standard React Router library(see the official documentation).
As the famous quote by Linus Torvalds, “Good programmers know what to write. Great ones know what to rewrite (and reuse).” Thus, understanding this error represents an opportunity for developers to learn and grow their skillset, simplifying codebase while ensuring efficient navigation within your Javascript applications. The key takeaway is that making use of components in their intended way, as prescribed by JavaScript libraries such as React Router v6, is critical to effectively leveraging their benefits and building robust applications.