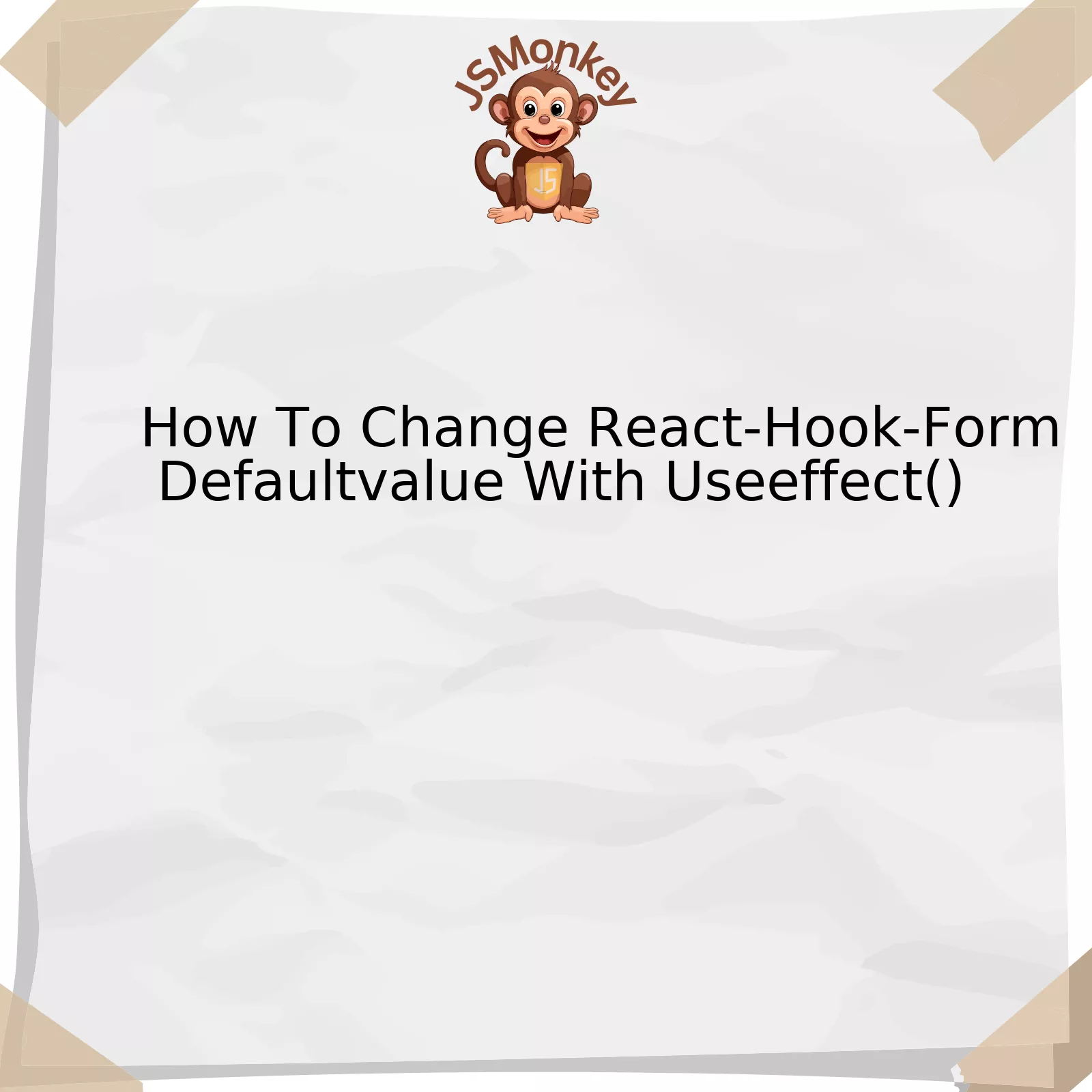
Steps | Description |
---|---|
Import necessary modules and initialize `useForm` | This step involves importing the required hooks from ‘react-hook-form’, namely `useForm`. Initialization of these hooks is then done in your function component. |
Define default values using `useEffect` | The `useEffect` hook allows you to perform side-effects in function components. Here, it’s used to set default value when the component mounts. |
Set new default values | If the data or state changes further down the line, these updated values are assigned as the new default values for the form with useEffect. |
Reset form with new default values | After defining the new default values, these can be applied to the form by calling the reset method from useForm, passing the new defaults as parameters. |
The process operates under specific steps designed to facilitate a smooth operation. Upon initiating your function component, pertinent modules are imported, including `useForm` from the React Hook Form library.
Once the necessary tools are in place, these hooks must be initialized within the functional component. This initialization establishes the groundwork for the user-input fields being managed by React Hook Form.
Subsequently, the `useEffect` hook comes into play. Used to run side-effect operations in functional components, `useEffect` sets the default value upon the component’s mount. It essentially creates an environment that reacts accordingly whenever dependencies undergo changes.
In scenarios where more data changes or state updated occur, a new set default value using useEffect needs to be defined. This step ensures the form is always current and accurately represents the most recent data.
In order to apply new default values to your form fields, a reset method embedded in `useForm` must be utilized. By passing the recently formulated defaults as parameters into this method, you can easily update your form to show the new standard inputs.
Taking into consideration the sage advice of Jeff Atwood, the co-founder Stack Overflow who once remarked, “Coding is not just about building things; it’s about building things that last,” applying React Hook Form in this way emphasizes the sustainable utility of function components alongside the responsive application of hooks and user-induced adjustments.
Understanding React-Hook-Form and DefaultValue
Observing
react-hook-form
, it’s recognized as a form library that minimizes the amount of re-rendering occurring in your React form, meanwhile offering out-of-the box form validation. By using HTML form semantics, it improves overall performance. An integral part of this library is the
defaultValue
attribute used for setting initial values of input fields.
The
defaultValue
can be changed using the
useEffect()
hook, which comes inherently from React. However, larger understanding entails understanding that the
useEffect()
hook is used to perform side effects in function components like data fetching, manual DOM manipulations, etc. The hook is triggered at different stages of component life-cycle depending on its dependencies.
However, suppose you are looking to change the
defaultValue
with
useEffect()
. In that case, it may not yield expected results because
defaultValue
only applies during the initial render of the component. So even if
useEffect()
updates the value later, it won’t reflect in the rendered component.
To achieve such functionality where you want to alter field value post initial render based on certain conditions, you should use the
Controller
component wrapper of
react-hook-form
.
Following is an illustrative representation:
javascript
import { useForm, Controller } from ‘react-hook-form’;
function App() {
const { control } = useForm();
return (
field.onChange(event.target.value.toUpperCase())}
/>
)}
/>
);
}
export default App;
In the example above, any input provided in the wrapped input field is converted to uppercase. This is a simulation of how you could change datatype on
onChange
event.
As Brian Holt (@holtbt) wisely noted,
> “JavaScript’s global scope is like Voldemort’s horcruxes. Don’t put pieces of your soul there unless you absolutely have to.”
It teaches us to keep our state as isolated as possible and understand when to break this rule for necessity’s sake.
Delving into the UseEffect() Function: A Comprehensive Guide
Understanding the useEffect() function in React is paramount to building dynamic and responsive web applications. The cognitive process of understanding this hook function involves disentangling its uses, dependencies, and effects on your code structure.
UseEffect Hook in React
React’s
useEffect()
Hook is integral to controlling side-effects in a functional component. Whether you’re handling data fetching, subscriptions, or manually changing the DOM from React components,
useEffect()
is your go-to tool.
A key takeaway about
useEffect()
is that it runs after every render, including the first one. This makes it adaptable to updating changes on your component.[1](https://reactjs.org/docs/hooks-effect.html).
Changing React-Hook-Form defaultValue with UseEffect
In many scenarios, you may need to change the default value in a React-hook-form using
useEffect()
. To achieve this, you would essentially need
useEffect()
,
reset
from ‘react-hook-form’, and
useState
to manage state.
Let’s consider a hypothetical scenario: A form element whose initial value is fetched from an API and can be updated via user interaction.
Firstly, call the API inside a
useEffect()
function to set the initial state. This approach guarantees that you have the latest default value immediately when you need to reset the form.
html
const [defaultData, setDefaultData] = useState(null); useEffect(() => { fetch("your-api-url") .then((res) => res.json()) .then((json) => setDefaultData(json)); }, []);
Secondly, use the
reset
method from ‘react-hook-form’ to reset the form with the
defaultData
.
html
const { reset } = useForm(); useEffect(() => { if (defaultData) reset(defaultData); }, [defaultData]);
By using this approach, your form will always reset back to the initial default value fetched from the server, whether it was originally null or dynamically updated later.
“Most good programmers do programming not because they expect to get paid or get adulation by the public, but because it is fun to program.” — Linus Torvalds.
References
1. [React useEffect Doc](https://reactjs.org/docs/hooks-effect.html)
Steps to Modify React-Hook-Form DefaultValue with UseEffect()
One of the key factors of dealing with forms in React is to modify default values dynamically based on specific conditions. Here, we would use the power of the useForm hook along with the useEffect hook from React to achieve our goal. The useForm hook comes from the React-Hook-Form library. Below are the steps to follow when modifying the defaultValue property of a field in a form using useForm and useEffect:
Steps | Description |
---|---|
Begin by Importing Necessary Hooks |
Start off by importing useForm hook from ‘react-hook-form’ and useEffect hook from ‘react’.
<script src="https://unpkg.com/react-hook-form@latest/dist/index.umd.js"></script> |
Initialize useForm Hook | After importing, initialize the useForm hook. This would give you methods such as register, handleSubmit, watch and so on which helps us to interact with form fields. |
Create an Input field with Register | Create your form fields (for example, an input field) and implement control by calling register() with the field name within it. This now means that any value inserted into this form field can be accessed through the useForm hook’s functionality. |
Integral UseEffect Hook | We call the useEffect Hook. This hook runs whenever the components it depends on update, this would ensure that any change made to the provided dependencies triggers a rerender. |
Changing the Default Value | In the body of the useEffect hook, we can change the default value of our form field by using the setValue method from useForm. In there, we can modify the default value to whatever dynamic value we wish. |
Note: Usage of these hooks will require you to have a fundamental understanding of how hooks work in React.
Following these instructions accurately would ensure your defaultValue changes dynamically depending on the situation. This is particularly necessary in scenarios where your form fields depend on other variables or state values in your application.
The great aspect about this approach is that you are leaning on existing tools within the React ecosystem “React.Hooks” which simplifies managing the form states within your components as backed up here.
Ultimately, when it comes to UI development – whether web or mobile, having basic knowledge and working with forms are essential. As developers, we need all the techniques possible such as dynamic form values, to make the UX better and more interactive – Ethan Marcotte.
Remember the crucial principle of coding is learning to solve problems innovatively. Hence, modifying the defaultValue with useForm and useEffect isn’t just a way to overcome an issue but also an adoption of a technique that can be used adventurously across numerous projects.
Practical Applications and Best Practices in Changing React-Hook-Form Defaultvalue With Useeffect()
When we talk about changing the `defaultValue` in a react-hook-form, `useEffect()` plays a crucial role. The main usage of `useEffect()` in this scenario is when you want to set a default value for your form fields based on asynchronous data.
Using `react-hook-form` together with React’s `useEffect()` to change default value makes form handling in React applications significantly cleaner and more manageable. Here’s how it all comes together:
A common use case where `useEffect()` is quite useful is when you’re fetching data from an API and then populating the form fields once the data is available.
Here is a basic example of how it works:
import { useEffect } from 'react'; import { useForm } from 'react-hook-form'; function App() { const { register, setValue } = useForm(); // Fetching data (simulated here with a Promise) const fetchData = () => new Promise((resolve) => setTimeout(() => resolve('Data fetched from API'), 2000)); useEffect(() => { fetchData().then((data) => { // setting up input's value setValue('myFieldName', data); }); }, []); return ( <form> <input {...register('myFieldName')} /> </form> ); } export default App;
In this sample code snippet, a call to an API or other asynchronous task (we emulate that with a Promise) happens within the `useEffect()`. Once the API returns the data, `setValue()` from `react-hook-form` is invoked to set the value of the field.
It’s essential to note the following points for best practices:
– Make sure you add all dependencies to the dependency array of `useEffect()`. This will prevent unnecessary re-renders and API calls. In our example, an empty array is provided, which means the `useEffect()` will be run once, similar to componentDidMount in class components.
– Be aware of the cleanup function in `useEffect()`, which you could use to cancel any ongoing API requests when the component unmounts.
Steve Jobs once said, “Details matter, it’s worth waiting to get it right”. With this in mind, understanding how to properly utilize hook like `useEffect()` alongside libraries such as react-hook-form can truly push your React applications to the next level and beyond.
For additional reading pleased find [The React Help Page](https://reactjs.org/docs/hooks-reference.html). For even more detailed guidance and information on `react-hook-form` please visit their official [Documentation Page](https://react-hook-form.com/api/useform/register).
The process of changing the default value with `useEffect()`, in a react-hook-form, is a concept that commands thorough understanding and correct application. This particularly needs emphasis on three core stages – integrating React hooks, setting the default value, and making modifications with `useEffect()`.
“The only way to make sense out of change is to plunge into it, move with it, and join the dance.” – Alan Watts
React hooks, a revolutionary feature introduced in React 16.8 version, has led developers to reuse stateful logic and enable components to have a state under functional components. The react-hook-form, which comes bundled with features like fast re-rendering and build optimization, allows you to work even more efficiently with these hooks.
Integrating React hooks into your application aids in managing your form state within the component’s functional structure. This fits naturally into introducing useState for local variable management, providing you an upper hand when dealing with form fields.
Within the realm of react-hook-form, setting the default value plays a crucial role when intending to present the user with pre-filled field values. This eases user interaction, particularly in scenarios where you are handling update operations or building forms reliant on previous data.
However, recalibrating this default value necessitates familiarity with `useEffect()`. As a part of React hooks, `useEffect()` enables side effects inside function components—one such side effect being the alteration of defaultValues in react-hook-form. By utilizing dependencies array in `useEffect()`, you can orchestrate changes based on events or prop/state modifications.
This entire approach might seem a chunk of complex tasks assembled together. Yet, these strides are highly rewarding as they empower your forms with dynamic defaults while keeping up with the ever-evolving ecosystem of users’ expectations.
Read More about useEffect()
Read More about DefaultValues
Hence, remember: While working with react-hook-form and defaultValues, `useEffect()` is not just a tool but an extension of your developer’s prowess for engaging user interaction.