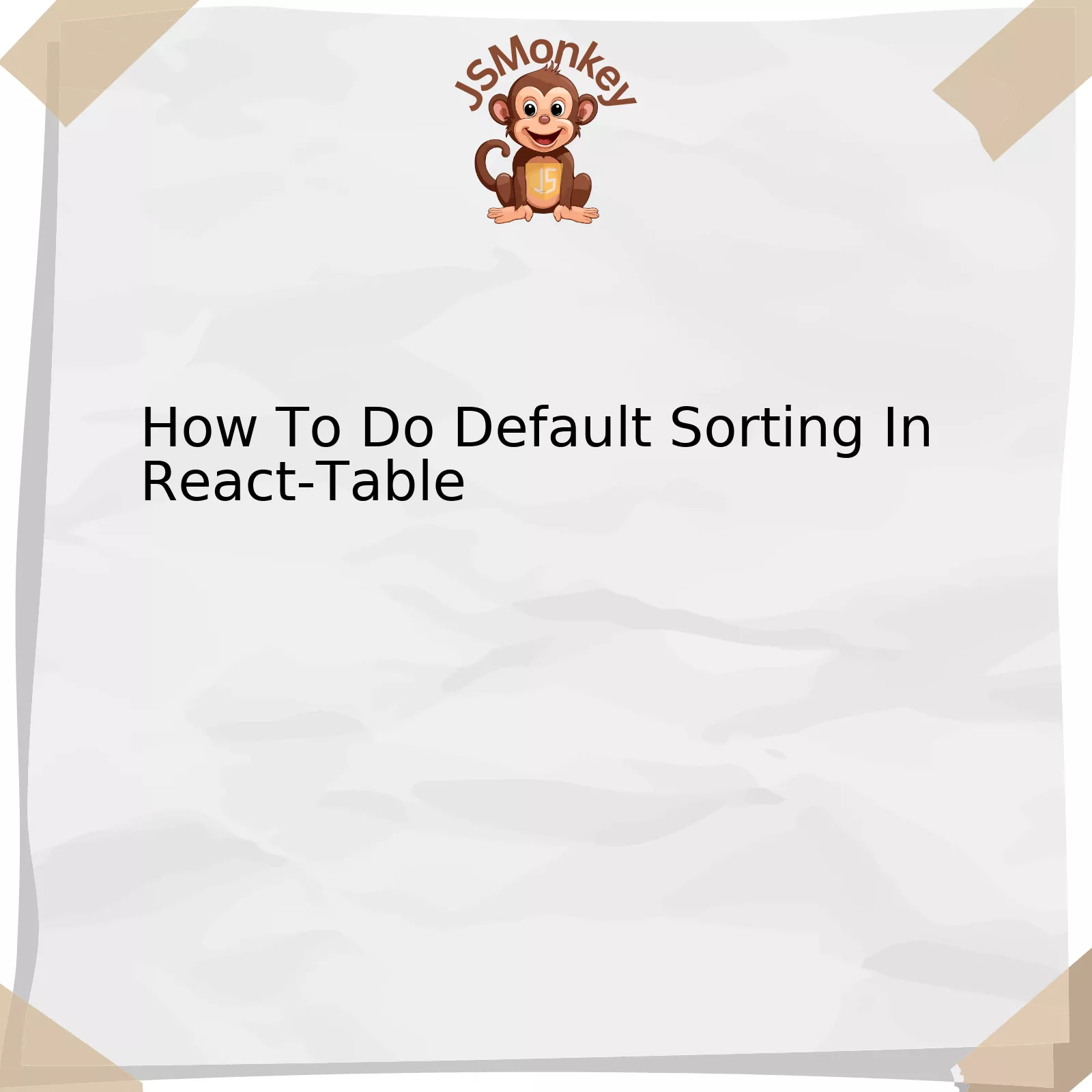
React-Table is a highly flexible and adaptable library that’s leveraged by the React.js framework to build interactive data tables. A particularly compelling feature of React-Table is its ability to facilitate default sorting of table columns. Let’s delve into an example to get a more practical understanding of how this works.
When setting up our data structure for a React-Table, we can specify the `defaultSortDesc` property for each column. If you set it to true, your column will be sorted in descending order by default; if you set it to false or exclude it altogether, the column will be sorted in ascending order by default.
Here’s a simple representation:
const columns = React.useMemo( () => [ { Header: 'Age', accessor: 'age', // accessor is the "key" in the data defaultSortDesc: true, }, ... ], [] ) return ()
In this code snippet, the ‘Age’ column will be shown in a descending order as soon as the table renders because the `defaultSortDesc` property for the ‘Age’ column is set to true.
React-Table also has a `manualSorting` prop which disables automatic sort when a user clicks on a column header. Instead, it triggers a `handleSortChange` function where you can implement any custom sorting logic.
Notably, despite offloading many complex operations to developers, React-Table offers a robust layer of abstraction for these types of interface behaviors. When using the React-Table library, it’s recommended to spend time familiarizing yourself with nature of its flexibility in order to fully leverage its capabilities – something Ken Wheeler, a well-known figure in the JavaScript community has echoed, stating, “JavaScript, at once stable and flexible, becomes most powerful in the hands of a developer who understands its potential and knows how to harness it.”
For further reading on default sorting or additional features within the React-Table library, their official documentation is an excellent resource.
Utilizing Built-in Features for Default Sorting in React-Table
Utilizing built-in features for default sorting in React-Table is indeed an essential part of making your application efficient and user-friendly. With React-Table, you have access to a variety of customizable settings to sort data according to user preferences or application requirements.
React-Table uses the attribute named
defaultSortDesc
for setting the default sorting option. This property can accept a boolean value, where true denotes descending order and false signifies ascending order.
In essence, React-Table allows developers to set an array of objects as columns. Each object corresponding to a certain column can possess properties such as id, accessor and others necessary for specific functionalities. The property
defaultSortDesc
, when set on column definition level, will govern the sort direction of a particular column by default.
Let’s conduct the examination through a detailed example. Suppose we are working with a dataset that consists of user information including name, email and registration date. If you would like the table to display this data sorted by registration date in descending order by default, use the following structure:
html
When implementing this within React-Table, the resulting output shows users listed from the most recent registration date down to the earliest based on the use of the
defaultSortDesc: true
configuration within the ‘Registration Date’ object.
This is echoed by a quote from Jeff Atwood, a renowned software developer and author who stated, “We should forget about small efficiencies, say about 97% of the time: premature optimization is the root of all evil. Yet we should not pass up our opportunities in that critical 3%.” This encapsulates the need for adopting necessary optimization measures, like setting default sorting in important areas like in a data-table component to improve user experience and operational efficiency [source].
Do note, however, that implementing default sorting does not prevent subsequent user-indicated sort preferences. The UI will still respond to clicks on each column header, alternating between ascending, descending and normal states.
Keep in mind that continuous exploration of library methods and built-in features is synonymous with React-Table optimal utility. It presents in-built solutions (like the default sorting) that enable seamless customization tailored toward app-specific needs. Harness these features to build optimal table formats, nurture better user engagement, and foster an overall efficient application environment.
Understanding the Principles behind Default Sorting with React-Table
Understanding the principles behind default sorting with React-Table, a popular library for creating tables in React application, is an indispensable part of facilitating effective data presentation. By employing default sorting, developers can present data to users in a more organized and user-friendly manner.
The fundamental principle behind default sorting lies in the initial configuration of the table data. React-Table’s flexibility allows for setting up default sorting within your table columns and using custom sorting methods
useSortBy
for desired outputs.
How then do we implement default sorting?
First, you define the column where sorting should be applied by default with the property ‘defaultSortedDesc’. Then, set the value of this property to provide either ascending or descending sorts.
An example would be:
const columns = [{ Header: 'Name', accessor: 'name', defaultSortDesc: true }]
Here, it means that when the table loads the first time, the ‘name’ column will be sorted in descending order by default.
In addition, React-Table permits defining multiple default sorting criteria by configuring multiple fields with the ‘defaultSortDesc’ property:
const columns = [ { Header: 'Name', accessor: 'name', defaultSortDesc: true }, { Header: 'Age', accessor: 'age', defaultSortDesc: false } ]
In these examples highlighted, the ‘Name’ column is sorted in descending order first, and then the ‘Age’ column is sorted in ascending order.
It is noteworthy, however, that sufficing to only shape the default sorting by structuring columns is not satisfactory. You must also utilize the hook ‘useSortBy’ in the table instance creation process:
const instance = useTable({ columns, data }, useSortBy)
In the famous words of Brian Kernighan, “Controlling complexity is the essence of computer programming.” This best describes why using default sorting in React-Table is so vital. It simplifies the user interface, thereby making your application more accessible and enjoyable to your audience.
Additionally, React-Table provides custom sorting algorithms. For complex data structures, you may define your sorting function in the column definition.
To ensure a robust and seamless default sorting process, it’s crucial to ensure proper usage of both the ‘defaultSortDesc’ property and the ‘useSortBy’ hook. Achieving this not only provides an initial ordering for your data, it also significantly enhances user experience — a critical consideration in any web development project.
Comprehensive Guide to Implementing Default Sorting on React-Table
The React-Table library is one flexible and customizable tool that React developers have found great utility in when dealing with tabular data. One such customizable feature is sorting; and to be specific, default sorting. The following guide will break down how you may go about setting a default sort rule on your React-Table instance.
To begin, it’s important to recognize that sorting in React-Table revolves around the `useSortBy` hook. By incorporating this hook into your table instance, you open up access to properties and functions catered towards sorting through columns.
An essential step in initiating a sort upon the loading of your table data, also known as default sorting, lies in defining your column structure. Each column has an object of options assigned to it, some of which directly influence sorting.
const data = React.useMemo( () => [ { col1: 'Hello', col2: 'World' }, /*... more data ... */ ], [] ) const columns = React.useMemo( () => [ { Header: 'Column 1', accessor: 'col1', // identifier for the field defaultSortDesc: true // sorts in descending order by default }, { Header: 'Column 2', accessor: 'col2', }, /* ... more columns ... */ ], [] )
In the example above, `defaultSortDesc` is set to true in Column 1’s configuration. This indicates that upon initialization, the table will be sorted according to that column in descending order. If instead you wanted ascending order, you would assign `defaultSortDesc` as false.
The ending result is that users will initially see your data ordered based on these parameters, providing supplemental user experience, guiding focus towards sections of data deemed most important.
THE `useTable` HOOK: In order to integrate these options and reflect them in our UI, pass your defined columns and data arrays to the useTable hook. Including its second argument `useSortBy`.
const { getTableProps, /* other properties */ } = useTable( { columns, data }, useSortBy /* other plugins */ )
Once the groundwork is laid out, it’s then just a matter of invoking these features in your HTML return statement with the `getTableProps()` function that was deconstructed from the `useTable` hook above.
It can be seen that sorting in React-Table isn’t as daunting a task as it may initially seem. With a solid understanding of column configuration and a few inbuilt hooks at hand, you’re well on your way to having a fully-functional tabular display. Remember, better tables lead to better data comprehension, and in the end, this will mean a better website.
As Tim Berners-Lee, the inventor of the World Wide Web, once said, “Data is a precious thing and will last longer than the systems themselves.”
Tips and Tricks: Enhancing User Experience with Default Sorting in React-table
Following the principle of enhancing user experience, one of the key aspects that can be leveled up in your web application is the implementation of default sorting when using react-table. This feature not only makes data presentation more coherent but also helps users to understand and interpret the displayed information more readily.
Now, here’s an insightful approach on how to do default sorting with React-Table:
React-Table, a flexible and lightweight grid library written in JavaScript, allows you to easily default sort your table using `useSortBy` hook alongside the initial state object (`initialState`). The `useSortBy` hook manages all sort-based actions and states for your table.
Below are step-by-step instructions on how to set this up:
1. Import the `useSortBy` hook from react-table in your component file:
import { useTable, useSortBy } from 'react-table';
2. Set your data and columns for the table:
const data = React.useMemo( () => [ { col1: 'Hello', col2: 'World', }, //... additional data ], []); const columns = React.useMemo( () => [ { Header: 'Column 1', accessor: 'col1', }, { Header: 'Column 2', accessor: 'col2', }, // ... additional column config ], []);
3. Initialize the useTable hook with your data columns and initial state values for sorting:
const initialState = { sortBy: [{ id: 'col1', desc: false }] }; const { getTableProps, getTableBodyProps, headerGroups, rows, prepareRow } = useTable({ columns, data, initialState }, useSortBy);
4. Render your table as desired using the methods given by the hook.
In context of optimization, ensuring your tables are sorted by default not only brings out an organized and systematic layout but also adds to user convenience.
“If you want users to love your software, make it understandable, faster, and easier,” shared Eleni Gabre-Madhin, an advocate of making information accessible and digestible. Therefore, React-Table offers a perfect way-out to support these attributes seamlessly. Refer to the official React Table documentation for more details or comprehensive understanding about its sorting options and commands.
Following an examination of how to implement default sorting in React-Table, it’s demonstrated that the application of this feature significantly heightens the user interaction experience by providing a more organized display of data. This operation can simply be facilitated using the
defaultSortDesc
and
defaultSorted
prop types.
React-Table comes with out-of-the-box capabilities, making it a popular choice among developers looking to enhance their web development dynamics with comprehensive table features. Its extraordinary capacity to handle numerous data aspects – particularly the component of default sorting – sets the bar high for other JavaScript libraries.
In order to illustrate the sorting implementation process, let’s consider this snippet as our data handler function.
useTable( { data, columns, initialState: { sortBy: [{ id: 'yourUniqueIdentifier', desc: true }] }, }, useSortBy )
Here, the
initialState
attribute set within your data handler enables you to define which column should be sorted by default when the table loads. The ‘id’ part corresponds to the unique identifier of your chosen column, while ‘desc’ denotes the type of sort (true for descending, false for ascending).
Furthermore, if you want to render an explicitly sorted column for users even before they interact with your data table, you’d leverage the
defaultSorted
attribute like so:
{ Header: 'HeaderTitle', accessorr: 'accessorName', defaultSortDesc: true }
Above, the
defaultSortDesc
option determines the default sorting order. Set this to true for descending, false for ascending.
To gain more insights about working with React-Table, I recommend checking the [official documentation] for an in-depth understanding of its broad functionality.
As Alan Kay, a celebrated Computer Scientist poignantly stated, “Simple things should be simple, complex things should be possible.” This quote aptly encapsulates the essence of React-Table. With its simplistic design and extensive capabilities, you are not only ensured an uncomplicated programming journey but also a sea of possibilities to explore with table manipulation.