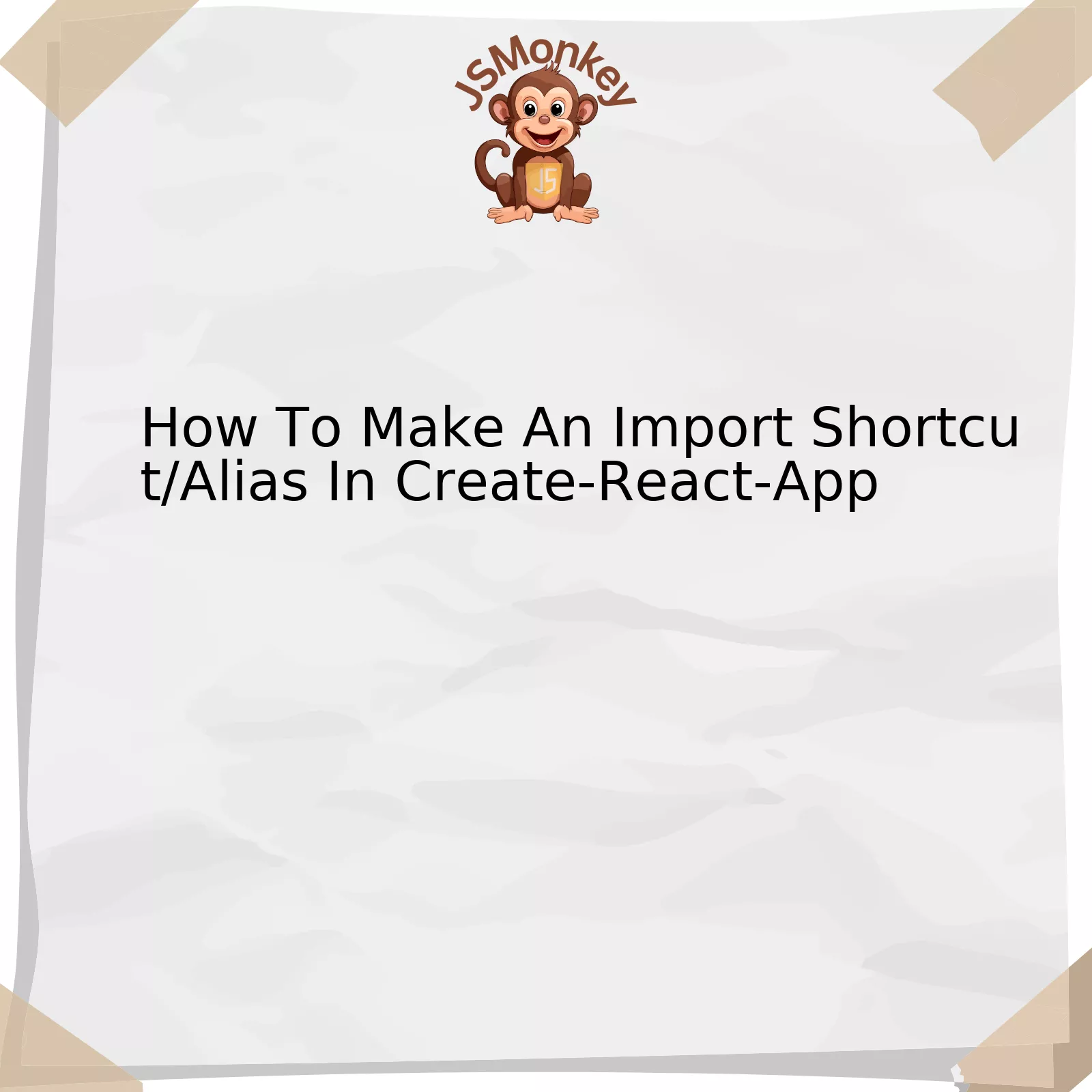
Step | Description |
---|---|
Initial Setup | Create a new React application by running the command
npx create-react-app my-app in your terminal. |
Add .env File | Create a file called .env in the root of your project. This will hold your environment-specific variables. |
Add Path Aliases | In the .env file, add aliases for paths that you frequently use like
REACT_APP_COMPONENTS=./src/components . The keys must start with REACT_APP_ . |
Import Usage | To import files using an alias, utilize the syntax:
import MyComponent from 'components/MyComponent' |
Restart Server | If your development server was running while adding these changes, shut it down and restart it for the aliases to take effect. |
Path aliasing is a powerful feature that makes maintainable and scalable code easier in large applications. We often follow a certain file structure in apps and find ourselves traversing through many folders to import different components or utilities. Over time, this can become tedious and lead to cluttered import statements.
With Create-React-App (CRA), we can assign alias routes that map to specific directories. This enables to have neater, succinct, and efficient import statements. Setting up import shortcuts might seem like an unnecessary task for smaller projects, nonetheless, it becomes invaluable as the project size increases.
The first step is to create a new React application if you haven’t done this already. By executing
npx create-react-app my-app
in your terminal, a fresh CRA setup is initiated. With your app created and set up, move on to creating an .env file within the root directory of your project. This file will contain all your environment-specific variables, including paths.
For instance, you may have a folder named ‘components’ that you frequently access. In the .env file, assign an alias to this path. The important thing to remember is that all variable names should begin with `REACT_APP_`. So, it would look something like this:
REACT_APP_COMPONENTS=./src/components
.
With aliases added, you can import files using these routes instead. For instance,
import MyComponent from 'components/MyComponent'
. You’ll notice that our import statement is crisp and eliminates the need to traverse the elaborate directory structure.
Note: If your development server was running while making these changes, shut it down, and run
npm start
again to restart the server. This enables the applied changes to kick-in and make use of the newly defined path aliases.
As Don Knuth, a renowned computer scientist, said, “Programs must be written for people to read, and only incidentally for machines to execute.” Creating import aliases opens avenues for increased developer productivity, enhanced code readability, and better project maintainability.
Understanding Create-React-App Import Shortcuts: Key Benefits
Utilizing import shortcuts, or ‘aliases,’ in Create-React-App (CRA) proves to be a beneficial practice for any JavaScript developer aiming at maintainable and readable code. CRA, as you may know, is a highly esteemed tool used to bootstrap React applications from the ground up without having to deal with complex configurations.
Creating import aliases essentially involves defining a short, unique identifier that pertains to an import path inside your application. When you need to import something from this path, you merely call its alias instead of writing out the full lengthy path. To showcase the difference between both approaches:
With default imports:
import SomeComponent from '../../../../components/SomeComponent';
With import aliases:
import SomeComponent from '@components/SomeComponent';
Here are some key benefits of utilizing such practice:
• **Convenience**: Coding becomes more streamlined when you’re no longer typing out long relative paths.
• **Cleanliness**: A clean codebase is always appreciated by developers. Import shortcuts uphold this spirit by removing cluttering paths.
• **Efficiency**: Time spent searching through directories can be reduced significantly.
• **Maintainability**: If folders get restructured, only the alias would need an update over going through every file that imports from that folder.
• **Readability**: By naming aliases intuitively, any developer can understand where the import is coming from.
There are some steps involved when setting up these import shortcuts in your CRA project:
**1. Installing necessary plugins:**
Firstly, you’ll need to install `babel-plugin-module-resolver`. This Babel plugin helps in remapping the import paths.
npm install --save-dev babel-plugin-module-resolver
**2. Configuring .babelrc or babel.config.js:**
After installation, the next step is the configuration of `.babelrc` or `babel.config.js` files. Let’s use `.babelrc` as an example:
{ "plugins": [ ["module-resolver", { "root": ["./src"], "alias": { "@components": "./src/components", "@utils": "./src/utils/" } }] ] }
Here, `root` is set to `./src`. This means that the root directory for your application code starts at this level. The aliases are then defined under `alias`.
**3. Configure jsconfig.json:**
Also, Create React App supports `jsconfig.json` out of the box which we set up similar to `.babelrc`:
{ "compilerOptions": { "baseUrl": "src", "paths": { "@components/*": ["components/*"], "@utils/*": ["utils/*"] } } }
With these steps undertaken, you can now import a file from your defined paths easily using your shortcuts.
If these abilities amaze you, it’s time to astonish yourself even more with Steve Jobs’ insightful words on technology: “Innovation doesn’t mean saying yes to everything. It says no to thousands of things to be sure that we don’t get sidetracked focusing on the irrelevant.”
To import and manage modules conveniently, it seems like Mr. Jobs would agree that import shortcuts in CRA are indeed focused and relevant.
Implementing Import Shortcuts or Aliases in Your Create-React-App
Working with Create-React-App (CRA) offers a simplified developer experience in building single-page React applications. By default, however, CRA doesn’t support import shortcuts/aliases – a feature that can make managing your file and import structures significantly easier and efficient.
To implement these import shortcuts or aliases in your CRA without ejecting, you can introduce additional configurations, such as those offered by third-party modules like CRACO (Create-React-App-Configuration-Override) and Module Resolver Babel Plugin.
The following steps illustrate how to make an import shortcut/alias in CRA using the CRACO and Babel Plugin Module Resolver:
1. Installing Required Modules:
First, we need to install the necessary dependencies to our project. Run following command lines to install both CRACO and the Babel plugin:
npm install @craco/craco npm install babel-plugin-module-resolver
2. Updating Scripts:
Once the above installations are complete, update your package.json file’s scripts section to use CRACO instead of react-scripts. This change ensures CRACO handles the application startup configuration, enabling us to slightly bend CRA’s fixed webpack configurations.
json
"scripts": { "start": "react-scripts start", "build": "react-scripts build", "test": "react-scripts test", "eject": "react-scripts eject" }
to
json
"scripts": { "start": "craco start", "build": "craco build", "test": "craco test", "eject": "react-scripts eject" }
3. Creating Configuration File:
In your project root directory, create a new craco.config.js file. This is where we will configure our path aliases.
4. Setting Up Aliases:
Inside the craco.config.js file, we can define our import shortcuts or aliases. For example:
javascript
const path = require(`path`); module.exports = { babel: { plugins: [ [ 'module-resolver', { root: [path.resolve('./src')], alias: { components: './components', utils: './utils' } } ] ], }, };
In this configuration, ‘components’ and ‘utils’ represent shortcut aliases for their respective directories relative to the src directory.
With these steps completed, you have now successfully configured import shortcuts or aliases in your create-react-app. As you might notice, such configurations significantly simplify and enhance your code’s readability, especially when dealing with larger applications that include deeply nested file structures.
Remember what Abelson and Sussman once said about Computer Science, “Programs must be written for people to read, and only incidentally for machines to execute.” Keeping your code readable and maintainable is a crux of effective programming. Thus, shortcuts/aliases are one example on how modern day JavaScript frameworks like ReactJS ease out the complexity involved in managing larger projects.
Step-by-step process to Make an Effective Import Shortcut/Alias in Create-React-App
The process of creating import shortcuts or aliases in Create-React-App necessitates an understanding of JavaScript modules and webpack configuration. This can simplify and streamline your code by removing the need for convoluted relative path imports.
Here are the steps to accomplish this:
1. Install Required Packages
Firstly, install the required packages using npm or Yarn:
npm install --save-dev react-app-rewired customize-cra
or
yarn add --dev react-app-rewired customize-cra
These packages allow you to override Create-React-App’s webpack configurations without ejecting.
2. Configure package.json
Next, update the `scripts` section of your `package.json` file. Replace
"react-scripts"
with
"react-app-rewired"
.
For instance, you would change:
"start": "react-scripts start"
to:
"start": "react-app-rewired start"
Perform similar changes in test, build and eject scripts.
3. Set Up config-overrides.js
Create `config-overrides.js` at the project root level. Assign it the following settings:
const { alias } = require('react-app-rewire-alias') module.exports = alias({ 'Components': './src/components', 'Utils': './src/utils' })
This example creates aliases for components and utilities directories within the source directory.
4. Use Your Aliases
Now, instead of importing a component utilizing a relative path – for instance:
import MyComponent from '../../../../components/MyComponent'
You can simply use:
import MyComponent from 'Components/MyComponent'
This process helps in simplifying your imports and enhances the readability of your code. Creating aliases can be particularly advantageous when working with complex projects that contain a multitude of directories and subdirectories. It’s important to note that Webpack is ultimately responsible for managing these import paths [1].
As Kent Beck, an American software engineer and the creator of extreme programming, once said, “I’m not a great programmer; I’m just a good programmer with great habits.” Adopting the practice of using import shortcuts or alias in Create-React-App is one such habit that can ultimately lead to an improvement in overall coding quality.
Troubleshooting Common Issues with Creating Import Alias using Create React App
Troubleshooting common issues while setting up import shortcuts/aliases within Create React App can indeed be tricky. However, understanding the underlying causes for some of these issues could provide the roadmap to resolving them. Keep in mind that the main intention behind using import aliases is to create a scalable and maintainable codebase by organizing imports neatly, thereby enhancing developer experience.
One common issue observed while creating import alias might be related to non-recognition of alias. You may have set an alias in your webpack configuration but the application does not seem to interpret it correctly. The underlying cause here could be a misconfiguration, improper usage of alias, or even incorrect path specification.
Solution:
Take note of how Create-React-App handles customization of Webpack configurations. Unlike applications initialized with custom tools where you have direct access to webpack config file, Create-React-App abstracts this away, which means you don’t modify webpack.config.js directly. Instead, you would need to “eject” the application using
npm run eject
command or use tools like react-app-rewired (which allows you to customize your configurations without ejecting). After this step, check that the correct paths are aliased correctly in your configuration.
Another common problem stems from JavaScript testing frameworks, specifically Jest, failing to resolve modules by their aliases.
Solution:
Just like webpack, Jest configuration needs to be updated to understand module aliasing. In Jest’s case, there exists a configuration option called ‘moduleNameMapper’ that allows regex style matching on your module imports. By providing a mapping of regex -> directory path, Jest will properly route your tests and component files based on your moduleNameMapper that resides inside your package.json.
Aaron Russo once noted, “Computers make fast, very accurate mistakes.” In a similar vein, failing to reboot your development server during the configuration changes could cause persisting issues.
Solution:
Restart your development server. Many times, caching or warm start of the development server might not reflect the new changes in webpack alias understanding. Restarting the server would clear those temporary files and pick up the updated config files effectively.
In conclusion, while setting up import shortcuts/aliases is a beneficial process, it’s rarely without potential pitfalls. Establishing an understanding of these problem causes and their corresponding solutions can aid your troubleshooting experience within Create React App environment amiably.
Trouble | Resolving Steps |
---|---|
Alias Not Recognized | Update Webpack Configurations |
Jest Cannot Resolve Alias | Modify moduleNameMapper in Jest Config |
Persisting Alias Configuration Issues | Restart Development Server |
Remember, as much as code organization is important for any application, completely grasping the architecture of the tools used also plays a crucial role in actively debugging associated issues.
In order to simplify the process of importing files in a Create-React-App project, one can leverage Webpack’s resolve.alias property behind the scenes. An import shortcut or alias facilitates easy accessibility to directories, preventing us from dealing with relative paths in deeper components.
Let’s delve further to grasp this concept:
A practical demonstration:
Say you have a folder called ‘components’, housed inside the ‘src’ directory. If you are in a deeply nested component and need to import another component from ‘components’, you would typically use a relative path, something like ‘../../../components/ComponentName’.
Transform into an alias:
You can convert it to an alias, for instance ‘@component’. Once this happens, irrespective of which file you are presently working on, you just need to refer ‘@component/ComponentName’ to import any file from your ‘components’ directory.
Create-React-App & Custom Paths Module:
Since ejecting a create-react-app can be cumbersome, a package named customize-cra could be used to override webpack configurations without ejecting. It is paired with ‘react-app-rewired’ to provide straightforward customization control.
npm install --save-dev react-app-rewired customize-cra
In your package.json file, tweak your scripts section as follows:
{ /* ... */ "scripts": { "start": "react-app-rewired start", "build": "react-app-rewired build", "test": "react-app-rewired test", "eject": "react-scripts eject" } }
To add an alias handler, a new
config-overrides.js
file should be created at the root level, where you implement
addWebpackAlias
, adding shortcuts as per need.
const path = require('path'); const { override, addWebpackAlias } = require('customize-cra'); module.exports = override( addWebpackAlias({ ['@components']: path.resolve(__dirname, 'src/components') }) );
This configuration allows React to recognize ‘@components’ as a shortcut for the components directory.
As Andrew Biggadike rightly said, “Well-engineered code is just as stimulating as well-designed software architecture.” In conclusion, leveraging the aliasing provision in webpack configurations in a Create-React-App project promotes code manageability, readability and efficiency significantly.