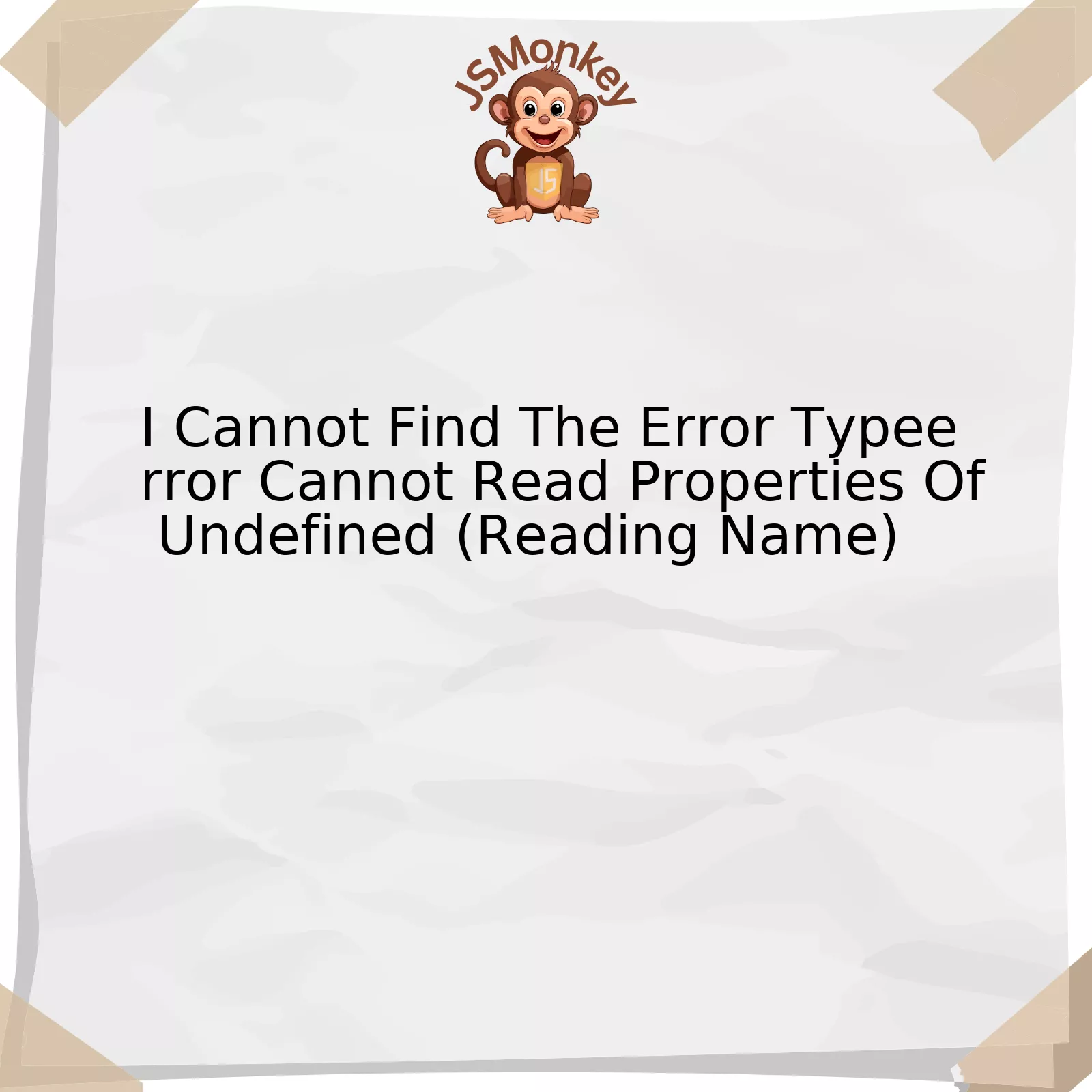
The error message “TypeError: Cannot read properties of undefined (reading name)” commonly occurs when JavaScript attempts to access a property or method of an object that’s undefined. It implies that at a certain point, your code is trying to read the property `name` of something that does not exist. Here is a structure explaining the scenario:
Error | Cause | Solution |
---|---|---|
“TypeError: Cannot read properties of undefined (reading ‘name’)” | This error typically originates from attempting to use a property or method on a variable that’s currently undefined. | To rectify this, ensure that the variable you’re referring to has been defined before you attempt to access its properties or methods. |
Picture this real-world scenario: You have an Object `employee` which should contain the property `name`.
var employee = { id: 1, location: "USA" }; console.log(employee.name.first);
Here, the console would log an error that says “TypeError: Cannot read property ‘first’ of undefined”, because there isn’t a property called `name` in your `employee` object, yet you’re trying to access `first` from it.
To resolve such issues, check whether the parent object or property exists before referring to its child attributes. Applying this to our example, the fix would look like this:
var employee = { id: 1, location: "USA" }; if (employee.name) { console.log(employee.name.first); } else { console.log('Name is not defined'); }
As Steve McConnell, author of Code Complete, reminds us: “Good code is its own best documentation.” This ORM error is a prime example of how code errors can often be traced back to simple omissions or oversights during programming. Applying diligent checks, employing thoughtful debugging, and maintaining strict adherence to defined data structures will guard against such issues.
Understanding the TypeError: ‘Cannot Read Properties of Undefined’
The error “TypeError: Cannot read properties of undefined (reading ‘name’)” in JavaScript is quite a common one, and can often be difficult to resolve. This error message means that your code is trying to access an object property (in this case named ‘name’) on something that’s `undefined`. Typically, this issue arises from unsuccessful or incorrect attempts to reference a variable or function, potentially arising from scoping issues, asynchronous operations misunderstanding or inherent code hazards such as null values.
1. Variable Or Function Is Not Yet Defined:
Here is a code snippet illustrating this type of error:
let person; console.log(person.name);
In the above example, person is undefined because we have not assigned any value to it; when we try to get access to the ‘name’ property of ‘person’, it throws a TypeError.
2. Incorrect Scoping:
Another possibility where the error might emerge is when you try to reference a variable or function outside of its scope.
For instance, while using a local scope variable in a global scope:
function showPerson() { let person = { name: "John Doe" }; } console.log(showPerson().name);
In this example, ‘person’ does not exist in the global scope, thus calling ‘showPerson()’ returns ‘undefined’. Trying to call ‘name’ of ‘undefined’ raises the TypeError.
3. Asynchronous Operations:
This error could also occur if your code includes asynchronous operations, and you’re attempting to access the data before it has been loaded.
4. Null Values:
Using dot notation with NULL values will also throw this error as they don’t contain any properties.
Reading errors is crucial in understanding how JavaScript interacts with your website or application. Additionally, various debugging tools can assist in diagnosing and resolving these issues.
To substantially reduce the occurrence of such errors, always initialize your variables and ensure that you’re not trying to access information before it is available. Also, consider using `typeof` or optional chaining (`?.`) for preventing runtime TypeError problems.
As Steve McConnell mentioned, “Good code is its own best documentation.” Ensuring your code is clean, well-commented, and handled to catch potential type errors can mitigate a lot of the guesswork in JavaScript development and enhance its readability.
For more detailed description and solutions about this kind of error checkout MDN Web Docs.
Methods to Identify and Debug ‘Undefined’ Properties Errors
Indeed, ‘Undefined’ Property Errors are a common issue in JavaScript programming, and they often manifest as a TypeError stating that the program cannot read properties of undefined. This error generally appears because some part of your code is attempting to access a property or method on an object, which hasn’t been initialized yet.
There are several viable strategies for identifying and debugging these types of errors to keep your code functional and robust:
Initialize Objects Properly: One of the most frequent sources of this error is related to how objects are initialized. If an object isn’t given an initial value, accessing any of its properties will result in an ‘undefined’ error message. Always ensure to initialize objects before you attempt to access their properties or methods.
For example:
let user = {}; // Initialize an empty object. user.name = "John"; // Now you can safely assign properties to the object.
Validating User Inputs and Responsiveness of External APIs: Always validate user inputs before working with them. Also, when your code depends on data from external APIs, ensure to handle cases where the API call fails or does not return the expected format of data.
Using Conditional Statements: Check for existence of a property before attempting to use it. A common practice is to use conditional statements to check if a property exists. The typeof operator is very useful in these situations.
Example:
if (typeof user !== 'undefined' && typeof user.name !== 'undefined') { console.log(user.name); }
Utilizing Browser Console: Use the console in your browser to identify where the error is coming from. Chrome DevTools is one such tool that allows developers to see what line threw an error and even pause execution at that point to inspect variables.
In relation to the specific error you’ve mentioned – ‘TypeError: Cannot read properties of undefined (reading ‘name’)’ – this error is stating that you’re trying to access the property “name” on something that is currently “undefined”. More often than not, this indicates that an object or variable is being used before it has been initialized or assigned a value.
Here’s what Elon Musk, CEO of SpaceX and Tesla, once said about debugging: “Debugging is like being the detective in a crime movie where you are also the murderer.”
This situation truly expresses our scenario, where we have to meticulously search for our own mistake in the code and rectify it.
Therefore, prudent use of initialization, validation, and conditional statements, along with leveraging browser development tools, can be highly efficient in identifying and resolving ‘undefined’ errors due to reading properties of undefined variables or objects.
Exploring Solutions for ‘TypeError Cannot Read Properties of Undefined (Reading Name)’
Your question pertains to one of the common issues faced by JavaScript developers: ‘TypeError Cannot Read Properties of Undefined (Reading Name)’. This error mainly occurs when you try to access a property or method on an undefined object in JavaScript. For instance, if you’re trying to get the name property from an undefined object, you’ll encounter this error.
Below are some potential solutions to resolve this issue:
Checking for Null and Undefined
Before trying to access the properties or methods of an object, ensure that the object is not null or undefined. You can use the
typeof
operator to check the object’s type before attempting to access its properties.
if (typeof myObject !== 'undefined' && myObject !== null) { console.log(myObject.name); }
Using the Optional Chaining Operator
JavaScript introduced optional chaining (
?.
) in ES2020, which allows you to read the value of a property located deep within a chain of connected objects without throwing an exception.
// The code below will only log myObject.name if myObject exists. console.log(myObject?.name);
Initializing Default Object
Another solution would be to initialize a default object. If your function anticipates an object as a parameter, but doesn’t receive any, it may throw an undefined error. To prevent it, you can initialize a default object.
function myFunction(myObject = {}) { console.log(myObject.name); }
Bram Cohen, the creator of BitTorrent, once said, “The key to performance is elegance, not battalions of special cases”. The same principle applies here. It’s all about putting in the right checks and balances while writing the code to handle exceptions. Remember not to assume the existence of your object, but always check before accessing its properties.
For more details and solutions specific to different contexts, you may refer to Mozilla Developer’s Guide on Control Flow and Error Handling.
How to Prevent Future TypeError: ‘Cannot read properties of undefined’ Issues
Recognizing and preventing “
TypeError: Cannot read properties of undefined
” issues in JavaScript can significantly augment the application robustness. This error commonly occurs when you try to access a property or method of an object that has not been defined. To keep this relevant to your query – “
I cannot find the error TypeError: Cannot read properties of undefined (reading 'name')
“, here are some insights and solutions:
Deduction of Root Cause
There could be different reasons for this error, such as:
- The object you are trying to use does not exist.
- The variable you are referencing is defined after your code tries to access it.
- Your code attempts to access a property or method within an undefined part of an object chain.
Imagine the following scenario:
let user; console.log(user.name);
Here, you’re trying to log
user.name
, where user is
undefined
. JavaScript engine won’t find the property ‘name’ on something that’s undefined, resulting in “Cannot read properties of undefined” error.
Mitigations Techniques & Preventions
To mitigate and prevent occurrences of these errors in the future, consider the following approaches:
- Using Optional Chaining: New to ES2020, the optional chaining operator
?.
permits reading the value of a property located deep within a chain of connected objects without having to expressly validate that each reference in the chain is valid.1 For example,
user?.name
will either return the ‘name’ or
undefined
if ‘user’ is not defined, thereby averting the TypeError.
- Ensuring Presence Before Access: Always ensure that an object exists before trying to access its properties. If variable
user
could be undefined, a check like
if(user){console.log(user.name)}
can prevent attempts to read properties of undefined.
- Use Try-Catch: Use try-catch blocks around code that might throw a TypeError. The catch block can process the error or log it for debugging later.2
- Initializing Variables: If a variable is declared, but not defined, JavaScript assigns it the value of
undefined
. Insist on proper initialization of variables and objects during declaration.
Code Refactoring Example
Refactoring the earlier scenario considering mitigation techniques:
let user; if(user){ console.log(user.name); }
Or even better with ES2020’s optional chaining
let user; console.log(user?.name);
Both these refactored codes will not throw a TypeError.
The famous computer scientist Alan Kay once said, “Simple things should be simple, complex things should be possible.”3 With diligent error handling in our code, we keep moving closer towards this ideal in JavaScript programming.
From a developmental perspective, the error message “TypeError: Cannot read properties of undefined (reading ‘name’)” is one that often leaves developers perplexed. This issue typically arises from trying to access the properties of an object which has not been properly defined. The fundamental principle being violated here is attempting to manipulate or interact with an element that hasn’t been initialized.
When facing such an error message, your first point of action should be to identify where the undefined variable exists in your codebase. Debugging tools are essential for this process, alongside thorough examination of the code, as it allows you to precisely pinpoint potential issues.
However, the solution isn’t always as straightforward. Isolating the problem could entail examining if the object was ever defined – perhaps it was but then its value became undefined at some point throughout the execution of your program. Indeed, due diligence must be implemented in order ensure every aspect of your code functions as expected.
The ‘TypeError’ issue underscores the importance of in-depth understanding of the JavaScript language, specifically regarding objects and variables, their scope, and how they function within the context of the entire application. Few other coding languages emphasize on the usage of objects and variables as much as JavaScript does.
Here is an example of a bad piece of code leading to this error:
var student; console.log(student.name);
To fix this error, make sure you initialize objects before calling their properties:
var student = { name: 'John' }; console.log(student.name);
As Bill Gates once said, “Everyone should learn how to program a computer because it teaches you how to think”. Resolving such errors builds our problem-solving skill set, and enables us to delve deeper into the idiosyncrasies of JavaScript. (source) This error not only reinforces these sentiments, but also challenges developers to redefine their understanding of JavaScript and its inherent structure.