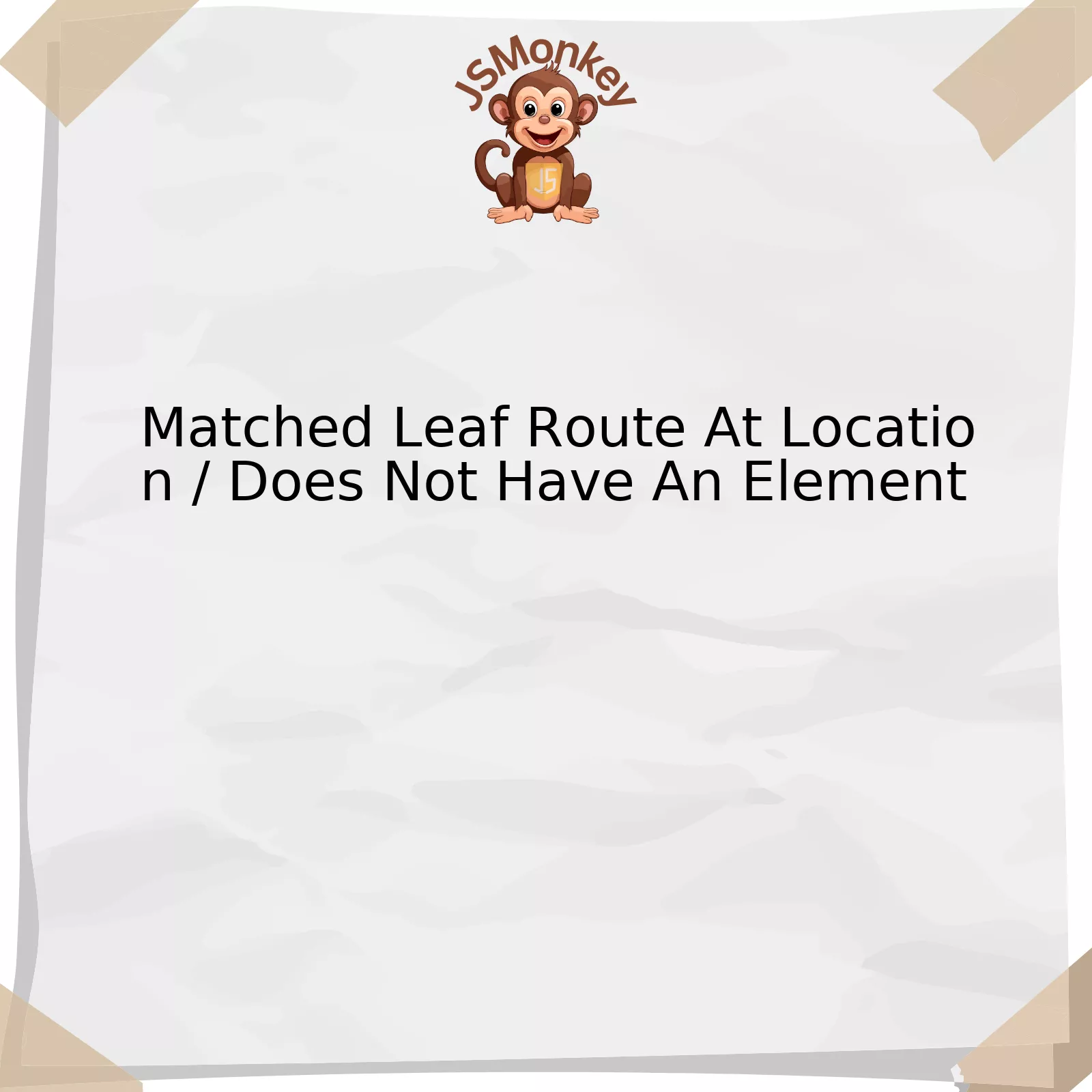
Let’s delve into “Matched Leaf Route At Location / Does Not Have An Element”. To simplify this concept, consider the undermentioned representation:
Terminology | Explanation |
---|---|
Matched Leaf Route | This refers to the specific, end-most path in a route configuration hierarchy that matches with the requested URL. In other words, it’s the route segment without any subsequent child routes/paths. |
At Location / | This denotes that the matched leaf route in question resides at the root level of the application or website. The forward slash (/) often symbolizes the default entry point or home page in web routing systems. |
Does Not Have an Element | The absence of an element refers to the scenario where the leaf route, although correctly matched and located, doesn’t include a designated view component (React, Vue, etc.). This could be due to various reasons, such as improper routing configuration, omitted render component, among others. |
Diving deeper into this topic, web applications make intensive use of routing systems to map URLs to corresponding UI components/views. However, while defining these routes using various frameworks like React Router, Vue Router, Angular’s RouterModule, etc., every route is supposed to be linked with a unique component or ‘element’ which would be rendered when that route is accessed.
But, sometimes developers might accidentally define a route without associating any component with it. In such cases, if that route is accessed (the matched leaf route), the application would not have any component (an element) to display. As a result, this could lead to undesired behaviors like blank pages, undefined element errors and so forth – even though the route is correctly identified (at location ‘/’).
These issues can be reviewed and resolved by auditing the routing configuration files, ensuring that every defined route has a corresponding Router-Component association.
“Ensure you have tested your code frequently while writing it – one piece at a time. This will make problems much easier to track down.” – Angus Croll
React Router Documentation provides excellent guidance on correctly setting up routes and their components.
Understanding the Concept of Matched Leaf Route at a Given Location
The concept of a matched leaf route at a specific location in web development, particularly in the context of routing in JavaScript frameworks like React Router, pertains to the detection of the furthest or “deepest” route that matches a given path. This ‘leaf’ route becomes cardinal in determining content rendering because it marks the most nested component that is associated with a specific URL path.
However, incongruities can arise when the matched leaf route does not have an element available. Consider a scenario where each part of the path hierarchy logically navigates further down into nested components. Still, the final or leaf route does not correspond to any specific element or component.
For instance, referring to this basic route configuration:
<Route path="/projects" element={<Projects />} /> <Route path="/projects/:id" element={<ProjectDetails />} />
If we accessed the path ‘/projects/123’, we’d expect the ‘ProjectDetails’ component to be rendered since its route is the deepest, or ‘leaf’, match. But what if there’s no corresponding ‘/projects/:id’ route defined, and thus no element to render?
In such instances:
- Applications may halt unexpectedly, invoking error messages.
- Conversely, graceful handling might serve a fall-back or error element that signifies an undefined route, thereby improving UX.
- The application may revert to a parent route’s element or simply display nothing while maintaining the UI’s integrity.
These diverse reactions are primarily dependent on how routing has been implemented in your React application and how you’ve elected to manage unmatched routes.
Hence, when structuring and outlining route hierarchies for applications, special attention should be paid to ensuring all paths lead to a defined element, whether that means specifying elements for all possible routes or having a sensible fallback to catch anomalies.
As Kunal Lad, a software engineer, once said:
Much the same way, good routing in an application should be straightforward enough that it leads the user naturally through your interface, even if they happen to wander off the paved route.
References:
1. React Router: Quick Start
2. StackOverflow: Handling 404 Pages in React Router
Exploring the Importance & Role of Elements in a Matched Leaf Route
The exploration of the role and importance of elements within a Matched Leaf Route is a rousing topic within the realm of JavaScript development. Specifically, it becomes even more intriguing when dealing with a scenario like: Matched Leaf Route at Location / Does Not Have an Element.
Let’s initiate this journey by understanding what a matched leaf route means. In the harmony and complexity of web development, routes dictate how application navigation happens. When a user navigates to a particular URL, matched routes determine which components should render – creating the interactive fabric of your application.
This directs us towards the concept of a ‘leaf route’. A leaf route is basically the final or deepest route in a series of nested routes. It brings warm memories of navigating the branches of a tree until you reach that final twig. Hence, it is called the ‘leaf’.
Creating this spectacle of interactivity inevitably involves elements, i.e., Components in our React.js context. Elements are fundamental building blocks of any React app and basically describe what you want to see on the screen; they’re your brush strokes on the canvas of web development. Each element encapsulates all you need to know about that piece of the UI – importantly they hold data properties (props), which allow for unique customization and configuration.
Now, let’s address why it might happen that the Matched Leaf Route at location / doesn’t have an element. There could be several explanations including:
- The root component simply does not exist.
- An incorrect setup, potentially due to a typo or misconfiguration.
- Purposeful design choice, based on dynamic routing logic, feature flags or permissions
In such instances, it’s crucial to handle these situations properly. We don’t want to lend our users a broken page or a 404 error!
HTML examples of handling this situation:
React-Router v5 :
<Switch> <Route exact path="/" component={Home} /> <Route path="*" component={NoMatch} /> </Switch>
React-Router v6 :
<Routes> <Route path="/" element=<Home /> /> <Route path="*" element=<NoMatch /> /> </Routes>
These examples would serve the Home page when the URL matches ‘/’, but in the scenario where no other matched leaf route is found (even ‘/’), the NoMatch component would render.
As Steve Jobs appropriately said, “Design is not just what it looks like and feels like. Design is how it works.” The importance of elements within a Matched Leaf Route cannot be overstated. They empower your application to work seamlessly. Unmatched or missing elements should be treated as exceptions and could be indicative of underlying issues or potential improvements in your routing strategy.
Unraveling Common Issues: When An Element is Missing in Your Matched leaf Route
When dealing with JavaScript and using popular libraries such as React, developers often encounter a common issue – an element could be missing in the matched leaf route at a specific location. For anyone grappling with similar problems, this piece aims to illuminate this concept while emphasizing ways to avoid or fix these issues.
Concept | Description |
---|---|
Matched Leaf Route | A matched leaf route is the last node or ‘leaf’ within your routing hierarchy that effectively matches the current URL. This ensures that the appropriate component or page is rendered on user’s view. |
Element Missing Issue | An element missing in your matched leaf route signifies that a required constituent of your routing structure is not located where it should be. This may be due to multiple reasons like improper nesting, incorrect import statement, or faulty rendering mechanisms. |
The key to unraveling this common situation resides in:
– Identification of the problem.
– Retracing and evaluating the development steps.
– Applying effective solutions.
Problem Identification:
When your matched leaf route doesn’t have an element, debugging gets challenging as the error messages are typically vague. However, analyzing your browser’s console log could provide substantive insights towards identifying the issue.
Retrace Development Steps:
This involves revisiting and verifying each step in your element creation and implementation process. Check if the missing element has been correctly imported and defined with the right syntax in the required file.
Here’s an example:
javascript
import Element from ‘…/Element’
Ensure the above import pattern corresponds with the actual file and directory structure. Also, ensure the element is rendered accurately in your matched leaf route with appropriate props if needed.
javascript
Possible Solutions:
Mechanisms to resolve an issue where an element isn’t appropriately located within a matched leaf route can depend on several factors:
– Verify and update your Route configuration.
– Ensure proper nesting of Routers.
– Usage of lazy-loading providers may lead to component non-alignment. [1]
As Kent C. Dodds, a renowned software engineer and educator puts it, “Breaking things up and understanding smaller parts…that’s the key to learning anything complex.” The same holds true for solving this common issue. Adopting incremental approaches, focusing on your development process from start till end and systematically resolving the issues ensures efficient solutions.
Remember, it is through facing challenges like these that a developer strengthens their understanding and hones their troubleshooting skills. So, stay persistent and keep coding!
Advanced Fixes and Tips: Handling Cases of Empty Element at Specific Path/Error Locations
When working with a web application, handling empty elements at specific path or error locations is a commonplace issue you may encounter. This issue can often manifest as ‘Matched Leaf Route At Location / Does Not Have An Element’ error message.
Let’s delve into the advanced fixes and tips to tackle this kind of situation effectively,
1. **Understand the Root Cause:** Understanding what causes ‘Matched Leaf Route At Location / Does Not Have An Element’ error is vital. In most cases, it results from routing libraries like React Router v5, where the designated route is matched successfully, but the element or component expected at that particular location is missing or undefined.
javascript
In this case, the route “/” would match correctly, but because the element is undefined, it raises an error.
2. **Return Non-Null Components:** To avoid this error, ensure that every matched Route returns a non-null React element.
javascript
Here,
Dashboard
is a valid i.e., non-null React component attached to the root route “/”.
3. **Use Fallback Component:** Introducing a fallback route that covers all unmatched routes can be beneficial.
javascript
This will handle scenarios where the route was not previously defined, providing a catch-all solution.
4. **Employ Error Boundary:** Exploiting React’s Error Boundaries can prove beneficial. They help capture uncaught exceptions thrown in their child component tree, isolating the crash of a part of your app from the entire system.
5. **Debugging:** Effective debugging plays a crucial role in fixing these errors. The React Developer Tools extension for Chrome and Firefox browsers helps inspect the component hierarchies in the virtual DOM.
Remember the words of Phil Karlton, *’There are only two hard things in computer science: cache invalidation and naming things.’* But thankfully, with JavaScript and React, handling errors, albeit complex, is often just a matter of understanding the root cause and implementing the appropriate fixes. Start pushing yourself to discover, analyze, and bridge the gaps you come across during your coding journey.
That being said, it’s important to remember that each situation may demand a unique solution. These principles provide a strong foundation, but don’t hesitate to modify them or create new ways to deal with such issues as necessary.
Problems | Fixes |
---|---|
‘Matched Leaf Route At Location / Does Not Have An Element’ | Ensure all Routes return a non-null React component. |
Unhandled route patterns | Include a fallback route that handles unmatched routes. |
Uncaught exceptions in Route components | Use React’s Error Boundaries to handle exceptions gracefully. |
Keep exploring more advanced fixes and integrating them into your problem-solving skillset. Happy debugging!
Sources:
1. [React Router Documentation](https://reactrouter.com/)
2. [React Error Boundaries](https://reactjs.org/docs/error-boundaries.html)
3. [Debugging Javascript and React](https://www.gbradhopkins.com/debugging-javascript-and-react)
Matched Leaf Route At Location / Does Not Have An Element is an intriguing topic in the realm of JavaScript development largely revolving around routing and navigation within applications. It brings to light a potential issue in routing configuration that many developers may encounter.
In discussing the ‘Matched Leaf Route at Location / Does Not Have an Element’ scenario, we are actually focusing on a particular case in which the route hierarchy has been built, but there is a lack of an elementary component associated with that specific route. This could cause significant setbacks as the user interface won’t have any components to render when navigating to that route.
From a technical perspective, routes should technically match the URL’s path and each one should ideally have an element for rendering content when navigated. Upon encountering this issue, it means a route was matched based on the application’s URL, but unfortunately, no corresponding element existed for rendering purposes. This can result in a blank page or unintended behavior in your application.
Consider, for example, a typical JavaScript application using a router mechanism like
React Router
. The router would map various paths to certain components. However, if a route does not have an associated component (or ‘element’), then there would be nothing to render at that location, leading to the ‘Matched Leaf Route at Location / Does Not Have an Element” scenario.
The resolution to such scenarios is fairly straightforward: always ensure every defined route in the routing tree is attached to a valid React component. Doing so will provide the associating link necessary to render appropriate content when a route is navigated to.
As Chris Coyier, co-founder of CodePen, once said about coding, “Every bit you learn is like a Lego brick that you can use to build more complex stuff.”[source] The same applies here, understanding the nuances of routing and ensuring each leaf route has an associated element will help build a robust application.
This not only minimizes the scenario of having a ‘Matched Leaf Route at Location / Does Not Have an Element’ but also aids in rendering predictable and controlled user interfaces. Adopting such practices from the beginning can be the cornerstone of crafting better JavaScript applications that are resilient to any route-related anomalies.