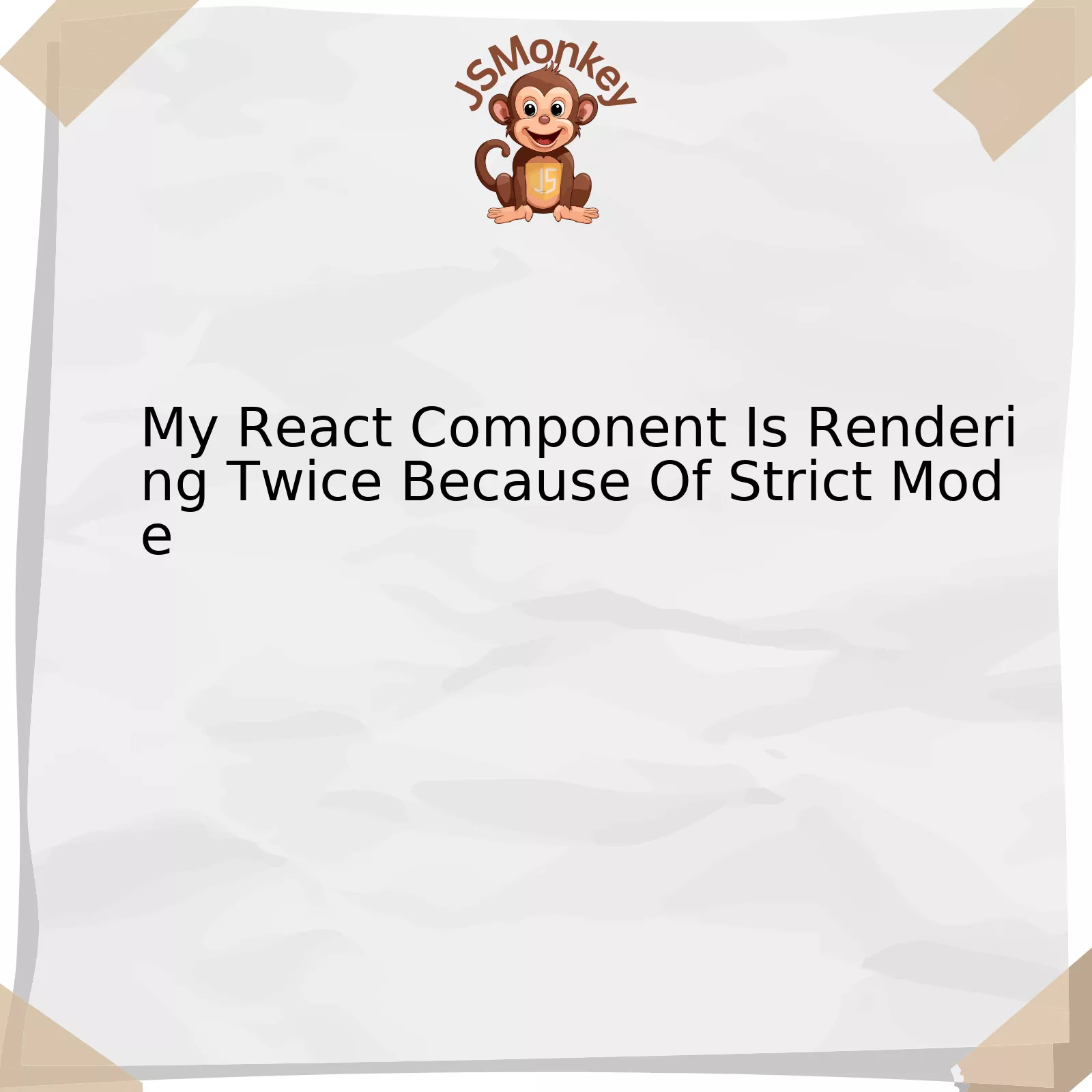
In the world of React development, there’s a lesser-known feature that developers often overlook called “Strict Mode” which sometimes leads to components rendering twice. Naturally, this raises concerns about performance and optimization.
Let’s take a look at a condensed schema of Strict Mode Effects:
Tag | Description | Behavior |
---|---|---|
<React.StrictMode> |
This is a helper component that enforces best practices in your application. No visual UI will be rendered for this. | Components within this tag may render twice |
Within the `
Rather than seeing Strict Mode’s doubling rendering as a problem, it should be viewed as an advantageous system for unfolding harmful component behaviors during development. It’s an up-front investment that makes the code base easier to maintain, troubleshoot, optimize, and understand over time.
“The task of the software development team is to engineer the illusion of simplicity” – Grady Booch.
Perceptive use of tools such as Strict Mode keeps the complexities at bay, and allows developers to orchestrate the illusion of simplicity in their systems.
[Reference Link](https://reactjs.org/docs/strict-mode.html)
Understanding React’s Strict Mode and Component Rendering
React’s Strict Mode is a beneficial tool for developers working closely with the React JavaScript library. Activating
StrictMode
in your React application provides highlighting of potential problems in an application at development time, aiding developers in writing cleaner, more efficient code.
When you implement
StrictMode
, you might notice an interesting occurrence – your components seem to be rendering twice. Although this might initially seem concerning, it’s important to note that this is an intended feature designed to help find potential issues in your code.
The primary purpose of this double rendering is to expose errors during the development phase by enforcing idempotent operations inside components. This means all operations within the component produce the exact same outcome regardless of the number of times they are invoked.
Components under Strict Mode |
Without Strict Mode – Single Render |
With Strict Mode – Double Render |
Herein lies the question: _Why does this happen only in
StrictMode
?_ Let’s dive deeper into the concept to understand.
This extraneous render effect in
StrictMode
happens exclusively in development mode. It appears intentionally to assist in detecting any unpredicted side-effects within the render phase of a component. Some examples of side effects can be data fetching, subscriptions, or manually altering the DOM. When these side effects occur arbitrarily within the render phase, it could lead to unwanted inconsistencies and errors within your application.
Take this brief snippet for instance:
function ExampleComponent() { console.log('Component rendering'); // Component logic here... return ( <div> ... </div> ); }
Activating
StrictMode
causes “Component rendering” to appear twice in the console during development mode. This helps you identify and eliminate problematic code patterns that may lead to errors in your application’s performance and user experience.
It’s also worth mentioning that despite the redundant rendering, there’s no tangible effect on the end-user or the production build of your application. Jake Archibald, a developer advocate at Google, once said: “The first load is going to be heavy for a number of reasons. You might not always be shipping what’s fast but rather what’s less likely to fail.” Therefore, a secondary render doesn’t mean double the execution time nor does it changes your UI due to the reconciliation process.
In essence, React’s
StrictMode
double-rendering feature helps improve the resilience of your application and doesn’t affect where its output is rendered in the DOM, thus making your development process more efficient and effective.
For further reading on React’s
StrictMode
, you can visit the official React Documentation.
Reasons Behind Your React Component Rendering Twice
Equipping your React component with the latest development practices, you may have wrapped it inside
React.StrictMode
. This external wrapper has been provided by React to assist you in detecting potential problem points within your application during its development stage. Now, you might be wondering – Why is your React Component rendering twice? The Simple and straightforward answer to this question lies in the concept of React’s Strict Mode.
Let’s delve a bit deeper into the subject.
React’s Strict Mode is a handy tool for developers targeting improvements in their application, specifically concerned with betterment concerning component lifespan methods and unexpected side effects—resulting in a robust, effective, and efficient application.
But, why does this lead to rendering your components twice?
When you wrap your components inside
React.StrictMode
, it deliberately invokes render lifecycle methods, constructors, and other related functions twice (only in development mode) to help you fetch unwanted patterns or habits, which are generally not recommended. Thus, what you are seeing is an intended feature, not some sort of error or anomaly.
Here’s a simple example:
import React from 'react'; class Parent extends React.Component{ constructor(props){ super(props); console.log('Parent Constructor'); } render() { return (); } } class Child extends React.Component{ constructor(props){ super(props); console.log('Child Constructor'); } render(){ return ; } } export default Parent;
In this example, when you run this code in development mode, both ‘Parent Constructor’ and ‘Child Constructor’ will be logged twice in the console.
The important thing to note here is that this double rendering and logging will only occur in development mode. Once you move your application to production mode, everything will be rendered just once as usual.
Remember these key points:
– Your components are not rendering twice because of some error or discrepancy, but due to deliberate invocation by React’s Strict Mode.
– The double rendering only happens in the development stage – and not at all in the production stage.
– Don’t panic or perceive it as an anomaly, but rather see it as a part of the improvement process.
As React Doc quotes,
“Strict mode can’t automatically detect all types of side effects, but it can help you spot them by making them a little more deterministic. This is done by intentionally double-invoking some methods.”
This enables developers like you to detect potential problems in your codebase and amend them for an impeccable user experience.
Solving the Twin Render Dilemma: Strategies Applied
A phenomenon observed when debugging in development mode of React applications is known as the “Twin Render Dilemma”. For a developer, it may feel like an enigma where every component seems to render twice, hence deeming it as a potential performance issue. This behavior, particularly noticeable when using console.log(), is often caused by the usage of
<React.StrictMode/>
.
Understandably, your curiosity might lead you to question if strict mode is causing unnecessary re-renders and consequently affecting your app’s performance. However, in reality, this double render behavior is indeed intended.
Why does using
<React.StrictMode/>
cause a component to render twice?
The
<React.StrictMode/>
wrapper was introduced with React 16.3 for providing assistance in identifying potential problems in an app during the development phase. This feature comes into useful play during the application testing phases where notions such as understanding legacy context API, detecting unexpected side effects, and more can be solidified.
React intentionally doubles the invocations of render function, state updater functions, among others, in development mode, to help developers spot bugs related to idempotency issues.
Liked Mark Twain once stated, “The secret to getting ahead is getting started.”. Hence, understanding how
<React.StrictMode/>
works is a great starting point in dealing efficiently with these twin render instances in our React applications.
Notably, these additional render cycles only occur in the development build and not in production. Thus, you won’t have to worry about the ‘twin render’ affecting your final application’s performance.
Strategy to tackle the Twin Render Dilemma
It’s important keeping your workflow efficient in spite of all this. If the extraneous logging output that results from this is causing clutter in your browser’s console and affecting the debugging process, you can momentarily comment out the
<React.StrictMode/>
wrapper during debugging.
Alternatively, a workaround to avoid ‘Twin Render Dilemma’ consistently might be putting your console log statements in a useEffect hook.
useEffect(() => { console.log('This log will only run once or upon dependencies change'); }, [])
Just remember to keep the
<React.StrictMode/>
wrapper uncommented when pushing your code for ensuring that React’s rigorous testing assertions still apply.
Through understanding the intent behind
<React.StrictMode/>
‘s operations, we are better positioned as developers to solve complex issues. It may appear as an unwelcome nuissance but is in fact, serving a remarkable purpose of reinforcing best development practices.
Further reading on React.StricMode.
Practical Implications of a Double-rendered React Component in Strict Mode
If you notice that your React components are rendering twice, it may be because of the Strict Mode wrapper. The use of
React.StrictMode
is a significant component in debugging within the React framework.
Strict Mode is essentially a tool for highlighting potential issues in an application during development stage. It does not render visible UI and does not impact the production build.
You might be utilizing Strict Mode if you see something similar to this:
html
In the context of ‘double-rendering’, Strict Mode actively triggers duplicate lifecycle methods to signal the presence of any state mutations occurring within these lifecycle phases. This is instrumental in identifying unexpected side effects which frequently lead to bugs in the code. However, the double rendering only happens in development mode.
Bartosz Szczeciński, a software engineer at GFT Poland, has once stated “The impact of double rendering on performance can generally be neglected as it helps expose potential problems in an app during development.”
While this means more computation power is required, it also means that potential bugs linked with State and Lifecycle Methods can be nipped in the bud, improving overall code quality and maintainability.
Despite many developers expressing concerns about double-rendering, it’s important to remember the behaviour is intentional and occurs only during the developmental phase. When you progress to the production build, this double-rendering feature is disabled, ensuring optimum performance for end-users.
Keep it in mind that some exceptions exist such as the
render
or
componentWillUnmount
functions, which do not execute twice even in the development build due to potential harm they could bring.
Avoid falling into the trap of assuming double rendering denotes an issue with your React Application. Strict Mode’s double render is a means to an end, providing us with invaluable safety measures, particularly when navigating the often complex states and lifecycles within React Components.
_Detailed information on Strict Mode can be found at the official React documentation page._
Delving deep into the subtle issue of your React component rendering twice due to Strict Mode, one reveals an important facet of web development and utilization of Javascript Libraries such as React. As developers dive deeper into the realm of Javascript, these issues are not infrequent and need to be analyzed with composure and precision.
Essentially, when you see that your React Component is rendering twice it means that you are operating within the confines of React’s Strict Mode React Documentation. This is not a bug or a glitch, instead, it is a deliberate mechanism designed to aid in detecting potential problems in an application during its development phase.
One should keep in mind that:
- Strict Mode doesn’t render twice for every update. It’s more like Strict Mode can double-render to a subset of your components.
- The double rendering does not occur in production builds, thus keeping your performance as optimized as possible.
- It focuses on assisting developers to perform the coding practices that are in alignment with the latest React paradigms.
Turning off Strict Mode would certainly stop the double render but it is not always the optimal solution as it may leave potential issues undetected. If you want to prevent the double rendering, consider refraining from usage of deprecated lifecycle methods and also isolate any side-effects or subscriptions in your code, especially within
componentDidMount
,
componentDidUpdate
and
componentWillReceiveProps
.
As quoted by Jeff Atwood, “We should forget about small efficiencies, say about 97% of the time: premature optimization is the root of all evil.” So, while dealing with issues like these, it’s prudent to bear in mind that the double rendering is only a development stage feature and won’t come into play in production builds. Let us don the mantle of progress and optimally use tools such as Strict Mode to create efficient, robust and futuristic web applications.