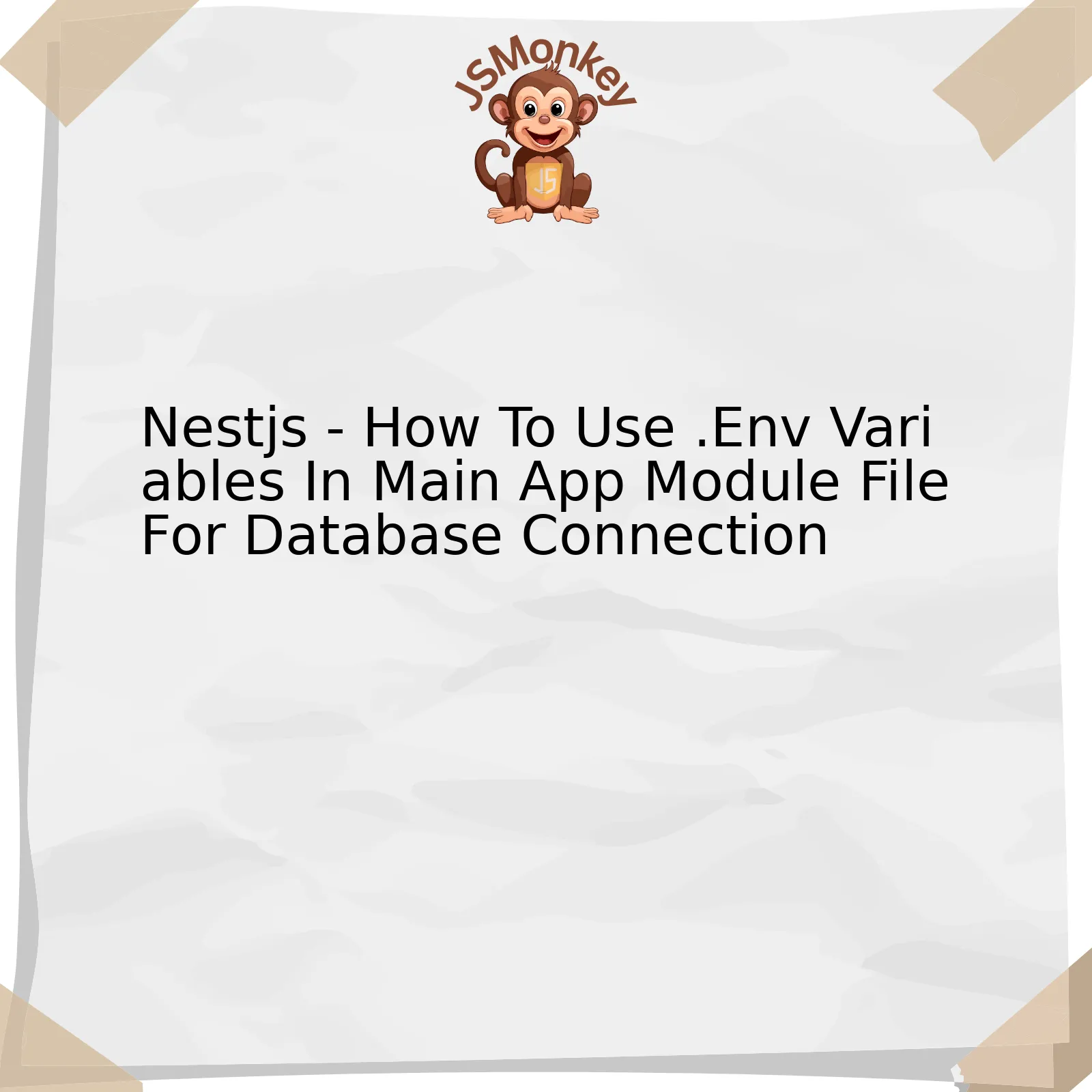
To begin, Nestjs is a progressive Node.js framework for building reliable and scalable server-side applications. It supports the use of .env variables, which helps in segregating configuration details from app logic by keeping them in a separate file. To utilize .env variables in the main App module file for database connection, we must follow certain steps, illustrated through a table:
Step | Action |
---|---|
Install dotenv |
npm install dotenv |
Create .env file | Create a .env file in the root directory and declare your variables |
Import and configure dotenv | Implementing
require('dotenv').config() at the top of the main.ts file |
Use process.env | Variables can be accessed using
process.env.YOUR_VARIABLE |
Each step within this flowchart contributes to the process of instantiating .env variables within the primary application module file for database connectivity.
The initial stage involves installing the ‘dotenv’ package that loads environment variables from a .env file into `process.env`.
Subsequently, the creation of a .env file at the root level of your project where all the necessary environment variables will be stored takes place. An example of such a variable could be `DATABASE_URL=mongodb://localhost:27017/nestJsDB`.
Next, dotenv is incorporated into the main file (‘main.ts’) of the application. This is executed to read the variables stored in .env file and store them into `process.env`.
Finally, within your App module, you can utilize variables from .env file using process.env.
This strategic approach paves the way for managing settings specific to different environments such as development, test, and production seamlessly without distorting the codebase, thereby aiding robust database connectivity.
As Martin Fowler, a renowned software developer once said, “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” Following clear procedures like these not only simplifies the task at hand but also makes your code easier to comprehend by others.
Leveraging .Env Variables in NestJS for Database Connectivity
When building applications with NestJS, environment variables can provide a highly efficient method for managing different configurations. This technique is adaptable and equally effective in test, development, and production environments. One common use case for this is database connectivity. You want to centralize the database connection settings and manage them effectively.
Connectivity database settings defined as environment variables aids security and ease of management. It assists in keeping sensitive information such as Database name, username, password, and host off the codebase.
You may leverage the configuration module that NestJS offers. It helps abstract setting up environment variables, provides sensible defaults, and allows validation.
Step 1: Installing Configuration Dependencies
First, you need to install the necessary packages `@nestjs/config`(for creating config files) and `dotenv`.
npm i @nestjs/config dotenv
The package `@nestjs/config` would help us leverage environment variables within our application, while `dotenv` would parse our .env file’s variables.
Step 2: Creating and Configuring .env File
Create a `.env` file in the root directory of your NestJS app. Inside this file, define your database connection properties as shown below:
DB_HOST=localhost DB_PORT=5432 DB_USERNAME=user DB_PASSWORD=password DB_DATABASE=mydb
These represent your local database details you want the application to connect to.
Step 3: Accessing .ENV Variables for Database Connection
In order to access .env variables and use them to establish a database connection, you have to import the ConfigModule in the `main.ts` file. Then use it inside AppModule.
As stated by David Walsh, a recognized figure in programming, “Configuration files give developers an extra level of flexibility. They allow us to maintain important variables in secure locations while promoting code reusability.” Hence inside the AppModule, you can setup database connection like this:
javascript
@Module({
imports: [
ConfigModule.forRoot(),
TypeOrmModule.forRootAsync({
useFactory: (configService: ConfigService) => ({
type: ‘postgres’,
host: configService.get(‘DB_HOST’),
port: configService.get(‘DB_PORT’),
username: configService.get(‘DB_USERNAME’),
password: configService.get(‘DB_PASSWORD’),
database: configService.get(‘DB_DATABASE’),
entities: [__dirname + ‘/**/*.entity{.ts,.js}’],
synchronize: true,
}),
inject: [ConfigService],
}),
],
})
export class AppModule {}
With the given setup, your application can then access these credentials and establish a database connection. This provides a more secure and manageable way to handle database connectivity.
Harnessing Environment Variables for Main App Module Configuration
In JavaScript application development, specifically within the scope of NestJS based applications, environment variables can play a crucial role in configuring and managing settings related to the main app module – particularly with regards to establishing database connections. Infusing these environment variables into your NestJs app improves the level of abstraction you have over your codebase. It ensures that you can manage key connection elements without making substantial changes to your code itself.
Managing the `.env` file allows you to alter your database connection details dynamically and discreetly, keeping such sensitive information out of your primary codebase. Within the core context of using environment variables with NestJS, the `dotenv` library is popularly implemented.
An optimal approach entails saving the database configuration details in an `.env` file which will typically appear as follows:
ini
DATABASE_HOST=localhost
DATABASE_USER=myuser
DATABASE_PASSWORD=mypassword
To incorporate the variables set in this file into your NestJS application, you make use of the following code snippet in your main.ts (main app module) file:
javascript
require(‘dotenv’).config();
You will then be able to access the specified environment variables using Node.js’ `process.env` object like so:
javascript
{
//…
TypeOrmModule.forRoot({
type: ‘mysql’,
host: process.env.DATABASE_HOST,
username: process.env.DATABASE_USER,
password: process.env.DATABASE_PASSWORD,
//…
}),
//…
}
This enables you to establish database connectivity by referencing your environmental variables rather than hard-coded values, conferring three major benefits:
– **Privacy**: Sensitive data such as login credentials are kept away from the primary codebase.
– **Efficiency**: Changes in your database settings do not necessitate changing your actual written code.
– **Portability**: Your application becomes flexible to move across various environments – be it testing, staging, or production – with minimal alterations to the underlying code, improving the efficiency of your deployment pipeline.
It’s essential to bear in mind however that the `.env` file should be added to the `.gitignore` file so as to maintain the privacy of sensitive details when deploying or sharing your repository.
As said by Joel Spolsky, a well-known software engineer and writer, “Good database architecture is all about making the system work efficiently while preserving data integrity.” andjudicious use of environment variables in your main app module would certainly lend credence to this statement by ensuring both efficiency and security.
Understanding the Use of .Env Variables in NestJS Database Connection
The utilization of .env variables in NestJS when establishing a database connection is integral to preserving the security and operability of your application. This approach aids in concealing sensitive information, such as database credentials, consequently reducing the vulnerability of your software to critical threats such as security breaches. Here, we will focus specifically on utilizing these variables in the primary app module file for a database connection.
To begin with, one must acknowledge that it is best practice to keep sensitive data like usernames, passwords or API keys out of your code. Environment variables let you separate this kind of data from your source code. Confidentiality is maintained even when the code enters a public domain like GitHub without requiring extensive modifications.
Let us understand the process of utilizing .env files in NestJS. Two vital elements are involved:
1. Encapsulation of environment-specific values in .env files:
One creates a .env document at the root location of the project, which embodies all relevant confidential details. For instance, a traditional set up for a PostgreSQL database could be:
DATABASE_HOST=localhost DATABASE_USERNAME=my_username DATABASE_PASSWORD=my_password
2. Utilization of the ConfigModule:
NestJS has an intricately designed configuration handling system, named Config Module. This module encourages the initiation of .env variables and assures their accessibility throughout the application. In order to install the module, use:
$ npm i @nestjs/config
Once the ConfigModule has been installed, you may incorporate it into your main app module. With support from the module, your application can now load the .env variables.
import { Module } from '@nestjs/common'; import { ConfigModule } from '@nestjs/config'; @Module({ imports: [ ConfigModule.forRoot(), ], }) export class AppModule {}
Using the .env variables in connecting your database involves integrating TypeORM module into your application.
import { Module } from '@nestjs/common'; import { ConfigModule, ConfigService } from '@nestjs/config'; import { TypeOrmModule } from '@nestjs/typeorm'; @Module({ imports: [ ConfigModule.forRoot(), TypeOrmModule.forRootAsync({ imports: [ConfigModule], inject: [ConfigService], useFactory: async (configService: ConfigService) => ({ type: 'postgres', host: configService.get('DATABASE_HOST'), username: configService.get('DATABASE_USERNAME'), password: configService.get('DATABASE_PASSWORD'), // remaining configuration }), }), ], }) export class AppModule {}
In this schema, the ‘host’, ‘username’, and ‘password’ properties are extracted directly from the .env file for the database connection. The `forRootAsync` method creates a dynamic module, providing us with the ability to inject dependencies into the options factory. We’re importing `ConfigModule`, which makes the `ConfigService` provider available within our `TypeOrmModule`.
This setup ensures that the sensitive information about your database connection is effectively hidden behind the .env variables – aiding both the security of your application codebase and its maintainability.
As Robert C. Martin once said, “The secret of good design is managing dependencies. The secret of good management is maintaining flexibility.” Replacing hardcoded database credentials with .env variables not only helps in containing possible threats but also enhances the adaptability of your software design across different environments.
Please keep in mind that the values held within the .env file should always remain confidential and must never be pushed into public code repositories. Always include ‘.env’ in your ‘.gitignore’ file when versioning using Git.
Practical Guide: Using .Env Files For Directing Database Connections In Your Nestjs App
.env files can serve as a powerful tool when managing database connections in your NestJS application. Through the use of these files, developers can create varying environments for development, testing, and production — all while keeping sensitive connection details out of version control. This practice is often paramount to maintaining robust database security.
In order to utilize .env variables in the main app module file of your NestJS app for handling database connections, these simple steps can be followed:
Installation:
The first step involves installing node’s package dotenv. This installs the .env functionality required in your Node.js applications. To install, you can run the following command in your terminal:
npm install dotenv
Create a .env file:
After installing dotenv, create a .env file in your root directory. In this file, you input your database connection string and any other environment specific variable like so:
DATABASE_URL=your-database-url
Note: It’s crucial to add the .env filename into your .gitignore file to prevent it from being pushed to your repository.
Accessing the .env Variables:
require('dotenv').config();
This line allows you to access the variables stored in your .env file:
process.env.DATABASE_URL
You can then use the code above in your app.module.ts file during the configuration of your database connection like so:
@Module({ ..., imports: [ TypeOrmModule.forRoot({ type: 'postgres', url: process.env.DATABASE_URL, entities: [Entity1, Entity2], synchronize: true, }), ], }) export class AppModule {}
Here, the value for url comes from the .env file you created. This keeps Nestjs flexible for different environment setup, and makes it easier to manage sensitive data.
Having separate .env files for your unique environments can provide greater control over your application configurations. Each environment (development, testing, production) can have its own set of unique variables which are loaded when the app runs in that given context.
Final thoughts:
As Jeff Atwood, a renowned programmer and author, once said, “Code is like humor. When you have to explain it, it’s bad.” Consequently, maintaining clean and expressive code through practices such as using .env files for directing database connections not only leads to better application security but also enhances readability.
Remember to refer to the [official NestJS documentation](https://docs.nestjs.com/techniques/configuration), along with the [dotenv package documentation](https://www.npmjs.com/package/dotenv), for more insights into managing configuration files for your application.
Harnessing the power of `.env` variables in a NestJS app module file for database connection is a quintessential part of enhancing security, maintainability and scalability in server-side applications. By using environment specific configurations, we can impeccably manage sensitive information like database credentials without exposing them directly within our codebase.
To integrate `.env` values in database connection, one usually begin by installing required packages – `dotenv` is a commonly used package in this case. Next, implementing the configuration, values are taken from the `.env` file, where specific environment variables are defined. With these environmental variables set up properly, you set up your main App Module file accordingly.
import {TypeOrmModule} from '@nestjs/typeorm'; import {ConfigModule, ConfigService} from '@nestjs/config'; @Module({ imports: [ TypeOrmModule.forRootAsync({ imports: [ConfigModule], inject: [ConfigService], useFactory: (configService: ConfigService) => ({ type: 'postgres', host: configService.get('DB_HOST'), // Defined in .env ... }), }), ], }) export class AppModule {}
The `host`, `port`, `username` are fetched directly from the `.env` file rather than being hardcoded. This ensures that the app remains flexible enough to handle different execution environments.
Subtly, the technique highlights a fundamental design principle, as stated by Martin Fowler, “Any fool can write code that a computer can understand. Good programmers write code that humans can understand,” thus, we write clean, readable, and modular codes that not only computers but also fellow developers can understand effortlessly. By encapsulating secret credentials off our codes, `.env` variables help us maintain an easy-to-understand, well-structured, and secure application codebase.
It’s compelling how environment variables bridge the gap between discrete staging environments and contribute to an overall maintainable and efficient application development. NestJS shines in its capability of providing such a seamless experience of managing environment-based configurations with minimal effort, facilitating smooth cooperation between developers, systems, and servers.
References:
– [npm dotenv package](https://www.npmjs.com/package/dotenv)
– [Nestjs TypeORM Module](https://docs.nestjs.com/techniques/database)
– [Nestjs Config Module](https://docs.nestjs.com/techniques/configuration)