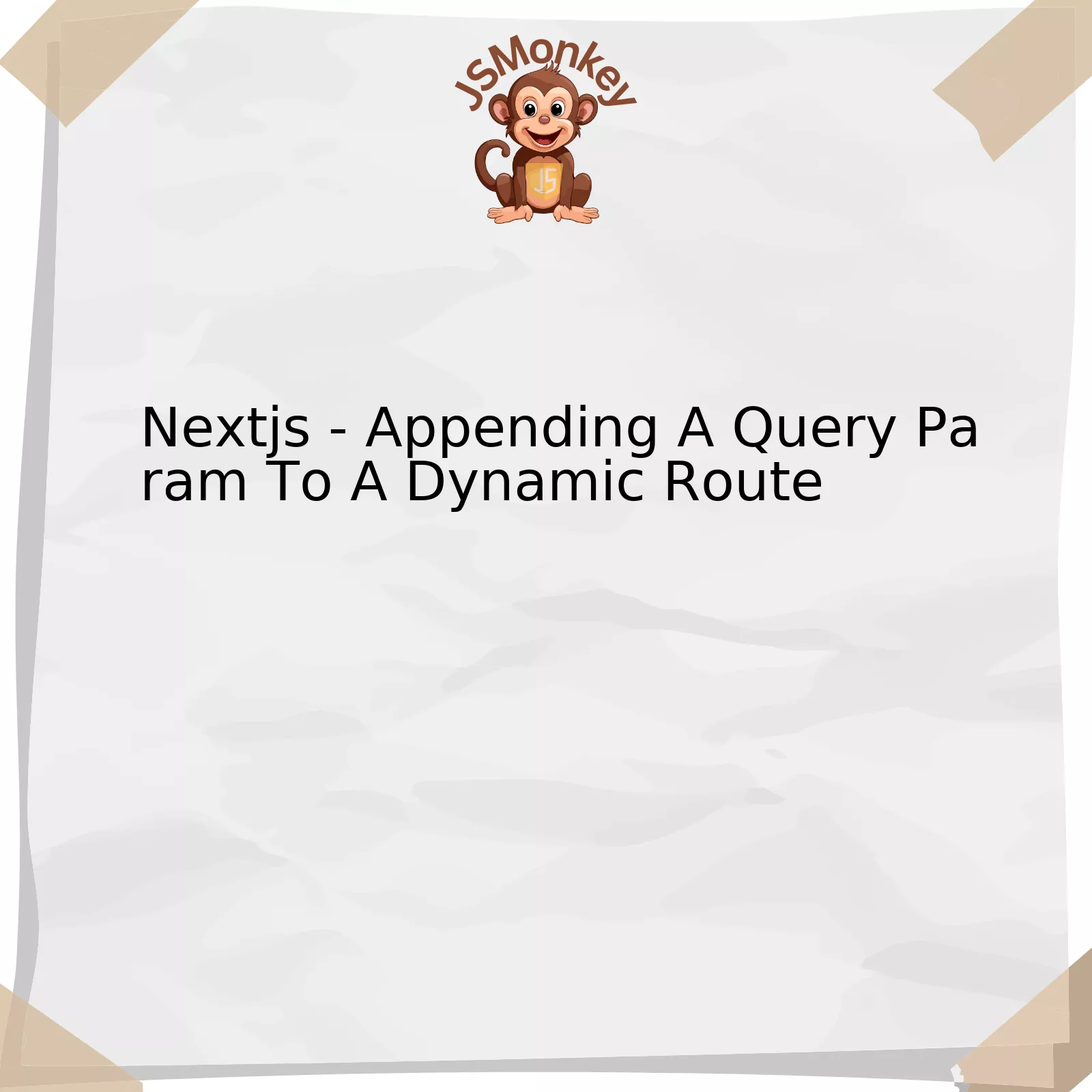
When working with dynamic routes and query parameters in Next.js, it combines simplicity and power to generate complex web applications. One of the features it offers is the ability to append a query parameter to a dynamic route.
Consider the following table which explains the key concepts related to this topic:
Term | Description |
Next.js | This is a React framework that enables server-side rendering and generating static websites for React-based web applications. It’s highly suitable for scalable and fast web applications. |
Dynamic Route | In Next.js, dynamic routes work by pre-fixing a page file name with bracket syntax, essentially “[param]”. These files will be turned into pages with the param value inserted into the generated URL. |
Query Parameter | A section defined or specified in the Uniform Resource Locator (URL) consisting of its values pairs separated by ‘&’. It’s also referred to as URL Parameters. |
Taking advantage of Next.js dynamic routing happens by creating a page with a file path `pages/post/[id].js`, here `[id]` is a dynamic segment and will match any route like `/post/1`, `/post/abc` and so on. Hence, our URL becomes dynamic.
To append a query parameter to a dynamic route, you can use the Next.js `router.push()` method. In a functional component, you may have an example like this:
const router = useRouter(); const handleOnClick = () => { router.push({ pathname: '/post/[id]', query: { id: '123', extra: 'yes' }, }); }
In the above code, `useRouter()` is a custom hook that gives you access to the router object. `router.push()` is used to append the query parameter. Here we have appended “extra=yes” to the URL. On clicking an element (Button/Link), it will navigate to the path “/post/123?extra=yes”.
Appending a query parameter to a dynamic route in Next.js is quite intuitive. It takes advantage of JavaScript’s flexibility and Next.js’s designed ease of usage to deliver on powerful functionality.
Peter Wilson, an acclaimed technologist once said – “The subtlety of web development really shows when working with dynamic URLs and routing. Understanding them can open up new experimental playgrounds.” Remembering this can be handy when tackling complex challenges related to routes and parameters while developing applications using Next.js.
Leveraging Dynamic Routes in Next.js
Dynamic routing is a quintessential feature in Next.js, which brings immense power and flexibility to routing for React applications. Speaking specifically about appending a query parameter to a dynamic route, several nuances of Next.js become apparent.
Diving into Dynamic Routes
Next.js uses a filesystem based routing mechanism, meaning the pages inside the
pages/
directory structure essentially determine the routes of a Next.js application.
For instance, a page named
pages/posts/[id].js
, conveys an indication that it represents a dynamic route
/posts/:id
.
However, when you have a requirement to append a query parameter to a dynamic route, you need to take additional measures which we will discuss next.
Appending Query Parameters
In order to append a query parameter to a dynamic route, you would typically use the
next/link
or
next/router
packages within your Next.js application.
Utilizing the Link component from
next/link
, a new href can be formed which includes both the dynamic path and a query string paramater concatenated:
<Link href={`/posts/${postId}?comment=open`} > <a>Title</a> </Link>
The term ‘open’ is an example of a query string parameter being appended to a dynamic route.
Alternatively, the useRouter hook provided by
next/router
can also cater to this scenario:
import { useRouter } from 'next/router' ... const router = useRouter() router.push(`/post/${id}?comment=${commentId}`)
This approach offers direct programmatic navigation capability with your newly-formulated query parameter added to the dynamic route.
Retrieving the Query Parameter
The same
useRouter
hook can be employed to access the query parameter within the component:
const router = useRouter() console.log(router.query.comment)
As you’ll find, this will log the comment’s value from the URL.
Let me cite a quote by Brendan Eich, the creator of JavaScript; he once said: “Always bet on JavaScript”. Here, it resonates rightly so, with Next.js – being built on JavaScript – offering an extensive routing mechanism allowing developers to cater sophisticated app structures in elegant and maintainable ways.
References:
For more detailed analysis you can check Next.js Official Documentation: Dynamic Routes.
Implementing Query Parameters in your Next.js Application
Appending a query parameter to a dynamic route in your Next.js application is an integral aspect of efficient web development. By utilizing query parameters, developers can enhance their applications by offering flexible navigation patterns, improved state management, and more intuitive user interfaces.
A typical URL including a dynamic route and a query parameter could look like this: `https://yourwebsite.com/user/[id]?display=full`. Here, the `[id]` portion indicates a dynamic segment of the path, mutable depending on usage context, while `?display=full` denotes a query parameter.
How to Implement
In Next.js, there are various methods for implementing query params into dynamic routes. Primarily, we’ll focus on employing Next’s built-in routing library or using higher levels libraries such as `next/router`.
1. Method with Link from “next/link”
One straightforward method involves using the `` component from the `next/link` package:
import Link from 'next/link'; .... User Profile
The `href` prop points to the page in your pages directory, which receives the value when rendering. The `as` prop is what the browser URL displays.
2. Pushing to Router Directly
Another approach is pushing a new URL to the router directly. This is commonly achieved with the `useRouter` hook:
import { useRouter } from 'next/router'; .... const router = useRouter(); .... router.push(`/user/${userId}?display=full`);
This is particularly useful when you want to programmatically navigate to a new page based on a certain event such as button click.
3. Using Router from ‘next/router’ for Server-Side rendering
import { useRouter } from 'next/router' .... const Component = () => { const router = useRouter() .... return ( <> {router.query.display? `You selected ${router.query.display}` : 'Loading...'} ) }
The `useRouter` hook can be used to access the query parameters.
Alan Turing, a pioneer in computer science, asserted, “We can only see a short distance ahead, but we can see plenty there that needs to be done.” In light of this, it’s important to take proactive steps in making your next.js applications more polished and efficient by properly implementing query parameters on dynamic routes.
To dive deeper into Next.js routing, you can visit the [Official Next.js Routing documentation].
Tips for Appending Query Params to Dynamic Routing in Next.js
Dynamic routing in Next.js forms the backbone of almost all single-page applications (SPAs) that need to manage different views. The process is fairly straightforward, but appending query params can add an extra layer of complexity. That said, with the correct approach and techniques, this won’t be a problem.
Let’s dive into some potent tips for appending query parameters to dynamic routes in Next.js:
The Usage of Router.Push : This method allows you to programmatically change the URL on your Next.js application, including adding or modifying query parameters while navigating to a dynamic route. Here’s an example:
router.push({ pathname: '/post/[pid]', query: { pid: '12' }, });
In the above code snippet, we’re changing the URL to /post/12.
Merge Object Properties with Spread Operator: When there are multiple query parameters to add or update, you should merge them into one object using the JavaScript spread operator. For instance:
const newQueryParams = { ...oldQueryParams, newParam: 'newValue' };
This code assigns new queries along with old ones, enabling the simple manipulation of multiple parameters.
Encode Query Parameters: Always remember to encode your query parameters when dealing with string values that include special characters. You can use JavaScript’s built-in global function –
encodeURIComponent()
. An example usage:
const encodedParam = encodeURIComponent('special@character');
Alternate between Static and Dynamic Links: When it comes to practical usage, developers may have to switch between static links and dynamic links often, depending on the requirements. It requires planning—dynamic routing shouldn’t be instantiated at every turn, nor should it be ignored entirely.
Adhering to these four steps could help facilitate smooth navigation through your application by efficiently managing the appending of query parameters to dynamic routing in Next.js. Refer Next.js Official Documentation for a more comprehensive understanding.
Finally, I would like to share an insightful quote from Martin Fowler: “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” Thus, no matter how complex the task is, ensuring your code’s simplicity and functionality should be your top priority.
Advanced Concepts: Combining Query Params with Dynamic Routes in Next.js
Next.js, an open-source React front-end development web framework developed by Vercel, provides robust capabilities for combining query parameters with dynamic routes when constructing advanced web applications. Combining query parameters and dynamic routes in Next.js entails effective navigation handling and URL manipulation based on the needs of your application.
When it comes to appending a query parameter to a dynamic route in Next.js, it requires understanding both the concept of dynamic routing as well as query parameters:
Dynamic Routing:
Dynamic routes serve to create paths that are not known or fixed in advance but rather depend on data obtained at runtime. Next.js simplifies the creation of dynamic routes through file-system-based routing where pages are automatically routed based on their filename and directory structure in the ‘pages’ directory.
For instance, if you have a page named
pages/posts/[id].js
, Next.js recognizes this as a dynamic route, with `[id]` being a placeholder for the actual data which is usually retrieved from a database or API.
Query Parameters:
Query parameters provide a method for passing variables in a URL, typically used to manipulate the view on the client-side without changing server-side data. They begin after a question mark (‘?’) in a URL and are often key-value pairs separated by ‘&’.
Appending a query parameter to a dynamic route in Next.js allows you to deliver additional information while navigating through the application without altering your existing routing structure.
Here’s how you might implement it:
Consider a blog post Detail page where you want to append a “comment” mode query param within the URL:
The dynamic route:
/posts/[id]
With appended query param:
/posts/[id]?mode=comments
Here,
[id]
represents the dynamic part of the URL (typically, the unique identifier for each post), and
mode=comments
is the query parameter, indicating that the comments view should be enabled in the Detail Page.
To navigate to this URL within your application, you might use Next.js’s
Link
component like so:
`<Link href="/posts/${id}?mode=comments">View Comments</Link>`
In this case, the `href` attribute includes both the dynamic path and the query parameters.
As Bill Gates once said, “The advance of technology is based on making it fit in so that you don’t really even notice it, so it’s part of everyday life”. This applies here as well. With Next.js’s dynamic routes and query parameters, you can achieve a complex navigation system while providing a seamless user experience, as Mr. Gates suggests.
Topic | Overview |
---|---|
NextJs – Appending a Query param to a Dynamic Route | While advancing with your application’s functionality, there may come a time when you need to append query parameters to dynamic routes in Next.js. It’s essential to remember that doing this task should take into consideration both the user experience and the SEO.
The fundamental method revolves around using the router object that comes built-in with Next.js. The query parameters can be added through the push or replace methods of the router . Using these tools, Next.js delivers smooth navigation while appending a query parameter to a dynamic route. Success with appending query parameters ultimately hinges on understanding how next.js handles routing via its file-system based router and utilizing it effectively. This might seem overwhelming at first but with practice, any developer mastering this technique can significantly enhance their web applications’ capabilities and efficiency. HTML provides us with an excellent platform to deliver more refined user experiences on our apps. It helps ensure our content is accessible across various devices and audiences. By incorporating dynamic routes with query parameters, it’s even easier to create tailored experiences for various users. “Programming isn’t about what you know; it’s about what you can figure out.” – Chris Pine When it comes to ‘Appending a Query Param to a Dynamic Route’ in NextJs, it’s important to have a solid understanding of how the framework’s routers work. That being said, one should make sure to remain continually updated, optimizing for SEO along the way will undoubtedly lead to improved website performance and user engagement. |