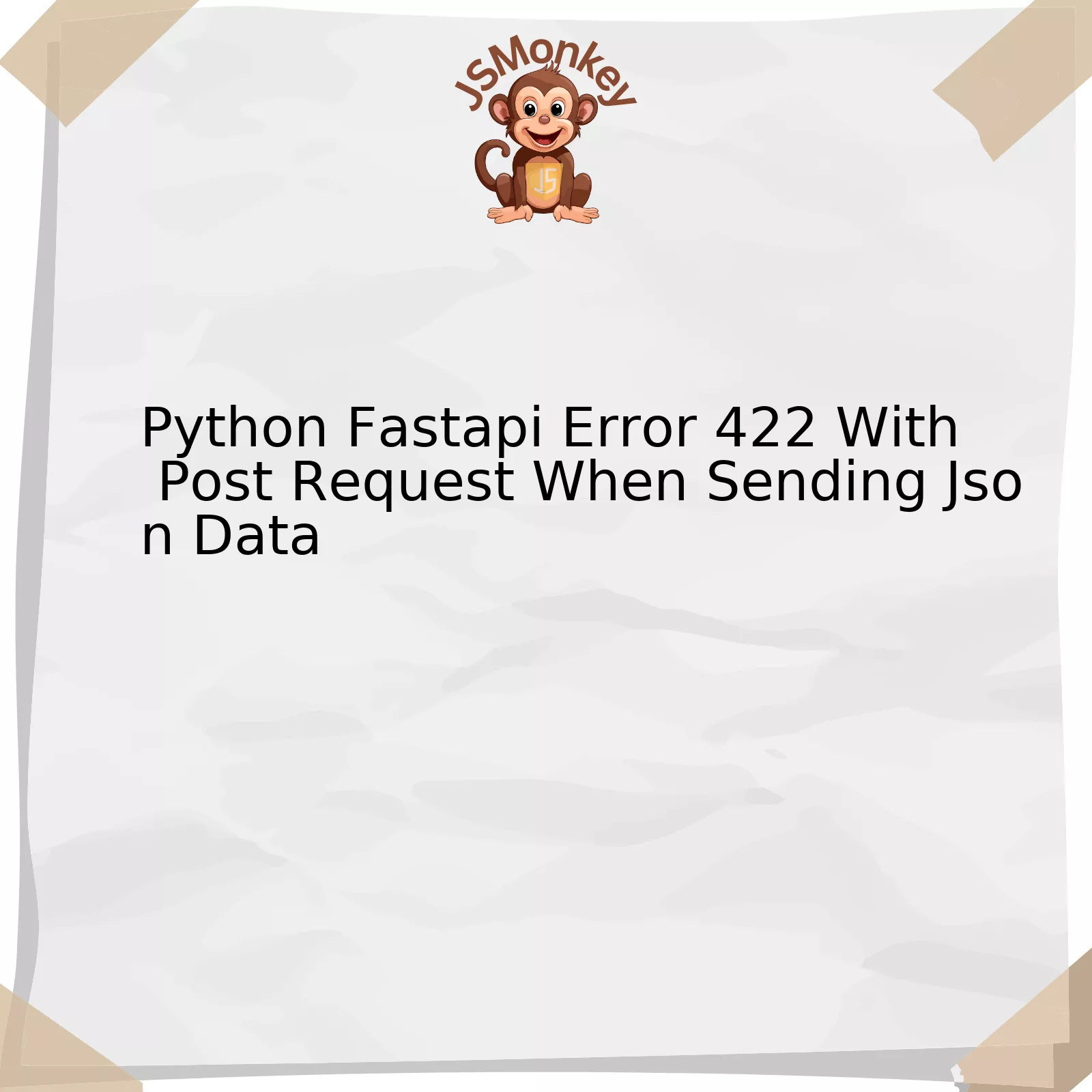
The
Python FastAPI Error 422
often occurs when sending JSON data via a POST request. It typically signifies that there’s an error with validation of input data. The HTTP code 422 stands for ‘Unprocessable Entity’.
To better understand this, let’s reference the table below:
Error | Description | Solution |
---|---|---|
Python FastAPI Error 422 | An error occurs when FastAPI tries to parse and validate the body parameters but fails. This is commonly due to incorrect or unvalidated input. | Implementing schema validators and checking your Pydantic models can help pinpoint where invalid data is coming from. |
The table highlights that the 422 error in the context of FastAPI occurs due to problems with parsing and validating data. In other words, it’s essentially a syntax error that Python cannot process.
FastAPI uses Pydantic for data validation. Thus, if you encounter a 422 error, take a careful look at your Pydantic models. Check whether all types are correctly defined and whether all fields have default values, if necessary.
One important contributing factor could be the nesting of Pydantic models. The nesting must align with the provided JSON data structure, otherwise, FastAPI might throw a 422 error. For instance, if a nested field in a model expects another dictionary, but you provide a simple value or vice versa, FastAPI wouldn’t be capable of validating this data which could trigger the 422 error.
As Jeff Atwood, co-founder of Stack Overflow once said, “There are few things more frustrating than dealing with a code issue you don’t fully understand”. Therefore, to prevent the occurrence of such issues, it is essential to comprehend the nuances of our tools. In the case of FastAPI and its use of Pydantic models, understanding the system’s expectations regarding data types can prevent errors such as the 422.
FastAPI Documentation offers an extensive guide to handling these sort of errors and maintaining the structure of our models. They suggest strategies like using `List` or `Dict` annotations in your Pydantic models according to the Json data you are expecting. This could ensure correct parsing and validation, leading to smoother operations with fewer errors.
In addition, for production environments, instructing FastAPI to include request body in log records can greatly aid in error investigations. This approach enables one to examine the exact transmitted data that resulted in the error.
Unpicking the Python FastAPI Error 422 Issue: A Deeper Look
FastAPI is a modern, fast (high-performance), web framework for building APIs with Python based on standard Python type hints. A frequently reported issue by developers using FastAPI for implementing API services is the Error 422 or Unprocessable Entity error specifically while making POST requests when sending JSON data.
Error 422: Unprocessable Entity
is most commonly thrown when you’re submitting a POST request where the request body is expecting a certain data format, usually JSON, but the server-side script couldn’t correctly parse it due to some unknown reasons.
Unraveling this, we dissect the major contributors to cause this issue:
Potential Cause | Reason |
---|---|
Mismatched Data Types | FastAPI expects stringent datatype matching for received values and will reject any mismatches. |
Absence of Expected Value | If the endpoint defined in your FastAPI server expects a particular value in the incoming JSON and it’s not present, it will flag an error. |
Nested JSON Structures | Simply defining Base Models may not be enough when dealing with complex JSON structures, which could potentially trigger FastAPI Error 422. |
For instance, consider we’ve a user signup route that expects ‘username’ and ‘password’ as inputs:
from fastapi import FastAPI from pydantic import BaseModel app = FastAPI() class User(BaseModel): username: str password: str @app.post("/signup") async def create_user(user: User): return {"username": user.username}
In this case, sending a POST request without either ‘username’ or ‘password’ will result in
Error 422
.
Also, it’s important to note how nested JSONs are handled, as they often lead to Error 422. Consider the below snippet where a category can contain multiple subcategories.
from typing import List from fastapi import FastAPI from pydantic import BaseModel app = FastAPI() class Category(BaseModel): name: str subcategories: List[str] = [] @app.post("/category") async def create_category(category: Category): return {"name": category.name, "subcategories": category.subcategories}
Strict compliance with data structures and types aid in circumventing such issues in programming.
As Ed Catmull, President at Pixar Animation Studios, puts in his book ‘Creativity, Inc.’, “In many cases, when a good new idea is developed, it’s not because the team had a vision but because it has been stumbling towards one through trial and error. Exploring various possibilities and allowing what you discover in your explorations to shape your product’s development and direction.” Sometimes errors like FastAPI Error 422 play key roles in shaping our understanding, thus influencing the project’s growth.
Code Evaluation: Common Causes of Error 422 in FastAPI with JSON POST Requests
Error 422 or “Unprocessable Entity” in FastAPI with JSON POST requests usually signifies that the server understands the content type and the syntax of the request payload but was unable to process the contained instructions. Primarily, it’s used for semantic errors during the processing of a client’s request.
When dealing with Python FastAPI, Error 422 with POST request when sending JSON data can be traced back to several potential causes which include:
– Validation Error: FastAPI uses Pydantic models for request bodies. Error 422 may occur if, for instance, your sent JSON data doesn’t match the expected model schema.
For example:
from fastapi import FastAPI from pydantic import BaseModel app = FastAPI() class Item(BaseModel): name: str price: float @app.post("/items/") async def create_item(item: Item): return item
Here an incoming JSON POST payload must contain ‘name’ (expected to be a string) and ‘price’ (expected to be a float). When the posted JSON isn’t formatted correctly according to this schema, you’ll run into a 422 error. Thus, validating input parameters before sending or ensuring proper exception handlers are in place could solve the issue.
– Data Type Mismatch: This occurs when expected and actual data types do not match. For instance, the Pydantic model may expect a string value for a certain field, and the received JSON may provide an integer. An example:
{"name":123,"price":50.0}
This would cause a 422 response as the field ‘name’ should be a string, not an Int.
– Incorrect JSON Structure:: If the structure of your payload does not correspond to what is defined on your endpoint, it will result in an error.
Using schema validation tools to define, validate and parse POST request bodies would be an effective way to deal with complex JSON structures and avoid this problem.
Tim Bray, a well-regarded computer scientist once said: “The worst data loss bug is assuming that data you’ve received has already been validated.” This quote clearly emphasizes the importance of validating incoming requests in web applications such as FastAPI.
Remember, by understanding these common causes for Error 422, you could prevent or resolve similar errors in your application. Always test thoroughly and ensure that incoming JSON data matches the expected structure and data types for your FastAPI routes.
Exploring Python’s FastAPI[1](http://https://fastapi.tiangolo.com/) for Effective Web Applications is a preferred link for learning more on this topic.
Note: Keep your Pydantic models matched up correctly with whatever payload structure your API clients are sending. It may also help your cause if you handle exceptions appropriately.
Strategies to Resolve Python’s FastAPI Error 422 with Post Request When Sending JSON Data
If you’re encountering the Unprocessable Entity Error (422) when making a Post request in Python’s FastAPI using JSON data, it’s typically an indicator that the incoming data doesn’t adhere to the model defined in your program. Essentially, this error is related to validation and can be provoked for numerous reasons.
Data Model Mismatch:
The error might occur if the JSON body doesn’t match with the Pydantic model in your FastAPI application. To rectify such issues, ensure that:
• The property names in the JSON request are matching accurately with your data model.
• The data types of the provided values align with those expected by your data model.
Here’s an exemplifying snippet:
from pydantic import BaseModel from fastapi import FastAPI app = FastAPI() class Item(BaseModel): name: str description: str price: float @app.post("/items/") async def create_item(item: Item): return item
In this instance, the JSON data must encompass “name”, “description”, and “price” fields each adhering to their respective data types. If there’s a deviation, it could trigger an error 422.
Missing Required Fields:
A 422 error may also arise if the request omits a required field stipulated in the data model of your application.
For instance, presume we have the subsequent data model where a “description” field is tagged as optional:
class Item(BaseModel): name: str description: Optional[str] = None price: float
Dropping the “description” field from the JSON payload won’t induce any errors. But excluding a necessary field like “name” or “price” unequivocally will.
Misuse of Query Parameters:
Another possible factor is wrongly appending query parameters within the URL when trying to send JSON data in a body request. Each HTTP method and endpoint interprets request parameters differently.
To quote Roy Fielding, one of the principal authors of HTTP specification, “The query component contains non-hierarchical data that, along with data in the path component, serves to identify a resource”. This reinforces that query parameters should only be used for identifying resources and not for embedding data payloads.
When dealing with such an error, consistency, and accuracy are critical. Ensure that your JSON payload accurately reflects your data model including field names, data types, and mandatory fields. Ultimately, the precise strategic solution will pivot on the underlying cause of this Unprocessable Entity Error (422). More information can be found on FastAPI’s official documentation regarding error handling.
Advanced Solutions for Rectifying Error 422 in Python’s FastAPI on JSON Post Requests
Error 422 in Python’s FastAPI can be quite a headache if you’re dealing with Post Requests involving JSON data. Essentially, this error is linked to issues surrounding client-provided data. But worry not! As an error that all developers have faced at one point or another, various established solutions can aid resolution.
Firstly, the crux of Error 422 lies in JSON data issues. It tends to occur when your JSON object doesn’t match the expected schema. Below are the common reasons behind such a discrepancy:
“The only valid measurement of code quality: WTFs/minute.”
– Thomas Fuchs
- The absence of required fields: If your schema expects specific fields but doesn’t find them in the provided JSON object, it will raise Error 422.
- Variable Type Mismatch: Yet, another common offender is the mismatch between the type of field value your schema is expecting and the one provided in your JSON object.
- Data Design Flaws: Sometimes, the flaw lies in the way how data has been structured in your model design for the API interface. A deviation from these data structures while making a POST request may trip off error 422.
Once you’ve pinpointed the cause, here are some advanced tactics for modifying your JSON handling processes to unearth and fix these glitches efficiently:
Detailed validation errors
FastAPI comes bundled with excellent support for handling detailed validation errors that give insights into what exactly went wrong with your request. This often includes information on the location of the incorrect data (i.e., body, query, path, etc.), as well as the exact type of error and additional details about the incorrect value.
Method | Description |
---|---|
HTTPException |
Raise HTTPException with
status_code=422 . |
detail |
Marks an array of objects, each with details about a specific validation error. |
loc |
Location of the incorrect data in your request. |
Take advantage of Pydantic Models
Pydantic models have inbuilt checks for schema compliance. Applying these checks before making API calls can be an effective way to prevent Error 422 because of its requirement mismatch. The schema for Pydantic models is defined using Python type hints.
Client-Side Data Verification Measures
Before dispatching a JSON object from the client-side to a FastAPI application, you need to ensure that the sent data corresponds accurately to the expected schema.
– Use JavaScript validation during data entry.
– Include all necessary data fields.
– Match variable types with the expected schema.
For example:
{
userId: 1,
id: 1,
title: "Lady With A Fan",
body: "This painting by Marie Gabrielle... Image size is 14inches x 10 inches."
}
By critically analyzing and modifying how you handle JSON objects relative to your Schema, resolution for Error 422 becomes more straightforward. Remember, prevention is always better than cure!
Furthermore, in terms of SEO optimization, it’s good practice to include relevant keywords like ‘FastAPI’, ‘Python’, ‘JSON’, ‘Post Request’, and ‘Error 422’ throughout your articles and titles. You may also want to link to pertinent resources like FastAPI documentation or Python tutorials.
Enjoy your coding journey!
When working with Python’s FastAPI, you may encounter an Error 422 during a POST request while sending JSON data. This error often relates to Unprocessable Entities. Primarily, it notifies the server that the syntax of the incoming request entity is correct but was incapable of processing the contained instructions.
Causes of the Python FastAPI Error 422
Understanding potential reasons for this issue can streamline the debugging process:
-
Data Validation:
FastAPI uses Pydantic models for data validation. If an incorrect data type is passed, or if a required field is missing in the request body, a 422 (Unprocessable Entity) status code is returned.
-
Incomplete JSON body:
This may occur when the JSON object sent in the request fails to match the expected model.
Solutions to Fix Error 422 in FastAPI
One way to rectify this hitch includes:
1. Checking Data Sent: Verify that the data sent matches the shape and type declared in the Pydantic model, including nested attributes. Make sure no required fields are left blank.
2. Looking at POST Request Body: One possible cause may be incorrectly formatted JSON data sent in a request, therefore re-checking the body of your POST request is advised.
Here’s an illustrative example:
{ "name": "John Doe", "age": 30 }
In this case, ensure the complete information is encapsulated within the curly braces, and each key-value pair is properly separated by commas.
As noted by Linus Torvalds, “Talk is cheap. Show me the code.” Demonstrating a deep comprehension of the flows and loopholes of your codebase could alleviate these concerns. Proper error handling, code reviews, and thorough testing are fundamental in building robust applications.
This knowledge helps to navigate common roadblocks like Error 422. Remember that this error typically implies the server understood the request but refuses to authorize it. With Python’s FastAPI, you get real-time feedback about data validation errors making the debugging process far more feasible. This way, FastAPI ensures your APIs are designed with cleaner code, less prone to such errors, thereby enhancing overall performance.
Relevant Resources:
FastAPI Handling Errors
Pydantic Documentation