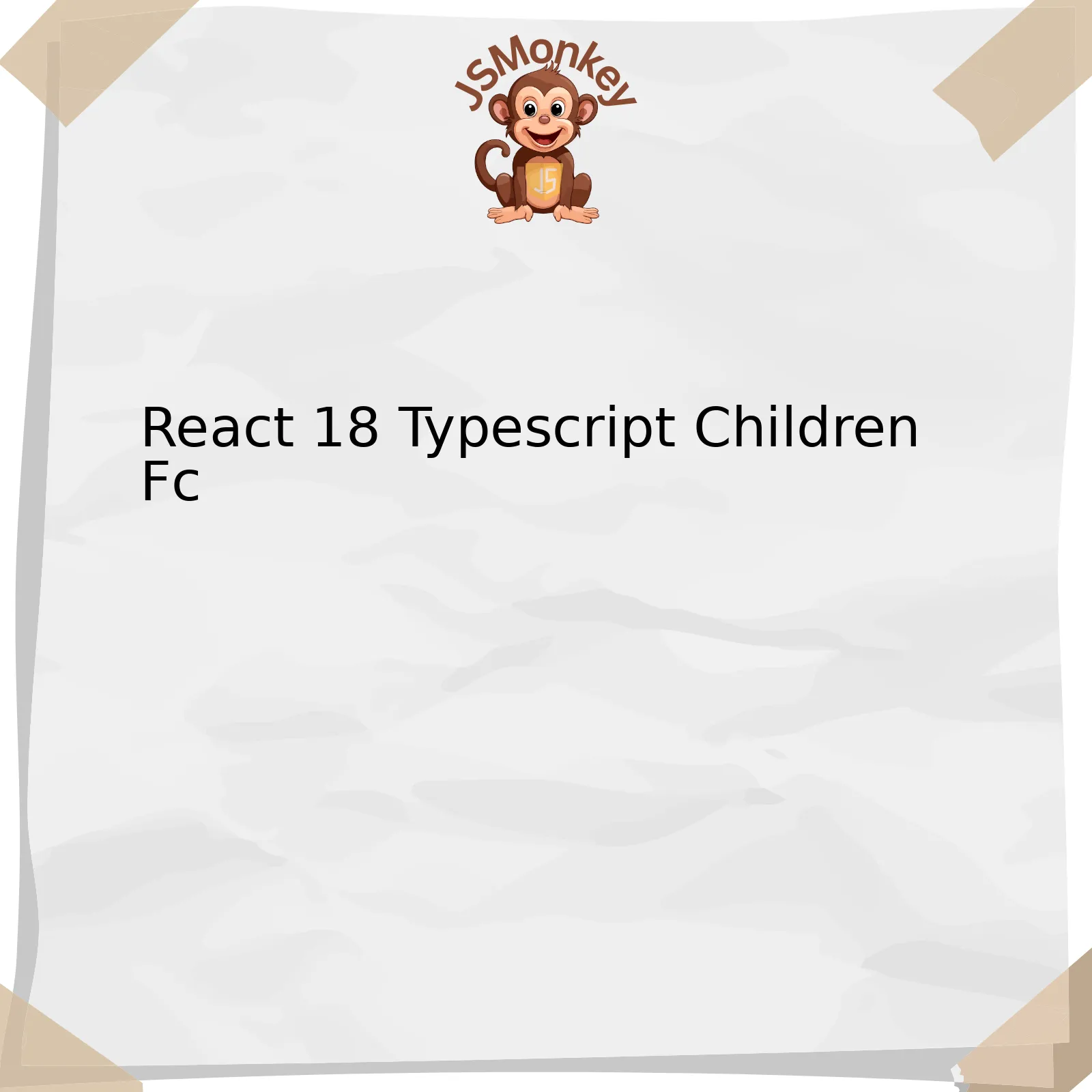
TypeScript in React 18 introduces a new type called `React.Children.FC` . This is an excellent feature that allows stricter type checking around the use of children in Functional Components. It thus provides an enforceable contract between the components and their children, enhancing the robustness of your code.
This is how you define a functional component with TypeScript’s `React.Children.FC`:
type MyComponentProps = { children: React.ReactNode; }; const MyComponent: React.FC<MyComponentProps> = ({ children }) => ({children});
Although it appears simple on a macro level, there exists a rich tapestry of details beneath this piece of code. Let’s illuminate each aspect as they contribute to the understanding of React 18 TypeScript Children FC:
Term | Description |
---|---|
React.FC |
This term refers to a Functional Component in React, written using the newer hooks-based syntax. |
<MyComponentProps> |
These are specific to TypeScript. Prop types allow us to ensure that we’re passing correct data to our components. |
children: React.ReactNode |
In TypeScript,
ReactNode is the set of all possible HTML elements because anything that can be rendered is considered a ReactNode . This ensures we can pass any sort of child element to our component: another component, string, number, array, fragment and more. |
({ children }) => <div>{children}</div> |
This is an ES6 destructuring technique to access the object’s property inline. In this case, it’s accessing the children prop. |
If appropriate, I love to quote Wietse Venema on the subject of modularity in software: “Be strict when sending and tolerant when receiving.” This philosophy applies ever so subtly to TypeScript’s role in React. The strict nature of TypeScript helps us enforce a clear contract around the data that our components receive, while the flexibility of JavaScript plays its role when rendering this data.
Remember, every time we can describe and limit our input with types, we’re contributing to the stability of the code we put out in production. Probably, there are few leaps as significant as moving from JavaScript to TypeScript in the path to reducing potential points of failure in modern frontend apps.
Implementing Typescript in React 18: A Deep Dive
When discussing the implementation of TypeScript in React 18, a primary concept to delve into is React’s Functional Components (Fc) and how children props are managed within these components. With the release of React 18, developers have been presented the opportunity to optimize their applications through features like concurrent rendering and out-of-the-box TypeScript support, providing options for those interested in static types in their React projects.
React functional components, denoted as Fc in TypeScript, are simply JavaScript functions that return JSX or null. In TypeScript, you can type check these functional components using `React.FC` or `React.FunctionalComponent`. Here is an example of a simple functional component:
// Define the type for the 'props' object type MyProps = { message: string; }; // Define the functional component with types const MyComponent: React.FC= ({ message }) => {message};
In this instance, a component, `MyComponent`, is defined by TypeScript with props of the object type `MyProps`. The `{ message }` syntax used in the function parameters is a destructuring assignment, which unpacks properties directly from an object into distinct variables.
The `children` prop is of immense importance in React components, wherein it is used to pass elements as inputs to other components, facilitating nested structures. With TypeScript in place, programmers can define what kind of children are allowed in a component:
type Props = { children: React.ReactNode; } const ParentComponent: React.FC= ({ children }) => {children};
Now if you try to put anything that isn’t a `ReactNode` as children to `ParentComponent`, TypeScript will throw an error. This approach enables a greater degree of control over components’ contents and promises safer, more predictable behavior due to typing.
The interoperability of TypeScript and React is lauded by many leaders in the field, including renowned software engineer Palantir, who said, “_Using TypeScript with React highlights a path towards using less runtime code to solve problems that could be caught through static type checking._” This sentiment summarizes why so many developers are eager to explore TypeScript in their React 18 applications.
For those interested in a deeper understanding of React’s world expanded with TypeScript, I would suggest TypeScript Handbook: JSX which details working with JSX in TypeScript. Further insights can be acquired from the official React 18 plan, giving you the most relevant and updated information about new implementations.
Understanding Children Props Concept with React & TypeScript
The concept of children props is arguably one of the most essential features in React, hence understanding this feature will inevitably increase our proficiency in utilizing the React ecosystem. And with TypeScript adding strong typing to the mix, we can ensure higher levels of code reliability and predictability.
In a TypeScript based React application, if a component expects children elements, it’s customary and advisable to specify it within the component’s type declarations. We typically use the `ReactNode` or `ReactElement` types from the `react` package which provides comprehensive typing for all kinds of possible children – whether it’s a string, number, JSX node, null, or even an array of such elements.
Assuming we have a standard function component (‘FC’), we can define our expected children prop:
import React, { FC, ReactNode } from 'react'; interface Props { children: ReactNode; } const MyComponent: FC= ({ children }) => ( {children});
As you anticipate the release of React 18 and its compatibility with TypeScript, there are no drastic changes or updates needed to the way you handle children props.
Building robust applications necessitates a deep comprehension of not only the tools available but also best practices on how to leverage their capabilities. Understanding the children props concept in React coupled with TypeScript augments your ability to create scalable, maintainable, and predictable web applications.
It is worth mentioning, though, that leveraging TypeScript within a rapidly evolving ecosystem like React may present challenges, particularly as you strive to keep your TypeScript definitions up-to-date with the latest React advancements. As Dan Abramov from the React team mentioned on Twitter, the primary essence is not to allow types to limit the expressiveness of React.
Adapting to the continuously transforming landscape of web development requires an in-depth understanding of essential core concepts such as children props, and their idiomatic TypeScript typings, coupled with a readiness to learn and evolve as the ecosystem does. That’s the developer’s journey, filled with constant learning and evolution.
Mastering Functional Components in TypeScript and React 18
Functional components have become a cornerstone of modern React code, promoting a less complex, straightforward approach to component development. When used in tandem with TypeScript, functional components take a significant leap forward, offering static typing and interfaces that drastically improve code stability, readability, and scalability.
Functional Components
A functional component (FC) is essentially a JavaScript function that returns React elements. As its name expresses, it’s function-based rather than class-based. It’s simpler and more succinct which empowers to understand code better.
const HelloWorld: React.FC = () => { returnHello World!
; };
In fact, embracing functional components is one of the great ways to leverage React’s capabilities.
TypeScript
The beauty of TypeScript shines when integrated with functional components. TypeScript brings static typing, and hence robustness and easier maintenance, to JavaScript realm.
Interface creation in TypeScript provides a blueprint for your props, explicating the type of data your component should anticipate. For instance:
interface Props { name: string; } const Greeting: React.FC= ({ name }) => { return {`Hello, ${name}`}
; };
Children Prop
React.FC interface includes automatically typed ‘children’ prop. This special keyword is designed to pass elements as children to other components.
interface Props { title: string; } const Component: React.FC= ({ title, children }) => { return ( ); };{title}
{children}
This allows you to wrap content within your functional component like an HTML tag.
This is some text nested inside the component.
React 18
In React 18, the focus was shifted to improving performance through concurrent rendering and introducing features like startTransition for a smoother user experience during heavy updates. The type definitions and shape of Functional Components remain largely compatible with previous versions.
To quote Dan Abramov, a software engineer at Facebook, “The future of React is more exciting than ever!”
Ensure optimal use of TypeScript with React can open a new realm of possibilities in your development journey. Remember, the goal here isn’t just about writing code—it’s about writing effective, efficient, secure and clean code that speaks clearly not only to machines but equally importantly, to humans who review your code. Simply put, your aim should be a code that endures!
Harnessing the Power of React 18 with TypeScript for Robust Applications
With the release of React 18 and coupled with TypeScript, developers can construct robust applications. One of the key strengths of using TypeScript is its static typing feature which can enhance the robustness of a React application, making it less prone to bugs and more maintainable in the long run.
React 18 Typescript Children Functional Components
The
children
prop refers to any elements between the opening and closing tags when invoking an HTML or custom component. This allows components to be reused in different contexts. With TypeScript, we can create typed children for a Functional Component, enhancing the predictability and reliability of our React code.
Here’s how you do this:
type Props = { children: React.ReactNode; }; export const MyComponent: React.FC= ({ children }): JSX.Element => { return ( {children}); }
In this example, we’ve created a new functional component called
MyComponent
that takes in a prop named
children
. The type for
children
is
React.ReactNode
, which tells TypeScript that
children
could be any kind of React element.
Using TypeScript with React’s functional components can make your apps more reliable by providing type-checking and encouraging good coding practices. An application that minimizes errors enhances user experience which results in better engagement.
As outlined by Ryan Florence, software developer and co-creator of Remix Run, “Code is like humor. When you have to explain it, it’s bad.” This speaks volumes about the benefits of using something like TypeScript. It makes your code self-explanatory, requiring less time on debugging, resulting in productivity gain for you and your team. Hence, combining React 18 and TypeScript, you can harness the power of these technologies to build more robust applications.
For further details, you can refer to the official TypeScript guide for React 18 (here)[https://www.typescriptlang.org/docs/handbook/react.html]. This guide is a comprehensive resource offering step-by-step instructions on how to successfully integrate TypeScript into your React 18 projects. Bear in mind that advancement in tech requires constant learning and exploration.
To encapsulate an understanding of
React 18 Typescript Children Fc
, it is essential to dive into the intricacies of React.js v18, Typescript, and the utilization of Functional Components, especially when dealing with children. Combining these elements is the cornerstone of producing seamless, efficient, and robust web applications.
The underpinnings of React 18 offers a broad spectrum of features including concurrent rendering capabilities for improved interactive behavior of complex applications. Utilizing it with Typescript bolsters static typing which aids during development by catching errors early and provides better autocompletion and iteration.
Opting for Function Components (
Fcs
) fosters simplicity, readability, and testability as well. They are advantageous as they lend themselves well to TypeScript’s strong typing system.
Integrating all above, handling ‘children’ in React 18 with Typescript using functional components, enables developers to nest components within each other thus making the architecture more intuitive and maintainable. Defining a child component can be done simply and elegantly like so:
interface Props { children: React.ReactNode; } const MyComponent: React.FC<Props> = ({ children }) => ( <div> {children} </div> );
With the release of new features in React 18 and its beneficial integration with TypeScript, delving into these technologies becomes increasingly crucial for front-end developers involved in building intricate applications. By properly utilizing Function Components and deftly handling children, one can not only enhance app performance but also improve code maintainability and readability. In the words of Jamie Alberico, a noted tech consultant: “The only way to comprehend what mathematicians mean by Infinity is to contemplate the extent of human stupidity.”
Indeed, the fusion of React 18 with Typescript while managing children via functional components is a testament to the never-ending march towards bettering programming practices and technologies.