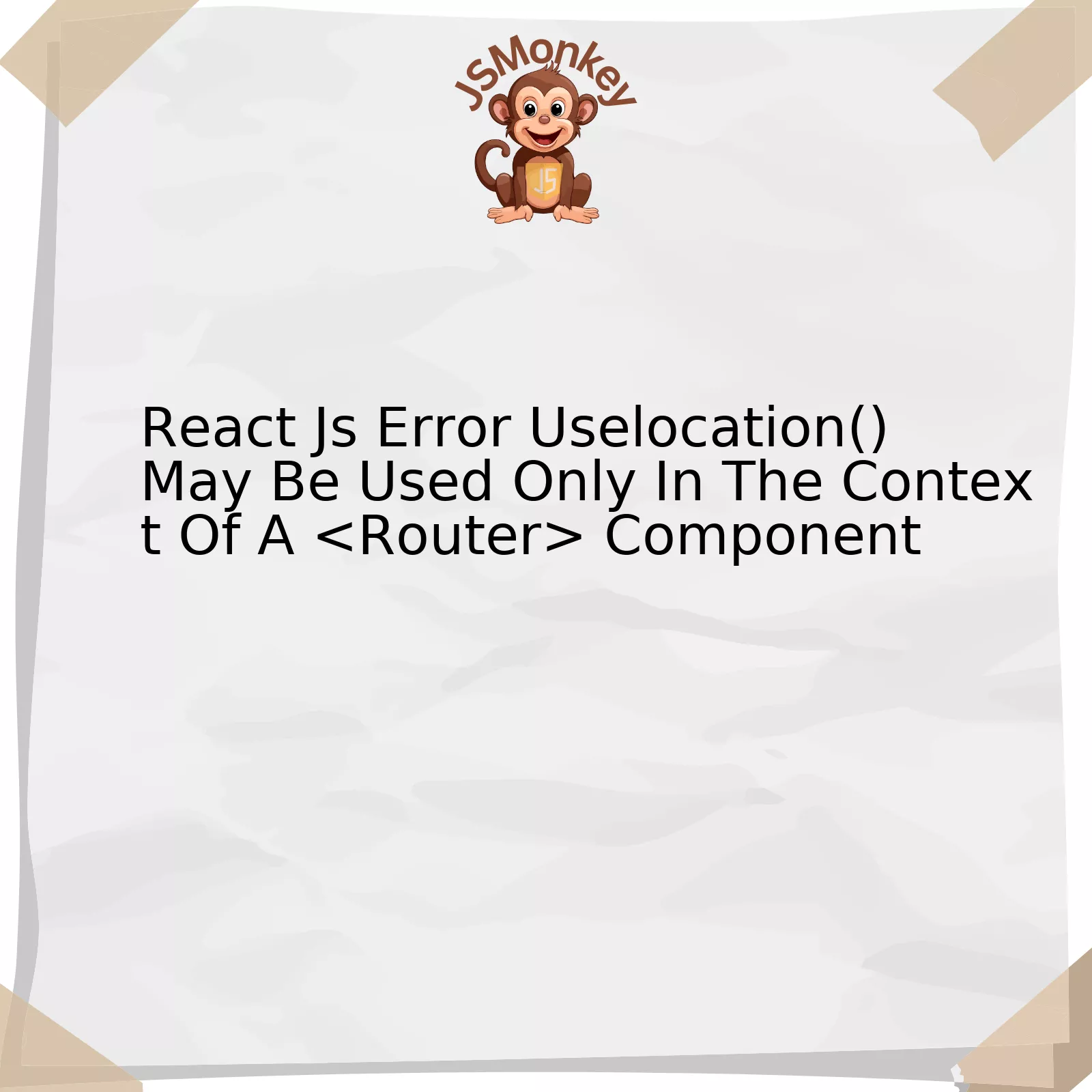
import { BrowserRouter as Router } from 'react-router-dom';
function MainApp() {
return (
);
}
Heed the remark of James Gosling – “The measure of success is not whether you have a tough problem to deal with, but whether it’s the same problem you had last year”.
This underscores the importance of continuous learning and application in programming. Therefore, understanding the context of components and hooks in React JS is vital for tackling similar issues effectively in future. Remember that the aim is always to strive towards creating robust, maintainable applications that provide users with an exemplary experience.
Understanding the UseLocation Hook in React JS
The
useLocation
hook is a part of the React Router library, which is commonly used in React.js applications to manage navigation. This hook provides access to the current location object that contains information about the current URL. This location object could include properties such as pathname, search parameters, and hash.
An error “React Js Error Uselocation() May Be Used Only In The Context Of A <Router> Component.” appears when you’re trying to utilize the
useLocation
outside of the context of a
Issue | Solution |
---|---|
useLocation used outside of a router component |
The component utilizing
useLocation needs to be wrapped in a |
useLocation used in a function invoked after the component renders |
Ensure all hooks are called from the top-level function body of a component before any other codes, including conditionals and loops |
Here’s a coding example of how you should properly use the
useLocation
hook:
HTML
import { BrowserRouter as Router, useLocation } from ‘react-router-dom’;
function DemoComponent() {
let location = useLocation();
return
;
}
//Using the component inside a Router component
export default function App(){
return (
)
}
In this example, we import the `BrowserRouter` from `react-router-dom` and use it to wrap the `DemoComponent`. Inside `DemoComponent`, we call `useLocation` which now has access to router’s context.
As a quote from Eric Elliott, a veteran software developer, goes: “Programming isn’t about typing, it’s about thinking.”
This underscores the importance of understanding the issue and approach to resolve it correctly. Grasping the concept behind hooks, like
useLocation
, and how they interact with the rest of your application, is an essential part of effective React.js programming.
Further reference about
useLocation
can be found on this React Router Documentation page.
Common Errors with UseLocation() Outside of Router Context
React, a popular JavaScript library for creating intuitive user interfaces, has a strong component system at its core. One such key component is the `useLocation` hook. However, developers often encounter an error when using this hook outside of a `
To comprehend this discouraging error properly, it is crucial to first understand the pivotal role that the `
Understanding the
<Router>
<Router>
Context
The `
This wrapping functionality also gives nested components access to vital navigational functions and states via React hooks, an example of which is the `useLocation` hook. Hence, it is paramount to use these hooks within the context, or say beneath the hierarchy, of a `
When the aforementioned error occurs, it suggests that the `useLocation` hook is being used outside of this beneficial router context, an action which the React router package does not permit.
Addressing the Error
To remedy this issue, ensure that your components, especially those employing the `useLocation` hook, are indeed located within the `
<BrowserRouter> <App /> </BrowserRouter>
In the above code snippet, the `
React’s Component Hierarchy Importance
The importance of observing React’s component hierarchy is encapsulated in this quote from Ryan Florence, a co-author of React Router:
“What if you could nest UI components and they would manage their own data and state automatically? What kind of world would that be?” – Ryan Florence
In conclusion, ensuring that you properly use the `useLocation` hook within the confines of the `
How to Correctly Implement UseLocation() within a Component
React’s `useLocation` hook is an indispensable part of the React Router package. It provides an object which encapsulates the current location. This useful information includes the current URL path, search parameters, and more, facilitating navigation and routing tasks within a single-page React application. If you’re encountering the error “uselocation() may be used only in the context of a
The rule to abide by regarding `useLocation` is that it must always be applied within components that are children of a
Consider this incorrect code:
html
function App() { const location = useLocation(); return({/* Other components */}); } ReactDOM.render(, document.getElementById('root'));
In this instance, `useLocation` is being used in the `App` component that isn’t wrapped by a
To rectify the issue, ensure `useLocation` is utilized within a component enclosed by a router, e.g.,
html
import { BrowserRouter as Router, useLocation } from 'react-router-dom'; function Header() { const location = useLocation(); // Further code... } function App() { return (); } ReactDOM.render( {/* Other components */} , document.getElementById('root'));
In the modified example, we’ve imported `BrowserRouter` from `react-router-dom`, and employed it to wrap the `App` component’s content. Now, the `Header` component, where we’re using the `useLocation` hook, correctly resides within the context of `
As Doug Crockford, JavaScript developer and author once said, “The good thing about reinventing the wheel is that you can get a round one”. While this quote might seem tangentially related, it emphasizes the concept of understanding what goes behind the scenes in coding and not just correcting errors superficially. In the context of `useLocation` in React Router, comprehending how routing context functions behind scenes helps developers to rectify not just this specific issue, but pre-empts potential mistakes when implementing other features related to routing or using react hooks.
Best Practices for Utilizing useRouter and useLocation in React.js
In the JavaScript library, React.js, navigation handling is vital for creating dynamic and engaging web applications. One such technique involves the use of useRouter and useLocation hooks in React.js. However, its effective usage requires some best practices to avoid errors including “useLocation() may be used only in the context of a
It’s crucial to understand that both useRouter and useLocation are hooks provided by ‘react-router-dom’. They provide valuable navigation information and functionalities within components. Both hooks must be used in the context of a
<Router>
component to operate properly.
“Proper usage of Hooks in React.js can tremendously affect the functional flow rendering your application more interactive. To get the most of hooks like useRouter and useLocation, you need to ensure that they operate cohesively keeping in line with the necessary conventions.” – An anonymous React.js developer
• First, wrap your entire app in the main entry point file inside a Router component. The type of router applied (BrowerRouter, HashRouter, etc.) depends on your specific requirements. Here is a simplified example:
<BrowserRouter> <App /> </BrowserRouter>
By doing this, all child components have access to the routing functionality provided by react-router-dom. If this step isn’t taken care of, you will encounter the aforementioned error because these hooks won’t function without a router in context to work with.
• Second, utilize useRouter and useLocation only inside functional components or custom hooks. They cannot be used in class components or outside the functional components’ body. Because React Hooks inherently rely on the scope of the functional component they reside within, any attempt to call them outside a component results in an error message. This usage maintains the encapsulation of functionalities, ensuring code cleanliness.
• Third, refrain from using these hooks conditionally or inside loops. It may lead to unexpected behavior since the order of hook calls matters in React.
• Best practice is to use useLocation for accessing the current location object instead of ‘withRouter’. The latter method was used with class components and often causes needless complexity with function components. The useLocation hook provides a more ergonomic and efficient way to handle these needs.
For instance, if you want to get access to the query parameters in the URL, you’d do something like:
import { useLocation } from 'react-router-dom'; const SearchPage = () => { const location = useLocation(); let query = new URLSearchParams(location.search); let searchTerm = query.get('search'); }
In summary, navigating efficiently in React.js mostly depends on having a good understanding of useRouter and useLocation hooks. Ensuring they’re correctly placed within the Router’s context will mitigate issues and enhance your web application’s routing logic. For more detailed information on these hooks, you can refer to the [official documentation](https://reactrouter.com/web/api/Hooks) of ‘react-router-dom’.
The error message “useLocation() may be used only in the context of a
Understanding this error requires comprehending the concept of Hooks and Context API in ReactJS. Hooks open up a significant number of features directly in your functional components, with useLocation() being one of them. This custom Hook makes it convenient to access the current location object which contains information about the current URL.
Understand | Implement | Solution |
---|---|---|
The error occurs when useLocation() is invoked outside a <Router> | Establish that all calls to useLocation() are within the Router’s boundary | Any call to this function can be nested under one of the standard pre-supplied routers |
ReactJS utilizes the Context API to propose a method to pass data through the component tree without having to pass props down manually at every level, such as implementing user authentication. The rule that Hooks must be called at the top level ensures that they follow the same order on each render, and this includes the react-router-dom Hooks.
This process helps in maintaining an orderly code structure while cutting down on unnecessary complexity. However, if there is any misuse or misunderstanding regarding these principles, it likely results in the conspicuous “useLocation() may be used only in the context of a
In software development, the journey is just as significant as shipping code and to quote a renowned technologist John Johnson – “First, solve the problem. Then, write the code”. Resolving this issue can potentially give you a higher understanding of how routers and Hooks work in React.
Always remember good practices to avoid this error:
* Make use of
React.Fragment
if needed to wrap multiple router-related components.
* Maintain a well-structured route configuration layout.
* Keep the routes associated with react-router-dom defined clearly.
Instead of trying to fight against or bypass the tools, it will always be more beneficial to grasp the underlying principles at play and adapt accordingly. That’s where true coding mastery lies.
To learn more about hooks, locations, and context in ReactJS, visit Official ReactJS Documentation.