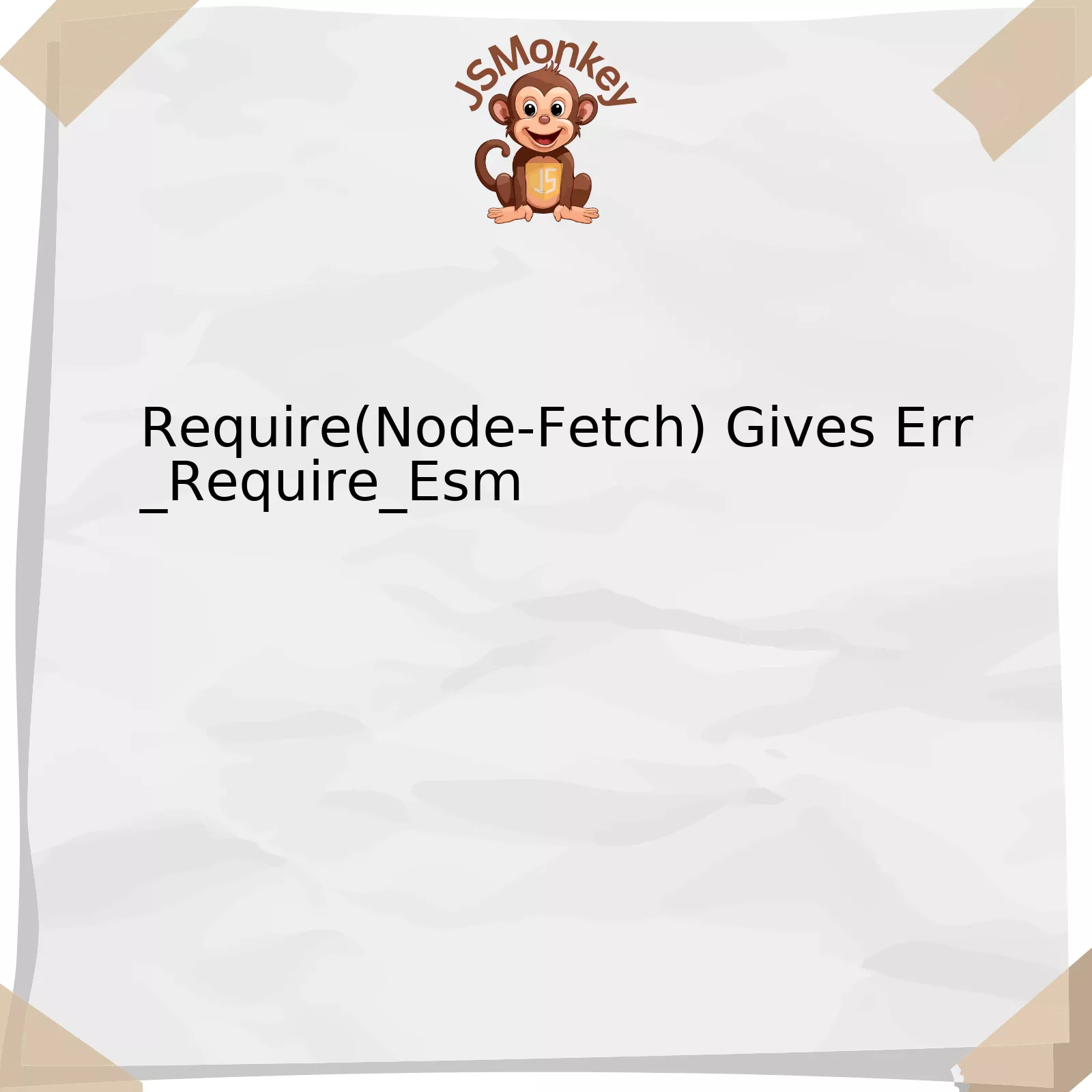
The issue of ERR_REQUIRE_ESM is a common occurrence when using Node.js. This error message indicates that the code contains ECMAScript modules which require ES6 syntax loading. When attempting to use the “require” function with an ES module (ESM), Node.js throws this error, preventing the software from running.
Parameter | Description |
---|---|
“node-fetch” | This is a light-weight module that brings the Fetch API to Node.js |
“ERR_REQUIRE_ESM” | Error thrown when Node.js encounters ECMAScript modules that need ES6 syntax loading |
“require()” function | A function used to import modules in Node.js. Not compatible with ECMAScript modules |
Node-fetch, being an ESM and not a commonJS module, runs into the mentioned interruption when we run “require(‘node-fetch’)”, giving rise to ERR_REQUIRE_ESM. As put aptly by Douglas Crockford, “ES6 was a significant change in JavaScript’s evolution”.
There are simple yet effective solutions to circumvent this issue:
Converting require() to import:
In Node.js, ECMAScript modules and CommonJs modules have different import styles. ESM utilizes “import … from …” syntax while Commonjs uses “require()”. Therefore, Navigating from require(“node-fetch”) to import fetch from “node-fetch” will potentially fix this issue.
A sample conversion from require to import can be portrayed like so.
Using the require function:
const fetch = require('node-fetch')
Switching to import:
import fetch from 'node-fetch'
Making use of “.mjs” extension:
Adopting the “.mjs” file extension when dealing with ECMAScript modules can be beneficial. This action influences Node.js to treat the scripting file as an ESM.
While this does not involve any changes in code, all you have to do is ensure that your file where you are trying to import ‘node-fetch’ has a ‘.mjs’ extension instead of ‘.js’.
Implementing the correct techniques and understanding the underlying basis of modules in JavaScript plays a crucial role in dealing with such errors, as it adds depth to understanding how ECMAScript modules interact with Node.js.
As per _Robert C. Martin_, “Clean code is not written by following a set of rules. You don’t become a software craftsman by learning a list of heuristics. Professionalism and craftsmanship come from values that drive disciplines.”source. The error ERR_REQUIRE_ESM is not just an obstacle but an opportunity to fulfill these values, providing us insights on better coding practices.
Understanding the Require(Node-Fetch) ERR_REQUIRE_ESM Issue
The
require(node-fetch) ERR_REQUIRE_ESM
error is likely caused by attempting to use a package that has migrated from CommonJS (CJS) to JavaScript’s standard module system ECMAScript modules (ESM). This change may be the root of the error message.
Firstly, it’s paramount to understand that Node.js traditionally used the CJS for its module system. However, ESM has been slowly gaining traction because of its various advantages, including enhanced performance and compatibility with browser-based Javascript.
To exemplify, node-fetch, a light-weight module inspired by window.fetch function in browsers for Node.js, migrated from CJS to ESM from v3.0.0 onwards, thereby leading to potential issues when trying the conventional
require(node-fetch)
syntax.
Debugging the ERR_REQUIRE_ESM error
If you’re encountering a
ERR_REQUIRE_ESM
problem in your project while using the require(‘node-fetch’) function, it essentially means that your code is treating this module as a CommonJS but it’s an ECMAScript module.
In such situations, refer to these following fixes:
• Using an older version of node-fetch: This is not a recommended approach, since old packages will lack the recent patches and features, but it can serve as a quick temporary fix. Switching to
"node-fetch": "^2.6.1"
might solve your issue momentarily.
• ESM Syntax: You need to switch from require() syntax to dynamic imports or static imports when using Node.js v14 or newer versions. Replacing
const fetch = require('node-fetch')
with
import fetch from 'node-fetch'
might resolve the error.
• Migrating to ESM: In this approach, you’d modify your entire project to use ESM instead of CommonJS. This is the most tiring but also forward-looking solution, as ESM is gradually becoming the industry standard.
Sy Brand, PM at Microsoft for TypeScript says: “I can understand that it’s frustrating when things break, but I can only recommend learning about these things and make an informed choice when deciding what libraries to use”
To genuinely counteract errors like
require(node-fetch) ERR_REQUIRE_ESM
, one must not only be wise in their tool selection but also remain abreast to changes in JavaScript technology. For additional guidance, you can visit Node.js’ official ES Modules documentation.
Exploring Possible Causes for the ERR_REQUIRE_ESM Error
The `ERR_REQUIRE_ESM` error in Javascript can surface for a multitude of reasons, particularly when you’re using Node.js modules. If a developer comes across this error when attempting to require `node-fetch`, it’s essential to understand that the root cause lies in the transition from CommonJS (CJS) which uses `require()`, to ECMAScript Modules (ESM).
There are several possible reasons you might come across this error:
Use of Latest Version of Node Fetch
If your project implements the new version of node-fetch (v3.0 or later), it has moved entirely to ESM, and no longer supports the CJS `require()` syntax. This change could be one reason why you’re facing the `ERR_REQUIRE_ESM` error.
For example, attempting to import node-fetch with this line of code will throw an error:
const fetch = require('node-fetch');
A replaceable solution would be to refactor your import statement as follows:
import fetch from 'node-fetch';
However, this modification needs your application to have support for importing ESM modules.
Lack of ‘type’: ‘module’ in package.json
One possible solution is to include “type”: “module” in your package.json file. By doing so, you instruct Node.js to treat `.js` files as ESM. You’d then get an outcome similar to below:
{ "name": "example_project", "version": "1.0.0", "type": "module" }
Remember that after setting “type”: “module”, you should use the `.cjs` extension for scripts that should load as CommonJS.
Third-party libraries
Certain NPM packages or third-party libraries not designed to work with ESM could lead to conflicts. Check any third-party dependencies for compatibility issues or see if they require updates.
Node.js Version
Ensure that you’re running a more recent version of Node.js that supports ESM. From Node.js 13.2.0 onwards, ESM is fully supported and doesn’t necessitate experimental command line flags.
As Jamie Kyle, a prominent figure in the JavaScript Community wrote, “JavaScript users are willing to make large changes to improve their workflows.” This sentiment holds true as the JavaScript community navigates the transition from CommonJS to ECMAScript Modules, overcoming challenges like the `ERR_REQUIRE_ESM` error along the way.
For an in depth understanding, here is a complete resource detailing how Node.js implements ECMAScript modules.
Remember to continually upgrade Node.js and your packages to the latest versions, manage your import statement properly, and handle third-party libraries delicately. This commitment can assist you in maintaining your Javascript code clean, understandable, and most importantly, error-free.
Steps to Resolve the Node JS ESM Issue in Your Application
Addressing the Node JS ESM (ECMAScript Modules) issue requires understanding the core difference between ECMAScript modules and CommonJS, which Node.js has traditionally used. The error message “Err_Require_Esm” typically pops up when you’re trying to utilize ‘require’ to import an ECMAScript module. Recent updates to many npm packages, such as “node-fetch”, have converted them into pure ESM packages, hence causing incompatibility issues with applications still using ‘require’ statements.
I will present a process to resolve this issue based on a typical Node.js application:
1. **Convert Your Project to ESM Format**: Instead of using CommonJS syntax for importing/exporting modules, you’ll need to migrate your project to the ECMAScript module system. Consider this small bit of code where I’ve used ESM syntax for importing and exporting modules:
import fetch from 'node-fetch'; const fetchData = async (url) => { const response = await fetch(url); return response.json(); }; export default fetchData;
2. **Update Your Package.json File**: Following the conversion to ESM, you must set the “type” field to “module” within your package.json file. This modification instructs Node.js to treat ‘.js’ files as ESM. Your package.json should look like this:
{ "name": "your-project-name", "version": "1.0.0", "description": "", "type": "module", ... }
3. **Replace ‘require’ with ‘import’**: Node.js recommends replacing ‘require’ with static ‘import’ or ‘export’ statements. As per the compatibility notes on Node.js doc, due to its support for asynchronous loading and other features, ESM is recommended for new projects [1].
4. **Use Dynamic Import If Necessary**: In scenarios where migration to ESM is not feasible, you might consider using the ‘import()’ function which returns a promise for the module, which can be awaited.
import('node-fetch').then((fetch) => { // use fetch here });
Remember, making major changes to an application’s architecture and codebase should only be undertaken after careful consideration. When all possible solutions have been exhausted, try reaching out to knowledgeable peers or seek advice from reputable online sources.
In the words of Robert C. Martin, renowned software professional, “The only way to go fast, is to go well”. Therefore take your time with this transformation process, ensuring that the ECMAScript modules are properly integrated into your Node.js application, helping it to run smoothly.
Technical Insights: How to Avoid The Common Require Issues In Future
When dealing with the issue surrounding “Require(Node-Fetch) Gives Err_Require_Esm”, it is crucial first to understand that this error is primarily caused by the new ECMAScript modules (ESM) in Node.js. Essentially, this error suggests that a developer is attempting to “require” an ECMAScript module, which can lead to challenges when not handled appropriately.
For improved clarity, ECMAScript modules are now the official standard format to package JavaScript code for reuse. However, developers must keep in mind that ESMs are only fully available in contemporary versions of Node.js. As such, these updated methods of handling and implementing module structures might intervene with traditional require module operations, leading to the occurrence of the said error.
To grasp how best to avoid common require issues in the future, consider the following key insights:
– ESM Mismatch: Always remember that the issue generally arises due to the mismatch of ESM with CommonJS patterns of coding. This means old codes were run on the system through the CommonJS module system and now when those codes are required using ESM, errors like this might pop up. Therefore, adapting your codebase to be compatible with both ESM and CommonJS can help preemptively mitigate this problem.
– Detailed Insight of Code: With JavaScript coding, gaining a detailed understanding of the code’s shared and encapsulated units presents a valuable technique to help avoid future challenges. Familiarize yourself with the required patterns when importing and exporting components can certainly prove beneficial.
– Understanding Module Systems: Node.js uses two major types of modules, including CommonJS and ES Modules. Grasping the inherent differences between these contemporary module systems will better equip you to handle integration and interoperability issues, thus mastering proactive error prevention.
Robust online resources exist to guide your comprehension of these module operations, such as Node.js official documentation here.
// CommonJS - Old way const fetch = require('node-fetch'); // ESM - New way import fetch from 'node-fetch';
In a related topic, Brendan Eich, the creator of JavaScript, once said, “Always bet on JS”. There’s no better reminder of this error’s occurrence to remind you that, just like in every programming language, errors and bugs are common. They are also vital stepping stones toward better masteries of the language itself.
Despite the changes put in place or integration problems that may arise with time, it is essential to note that the fundamental concepts stay intact and adaptable. Hence, understanding, learning more about the intricacies of JavaScript, and its evolving nature will not only help avoid these common require issues but also enhance your coding expertise overall.
Demystifying the Error: Require(Node-Fetch) Gives Err_Require_Esm
Looking beneath the surface, `Err_Require_Esm` is an error thrown by JavaScript when trying to use Node-fetch library in a common JS environment without ES modules. JavaScript has been evolving rapidly and this involves migrating from CommonJS to ECMAScript Modules (ESM), leading to potential complexities like these.
Solution:
The immediate workaround for tackling this issue is circumventing the use of import statements. Instead, you might consider reverting back to using a version of node-fetch that supports CommonJS. The following piece of code demonstrates it:
const fetch = require('node-fetch@2.6.4');
This ensures that you are importing a ComonJS compatible version of node-fetch effectively nullifying the error.
Support for ESM
While support for ESM in Node.js is behind experimental flags in versions previous to 12, in Node.js 13 and later it becomes a stable feature. For applications relying on ESM, upgrading to a recent Node.js version should resolve this problem. This transition clearly indicates a trend towards embracing standard ECMAScript modules as more libraries join this shift.
Another Option: Use ‘isomorphic-fetch’ Instead
If backward compatibility or specific project constraints make updating node version or switching between standards inconvenient, the ‘isomorphic-fetch’ library might offer an alternative. It follows the same syntax as node-fetch and adds universal fetch implementation for both client and server.
Here’s a link
where you can learn more about isomorphic-fetch.
As Eric Elliott, a renowned JavaScript expert puts it, “Any application that can be written in JavaScript, will eventually be written in JavaScript.”
This scenario precisely portrays the evolving landscape of JavaScript and it’s a routine part of working with this dynamic language. Facing an `Err_Require_Esm` while requiring node-fetch is just one such instance aiding us to upskill and keep ourselves abreast with these transformations.