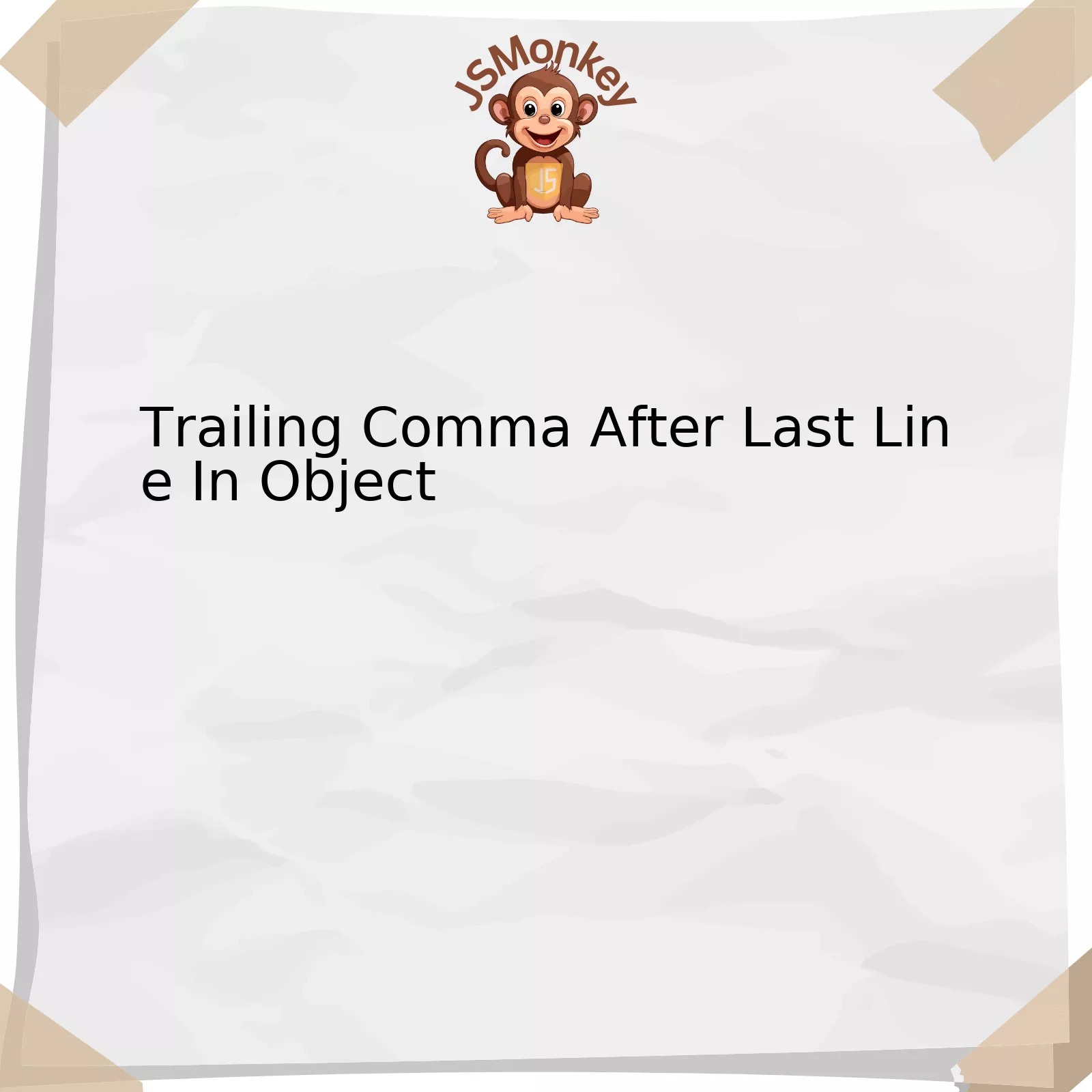
The topic in focus here is ‘Trailing Comma After Last Line In Object’. Let’s use a table to clearly understand the concept and its effect on Javascript objects.
html
Without Trailing Comma | With Trailing Comma |
---|---|
var obj = { |
var obj = { |
In JavaScript, an object is made up of key-value pairs. As showcased above, the first depiction doesn’t have a trailing comma after the last line while the latter has one. Both definitions are valid; what changes is essentially related to style guidelines and preferences, versioning control systems (VCS), and code readability and manipulation.
A couple of reasons for considering the inclusion of a trailing comma:
– **Versioning Control Systems**: In VCS like Git, when lines are added or removed, only those particular changes get tracked. When an item with an appended comma gets introduced into an already existing list without any trailing commas, two lines register as altered: the new line and the preceding line that now carries an extra comma. For cleaner, more efficient diff-logs, many favor the trailing comma approach.
– **Modify Simplified**: If you want to reorder items within an object or array, it’s much easier when each item concludes with a comma (including the last item). It helps prevent accidental syntax errors and ease code manipulations.
However, old versions of Internet Explorer (IE9 and below) would throw errors with trailing commas. In current times, this issue is negligible but worth noting.
Here’s a quote on the overall essence by Nicholas Zakas, an influential figure in the JavaScript community: “The biggest reason I advocate for trailing comma usage is that it gives us cleaner diffs in version control systems.”
(Source)Understanding the Concept of a Trailing Comma in Object
The concept of a trailing comma, also sometimes referred to as a dangling or hanging comma, is one common in JavaScript and other programming languages. This syntax feature offers several practical uses that benefit developers. Its application, especially in Javascript objects, is worth discussing for its relevance in code readability, maintainability and version control efficiency.
A trailing comma is found after the last property-value pair in an object, array, or function parameters list, just before the closing bracket or brace. In JavaScript objects, it looks something like this:
let obj = { color: 'blue', size: 'large', };
At first glance, you may think it’s a typographical error or violation of coding conventions. However, it’s not only allowed but recommended by some coding style guides, like Google’s JavaScript Style Guide and Airbnb’s JavaScript Style Guide.
Let’s delve into the reasons why:
– Maintainability: Adding a new line to your object in the future requires fewer changes with a trailing comma already present. It avoids the need to add a comma to the preceding line, meaning less potential for simple syntax errors when modifying your code.
– Cleaner PR diffs: In terms of version control, trailing commas produce cleaner diffs in pull requests. When a new line is added without modifying existing ones, review processes become easier and more efficient as only true changes are reflected.
– ES2018 (ES9) functionality: Modern ECMAScript standards have cemented support for trailing commas even in function parameter lists, enabling consistent usage throughout varying contexts.
However, it’s important to note that the trailing comma has been a contentious feature across language versions. In ECMAScript 3, trailing commas in object literals were considered syntax errors. They were made allowable by ECMAScript 5, while Internet Explorer releases prior to version 9 did not conform with this change. It’s hence essential to consider browser compatibility when using this feature.
As Douglas Crockford, a tech influencer and author said, “There are lots of little things that add up to JavaScript being a quite good language, and I’m not embarrassed to admit it.” Trailing commas may seem like a minor aspect, but they exemplify how even small features can impact overall development process efficiency.
Impact of Trailing Commas on JavaScript Code
The use of trailing commas in a JavaScript Object can seem to be a somewhat inconsequential element when writing your code, but they do have an impact. Also known as ‘dangling commas’, ‘comma chasers’, or even ‘terminating commas’, these are additional commas that appear after the final property-value pair in an object, array, or argument list.
At first glance, it might seem odd to add an extra comma where no further properties are expected. However, there are certain advantages to using trailing commas in your JavaScript code.
In the context of objects, a trailing comma looks something like this:
var myObject = {
property1: “value1”,
property2: “value2”,
};
Advantages of using trailing commas in such instances include:
• **Easier Code Modifications**: When a new property-value pair is added at the end of the object, you will not have to worry about adding a comma to the previous line. Hence, the diffs in version control systems will show only the lines you have altered, making your modifications more clear and less prone to error.
• **Preventing Syntax Errors**: Forgetting a separating comma is a common mistake that JavaScript developers make. While modern development tools often catch such issues, trailing commas help by removing one possible point of failure.
It’s important to note that the use of trailing commas can potentially cause issues.
• **Backward Compatibility Issues**: Trailing commas are not supported in ECMAScript 3 or older versions, leading to syntax errors. If your script needs to run in Internet Explorer 9 or below, it’s better to avoid using trailing commas.
• **Array Length Differences**: In JavaScript, the `.length` method on arrays counts the elements within it including commas. The addition of trailing commas could lead to miscounts of array lengths and unexpected results.
As Mozilla’s JavaScript Guide good coding practice would be to always use them, or never use them.
“For objects, trailing commas have little downside and some upside. My vote is that for objects, you should feel free to do it,” says Flavio Copes, an experienced software developer. I tend to agree with this sentiment but also emphasize the importance of keeping in mind your target environment before proceeding to use trailing commas.
By understanding these points, you can make effective use of trailing commas in JavaScript code, which would help to improve code clarity, manageability, and reduce human errors.
Addressing Syntax Errors: The Case for Trailing Commas
Trailing commas in JavaScript can seem like a stylistic choice, however, they carry significant weight in terms of contributing to clean and error-free code. Especially interesting is the case of including a trailing comma after the last line in an object. Here, we highlight the crux of this argument and simultaneously elucidate why it’s worth talking about.
One fundamental aspect that you might need to know is the term ‘trailing comma’, also colloquially known as the ‘comma dangle’. To boil it down to its essence:
let myObject = { key1: 'value1', key2: 'value2', key3: 'value3', };
The trailing comma is that little chad hanging there at the end of our
myObject
, right after
key3: 'value3'
. Now let’s see how such a seemingly insignificant character offers beneficial impact on your JavaScript syntax:
– **Enhanced version control system diffs**: Adding a new property without a trailing comma forces us to change two lines (the new property and the addition of a comma to the previous one). This can cause extra noise in your version control system diff. Alternatively, with a trailing comma, we are only required to alter one line, making our changes more atomic.
– **Ease of add/remove/rearrange code**: Trailing commas make it easier to change or rearrange your code. Furthermore, it makes it less likely that you will introduce syntax errors, especially when quickly adding or deleting properties within an object.
– **Cleaner and clearer code reviews**: When working within a large team of developers, cleaner diffs mean cleaner and clearer code reviews. Therefore, using trailing commas could indirectly contribute to enhanced team productivity and smoother code collaboration.
Taking these factors into account, modern JavaScript (ES5 and beyond) allows for trailing commas in object literals. However, as we dip our toes deeper into the inconsistencies and peculiarities of browser implementations vis-à-vis trailing commas(O’Reilly Reference), Internet Explorer does not support trailing commas in JavaScript. As such, for those needing to write JavaScript compatible with IE, a linter like ESLint can help by specifying
"comma-dangle": ["error", "never"]
rule (ESLint Rules).
“Code is read far more often than it’s written – make sure it’s readable, atomic and doesn’t do more than necessary,” stated Guido van Rossum, author of Python language.
Therefore, while seemingly small, including a trailing comma after the last line in an object might lead to better code practices overall, contribute to a smoother development workflow, and help create manageable version control systems.
Transitioning to ES2017: Harnessing the Power of Trailing Commas
The introduction of ES2017 into the world of JavaScript garnered much attention from developers due to its numerous beneficial features. One particular feature intriguing developers is the trailing comma, also known as the dangling comma. Utilizing this feature can lead to a more efficient and cleaner coding experience. Particularly, it offers solutions on an issue that typically plagues large code bases with numerous objects: adding new properties or items at the end.
The Role and Advantage of Trailing Commas
So, you’ve probably encountered a pesky syntax error while inputting a comma after the last property in your object. This can be particularly frustrating when dealing with extensive array lists or complex nested data structures. ES2017 introduces trailing commas to resolve this problem.
const myObject = { name: "John", age: 30, city: "New York", };
By using a trailing comma after the last property, editing and managing such code becomes less error-prone. If another developer adds a new property line to the end, they won’t accidentally cause a syntax error by omitting the necessary comma at the end of the previous line.
Enhancing Version Control Systems
The trailing comma is not only functional but also works to the advantage of version control systems like Git. In cases when only a single line is altered in a multi-line array declaration or object literal, a trailing comma results in cleaner diffs. The version control system recognizes without confusion what line has been modified, added, or removed.
Browser Compatibility Issues and Resolution
While ES2017 encourages the use of trailing commas, keep in mind that JavaScript specifications before ES5 do not support them, which can potentially cause browser compatibility issues. To get around this, Babel, a popular JavaScript compiler, can be used to compile your code down to ES5, eliminating any issues created by trailing commas in the process.
ESLint: Enforcing Trailing Commas
For consistency and to avoid syntax inconsistencies across different pieces of code, a rule regarding trailing commas could be added with ESLint tool. This ensures every last item or property within an array or object will have a trailing comma. The following is an example showing how you can enforce such a rule.
// .eslintrc { "rules": { "comma-dangle": ["error", "always-multiline"] } }
As Bill Gates once said, “The first rule of any technology used in a business is that automation applied to an efficient operation will magnify the efficiency. The second is that automation applied to an inefficient operation will magnify the inefficiency”. Hence, using tools like Babel and ESLint along with trailing commas allows for cleaner, more efficient JavaScript code.
Relevant resources:
Understanding the role of the trailing comma in JavaScript objects can best be understood in terms of cleaner code management and reduced error potential. Placing a comma after the last property-value pair in an object is not only completely legal according to the ECMAScript 5 (and later) standard, but it offers some practical benefits as well:
• Git Differentials: Git’s diff command provides a clear, line-by-line comparison of changes made to the code. If items are added to your JavaScript object without a trailing comma on the preceding line, the differential rendered by git will show two changes: the new line, and the modified line where a comma was added. By consistently employing trailing commas, your git diffs become clearer, showing only lines with entirely new or removed information.
• Coding Efficiency: It’s easier and less error-prone when adding or removing properties from a JavaScript object to maintain the trailing comma on all lines. It’s easy, during rapid development, to omit the comma altogether when adding new properties or swap lines around. Also, if you’re grouping similar properties together for readability, you don’t need to worry about remembering to add the comma when you insert new lines within the object.
• Code Style Consistency: Trailing commas help to ensure that every line in your JavaScript object adheres to the same format, improving consistency across your codebase.
Just be cautious about wider compatibility. For instance, Internet Explorer 8 and older versions do not support trailing commas in JavaScript objects, so mind the necessity of broad browser support in your project.
It’s worth noting, however, as Douglas Crockford, the creator of JSON, comments, “There are no good parts in the comma. It gets things wrong.” [http://crockford.com]. The essence of his statement is a note of caution that, while the trailing comma can be useful for code management, it may lead to issues if not handled correctly, such as compatibility problems with certain older browsers.