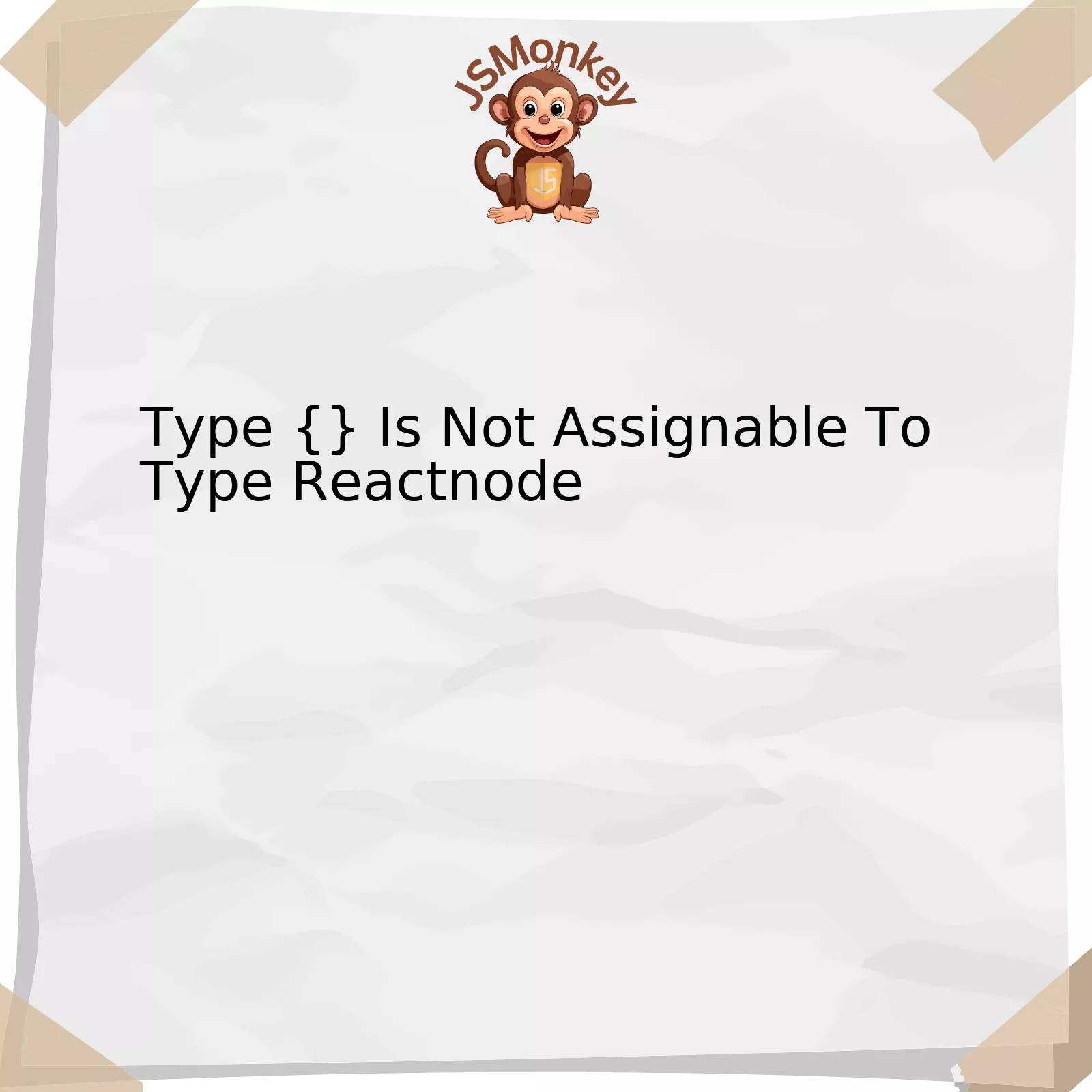
When you encounter the error “Type {} is not assignable to type ReactNode” in the JavaScript library React.js, it indicates a conflict between data types during the assignment operation. Now let’s dive deeper into this issue with a structured representation below.
Term | Description |
---|---|
Type {} | This refers to an object type in JavaScript. An empty object {} has no properties or methods. |
ReactNode | A concept from React.js which represents a type that can be rendered. This includes React elements, strings, numbers, null, undefined, and arrays of ReactNodes. |
“Type {} is not assignable to type ReactNode” | This error occurs when trying to assign an incorrect data type (e.g., an empty plain object) to something expecting a ReactNode. Since an empty object is not renderable on its own, it cannot be assigned to a ReactNode without violating TypeScript’s strict typing. |
Delineating this error, it requires us to have a solid understanding of TypeScript’s static type checking system. When we use TypeScript with React.js, every variable, function parameter, and object property has a specific type that can’t be changed later. So, if we attempt to assign an empty object `{}` to a property of `ReactNode` type, TypeScript throws this error because an empty object isn’t something that React can render directly to the DOM.
The best way to resolve these sorts of TypeError is by ensuring that the right data types are used in the correct context – essentially, always assigning a renderable type to a ReactNode. It might range to using React components, JSX, strings, numbers, or arrays of the same, but plain empty objects are off the table.
Coding example:
javascript
const validReactNode: ReactNode =
; // This is fine
const invalidReactNode: ReactNode = {}; // TypeError: Type {} is not assignable to type ReactNode
“Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” – Martin Fowler, British software developer. This quote underscores how crucial it is for developers to write code that is logically accurate and contextually right, which includes assigning the correct data types, just as in our ReactNode case.
For further reading, please consult React’s documentation on TypeScript with React, which provides comprehensive discussion and examples on this topic.
Understanding “Type {} is Not Assignable to Type Reactnode” Error
The error “Type {} is not assignable to Type ReactNode” in JavaScript, more specifically within the arena of React.js development, indicates a common issue regarding varying data types and object assignment. It’s fundamentally related to TypeScript, a statically typed superset of JavaScript that brings about strong typing along with other powerful developer tools.
The complexity ensues when the TypeScript compiler fails to compile your code due to a conflict between the data type you are trying to specify and the actual format or value the object consists of. You need to dissect this error into two key components:
• Type {}: In TypeScript, an empty object {} signifies an item that doesn’t comprise any explicitly declared properties. It resembles the sketch of what an object should look like but devoid of substance or any unique characteristics. It is, in short, an ’empty shell’.
• ReactNode: On the flip side, ReactNode holds a broader definition — encapsulating every possible return type for a React component. This could be any data type: a string, a number, or typically, an element that returns a renderable entity.
Now making the connection, the error implies that TypeScript encounters issues while trying to assign an empty object (Type {}) to a variable which was expected to contain a ReactNode.
One way to overcome this issue would revolve around Type Assertion, commonly known as casting. Using the ‘as’ keyword provides you the capacity to define the type of value any given variable should hold.
A practical implementation example might look something like:
let example : React.ReactNode; example = {} as React.ReactNode;
This tiny piece of code gracefully resolves the issue by instructing TypeScript to view the empty object as a ReactNode variable.
Additionally, thinking about avoiding these errors in the future, it’s vital to embrace mindful best coding practices, such as:
• Adhering to clear and concise type declarations.
• Leveraging TypeScript’s powerful static typing to reduce the chance of these runtime surprises from occurring.
• Staying up-to-date with evolving versions of TypeScript and React.
Remember this quote from Andrew Hunt, author of ‘The Pragmatic Programmer’:
“No one in the brief history of computing has ever written a piece of perfect software. It’s unlikely that you’ll be the first.”
This emphasizes the need to handle exceptions gracefully while not being too hard on oneself for encountering them. After all, overcoming these hurdles makes us better developers.
To gain more depth about these concepts feel free to read about TypeScript Basic Types and
React Components.
Unpacking the Concept of Types in TypeScript and ReactNode
When diving into TypeScript and ReactNode, it’s fundamental to understand how types operate in these contexts. In the scope of TypeScript, a type-checking superset of JavaScript, types categorize values based on their properties — for instance, booleans signify true/false values, while numbers include float and integer values.
ReactNode is also a type peculiar to React, essentially covering anything renderable by a component, such as JSX elements, strings and numbers, or even other React components.
However, assuming that you’ve encountered an error message like “Type ‘{}’ is not assignable to type ‘ReactNode'”, this suggests that TypeScript’s type checker is attempting to ascertain whether a certain value or entity accords with the ‘ReactNode’ definition, but is failing. The curly braces ({}) signify an empty object, which does not meet the demands of what a ReactNode should be.
To clarify this issue a bit more, consider the following breakdown:
type StandaloneComponent = () => {}; type DisplayComponent = () => null;
In the above instances, both
StandaloneComponent
and
DisplayComponent
technically return valid JSX — yet only
DisplayComponent
satisfies the ‘ReactNode’ definition. This happens since ‘null’ is indeed a legitimate render output, being a valid ‘ReactElement’. Contrarily,
StandaloneComponent
, although passing syntax checks, will not actually render anything within a React context.
The soul of the machine is the processing of symbols, and taming the language we use to converse with it brings us closer to the harmony foreseen by pioneers like Edsger Dijkstra — “‘Program testing can be used to show the presence of bugs, but never to show their absence.'”
Seek solutions by tailoring your code in accordance with the requirements about what qualifies as valid JSX. Satisfying the ‘ReactNode’ type would then be seamless, ensuring that your TypeScript-enhanced JavaScript retains maximum clarity and safety. Use stringent typing responsibly to circumnavigate troublesome errors like “Type ‘{}’ is not assignable to type ‘ReactNode'”.
Resolving the Limitations of Using Empty Object as A Type in ReactJS
The use of empty objects as a type in JavaScript and consequently ReactJS can sometimes pose challenges. These limitations often manifest themselves when you attempt to assign the empty object type, denoted as
{}
, to a type
ReactNode
. This is because the type
ReactNode
in React encompasses a wide range of possible types, including string, number, boolean, array, and many more.
Using
{}
would imply that your component could render any data type without a logical fallback, or it won’t render anything at all if an error occurs. This is inconsistent with the expectations of a
ReactNode
, and will most likely yield a TypeScript error stating: “Type ‘{}’ is not assignable to type ‘ReactNode'”.
Error Statement |
---|
Type {} is not assignable to type ReactNode |
In order to navigate this issue we may use the
any
type, instead of
{}
, to denote that a variable can be of any data type. However, this approach should be used sparingly as it removes the benefits gained from static typing. Traditional static typing ensures that all your variables adhere to their declared specific types allowing for better maintainability and avoiding potential run-time errors.
An alternate solution would be the utilization of a more specific type – one that aligns with what the component is set to render. This practice, while requiring a little more setup initially, significantly simplifies debugging and promotes improved code readability.
A practical example would be if your component is designed to render text nodes only. The variable that holds the node value should be declared of type
string
, instead of the less specific
{}
or
any
.
let textNode: string = "This is a text node";
This code snippet will reduce the likelihood of encountering TypeScript errors since it accurately describes what type is expected and, by extension, what kind of data the component is capable of rendering.
The algorithmic thinker Donald E. Knuth once said – “Let us change our traditional attitude to the construction of programs. Instead of imagining that our main task is to instruct a computer what to do, let us concentrate rather on explaining to human beings what we want a computer to do.”
Best Practices for Troubleshooting and Avoiding TypeError in Your Code
The TypeError, which often reads “Type {} is not assignable to type ‘ReactNode'”, is a common error in the realm of JavaScript development. Its typical occurrence lies within codebases that utilize frameworks like React. Understanding and resolving this issue requires an appreciation for TypeScript’s static nature and React’s dynamic child element handling.
Firstly, think about TypeScript. It’s a strict syntactical superset of JavaScript that adds static typing. This means that it keeps track of the type of variable throughout the application’s runtime. If the programmer tries to change this type or arbitrarily assign an incompatible type, TypeScript will raise an error.
Then consider React. React uses the term ‘ReactNode’ as its basic, primitive node type. Elements on your page are either a string, number, boolean, null, DOM elements, or arrays; all signified by this one all-encompassing term. Nonetheless, in cases where you might wish to pass empty objects {} as children to your component, TypeScript isn’t happy because, technically, an empty object isn’t a valid ReactNode.
How can developers tackle this nightmare? With an array of practices:
- Use appropriate data types: Always ensure your types align correctly between variables, functions, and return values. In specific TypeScript-React cases, avoid passing an empty object as a ReactNode.
- Overspecification: Specifying data type in detail helps eliminate errors that could arise from wrong assignments. Instead of declaring just a plain object, define the details of the object: this arms TypeScript with additional knowledge of what it should expect.
- Utilize dedicated error-handling strategies: Try implementing generic error boundaries around components to localize potential problems without disrupting the entirety of your JavaScript project.
- Code reviews: A second set of developer eyes on your code can sometimes prove invaluable. They might see something that you’ve missed, or provide advice on alleviating this static-dynamic type imbroglio.
The most relate case to React can be seen here, where definitive answers and discussions can be found.
“I am always doing what I can’t do yet in order to learn how to do it,” Vincent Van Gogh once said. Remember this quote when facing any errors or challenges throughout your coding journey. Keep learning, keep growing, keep adapting.
The topic at hand, “Type {} Is Not Assignable To Type ReactNode” revolves around a common type declaration error encountered by developers working within ReactJS’s TypeScript environment. This programming conundrum often arises when a component’s children prop isn’t defined in a way that matches the actual value passed into it.
In the broad universe of Javascript objects, an empty object ({}) is a perfectly acceptable entity. However, the issue crops up when the same is expected to act as a ReactNode. ReactNode refers to the type used for children in React components and includes the possibilities of string, number, JSX Element, or even another function returning a ReactNode. A disconnect ensues as an empty object doesn’t match these designated types.
Few key points illustrating the crux of this underlying issue are:
• Type mismatch primarily leads to this error. While coding in ReactJS with TypeScript, defining the precise data types is crucial. Using the wrong type can lead to unforeseen errors.
• The use of generic objects can cause ambiguity. General-purpose objects, such as {}, don’t provide enough specifics about the type of contained values, sparking potential issues.
• Correctly mapping the component’s props can help resolve this issue. It ensures data passing between components confirms to the expected type structure.
Revisiting one’s grasp on variable typing in JavaScript and state management in React might open doors for elucidating the situation {newline}. An understanding of JavaScript objects and TypeScript fundamentals could come handy while debugging such issues. If a child component isn’t expecting an object but receiving one, developers should revise the component’s supposed data structure, or better yet, validate their proptypes thoroughly.
On one famous quote, Bill Gates once said, “Measuring programming progress by lines of code is like measuring aircraft building progress by weight.” In context to our current topic, trying to debug code without understanding the underlying structure and syntax is analogous to blindly judging the efficacy of code by its length. Hence, a deep dive into code, with an acute focus on the specificity of objects and types in JavaScript, prepares one to solve such errors.
However, all developers, beginner or expert, occasionally encounter red herrings as they innovate. Programming indeed mirrors life – it’s about learning, adapting and plowing ahead towards seamlessly functionality.