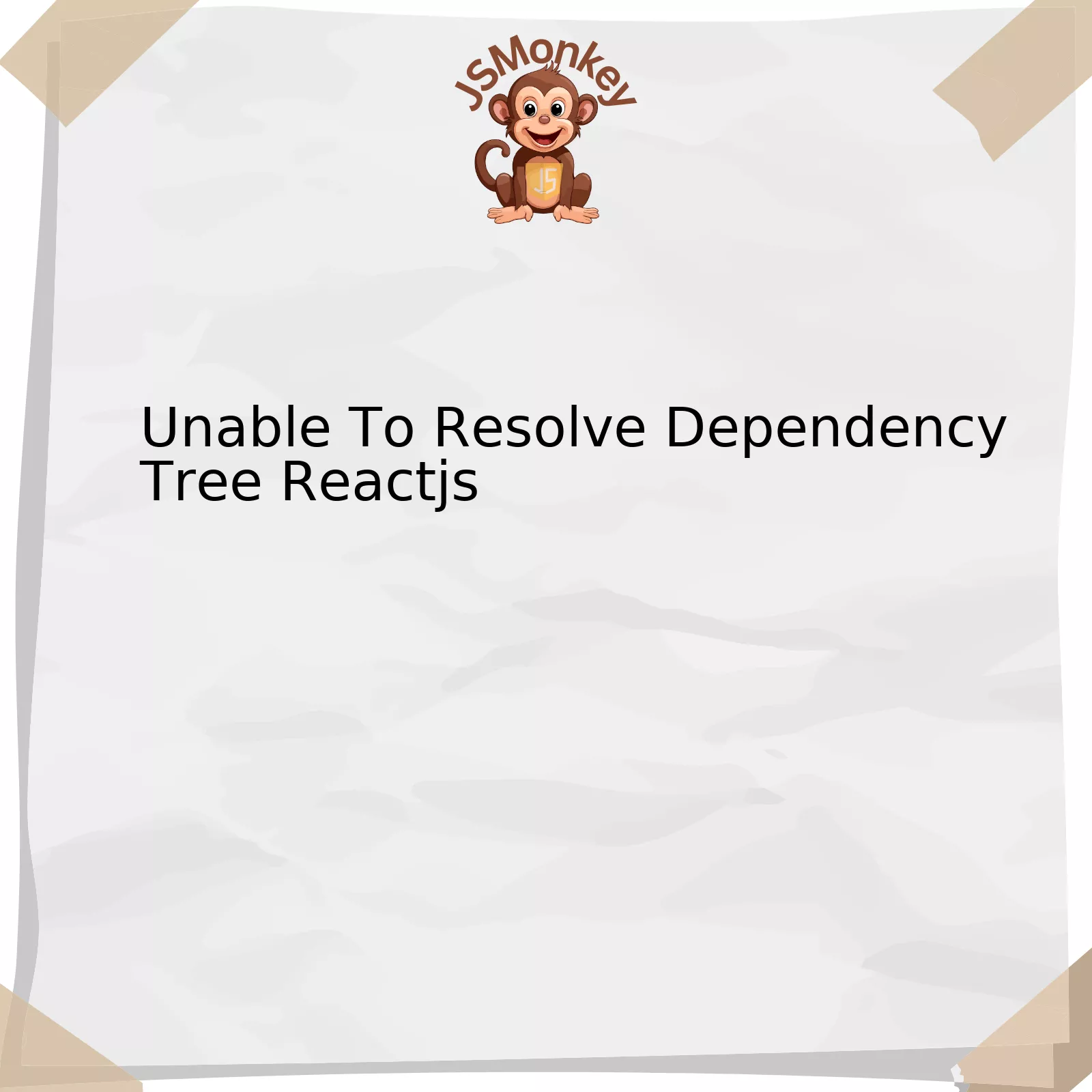
The issue of “Unable To Resolve Dependency Tree” in ReactJS is intricately linked with managing dependencies in the npm (Node Package Manager) ecosystem. Given scale of how many libraries and versions exist, conflicts often arise. Let’s delve deeper into this complex topic.
Firstly, we’ll briefly examine an illustrative table representing the potential confusion in resolving dependency trees:
Project A | Project B | Project C |
---|---|---|
Relies on Library Z v1.0 | Relies on Library Z v1.1 | Relies on Library Z v2.0 |
This table pithily displays dilemmas that could occur throughout multiple projects and dependencies; Project A may be reliant on a different version of a library than Projects B and C, causing collisions and making resolution problematic.
Now, let’s traverse through this mud of intertwined package dependencies.
The particular error message “Unable to resolve dependency tree” suggests that npm cannot solve the version requirements dictated by your project and its libraries. This nexus of dependencies forms what is referred to as the ‘dependency tree’. The nodes represent libraries your project is utilizing and there can be numerous collisions when libraries are asynchronous in their requirements for other libraries’ versions.
When you encounter this error, it’s generally due to one of these primary reasons:
– Libraries stated in your project require conflicting versions of another library.
– There are external issues related to the npm registry.
For the first issue, consider employing the command
npm ls
. This will log out your dependency tree along with problems so you can identify where collisions are occurring. To handle the second issue, attempt rerunning your installation commands.
There’s a reinstating quote from Addy Osmani (Google engineer) on this: “First do it, then do it right, then do it better”. This encapsulates the programming situation; first solve the immediate conflicts, and then revisit libraries causing persistent problems – you may require different versions or even altogether new solutions to satisfy your project needs.
Finally, npm 7 does have a dedicated feature to automatically install peer dependencies, which although helpful, can exacerbate conflicts. You can use the
--legacy-peer-deps
flag with npm for bypassing these auto-installations which might eliminate conflicts in some scenarios.
GeeksForGeeks provides an insightful exploration on this topic. Familiarity about intricate library configurations is indispensible for adept operation within the ReactJS environment.Understanding the Error: Resolving Dependency Tree in React.js
The error “Unable to Resolve Dependency Tree” in React.js usually occurs when trying to install packages or dependencies that have conflicting versions. These conflicts can interfere with your application’s process of building a correct dependency tree.
When you’re developing with React.js, the dependency tree is vital as it represents the interconnected hierarchy of all modules and libraries your application needs to function appropriately and deliver the desired performance.
Error type | Reasons | Solutions |
---|---|---|
Unable To Resolve Dependency Tree | Conflicting versions of packages or dependencies | Verify correct versions are installed, use ‘npm-force-resolutions’, downgrade npm/node.js version |
Finding the Cause:
- Incompatibility issues within the
package.json
file: It’s possible that the package you’re trying to add doesn’t match the version specified in the
package.json
file.
- Conflicting versions of global and local packages: If the global installation of a package is different from the local one, this kind of error can occur.
- NPM or Node.js version conflict: Sometimes, latest versions of NPM or Node.js have compatibility issues with certain packages, causing the error.
Solving the Error:
- Verifying Correct Versions: Make sure the installed packages or dependencies are compatible with each other and with the version specified in the
package.json
.
- Using ‘–force’ or ‘–legacy-peer-deps’: In some cases, using
npm install --force
or
npm install --legacy-peer-deps
may help resolve conflicts. However, these should be used sparingly as they can lead to unexpected results.
- Downgrading npm/node.js Version: If the issue is due to the npm or Node.js version, downgrading to a stable earlier version that is known to work with your set of packages could solve the problem.
As Alan Kay, a forefather of modern computing, once said: “Simple things should be simple, complex things should be possible.” Handling dependency errors indeed requires patience and systematic troubleshooting, but it conveniently encapsulates the process of coding: applying simple logical solutions to navigate around seemingly complex problems.
Further reading about this error and it’s solutions can be found in this StackOverflow thread.
Handling Common Causes for ‘Unable to Resolve Dependency Tree’
When resolving dependencies in React.js, it’s not unlikely to encounter the error ‘Unable To Resolve Dependency Tree.’ This issue often refers to inconsistencies between different package versions your project is trying to pull together.
If you’re dealing with this problem, understanding its common causes and knowing how to handle them could be a significant step toward effective troubleshooting and eventual resolution.
A Brief Overview of Dependency Management
In any given React.js project, you’re likely to depend on a number of external libraries or packages, each of which may, in turn, rely on other packages. To prevent chaos, these dependencies must be managed appropriately. This task primarily falls on npm (Node Package Manager) that keeps track of specific versions of every installed package using a special file called `package.json`. The problems begin once disparities emerge within these interlinked dependencies.
Dissecting the Dependency Error
The ‘Unable to Resolve Dependency Tree’ error can arise due to several reasons, including:
Incompatible Dependencies: If one library requires a version of another library that is incompatible with the version needed by your application or another dependency, you’re likely to encounter this error.
Force flag: Using the `npm install –force` command could lead to installation of incompatible dependency versions.
Lockfile Corruption: Over time, your npm `package-lock.json` can become corrupted or outdated, potentially leading to conflicts.
Handling the Common Causes
Addressing the ‘Unable to Resolve Dependency Tree’ error entails diagnosing and fixing the specific affliction affecting your project:
Updating Dependencies: In some cases, simply updating your packages might fix the issue. Use the following command to update all packages to the latest version:
npm outdated
npm update
Using npm as Local Dependency: If working with multiple developers on a project, variations in global installs of npm can cause issues. In such cases, installing npm as a local dev dependency could eliminate inconsistencies:
npm install --save-dev npm@latest
Installing Specific Versions: You might need to downgrade or upgrade specific dependencies to versions that are compatible with the rest of your project. A case might be when package A depends on version 1.0.0 of package B, but package C needs version 2.0.0.
Deleting and Rebuilding Lockfiles: Eliminating outdated or corrupted package-lock.json files and allowing npm to rebuild them from scratch can prove fruitful. To do so, you should run these commands:
rm package-lock.json node_modules
npm install
Useful Tools for Troubleshooting
In addition to these solutions, tools like npm-force-resolutions can come in handy when dealing with stubborn dependency issues, by overriding certain package versions defined deep within the dependency tree.
“First, solve the problem. Then, write the code.” – John Johnson. Understanding what causes ‘Unable to Resolve Dependency Tree’ errors and keeping this timeless wisdom in practice would potentially save you time debugging and keep your React.js coding efficient and enjoyable.
Best Practices for Managing Dependencies in React.js
The ability to manage dependencies effectively in React.js is crucial, especially when it comes to tackling issues related to the ‘Unable To Resolve Dependency Tree’ problem. The key strategies include choosing the correct versioning strategy, using package-locks, applying library code splitting and practicing regular updates.
Choosing the Correct Versioning Strategy
When it comes to resolving the dependency tree, one of the primary challenges usually arises from version mismatches amongst different packages. It’s recommended to align all dependencies to the same major version. Avoid using wildcard/star (*) in your
package.json
file as this could lead to incompatible versions of libraries being installed. Use tilde (~) for patches and caret (^) for minor updates which are generally safe.
Arin Bhowmick, Chief Design Officer at IBM, rightly said,
“Software development isn’t just about writing code. It’s also about what you do with it.”
Hence, stick to a release strategy that suits your project requirements, to ensure that new versions don’t break your build by initializing breaking changes. This aligns strongly with keeping a handle on your React.js dependency troubleshooting efforts(source).
Using Package-locks
The use of package-lock files such as
yarn.lock
or
package-lock.json
adds an additional level of security and predictability. These will lock down the exact version numbers of every package in your dependency tree. So, everyone working on the project will have the same versions of all dependencies to contain known compatibility. Make sure to commit these lock files into your version control system.
Applying Library Code Splitting
To optimize your application and avoid bloated bundles which might cause unresolved dependency issues, leverage code splitting technique. You can use the dynamic
import()
syntax in your React.js app to load parts of your application in isolation, thus reducing the size of your main bundle(source).
Practicing Regular Updates
Keeping all library dependencies up-to-date is an essential practice. Frequent updates not only ensure that you benefit from the latest features and performance improvements but also help to avoid ‘Unable to Resolve Dependency Tree’ complication, because newer versions often come with bug fixes or improved compatibility across other libraries.
Strategy | Description |
---|---|
Versioning | Avoid wildcard/star usage, use tilde or caret for safer versioning. |
Package-locks | Use package-lock files for locking down exact version numbers of every package. |
Code Splitting | Leverage code splitting technique to optimize your application. |
Updates | Stay updated with all library dependencies. |
While these practices are helpful in dealing with dependency management, do keep in mind that problems such as ‘Unable To Resolve Dependency Tree’ can still surface. When they do, reconsider the version numbers mentioned in your
package.json
file. Try the
npm ls
command to visualize the dependency tree and identify any potential conflicts(source).
Overcoming Challenges with npm and yarn dependencies
The predicaments faced when resolving an npm and yarn dependency chain with Reactjs can be intricate, especially when there’s a critical error such as “Unable To Resolve Dependency Tree”. This situation often occurs when the installed packages have conflicting versions that are unable to satisfy each other’s version requirements.
An in-depth drill-down into the problem:
1.
As
npm andyarnstruggle to find a version that all packages can work with, we encounter the "Unable To Resolve Dependency Tree" error.
2.
Since the maneuver comes out of attempting to update or add a new package, this makes it challenging to adapt your codebase to recent tools or libraries' versions.To iron out these issues:
•
One way is to use the--legacy-peer-depsflag during installation. With this approach, npm or yarn will ignore peer dependencies and avoid resolving the tree to mitigate compatibility errors. Use this command:
npm install --legacy-peer-deps•
Another optimal move would be to check for updates in the problematic packages. Regularly updated packages fix bugs, improve performance, and resolve compatibility issues with both older and newer versions of different packages. Utilizenpm outdatedor
yarn upgrade-interactiveto identify the packages that need updating.
•
Forcefully removing the problem-causing package(s) and reinstalling might also help since doing so ensures that you start afresh from a clean state. In this case, consider usingyarn remove package_nameor
npm uninstall package_namethen reinstall it.
Moreover, understanding npm and yarn intricacies, how they handle dependencies, and their constraints can streamline overcoming these challenges. As per Eric Elliott1, famous for contributing to software experiences such as Adobe Systems, Zumba Fitness, The Wall Street Journal, ESPN, and BBC, "Any app that can be written in JavaScript, will eventually be written in JavaScript." Hence, it remains imperative for developers to keenly and regularly acquaint themselves with evolving technologies and tools used in JavaScript environments.
References:
Eric Elliott Quote on Javascript's Relevance Today and In Future Development
Understanding the 'Unable To Resolve Dependency Tree' error in ReactJS is vital to maintaining an efficient and streamlined workflow in any development process. This does not merely indicate that a certain dependency required by your application or module is not present, but it underscores a more complex issue where the exact version expected by different dependencies in your codebase can lead to conflicts.Three primary reasons might be behind this issue:
1. You may have incompatible package versions in both your local environment and ReactJS project.
2. Specific versions of packages outlined in your project's package.json file might not match with those installed in your node_modules.
3. Your project's package-lock.json might have inconsistencies due to various installations and un-installations of modules.To overcome these issues, consider the following strategies:
* Regularly update all npm packages used in your project: A mismatch between the global and local versions of npm can often cause this error. Therefore, ensure your global npm registry is updated, using
npm install npm@latest -g, and then run
npm updateinside your project directory.
* Delete node_modules and package-lock.json: Sometimes, eradicating these files and reinstalling them can rectify inconsistent data errors. Remember to back up before eliminating any significant files.
* Using '--force' or '--legacy-peer-deps': The '--force' option forces npm to fetch remote resources even if a local copy exists on disk, which can aid in resolving dependency tree concerns. Alternatively, '--legacy-peer-deps' disables the automatic installing of peer dependencies; thereby, averting potential version conflicts.Remember, solving dependency tree errors require a keen understanding of your project’s unique requirements and dependencies, as well as the packages involved. It's crucial to continually track the lifecycle of your project, ensuring the compatibility of various components and their synchronicity with your codebase.
*"Programs must be written for people to read, and only incidentally for machines to execute." - Harold Abelson*