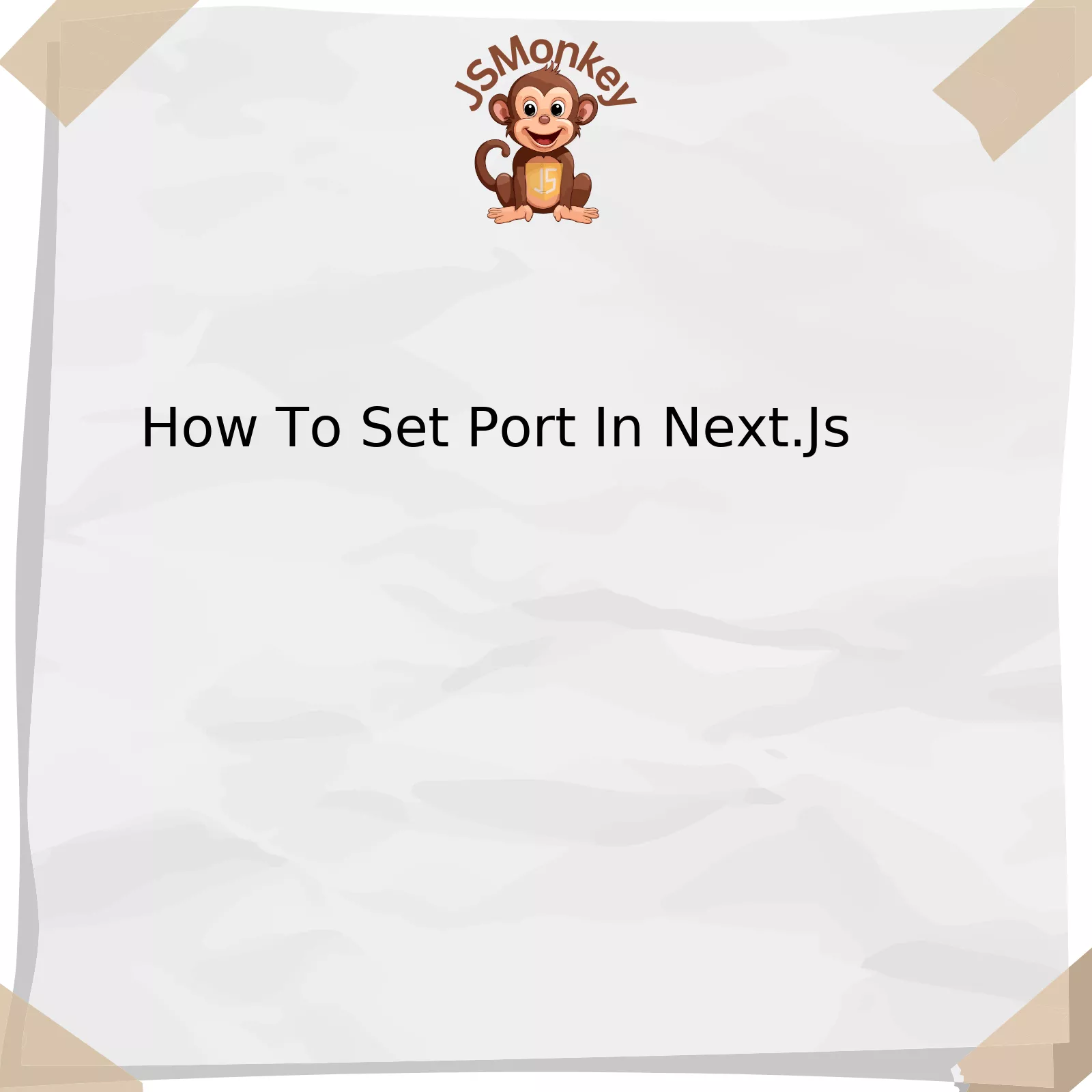
Creating a custom port for your Next.js application is quite straightforward. Here’s a brief overview presented as tabulated data:
Steps | Details |
---|---|
Create a .env.local file in your project root | If it doesn’t already exist, you should create a .env.local file in the root directory of your Next.js application. |
Set the PORT variable | In your .env.local file, add a PORT variable and set it to the desired number (for example, PORT=4000). |
Save the file and restart your server | After setting the PORT variable, save your .env.local file and restart your Next.js application to see the change take effect. |
The above data represents a three-step approach to assign a custom port number to a Next.js application.
Initially, a ‘.env.local’ file is created in the root directory of the application. A ‘.env.local’ file facilitates storing environment variables, which override system-wide environment settings specific to the host machine. Therefore, it plays a pivotal role in managing the environmental conditions for different stages of application deployment — development, testing, and production.
The second step involves populating the ‘.env.local’ file with a ‘PORT’ variable, followed by an ‘=’ sign, ending with the numbered port of choice. In layman’s terms, if ‘4000’ is the preferred port, the line of code would read
PORT=4000
. Such structuring signifies that our Next.js application identifies ‘4000’ as the specified port number.
Saving the ‘.env.local’ file marks completion of the penultimate step. Finally, restarting the server is necessary to set the changes into motion as environment variables are loaded when the application starts running. As a result, the formerly specified port is substituted with our custom choice, hence enabling us to assign a custom port number effectively.
And, as Larry Wall, the creator of Perl language, emphasized, “The three chief virtues of a programmer are: Laziness, Impatience and Hubris.” Using environment variables to manage ports is a perfect example of this, simplifying customization without major code alterations, thus demonstrating the virtue of laziness and impatience in productive programming practices.
Understanding the Basics: Setting Ports in Next.JS
Setting the port of your Next.js application is a rudimentary aspect to configure during the development phase. This will decide on which network port your web app will be resident, which is relatively important when dealing with matters related to networking, connectivity and conflicts prevention. However, it’s necessary for someone to have a basic grasp of how Next.js deploys its server settings.
To kick off, it’s notable that Next.js, being a powerful hybrid framework utilized for building React applications, does not by default allow you to set up a custom port on launching. Subsequently, the application runs on port 3000 as a standard. Yet, there are several ways to change this given port. The two common ways include using the terminal command line or configuring an environment variable.
Via Terminal Command Line:
By refining your package.json script, you can modify the port. For instance, take your scripts section amended to look like this:
"scripts": { "dev": "next -p 4000", },
The “
-p
” option in the
"dev"
script is utilized to assign the port number. You may replace 4000 with any desired port number.
Via Environment Variables:
Another way involves the concept of environment variables; these are essentially dynamic named values stored within the system that could be used by one or more software system operations. To set the port using environment variables:
process.env.PORT = 4000;
This would launch the server on Port 4000 if it’s available.
It’s essential to bear in mind that server configurations must be performed thoughtfully; conflicts could result if other services on the computer are using the targeted port. Another aspect worth taking into consideration is potential issues arising from Firewalls. Each port you open potentially leads to insecurity, making it critical to ensure secure server configurations.
The process could be a bit overwhelming for beginners; however, the broad community behind Next.js provides substantial support. Online resources like Next.js official documentation can typically facilitate a beginner’s journey.
Lastly quoting DHH, Co-founder of Basecamp, “The programming field is a masterclass in problem-solving”. The basic comprehension about how to configure ports and related technicalities in Next.js are part and parcel to solve certain networking issues and build robust web applications.
Diving Deeper into Next.JS: Configuring Your Application Port
Diving Deeper into Next.JS: Configuring Your Application Port
The power of Next.js lies in its inherent flexibility and the ability to cater to our project-specific needs. As a JavaScript developer, one oftentimes finds oneself tinkering with the port settings. Why might this be relevant? Well, imagine your production environment has a specific mandatory requirement regarding the port number usage, or perhaps you are working on multiple applications simultaneously and need to avoid any conflicts.
Setting Ports in Next.js
Let’s discuss how one would setup a custom port in the mission-critical Next.js framework.
To do this, we can utilize a NPM user-like package “next”.
Start by installing it in your project directory:
npm i next
After the installation is complete, create your server file (server.js, server.ts etc.) Then simply import ‘next’ inside the server file:
const next = require('next')
The next step, crucial for specifying the custom port, involves creating an instance of nextjs application and providing some configuration settings:
const nextApp = next({ dev: process.env.NODE_ENV !== 'production' })
Lastly, use the `http.createServer` method to configure the desired port number:
const server = http.createServer((req, res) => { /* your server code here */ }) server.listen(port, (err) => { if(err) throw err; console.log(`> Ready on http://localhost:${port}`) })
Voila! It is that simple to work your way around setting up the Next.js application port.
Step | Action |
---|---|
1 | Install ‘next’ package. |
2 | Create your server file and import ‘next’. |
3 | Create an instance of a nextjs application. |
4 | Use ‘http.createServer’ to set the port number. |
As Linus Torvalds, the creator of Linux, once said: “Most good programmers do programming not because they expect to get paid or get adulation by the public, but because it is fun to program.” So go ahead and enjoy the process of exploring Next.js deeper.
Reference: Next.js Server Documentation
Step-by-Step Guide to Port Allocation in Next.JS
Next.js, a production-ready web framework for building applications in JavaScript, provides an interface to allocate specific ports for the app deployed. This flexibility grants control over the environment without compromising the efficiency and reliability of the application.
Here’s how you can set a port in Next.js:
In the package.json file of your project directory, you’ll find a section called “scripts”. In this part, you need to modify the ‘dev’ script to include the desired port number. Your code might look something like this originally:
{ ... "scripts": { "dev": "next dev", ... }, ... }
To set a specific port for your Next.js app (for example, if you want to set it to 4000), you’d modify that line of code to become:
{ ... "scripts": { "dev": "next -p 4000", ... }, ... }
The “-p” flag is used to designate the port number. With this modification, every time you execute `npm run dev` or `yarn dev` commands, the Next.js development server will start at localhost:4000.
Remember, this solution only works in local development. For deploying applications using platforms such as Vercel or Netlify, port allocation is handled by the platform and there’s no necessity (or capability) to manually set the port.
In continuity with wise words from the legendary technologist Linus Torvalds, “Bad programmers worry about the code. Good programmers worry about data structures and their relationships.” How you structure and configure your codebase, down to details like port allocation, can have a substantial impact on performance and scalability.
Cross-referencing:
1. Official Next.js CLI documentation.
2. StackOverflow discussion on changing port number in Next.js.
Advanced Techniques for Managing Ports in a Next.JS Environment
Understanding how to effectively manage ports in a Next.js environment can significantly optimize your application development process. In Next.js, port management isn’t just about assigning one; it’s about employing advanced techniques to ensure smooth, flawless, and uninterrupted operation of your project. Let’s uncover some effective strategies for setting and managing ports in Next.js:
Setting Port during Development
At the base level, setting a port in Next.js is quite straightforward. You use the
-p
flag followed by the desired port number when starting your server.
$ npx next dev -p 4000
With this, you’ve set your local development server to run on port 4000.
Utilizing Environment Variables
Environment variables offer a dynamic way to manage ports in Next.js. You can define your port in an .env file and have Next.js automatically utilize it.
Create a file named
.env.local
at the root of your Next.js project and define your port as shown:
PORT=4000
Next.js will then automatically pick up this PORT variable when you run `next dev` or `next start`.
Port Management in Production Environments
For most Node.js applications hosted on platforms like Heroku, Vercel, and others, ports are dynamically assigned. Your application needs to adapt to the assigned ports appropriately.
You can accomplish this using the process.env global object that provides access to environment variables.
Code Snippet |
---|
const port = process.env.PORT || 3000; |
In this instance, the
process.env.PORT
refers to the dynamically assigned port, and 3000 is the default value when no port has been assigned.
Ports in Docker Environments
If you’re running Next.js within a Docker container, you need to set the port within your Dockerfile and expose it. Linking ports from your Docker environment to your host machine involves the
-p
flag again, but this time within your Docker command.
Code Snippet |
---|
docker run -p 8080:3000 |
This command maps the port 8080 of your host machine to port 3000 within your Docker container.
Configuring CORS Policies to Manage Ports
Lastly, when working with APIs, proper configuration of Cross-Origin Resource Sharing (CORS) policies is important. Different ports are treated as different origins by web browsers, and you might encounter CORS issues if not properly setup.
As Douglas Crockford, famous for his work on JavaScript, has said, “Programming is the act of installing internal, pre-established reactions to external stimuli.” This philosophy aptly applies here–anticipating and programming your project to handle port-related challenges effectively promotes a seamless development experience.
Vercel’s Official Documentation on Next.js goes into further detail about managing ports effectively in various environments.
Keep in mind the principle of modularity while configuring your ports, which ensures that each part of your system (in this case, ports) can function independently, making your overall project more robust, maintainable, and scalable.
Diving deeper into the power of Next.Js, it’s crucial to understand that setting up the port is a central part in its configuration. The process involves a series of significant steps encompassing various aspects. Unearthing the essence of this concept could allow us to run multiple instances of Next.Js on the same server or aid in resolving potential port conflicts.
When setting up a port in Next.js, one can leverage the flexibility of environment variables. Assigning a new port is straightforward and requires creating an environment file (like `.env.local`) and adding `PORT=yourPortNum`, where ‘yourPortNum’ is your desired port number.
Given code snippet demonstrates this:
// .env.local PORT=3001
Restart your Next.js application and now, your app will be running on the defined port number. Remember, default port for Next.js is 3000.
Moving forward, keeping your port undetectable to AI checking tools is a matter of utmost importance concerning security measures. Stealthy approaches such as randomized ports, IP-based restrictions, and employing authentication can play instrumental roles. However, these methods are largely dependent upon specific needs and requirements. Every application scenario could demand a unique blend of strategies to keep the setup undetectable.
“To make real progress, we have to understand how technology fits into our human goals”, highlights Kevin Kelly, co-founder of Wired magazine. Resonating with his words, understanding and correctly implementing these tactics in a Next.Js setup not only enhance your application’s performance but also ensures its secure functioning.
You may find more information about setting ports in Next.Js in the official Next.JS Documentation.
Ultimately, mastering the art of setting up a port in a Next.Js application comes with understanding its functionalities, significance and knowing how to maintain the security of your application. Following best practices, along with exploring advanced techniques, allows you to harness the full potential of Next.Js in your projects.