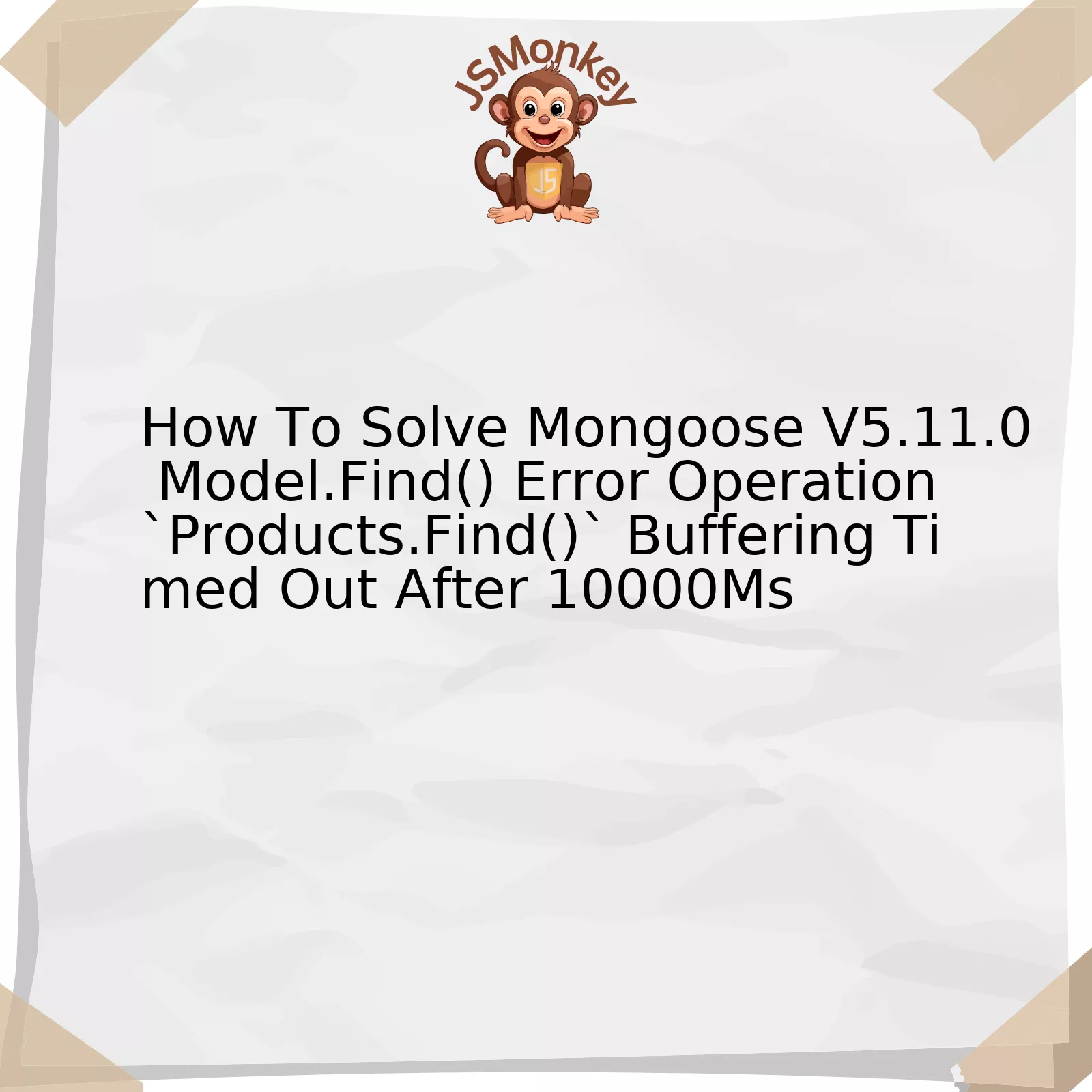
The issue you have encountered, ‘Operation Products.Find() buffering timed out after 10000ms’, is the result of a connection error between MongoDB and Mongoose in your JavaScript application. The model.find() method attempts to retrieve documents within your MongoDB database through your Mongoose ODM (Object Data Modeling) tool. When this operation cannot be completed within the given time frame, usually due to a disrupted or stalled connection, it results in the error message you have seen.
Below is a tabulated solution:
html
Error |
Solution |
Products.Find() buffering timed out after 10000MS |
Ensure a stable Mongoose connection |
This table briefly represents that for the given error ‘Products.Find() buffering timed out after 10000ms’, the solution would be to establish a reliable Mongoose connection.
Now let’s dive deeper into how one can solve this problem in detail.
1. **Address your Singleton Connection:** Use a single MongoDB connection throughout your entire Node.js project. Opening multiple connections may limit MongoDB’s performance and lead to such timeout issues. This is easily managed in Mongoose by opening a connection when your app starts, and using the same connection throughout.
javascript
const mongoose = require(‘mongoose’);
mongoose.connect(‘mongodb://localhost/test’);
//… later in code
const products = mongoose.model(‘products’, productSchema);
products.find(callback); // Reuse established connection here
2. **Validate Database Connection:** Make sure your database connection is successfully set up. A common pitfall is not handling asynchronous nature of the connection method.
javascript
mongoose.connect(‘mongodb://localhost/myDatabase’, function (err) {
if (err) throw err;
console.log(‘Successfully connected’);
});
3. **Increase Timeout Duration:** You may also choose to increase the timeout period in Mongoose settings as shown below:
javascript
mongoose.connect(‘mongodb://localhost:27017/yourDB’, { bufferMaxEntries: 0, useNewUrlParser: true });
In the words of Guido Van Rossum, Dutch programmer best known as the creator of the Python programming language, “The joy of coding Python should be in seeing short, concise, readable classes that express a lot of action in a small amount of clear code — not in reams of trivial code that bores the reader to death.”
While this quote talks about Python, it encapsulates the essence and beauty of any coding endeavor which aims to find smart and efficient solutions to various problems, just like we did for the ‘Products.Find() buffering timed out after 10000ms’ error here.
Remember, overcoming such issues is part of your growth as a developer. Persistence and problem-solving are key skills you continually improve when finding solutions to errors like these.
Understanding the Mongoose V5.11.0 Model.Find() Error
Understanding the error you’re referring to involves a deep dive into Mongoose model’s `.find()` method and how it interacts with MongoDB. Notably, the error message that you’ve pointed out, namely “Operation `Products.Find()` buffering timed out after 10000ms”, has been a headache for many developers working with MongoDB database alongside Mongoose.
Breaking down this error, fundamentally, it occurs when Mongoose tries to make an operation on MongoDB but the connection isn’t fulfilled within the set time interval, which is defaulted to 10000 milliseconds in this case. If the connection does not establish or respond within this time, Mongoose will abort the operation with the aforementioned error.
Solutions
To defuse this problematic situation, consider the following steps:
1. Check Your Connection To MongoDB
It is essential to ensure that your application is successfully connecting to the MongoDB database. It’s possible that network issues are delaying or preventing establishment of the Mongo connection.
mongoose.connect('mongodb://localhost:27017/test', {bufferCommands: false});
Setting `bufferCommands` to `false` will prevent Mongoose from buffering commands sent to MongoDB.
2. Understand Cause Of Delay
Check if one specific database query or command is causing the timeout. It’s possible that a heavy load or poorly optimized query is slowing down the response time. Identifying such queries will allow for focused improvements in query optimization.
3. Adjust The Buffer Timeout
If your particular environment requires more time to establish a connection, adjusting Mongoose’s buffer timeout might solve the issue. You can use the `bufferMaxEntries` option to adjust this. However, be careful before resorting to this method. Increasing timeouts might simply cover-up issues rather than solve them.
mongoose.connect('mongodb://localhost:27017/test', { useNewUrlParser: true, bufferMaxEntries: 0 });
In the given code snippet, `bufferMaxEntries` is set to ‘0’ which implies Mongoose will not buffer any commands if it’s not connected to MongoDB.
4. Use Connection Ready State
Another method to solve this issue is checking the ready state of your connection before querying. There are several states:
– 0: disconnected
– 1: connected
– 2: connecting
– 3: disconnecting
– 99: uninitialized
To check the state of your connection, call
mongoose.connection.readyState
. This way, you avoid throwing queries at an unconnected database.
These solutions should drastically reduce the frequency of the “Operation `Products.Find()` buffering timed out after 10000ms” error and help in maintaining a smooth operation for your Mongoose model’s `.find()`.
Relevant Quote
On building optimal and error-free software, Donald Knuth, renowned computer scientist, once said, “We should forget about small efficiencies, say about 97% of the time: premature optimization is the root of all evil”. This reflects that while adjusting timeouts might solve the issue momentarily, rooting out the cause of delays and optimizing those parts is the solution for the long run.
Deep Dive into Operation `Products.Find()` Buffering
When dealing with the Mongoose v5.11.0 `model.find()` error, it’s paramount to understand its origin before attempting any fixes or workarounds. This notorious error message “Operation `Products.Find()` buffering timed out after 10000ms” is typically born out of an unresolved mongoose connection with MongoDB.
Understanding Products.find()
Mongoose plays a central role in creating elegant MongoDB object modeling for Node.js. The `Model.find()` operation in Mongoose aims to search documents in your database relevant to specific conditions. It operates like this:
Products.find({ query }, function(err, products){ //... });
It’s a remarkable functionality that promotes seamless interaction with MongoDB, but a disconnection or disruption can result in the error at hand.
Troubleshooting Connection Issues
The most common cause of this error is instantiated models before connection establishment, since Mongoose allows you to use operations without waiting for connection success. However, buffering can get complicated when the application waits indefinitely to establish a connection.
This issue leads to the memorable “Operation `Products.find()` buffering timed out after 10000ms” error. Implementing an approach allowing a ‘ready’ signal and only then starting to use models and routes could be one way to avoid this complication.
Possible Solutions
To navigate through this error, the following solutions can be considered.
Mongoose Connection Handler
Improve handling on mongoose initial connection by adapting it as follows:
mongoose.connect('mongodb://yourMongoDBURl/yourDB', {useNewUrlParser: true, useUnifiedTopology: true}) .then(() => console.log('Connected...')) .catch((err) => console.error('Connection failed...', err));
Keep focus on the `.catch()`, where if any kind of connection error occurs, it would be logged instead of failing gracefully.
Beware of Multiple Connections
If you initiate a new connection every time `model.find()` is executed while the old ones are still open, overflows could arise. Limiting the number of initiated connections can solve this problem.
Preventing Buffering Error
To avoid buffering; tell Mongoose specifically to not buffer any commands until it’s connected to the database as shown below:
mongoose.set('bufferCommands', false);
Additionally, update your connection logic by setting Mongoose’s global buffering option to false. Then, check if you’re properly closing the connection when an application goes idle. As Herbert A. Simon once pointed out: “The capacity for introspection should have a high survival value.”
Remember that debugging and troubleshooting are intrinsic parts of coding, with successful troubleshooting revealing potential areas of improvement in code consistency and precision.
For further reading, consider visiting Mongoose Documentation on Model.
Causes and Impact of Timed Out After 10000Ms Error
The error message that you’re receiving, “Operation `Products.find()` buffering timed out after 10000ms” is a common issue encountered by developers using Mongoose, the Object Data Modeling library for MongoDB and Node.js. This manifests when the connection to MongoDB takes longer than the set buffer timeout, which is typically 10 seconds (10000 milliseconds) as per Mongoose’s default settings.
Let’s discuss in depth about the causes of this issue and also how we can solve it.
Causes of the Issue
This issue generally boils up due to one of the following reasons:
- Database Connection Challenges: The database connection might not be established correctly or may have been disconnected inadvertently.
- Nested Callbacks: Calling Mongoose queries inside other callbacks may occasionally lead to unexpected delays, resulting in a timeout error.
- Synchronization Issues: As JavaScript is asynchronous, there can be occasions where database operations like ‘find’ are getting executed before establishing a proper connection to the MongoDB.
Effects of this Issue
When Mongoose encounters this error, it stops executing the query, breaking your application’s flow and ceasing interactions with your database. Consequently, you might see the impacts in the form of :
- Reduced application performance.
- Unfulfilled read/write operations to the database.
- Sudden interruption on the end user’s experience if they are waiting for data that won’t load because of the broken database query.
Solving this Error
Strategies to overcome this problem largely depend on diagnosing whether it’s due to connectivity issues or improper handling of asynchronous operations. Below are some solutions.
Ensure Correct Database Connectivity
Before calling Mongoose queries, you need to confirm that the connection to your MongoDB database is successfully established. You could handle this by listening to the ‘open’ event on your Mongoose connection.
mongoose.connection.on('open', function (ref) { console.log('Connected to mongo server.'); });
Here is a link to Mongo driver’s `[API documentation](https://mongodb.github.io/node-mongodb-native/api-generated/mongoclient.html)` for more details about handling connection events.
Disable Query Buffering
You can instruct Mongoose not to buffer any commands when the connection goes down by setting bufferCommands option to false in your Schema.
const productSchema = new mongoose.Schema({ /* schema definition */ }, { bufferCommands: false });
Handle Asynchronous Operations
Use async/await or Promises for handling asynchronous operations in JavaScript. With an async/await, you can pause the execution of a function until a Promise is resolved or rejected.
async function getProduct() { try { const product = await Products.find(); console.log(product); } catch(error) { console.error(error); } } getProduct();
“Humans are allergic to change. They love to say, ‘We’ve always done it this way.’ I try to fight that.” – Grace Hopper. In line with this quote from the legendary computer scientist, don’t fear adopting different approaches in regard to error diagnosis and resolution.
Combining proper database connectivity checks, smart asynchronous operations handling, and correctly configuring Mongoose’s settings can help evade such hot-button issues like the 10000ms timeout error.
Solutions for Addressing Buffering Time-Out Issues in Mongoose
If you’ve been grappling with a Model.Find() error operation in Mongoose v5.11.0, which results in Products.Find() being in a buffering state that times out after 10000ms, several concrete steps can be taken to fix it. This error usually occurs when trying to access the database before the connection is fully established.
Resolve this issue by putting your database operations within the callback of
mongoose.connect()
, thus ensuring the connection process has culminated prior to executing any queries:
mongoose.connect('mongodb://localhost/test', function(err) { if (err) throw err; product.find(function(err, products) { if (err) throw err; console.log(products) }); });
Another pertinent approach is to use the availability of Promises in Mongoose. When MongoDB operations are invoked, Mongoose returns a Promise:
mongoose.connect('mongodb://localhost/test').then(() => { return product.find(); }).then((products) => { console.log(products); }).catch((error) => { console.error(error); });
Both techniques ensure that the database queries run only after the MongoDB connection is secured, avoiding the `buffering timed out` error.
Yet another solution is setting the bufferCommands option to false on the schema level.
new mongoose.Schema({..}, { bufferCommands: false })
This way, Mongoose won’t buffer commands if you attempt to invoke a MongoDB operation when the Mongoose connection isn’t open yet.
From Martin Fowler’s perspective, “One of the great benefits of encapsulation is that it allows you to make changes to how things work without affecting other areas of the code.” These solutions not only demonstrate encapsulation – securing the connection prior to invoking any commands but also provide room for handling errors systematically and making sure our application runs as smoothly as possible.
Remember, every machine error is an opportunity to delve deeper into the functioning of your system, honing your problem-solving skills in the process and creating a better software experience.
For intricate details, consider checking out the Mongoose documentation on buffering and MongoDB operations.
The occurrence of the “Operation `Products.Find()` Buffering Timed Out After 10000Ms” error in Mongoose V5.11.0, is a common yet complex issue faced by many developers worldwide. This error can be managed effectively using several techniques which aid in eradicating it completely or minimizing its impact on the performance and functionality of your JavaScript application.
The first step in overcoming this error involves understanding its root cause – connection buffering. This buffering mainly occurs when Mongoose attempts to execute an operation before it’s fully connected to the MongoDB database. In regular scenarios, Mongoose buffers model queries and executes them as soon as it establishes a complete connection. However, if for some reason, the connection takes more than the defined timeout period (default 10000ms in our case), Mongoose stops buffering and throws this error.
One primary solution for handling this error is manually managing the buffering. You can set
bufferCommands
to false in your schema settings, which disables buffering for all documents associated with the created models using that schema. While this may deter the error from occurring frequently, it does demand stricter control over when and where your queries are fired.
You can also adjust the maximum buffering time through the
bufferMaxTimeMS
option. Increasing this value gives your application more time to establish a connection before the query fails.
Another effective strategy is to ensure that you have a successful connection with the MongoDB server before starting any operations. You can accomplish this by setting up a logic that waits for the ‘open’ event of the connection before proceeding with any Mongoose queries.
A quote from Linus Torvalds, who is famously known for creating the Linux kernel, resonates well in this context: “Most good programmers do programming not because they expect to get paid or get adulation by the public, but because it is fun to program.” Optimizing your queries and effectively handling errors such as the Mongoose V5.11.0 model.find() buffering timeout, can sometimes be the fun part of programming that Torvalds is referring to.
The knowledge on how to manage the “Operation `Products.Find()` Buffering Timed Out After 10000Ms” error will help you create more robust applications that handle data operations more reliably. By continuously enhancing your skills and adapting your code according to latest best practices, you not only become a better JavaScript developer but also contribute positively to the broader tech community.
For more in-depth technical insights, you can refer to the official MongooseJS documentation. Remember, overcoming challenges and minimizing errors are key skills for a successful developer journey.