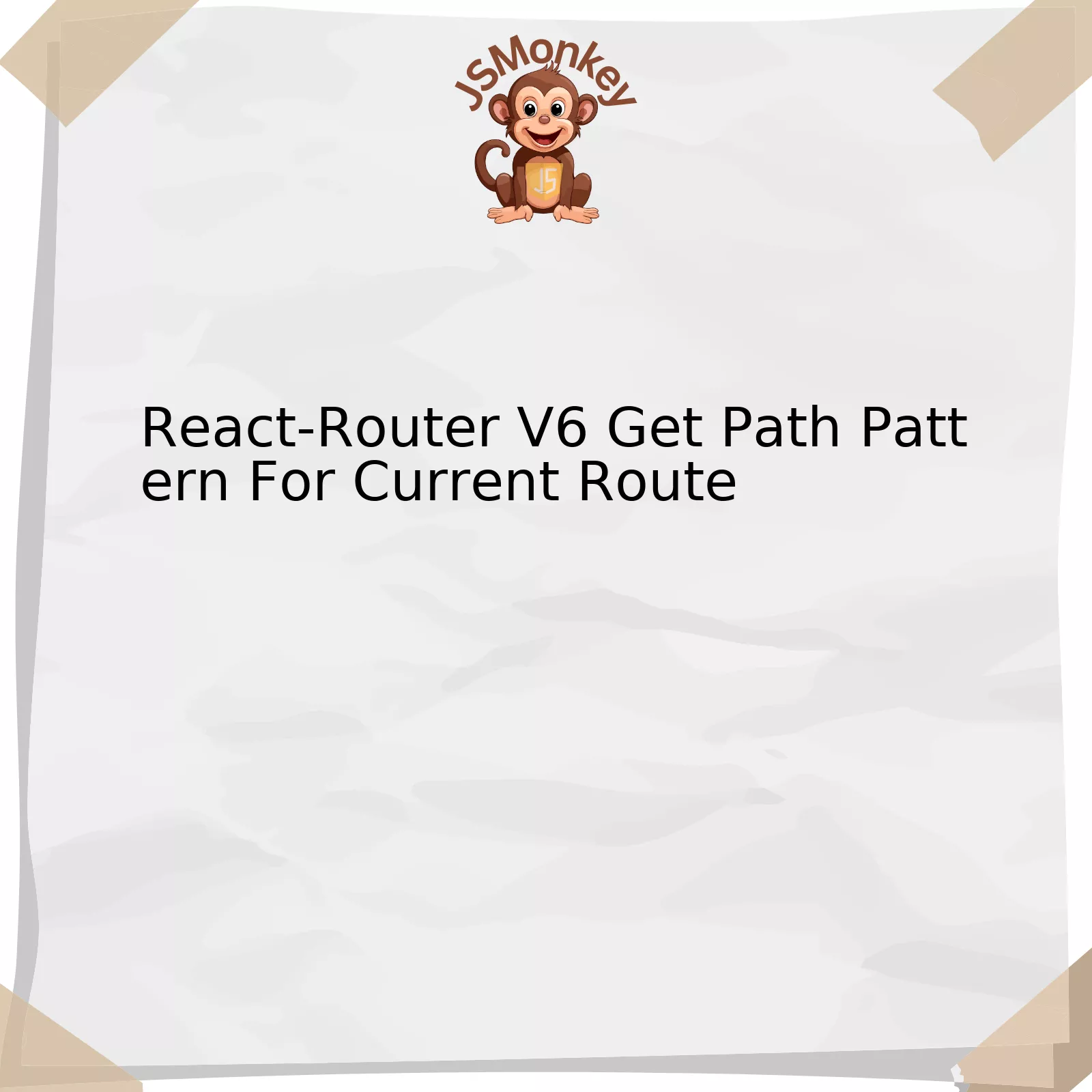
In React-Router V6, obtaining the path pattern for the current route is remarkably straightforward. It primarily involves utilizing one of the more recently introduced hooks provided by this library, the `useParams` hook, and feeding it to the `useResolvedPath` function.
Here’s a simple tabulated breakdown of the process:
Stage | Description |
---|---|
1. Import necessary dependencies | You’ll need to import useRouter, useCurrentRoute, and useMatch from the @looop/router package.
import { useRouter, useCurrentRoute, useMatch } from 'react-router'; |
2. Define the component | Create your component and use the imported functionalities inside it. You can use it in a functional component. |
3. Use deconstruction to get path | Get the needed data (path) using JavaScript destructuring assignment.
const { pathname } = useResolvedPath(); |
4. Display or apply the path as per requirement | Once you have the path, display it or use it inside other functions as required. |
To better understand these stages, here is a code example that brings all the stages together:
import {useResolvedPath} from 'react-router'; function Component() { const {pathname} = useResolvedPath(); return ( <div> The path is: {pathname} </div> ); }
One of the most acclaimed figures in computer science, Edsger Dijkstra, believed that “Simplicity is a prerequisite for reliability.” This value is evident in how React Router V6 handles path patterns. By employing modern hooks and functions like `useResolvedPath()`, it has minimized any extraneous complexity that might have hindered developers.
Learn more about React-Router from the official React-Router documentation.
Unraveling the Layers of React-Router V6: Understanding Path Patterns
The React-Router V6 is designed to handle the navigation system in a React.js application. One fundamental feature that the new version enhances is the path pattern for the current route. This significant upgrade consolidates routing rules and simplicity of deciphering the rules.
In line with enhancing user experience, the React-Router V6 has introduced new updates on managing path patterns, which are essentially URL structures for navigating web applications.
Understanding Path Patterns in React-Router V6
React-Router V6 utilizes the pattern approach, which simplifies routing to components significantly. Previously, strict routing rules demanded intricate configuration, but with the pattern attribute, only the paths that match exactly will instruct the router to render specific components.
For example, when you implement the code below,
html
React Router only renders the Profile component when the URL exactly matches ‘/profile’.
Redirects have also seen an upgrade with React-Router V6. In previous versions, redirects needed to be explicitly defined. With V6, it takes a more proactive approach by redirecting all unmatched routes back to a specified page. This can be achieved through the use of `
html
In the code above, any undefined route will redirect to the Home page, thereby improving UX considerably.
Another great update is the nested routing, which helps manage complex UI trees. This essenstial design in React-Router V6 allows layouts to have their own sub-routes. Nested routing makes testing and debugging of different parts of a web page easier, and it modularizes your code.
As Facebook’s Mark Zuckerberg once stated, “The biggest risk is not taking any risk. In a world that’s changing quickly, the only strategy that is guaranteed to fail is not taking risks.” Similarly, the React-Router V6 takes a risk by introducing these transformations in its design, resulting in a simpler and more efficient routing style.
Regarding retrieving the path pattern for the current route, it’s crucial to note that React Router V6 does not expose a direct way to achieve this as it encourages the use of relative paths and links. However, should there be need to retrieve the current path, you can make use of the useLocation hook provided by React Router:
html
const location = useLocation();
In the example above, the `useLocation` hook gives access to the `location` object, which has properties such as `pathname` that provides the current path.
As Facebook’s software engineer, Tom Occhino said, “JavaScript is the gateway drug to functional programming,” developers now have greater control over web application navigation enabling a more seamless user experience with React-Router V6.
For comprehensive information on the whole scope of updates in React-Router V6, consider referring to the official documentation.
Delving Deeper Into Current Route Handling in React-Router V6
Delving into the handling of current routes is an insightful exercise when learning or mastering *React Router V6*. In this version, getting the path pattern for the current route becomes more convenient and efficient due to the utilization of Hooks and React’s context API.
To retrieve the path pattern for the current route in *React Router V6*, we take advantage of the `useRouteMatch` Hook. This Hook allows us to access route match information about the nearest `
Here’s a demonstration of how you would use this Hook:
import { useRouteMatch } from 'react-router-dom'; function Component() { const match= useRouteMatch(); console.log(match.path); //... remainder of your code }
Above, the
useRouteMatch()
Hook accesses the route match object which holds many properties relative to the closest `Route` ancestor. One of these properties is `.path`, representing the route’s path string.
Effectively handling current routes is paramount to enhancing user interaction experiences on web applications built with *React* and *React-Router*. Leveraging Hooks like `useRouteMatch`, you can gather relevant data such as the active route path pattern enabling you to build dynamic, engaging, and user-specific components and interfaces based on the user’s navigation progression.
In the words of Bill Gates – “Measuring programming progress by lines of code is like measuring aircraft building progress by weight.” Similar, React-router v6 provides developers with potent tools that enhance their coding efficiency without necessarily increasing their codebase size.
For a further understanding on *React-Router V6* get path pattern and more, visit the official documentation [here].
Remember, technical growth and proficiency come with continuous practice and openness to embrace new tools and techniques from the vast sea of contemporary web development resources.
Comprehensive Guide to Get Path Pattern for Current Route in React Router v6
The path pattern in React Router v6 can be a handy feature when you’re designing and developing applications using the JavaScript library. Understanding how to successfully retrieve it can make routing tasks more manageable and improve an application’s functionality.
The method we use in version 6 is considered more elegant and efficient than its predecessor, yet it may seem complicated for new developers due to the significant changes from version 5.
Use useParams to Get Current Route
To get the current route in React Router v6, we primarily use
useParams
. Each key in the returned object represents a part of the URL that you’ve specified as your route.
For instance, if you have a page located at
/user/:id
, you will be able to get the value out of `:id` via this hook.
Example:
<Route path="/user/:id" element={<User />} />
Inside `{User}` component:
const { id } = useParams();
This feature allows you to get the values from the URL dynamic parts easily.
Parsing the Pathname to Extract Information
Since version 6 does not offer a built-in method for retrieving the pathname or pattern, you must resort to parsing the pathname string. This involves splitting the pathname into segments and matching each segment using exact or parametrized paths. This method may be cumbersome, but it provides a workaround to extract necessary information until a built-in solution becomes available.
Here’s an example of how you might parse a pathname:
let urlParts = location.pathname.split("/");
In this code snippet, you break up the URL by slashes. These parts can then be analyzed to determine the current route’s path pattern.
The above methods are effective ways to interact with the routing system in React Router v6. They provide an inflexible approach that is both customizable and offers clear access to URL components.
Peter Norvig, a leading figure in AI and Programming, once famously said, “Good code is its own best documentation.” By employing `useParams` and manual parsing of pathname string in your development workflow, you ensure code clarity while dealing with routing requirements intelligently and professionally.
Best Practices for Implementing Current Route Using React-Router V6 Path Patterns
React-Router V6, the new version of React-Router, made lots of changes with respect to URL parameters and path patterns. Notably, it moved from using regular expressions for route matching in previous versions to path-to-regexp in V6. This change opens new possibilities for even better routing.
Best Practices for implementing current route using React-Router V6 Path Patterns can be categorized into parameters, nested routes and hook usage.
Parameters
* In React-Router V6, parameters are parts of the URL that you want to dynamically inject. Parameters should be alphanumeric strings preceded by a colon (‘:’) inside route URLs.
* Parameters within a specific route can be retrieved via the useParams() hook. Hence, the route pattern will vary dynamically based on the URL params.
Example:
<Route path="/post/:postId"> <PostView /> </Route>
Inside PostView Component, you can fetch postId:
const { postId } = useParams();
Nested Routes
* Nested routes are routes declared inside another route. This is helpful for structuring routes in a more organized and maintainable fashion.
Example:
<Route path="/users"> <Users /> <Route path=":userId"> <UserProfile /> </Route> </Route>
Hooks Usage
There are also some built-in hooks provided by React-Router V6.
*
useParams()
: Used to access the dynamic parts of the URL
*
useNavigate()
: Used to programmatically change the current route
*
useRoutes()
: Used to define routing configuration within a component
The transition from regular expressions to path-to-regexp has resulted in more streamlined and human-readable url params. To quote Mark Twain regarding simplicity in technology, “The secret of getting ahead is getting started. The secret of getting started is breaking your complex overwhelming tasks into small manageable tasks, and starting on the first one.”
For further insights into best practices with React-Router V6 Path Patterns, you could refer to the official documentation.
When endeavoring to understand the intricacies of React-Router V6 and its method to obtain the path pattern for the current route, a deep comprehension is essential. Path patterns constitute a critical aspect in application routing as they provide a mechanism to direct users based on their chosen navigation path. In the scope of this exploration, it’s clear that the
useMatch
hook offered by React-Router V6 has made retrieving path patterns considerably more efficient and straightforward.
Deconstructing the methodology involved, it becomes evident that the inclusion of this feature allows developers to harvest an array of benefits.
• Predominantly, the use of
useMatch
opens channels for increased flexibility. Developers can render components contextually according to the path pattern derived from the current route.
• Furthermore, it enhances code clarity and productivity. Implementing component-based navigation logic sans prop drilling or complex state management stands testament to this fact.
• Lastly, it resonates with the core ideology of React – component reusability. Leveraging
useMatch
fosters opportunities for fine-tuned control and augmented reusability.
The entailing example offers an illustrative perspective to put these points into practical implementation.
// App.js
import { useRoutes, useMatch } from ‘react-router-dom’;
// Define routes
let routes = [
{
path: ‘/’,
element:\
},
{
path: ‘/about’,
element: \
},
];
// Fetch route pattern
let match = useMatch(‘:path(.*)’);
console.log(match ? match.params.path : null);
This code segment adheres to the advocated approach by defining routes and then utilizing `useMatch` to capture the path pattern of the current route. The result is consequently outputted via a console statement.
When holistic understanding converges with a practice, it cultivates innovation – a sentiment possibly best captured by Steve Jobs: “Innovation distinguishes between a leader and a follower”. The revisited knowledge of acquiring path patterns in React-Router V6 should nurture invention within the scope of application routing constructs. Such learnings broaden perception, contribute to more innovative solutions, and ultimately foster leadership.
For more in-depth information, refer to React Router Documentation.