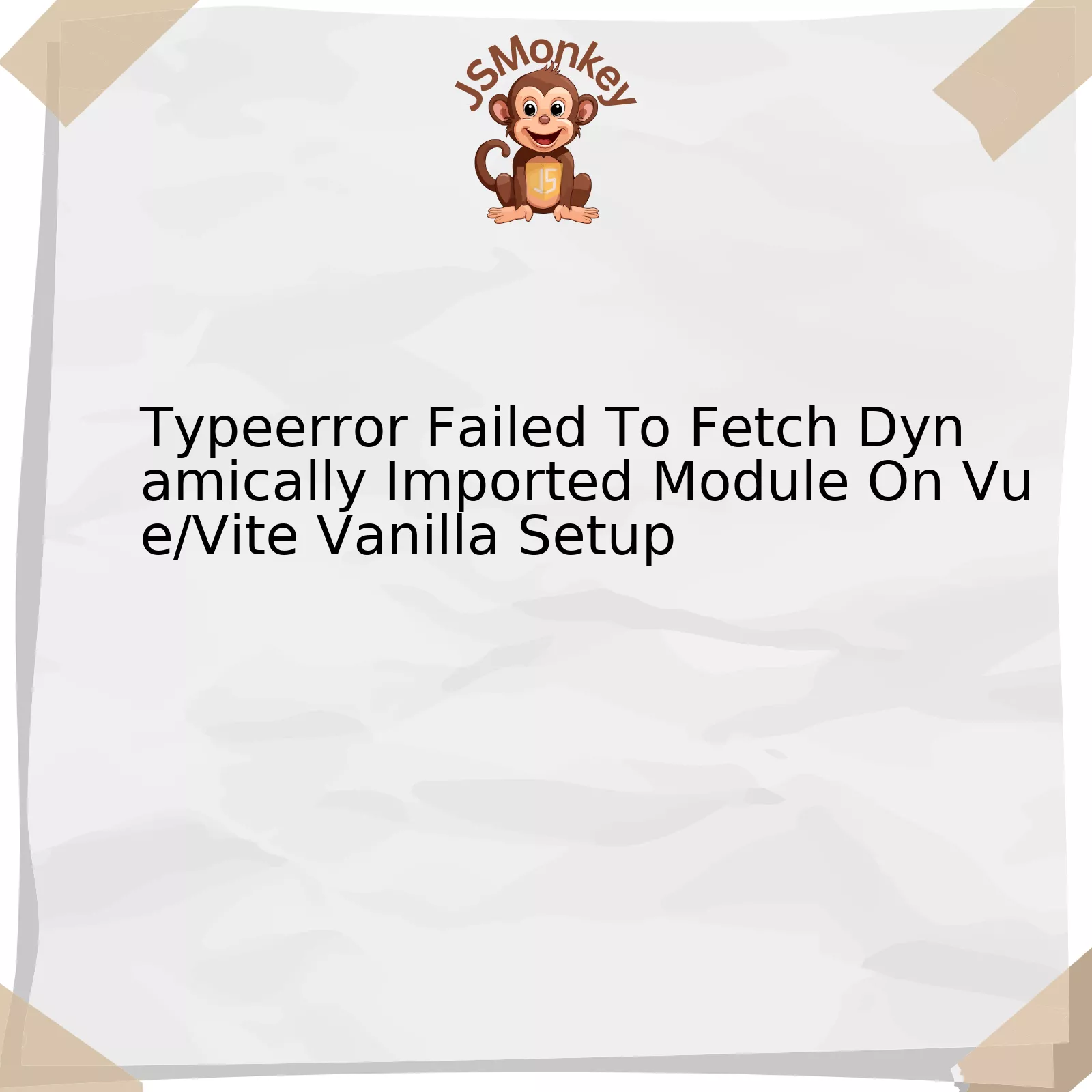
There is often a requirement to delve into the details pertaining to the TypeError Failed to Fetch dynamically imported module in Vue/Vite Vanilla Setup. A comprehensive way to understand this error is through the following visual representation:
Error | Possible Cause | Solution |
---|---|---|
TypeError: Failed to fetch dynamically imported module |
Issues related to module loading, which can occur due to incorrect paths, incompatible modules or network connectivity. | Check for the correct paths, compatible versions of modules and browser’s network tab for any failed requests. |
Upon examining the above information, it becomes apparent that the main root cause lies in issues with module loading. When you encounter this type of error (`TypeError: Failed to fetch dynamically imported module`) in a Vue/Vite setup, optimizing the setup of your dynamic imports could be the key to finding a resolution. One potential issue might be incorrect paths set for the modules, essentially meaning that the system can’t locate them. Here, meticulous checking would need to be done to ensure accurate path designations.
Another potential problem could be incompatibility between certain modules. This keeps them from effectively communicating and functioning together which ultimately leads to this failure. It’s crucial to check and verify that all your dependencies are up-to-date and well-matched.
Lastly, an overlooked aspect that can cause this error is problems with network connectivity. This largely applies to cases where your resources like scripts or modules are loaded over the internet, and network issues may prevent these resources from being fetched properly. You can troubleshoot this by checking your browser’s network tab to spot any failed requests.
A key factor in overcoming challenges like these is diligent debugging and an in-depth understanding of your setup. As Linus Torvalds, the creator of Linux, says, “The only way to do good work is to love what you do”.
Remember, most errors can be resolved via thorough inspection of your coding environment and constant learning.
Debugging TypeError in Vue/Vite Vanilla Setup
One of the most common problems with JavaScript programming involves TypeError issues in frameworks like Vue.js and bundlers like Vite. A specific instance that we are going to tackle is the “TypeError: Failed to fetch dynamically imported module” issue on a Vue/Vite vanilla setup. Debugging this error can greatly enhance both your application’s stability and your skills as a developer.
Understand this: At its roots, this problem typically occurs when Vue attempts to import a module dynamically, and due to any of a variety of factors, fails to do so successfully. The fundamental mechanism behind dynamic imports in JavaScript consists of splitting the code into different chunks, which are loaded only if and when required.
Complications may emerge from multiple sources:
– Historical server configurations
– Problems with correct paths
– File naming issues
When you notice this error message while working with Vue/Vite, it is a good exercise to verify some of these aspects:
1. Evaluate Your Server Configuration
The production server needs to send the appropriate header for the JavaScript files, e.g., `Content-Type: Text/Javascript`. Any discrepancy here might lead to this TypeError. Refer to MDN Web Docs for detailed information about HTTP response headers.
2. Analyze Import Syntax
Ensure that the syntax you use for dynamic imports is correct. It should look something like this:
import(`@/components/${componentName}.vue`);
Dotted in the import path could result in issues.
3. The Case of the Nomenclature
Casing and naming conventions in file names are crucial. Make sure all the naming rules align properly across your application.
Bill Gates once famously said, “Measuring programming progress by lines of code is like measuring aircraft building progress by weight”. Hence, learning to debug errors like this ‘TypeError: Failed to fetch dynamically imported module’ is essential. By understanding how these modules are loaded and how server configurations impact these processes, you can make your Vue/Vite applications more reliable and investor-friendly.
However, remember that every application is unique, and what works for one might not be suitable for another. The insights provided here should give you the fundamental concepts to start debugging your setup – take them, expand upon them where necessary, and hone your programming skills.
Understanding “Failed to Fetch Dynamically Imported Module” Error
When working with Vue/Vite vanilla setup in JavaScript, an error message like “TypeError: Failed to fetch dynamically imported module” could become a stumbling block. This issue typically emanates from unsuccessful attempts at importing JavaScript modules dynamically.
import()
, a function-like form enables you to load JavaScript modules akin to the static
import
statement but more robust and flexible. Inconsistencies or issues with this syntax cause your application to return the error in question.
Firstly, understanding what triggers this error provides insight into potential areas to focus on when rectifying it:
– **Improper Server Setup**: If modules are not correctly served from your server, then browsers can’t resolve them leading to this error. Considering that Vite, used primarily for developing via Vue 3, launches a development server automatically, misconfiguration or interruption of server processes might be a source of your frustration.
– **Wrong Module Path**: Developers often overlook the possibility of errors in paths referencing modules for import. The difference between relative (./module.js) and absolute (/module.js) paths plays a role in how they’re resolved by the browser.
– **Network Issues**: Networking barriers such as CORS policies could prevent Fetch API from successfully fetching the requested module. Bear in mind that Fetch API powers dynamic imports in JavaScript.
– **Cache Problems**: Modules cached incorrectly by either the browser or the service worker could lead to faulty fetches.
So how does one tackle these issues? Below are possible fixes tailored to the sources highlighted above.
– **Server Settings Examination**: To rectify improper server setup, experiment by manually launching your server or moving your project to another local/remote server environment. Reviewing Vite’s configuration settings might also shed light on problematic areas.
– **Verify Module Paths**: For wrong module paths, pay attention to the exact location of the files you wish to dynamically import. Crosscheck these with where your system is actually looking for them.
– **Check Network Policies and Settings**: As for network issues, confirm that you do not have a CORS policy preventing fetch operations from running as intended. Browser console logs might hint at this being the problem.
– **Clear Application Cache**: Debug cache problems by clearing your browser cache or deregistering service workers causing these mishaps.
Between the time I write this and when you take action based on these suggestions, there are chances that specifics of Vue/Vite setup may change. Hence, constantly checking Vite’s official documentation will ensure you are adopting the best practices as recommended by the creators themselves.
Leaning on wisdom in the field, I would like to bring forward a quote by Grace Hopper, one of the pioneers in the field of software development,
“The most dangerous phrase in the language is, ‘We’ve always done it this way.'”. This perspective could be enlightening when troubleshooting issues – never discard the potential solution just because it downplays conventional ways.
Solutions for Addressing TypeError in Vue/Vite Environment
Addressing the TypeError in a Vue/Vite environment, particularly one involving the failure to fetch a dynamically imported module on a Vue/Vite Vanilla Setup, can be met by adopting specific solutions. This problem could stem from circular dependencies issues, faulty import syntax, or due to some network problems. It is critical not to lose sight of the intricacies and technical nuances inherent in debugging such issues.
In the context of Vue.js, the issue: ‘TypeError Failed To Fetch Dynamically Imported Module’ might arise if there’s an error in the dynamic import statement or when the Vue/Vite module cannot locate the file. The primary way to address it would be verifying that there are no syntactic mistakes when defining the module. As an instance, inspect the path provided in the dynamic `import()`. If there’s a discrepancy in the location of your module, amend it accordingly.
// Suppose that 'MyComponent' is in the components directory let MyComponent = () => import('@/components/MyComponent.vue')
Also, ensure the server hosting your application is running as expected, another probable cause could occur if the server isn’t serving your Vue/Vite files correctly which halts successful imports of the modules. Additionally, take heed of caching issues and try clearing your browser cache occasionally, especially on significant updates.
Another possible reason for this error could be due to CORS (Cross-Origin Resource Sharing) policies. This isn’t a bug in Vue/Vite but rather a security feature implemented by browsers to prevent malicious scripts from contacting any servers. You may need to configure your server to allow requests from your Vue application or use proxying with something like webpack devServer to circumvent these restrictions.
To quote technology writer, Jeremy Keith, “One of the most salient features of our culture is that there is so much BS”. In relation to coding, understanding how certain aspects of code interrelate helps in the debugging process, whilst also ensuring we have a robust understanding of why errors like ‘TypeError Failed To Fetch Dynamically Imported Module On Vue/Vite Vanilla Setup’ happen and how to prevent them in the future.
Bear in mind that practices such as routine code maintenance, standardized coding conventions, and adopting Test Driven Development (TDD) are not only conducive for optimal coding environments but also in debugging and troubleshooting instances like this. Ultimately, approaching coding challenges with patience, systematic troubleshooting skills, and research would buffer considerably against unforeseen exceptions and TypeErrors.
Here is more information about dynamic imports in Vue.js.
Common Causes of TypeError | Solution |
---|---|
Syntax error during import | Check your import statements for proper syntax |
Server issues | Ensure the server being used to serve the application files is running correctly |
CORS Policies | Configure your server to allow requests from the Vue app or use a proxy to avoid these limitations |
In the grand scheme of problem-solving and code development, remember the profound words of Steve Jobs: “The biggest innovation in the twenty-first century will be at the intersection of biology and technology. A new era is beginning.” Be willing to learn, adapt and improve your knowledge base with every encountered challenge.
Preventing ‘Failed to Fetch’ Errors in Dynamic Module Imports
The “Failed to Fetch” error in the dynamic module import can be due to various reasons such as a faulty network, incorrect paths or URLs, Access-Control-Allow-Origin header issues, among others. When it comes to the TypeError Failed to Fetch dynamically imported module on Vue/Vite vanilla set up – this might occur if the paths or URLs are not correctly specified, there’s a server-side issue, or browser incompatibility problems.
Firstly, a significant point in handling ‘Failed to Fetch’ error is understanding how the dynamic import statement works. ECMA Script 2015 introduced modules into JavaScript, allowing us to separate our code into reusable parts. Here is an example showing how dynamic import work:
import('/modules/my-module.js') .then((module) => { /* do something with the module... */ });
Now, onto addressing the ‘Failed to Fetch’ error:
• Verify the Module Path: One of the probable reasons for the failure could be that the path does not exist or is incorrect. Ensure that the path you’re trying to import matches exactly with the physical location of JavaScript file.
• Check Server-side: You also have to ensure that the server is configured to be able to handle dynamic imports.
• Handle Cross-origin Resources: If you’re fetching data from different resources (cross-origin), you need to make sure the request has the appropriate CORS headers. Pay attention to the ‘Access-Control-Allow-Origin’ header, this must be correctly configured on the server-side to allow requests from your origin.
Brit Selvitelle, formerly at Twitter and currently CTO at Slack once said, “The best error message is the one that never shows up”. In line with his thoughts, proactively checking for errors and dealing with them ensures smooth code execution.
For Vue/Vite Vanilla setup, the process remains similar. Additionally, taking precautions like having a Vue/Vite setup that is compatible across different browsers and keeping an eye out for newer updates can prevent such issues.
An ideal way to eliminate the chances of encountering a “Failed to Fetch” error while working on dynamic imports in a Vue/Vite Vanilla setup is maintaining a thorough understanding of evolving JavaScript standards, keeping your setup updated, and ensuring proper server-side configuration.
You might want to delve deeper into dynamic import statement as described in Mozilla Developer Network or the Vue.js guide on module types.
Remember, “Anyone can dream up great ideas, but an idea is nothing until it’s realized, be it as a website, a physical product, an app, or a user interface.”– Jens Martin Skibsted. The same applies to error handling in programming. Thorough vigilance and structured debugging methods make code robust and error-free.
Taking into consideration the topic of TypeError: Failed to Fetch Dynamically Imported Module on Vue/Vite Vanilla Setup, we understand that it’s a common issue faced by JavaScript developers when handling Vue and Vite vanilla setup. This error typically occurs when there is an attempt to dynamically import a module which for some reason is not fetched successfully. We’ll discuss two main reasons why this might happen:
- Incorrect file path: If the specified path does not match where the intended file resides, it can lead to this error.
- Incompatible browser: Some older versions of browsers do not support dynamic imports. Hence, testing your application on various browser versions becomes important.
Debugging this error effectively requires scrutinizing your code for these potential pitfalls and implementing necessary changes. Sometimes, the error may not be in the file you’re trying to import but rather in one of its dependencies. So, care should be taken to check through all dependencies as well.
One efficient way of handling such issues is the use of
try
/
catch
blocks where you will try to fetch the module and then catch any error that could arise. Below is a simplified illustration of how to achieve this:
javascript
try {
const module = await import(‘./path/to/module.js’);
}
catch (e) {
console.error(e);
}
Remember what Linus Torvalds once said, “Talk is cheap. Show me the code”. Thus, not only understanding but also practicing bug fixing and debugging using actual code is crucial in enhancing your JavaScript development skills.
If you encounter further complications or unique scenarios related to ‘TypeError: Failed to Fetch Dynamically Imported Module on Vue/Vite Vanilla Setup’, online communities like Stack Overflow or the Vue.js forum can be of great help where experienced developers provide practical insights and solutions.