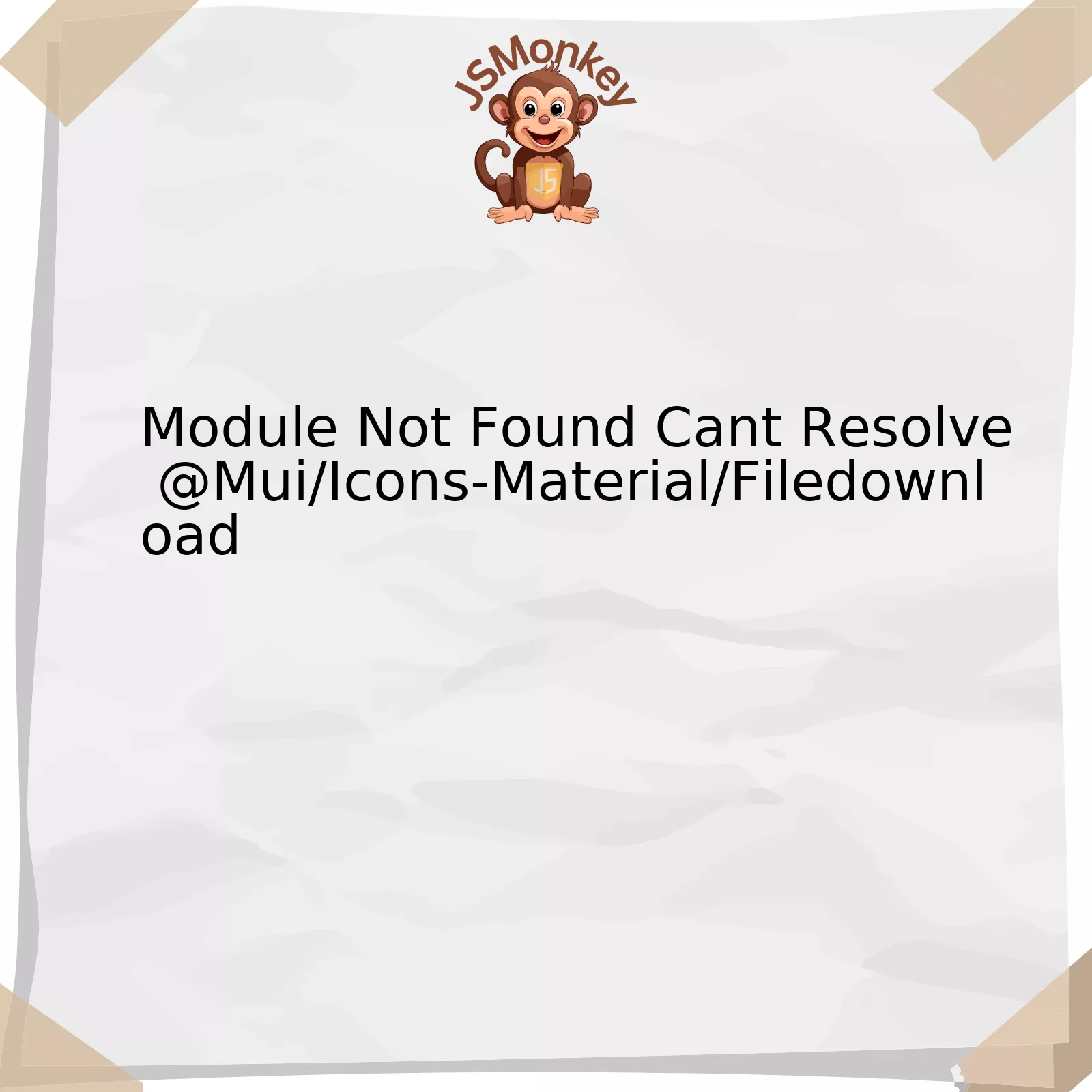
Often, the error message “Module Not Found: Can’t Resolve ‘@mui/icons-material/FileDownload'” indicates that there’s an issue where JavaScript is unable to locate or access a specific icon module you’ve attempted to implement in your application. Understanding the possible reasons and solutions can be key to resolving such problems efficiently.
Possible Causes | Corresponding Solutions |
---|---|
The ‘@mui/icons-material’ package isn’t properly installed. | Use the package manager (like npm or Yarn) to correctly install the relevant module by executing the command
npm install @mui/icons-material or yarn add @mui/icons-material . |
The import path specified for the ‘FileDownload’ icon might be incorrect. | Double-check both casing and spelling within your import statement. It should resemble something like:
import FileDownloadIcon from "@mui/icons-material/FileDownload"; |
A version discrepancy might exist between your application’s ‘@mui/icons-material’ module and other elements of your project. | Ensure all dependencies are aligned with each other in terms of versioning. Also, consider upgrading to the latest versions if possible. |
It should be noted that while the above details serve as standard troubleshooting steps in resolving this particular module not found error, the development landscape is dynamic and every situation may need an approach tailored to its unique circumstances and requirements.
To paraphrase Edsger W. Dijkstra, one of the pioneers of computer programming, “programming is one of the most intricate aspects of computational science and resolving errors – a fundamental part of it.” Pulling from his wisdom, navigating these errors and issues is an essential part of the development journey.(source)
Understanding the “Module Not Found: Can’t Resolve @Mui/Icons-Material/Filedownload” Error
When you encounter the error “Module Not Found: Can’t Resolve @Mui/Icons-Material/Filedownload” in your JavaScript project, it’s indicative of a misplaced or misspelled module within your program.
Reasons Behind the Error
Let’s dissect the possible reasons behind this specific error:
- Misinterpretation of the import statement: This problem could arise if the import statement is not correctly conveying to the application the exact location of the module “@Mui/Icons-Material/Filedownload”. Improper specification of the path promotes ambiguity that the system struggles to resolve.
- Absence of the required package: Ideally, the ‘@mui/icons-material’ should be installed in your node_modules directory. The absence of this package will result in a “Module not found” error. Errors could also emanate from missing dependencies within this package.
- Incorrect spelling or module name: TypeScript is case-sensitive, meaning ‘FileDownload’ and ‘filedownload’ are interpreted differently. Hence, writing the module name with incorrect letter casing or spelling could be generating the error.
Solutions
Armed with possible explanations behind the error, let’s explore solutions to rectify it:
- Install @mui/icons-material: Run
npm install @mui/icons-material
command in your terminal to ensure that the ‘@mui/icons-material’ package has been added into your node_modules directory.
- Ensure accurate spelling and correct usage of case sensitivity: As discussed earlier, ‘fileDownload’ is different from ‘FileDownload’. Make sure the module name’s casing and spelling match the original ones.
- Clear cache: Sometimes, issues can spring up from the cache, which could have stored outdated dependency information. You can use
npm cache clean --force
to clear the npm cache.
Practical Example
Firstly, import the Mui Icons package:
npm install @mui/icons-material
Then include FileDownload icon in your file:
import FileDownload from "@mui/icons-material/FileDownload";
Remember these commands need to be executed as per the correct sequence. Inappropriate sequenced execution might not resolve your problem.
“A good programmer is someone who always looks both ways before crossing a one-way street.” – Doug Linder, pioneer of Unix Operating System. In this context, it advises you to perform due diligence when it comes to coding or debugging with respect to capitalization, syntax, or even installing new packages. Doing so not only steadies your programming vessel but also saves the additional work that comes with spotting trivial mistakes.
For more in-depth understanding regarding dealing with module errors in JavaScript, I recommend visiting official documentation like [Node.js Errors Documentation](https://nodejs.org/api/errors.html) or community threads on platforms such as StackOverflow, GitHub etc. Trustworthy references are a great way to enhance your knowledge and understand diverse perspectives to handle distinct issues. Would love to hear your progress!
Remember, your quest for resolving errors makes you a better problem-solver and an efficient coder.
Troubleshooting Techniques for “@Mui/Icons-Material/Filedownload” Module Error
When we encounter a problem such as a module error in JavaScript, it’s useful to think of this not merely as an inconvenience but an opportunity to grow as developers. As Bill Gates once said, “It’s fine to celebrate success but it is more important to heed the lessons of failure.” In this specific case, your question pertains to the troubleshooting techniques for “@mui/icons-material/filedownload” module error and how it relates to the ‘Module Not Found Can’t Resolve’ issue.
Before delving into the potential solutions, let’s first understand the error itself. A ‘Can’t resolve’ error signifies the inability of the webpack bundler used by React.js applications to locate the specified ‘@mui/icons-material/filedownload’ module.
This could be due to any of the following reasons:
To address the issue at hand, let’s focus on several possible solutions:
1. Checking the spelling and case of the ‘@mui/icons-material/filedownload’ module:
The letter casing or the spelling specification of the module name should correspond exactly with how it is named in the module package. JavaScript is case-sensitive, which means @MUI/ICONS-MATERIAL/FILEDOWNLOAD is different from @mui/icons-material/filedownload.
Chaos famously characterized JavaScript’s flexibility as both its beauty and weakness, stating, “The two hardest things in computer science are naming things, caching problems, and off-by-one errors.”
2. Ensuring the correct installation of ‘@mui/icons-material/filedownload’ module:
Sometimes, simply reinstalling the module can resolve the issue. You can delete the node_modules folder and the package-lock.json file then run
npm install
again.
3.Path issues with the ‘@mui/icons-material/filedownload’ module:
Make sure that the ‘@mui/icons-material/filedownload’ is in the correct path where it’s supposed to be imported. A wrong or relative path could potentially lead to this problem.
4.Verifying the existence of the ‘@mui/icons-material/filedownload’ module:
It’s essential to confirm if the ‘@mui/icons-material/filedownload’ module still exists within the Material-UI library you’re utilizing. It’s possible that the required package does not exist anymore or has been replaced by a new one. If this is the case, the best thing would be to refer to the Material-UI documentation.
Remember, even as developers, who deal with logic each day, we still have no better tool than trial and error sometimes. Thomas Edison said it best: “I have not failed. I’ve just found 10,000 ways that won’t work.” Don’t be disheartened when issues seem too complex to handle because every issue resolved brings us nearer to becoming better developers.
Exploring Possible Causes of ‘@Mui/Icons-Material/Filedownload’ Resolution Issues
For a developer, encountering a “Module Not Found” error can be daunting. Specifically, if you are dealing with ‘@mui/icons-material/FileDownload’ resolution issues, the inherent problem could be attributed to numerous factors including deprecated modules, incorrect installation, path discrepancies, and version compatibility issues among others.
A. Deprecated Modules
The first possible cause of the issue might be due to deprecated modules. If ‘@mui/icons-material/FileDownload’ has been depreciated in the version of Material UI you are using, this module will no longer exist and hence, will result in an error.
// For example, this attempt to import the file download icon from material ui import FileDownload from '@mui/icons-material/FileDownload';
If this is the core issue, your best bet would be to review the official MUI icons documentation to find the new name of your desired icon.
B. Incorrect Installation
An improper or incomplete installation of the ‘Material UI Icons’ package can also lead to your code failing to recognize ‘@mui/icons-material/FileDownload’. This concern can be fixed by ensuring the library is correctly installed and updated. Try uninstalling and then reinstalling the ‘@mui/icons-material’ package:
// Uninstall the package npm uninstall @mui/icons-material // Reinstall the package npm install @mui/icons-material
C. Path Discrepancies
In some instances, the file path may not be correctly referencing your module. Check that in your React component, you are accurately importing ‘@mui/icons-material/FileDownload’.
// This line should exist at the top of your component import FileDownloadIcon from '@mui/icons-material/FileDownload';
D. Version Compatibility Issues
Incompatibility with other packages or the project itself could be a potential factor in the ‘Module Not Found’ error. Verify that your project’s dependencies are compatible with ‘@mui/icons-material’.
As Richard Branson has notably said, “Complexity is your enemy. Any fool can make something complicated. It is hard to make something simple.” This phrase resonates within the coding sphere too as sometimes the most direct solution might be the best one.
In conclusion, debugging should start from checking the documentation of the package for any recent changes. Staying updated with package documentation will not only keep you informed about deprecated modules but also educate you on newly introduced features and methods.
Optimal Solutions for Resolving ‘@Mui/Icons-Material/Filedownload’ Missing Module Errors
When dealing with the issue of a missing module in the scope of “@mui/icons-material/filedownload” in JavaScript, it’s critical to adopt an optimum approach for a quick resolution. The error frequently comes across as “Module Not Found Can’t Resolve @mui/icons-material/filedownload” and can cause considerable distress and function loss.
The anatomy of the error
The emergence of this specific error message is indicative of your project failing to locate the required ‘@mui/icons-material/FileDownload’ module. There are several underpinning reasons contributing to the occurrence of such an event, ranging from an inappropriate installation of material-UI icons to incorrect import statements.
Possible Causes
- Your current environment might be lacking the necessary material-ui icons package
- Inappropriate import name that deviates from the officially supported names
- Inaccurate path values when importing the module
Optimum Solutions
In order to nip this problem at its bud, one can consider the following effective strategies:
Installing or Reinstalling Material-UI Icons Package: It’s possible you may not have properly installed the material-ui icons, or perhaps forgot to install them altogether. Running the corresponding npm command below will rightly deliver the package to your development environment:
npm install @mui/icons-material
Correct Import Naming: An incorrect import name could be another potential cause for this kind of error. Pay careful attention to case sensitivity when writing import sentences since JavaScript distinguishes between upper and lower cases. The correct form should look like:
import FileDownload from '@mui/icons-material/FileDownload';
Check Project Dependency Tree: If all else fails, double-check your project dependency tree just to make sure all dependencies are correctly addressed. Update any packages if needed and ensure that your project aligns with the latest versions or with the ones that function properly. Debugging tools like npm ls or yarn list can aid immensely in reviewing your dependencies.
As asserted by Martin Fowler, an esteemed software developer:
“If you can get today’s work done today, but you do it in such a way that you can’t possibly get tomorrow’s work done tomorrow, then you lose.”
Therefore, it’s of utmost importance to keep revisiting this error by maintaining your package versions updated and periodically validating your import statements in order to maintain consistency. This will prevent progess limitation and bypass such frustrating errors in the future.
For more insights into solving similar issues, you may visit [JavaScript documentation](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Guide)
or explore [online programming forums](https://stackoverflow.com/questions/tagged/javascript) where global developers interact to discuss their problems and solutions.
The issue of “Module Not Found: Can’t Resolve ‘@Mui/Icons-Material/FileDownload'” typically arises when the system fails to find the specified module. To grasp this predicament thoroughly let’s delve into the core reasons scenarios and solutions.
Primarily, in JavaScript development, especially when working with React and notable UI libraries like Material-UI, it’s crucial to keep track of package versions as they often cause such difficulties. The ‘@mui/icons-material’ package contains a vast array of icons one might require, including the ‘FileDownload’ icon. However, issues can occur if you’re using an incorrect or antiquated version of the package.
The correct way to import the ‘FileDownload’ icon from Material-UI library would be following the next piece of code:
import FileDownload from '@material-ui/icons/FileDownload';
Another recommended route to circumvent this problem is by ensuring that the @mui/icons-material package has been correctly installed in your project. A simple installation or reinstallation command can often solve the issue:
npm install @mui/icons-material
or
yarn add @mui/icons-material
In case these aforementioned strategies fail to deliver a resolution, consider checking the assembled node modules or even your project’s configuration. Sometimes, minor misconfigurations can exhibit major discrepancies leading to anomalies like “Can’t resolve ‘@mui/icons-material/FileDownload'”. If any deviations are found within the configurations, rectify them immediately.
“Simplicity is prerequisite for reliability.” – Edsger Dijkstra. This quote underlies a key principle of coding and programming. One needs to keep their code structure modest and clear-cut to ensure reliable and glitch-free
So, strive to maintain updated packages. Regularly cross-check your project configurations and troubleshoot issues promptly with a systematic approach. It ensures that your JavaScript development process stays seamless, allowing you to craft efficient, high-performing applications.
For further expansive information on JavaScript development and troubleshooting, the StackOverflow community is a valuable resource, providing immediate solutions from experienced developers across the globe.
Remember, every problem has a solution; sometimes, it’s all about finding the right path to resolution and learning from each experience along your coding journey.