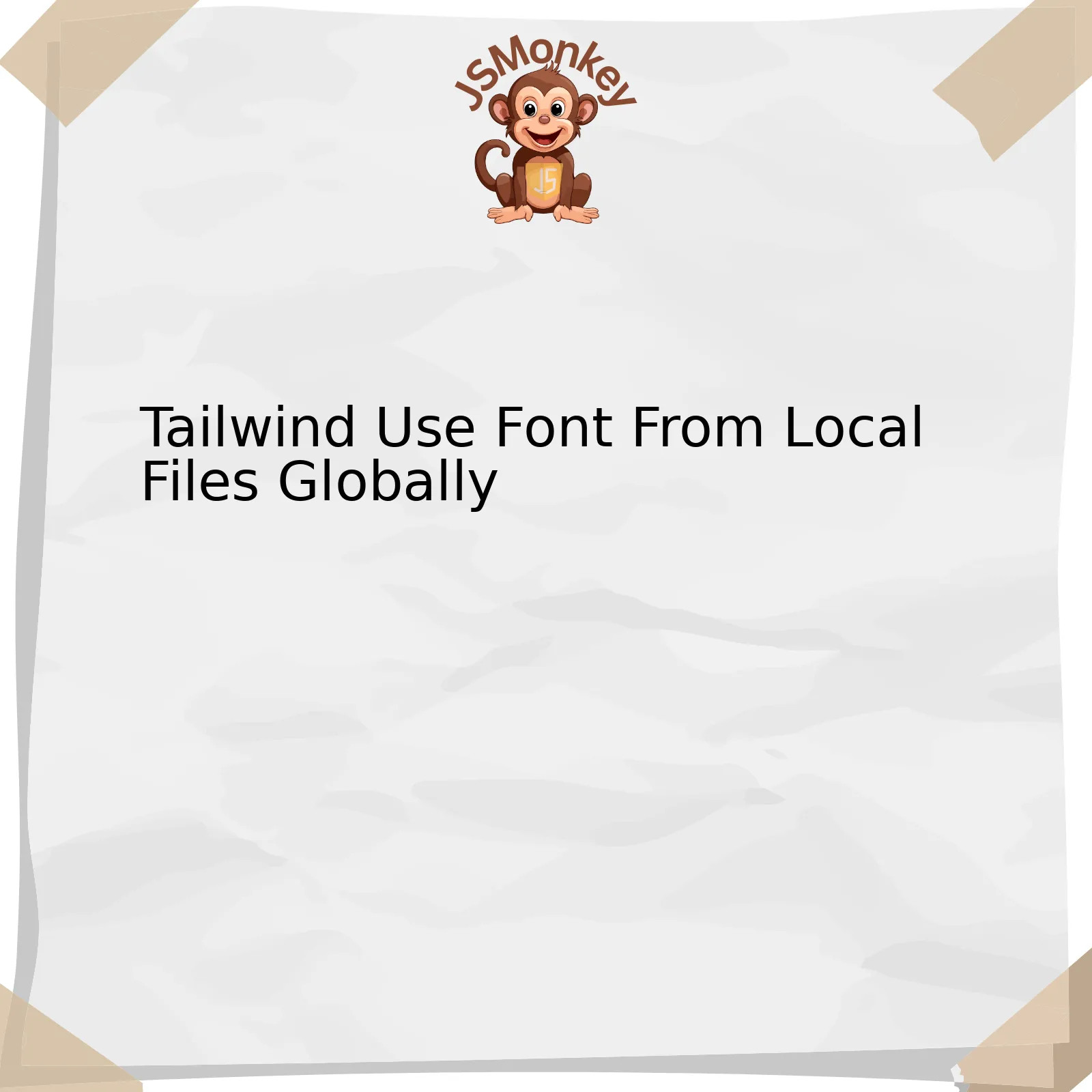
Tailwind CSS is a utility-first CSS framework that allows developers to customize designs directly in their markup. One of the features it provides is customizing fonts, which traditionally are imported from external files. However, there may be instances when you want to use local fonts with Tailwind CSS.
Here’s how we can define the font in our tailwind.config.js file:
javascript
module.exports = {
theme: {
extend: {
fontFamily: {
‘custom’: [‘CustomFont’, ‘sans-serif’],
}
},
},
variants: {},
plugins: [],
}
In this configuration, ‘CustomFont’ is the name of the font saved in your local files. Tailwind will look for this font first and if it doesn’t find it, it falls back to using ‘sans-serif’.
To make these fonts available globally, add @font-face atrule in your CSS file where you define your local file path.
@font-face { font-family: 'CustomFont'; src: local('CustomFont'), url('/fonts/CustomFont.woff2') format('woff2'), url('/fonts/CustomFont.woff') format('woff'); font-weight: 400; font-style: normal; }
It is important to ensure that the font names match exactly between @font-face in your CSS and the fontFamily section in your Tailwind config.
File Name | File Type | Path |
---|---|---|
tailwind.config.js | Javascript File | /project/root/tailwind.config.js |
@font-face declaration | CSS rule | /project/root/css/styles.css |
As per Eric Meyer, the author of CSS: The Definitive Guide, “The @font-face isn’t a property, but is a top-level CSS construct that has properties hanging off it.” This quote justifies the usage of @font-face rule as we cross-check and match our local font with the declared one in CSS repository.
Remember, when working with Tailwind and local font files, the crux is in aligning your tailwind.config.js file with your locals’ @font-face declaration. Be aware that incorrect paths or wrongly spelt font names can lead to unwanted results or fonts not being displayed at all.
For best practices, keep fonts in their dedicated directory, usually /fonts/, and ensure you reference these correctly within your CSS and JavaScript files.
Tailwind takes the configuration from your JS file, so ensure the names are correctly mentioned in the fontFamily object. It then becomes easy to apply these fonts globally by simply adding .font-custom to any classes where you want this font applied.
And there you have it, a succinct, step-by-step guide to using locally stored fonts globally in your projects using Tailwind. Remember, the key lies in maintaining consistency between your CSS and JS files. Happy coding!
Utilizing Local Files for Global Font on Tailwind
Tailwind CSS is a highly customizable, low-level CSS framework that provides utility classes to build your website design. While it comes with a set of fonts, in some situations you might require to use custom font files locally and apply them globally to your entire site. Following are the detailed steps to utilize local files for global fonts on Tailwind CSS:
Step 1: Install Necessary Dependencies
If you haven’t already done so, you’ll need to install Tailwind CLI and its peer-dependencies.
npm install tailwindcss@latest postcss@latest autoprefixer@latest
Make sure to include the @latest tag to ensure that you’re always using the most recent version of Tailwind.
Step 2: Include Custom Font Files
Place all the desired font files in a directory such as
./fonts
. These files could be of different formats like .woff, .woff2, .ttf etc., and would be used based on the support of different browsers.
step 3: Define Custom Fonts in the Tailwind CSS Configuration File
You can extend Tailwind’s default configuration by using the theme section of your tailwind.config.js file. In this case, you will add fontFamily property and mention path of your local fonts.
module.exports = { theme: { extend: { fontFamily: { 'custom': ['CustomFont', 'sans-serif'] } } }, variants: {}, plugins: [] }
In the above example, ‘CustomFont’ is the name of your custom font and ‘sans-serif’ is a backup font that will be used when the custom font fails to load.
Step 4: Add Global Font-Family to your CSS
To apply the font globally, in your global CSS file, you can declare ‘fontFamily’ for body tag.
body { @apply font-custom; }
In this code snippet, ‘font-custom’ is the name of your custom font specified in tailwind.config.js file.
Step 5: Build with Tailwind CSS
Now run
"npx tailwindcss build style.css -o output.css"
in your command line interface(CLI). After successful compilation, the font defined will be applied globally across the entire website by Tailwind CSS.
In the words of Ken Thompson, one of the renowned programmers, “When in doubt, use brute force.” Understanding how to approach solving coding problems will save you time and frustration. Leveraging Tailwind CSS powerful utility classes and configurations will definitely help to accomplish your demands just like using local fonts here.
For a more detailed walkthrough, consider checking the official Tailwind documentation. Here you get context-specific answers, as well as additional resources that can help you understand how to work effectively with Tailwind CSS.
Practical Steps for Setting Up Fonts Globally in Tailwind
When it comes to integrating local fonts globally in a Tailwind CSS project, there are some key steps that need to be undertaken. The major advantage of this approach is optimizing website performance by minimizing the reliance on external resources.
Step 1: Install and Setup Tailwind CSS
This step involves installing Tailwind CSS into your project through NPM or Yarn. If you are creating a new project, you might want to initial a new project first, then install Tailwind.
npm install tailwindcss
Step 2: Include Desired Local Font File(s) in Your Project
Local font files should be added to your project folders. An industry best practice is to create a separate “fonts” folder in your “src/assets” directory. You can store all your local font files here.
Step 3: Use @font-face Rule in Your Global CSS File
In your global CSS file, utilize the
@font-face
rule to specify your custom font. Below is a simplified example:
@font-face { font-family: 'MyCustomFont'; src: url('/src/assets/fonts/MyCustomFont.woff2') format('woff2'), url('/src/assets/fonts/MyCustomFont.woff') format('woff'); }
Here we give our font a name ‘MyCustomFont’ and then specify the source URL for the font files. We’ve used both WOFF and WOFF2 formats to ensure maximum browser compatibility.
Step 4: Extend Tailwind’s fontFamily Property
In your tailwind.config.js file, extend the default fontFamily property to include your custom font like so:
module.exports = { theme: { extend: { fontFamily: { 'custom': ['MyCustomFont', ...defaultTheme.fontFamily.sans], }, }, } }
With the above configuration, you are essentially telling Tailwind to prioritize ‘MyCustomFont’ before falling back to the default sans-serif font stack.
Step 5: Use Your Custom Font in Your Styles
Finally, make use of your custom font in your styles with the font-class:
<div class="text-4xl font-custom">This is my custom font!</div>
These steps should help you get started with setting up and using local fonts globally in your Tailwind CSS projects. Ensure you test across different browsers to reaffirm proper loading and rendering of your chosen typeface.
As Steve Jobs said, “Design is not just what it looks like and feels like. Design is how it works.” This process may seem meticulous, but excellent web design hinges on such attention to detail.Tailwind CSS Fonts Documentation
Understanding the Impact of Using Local Files as Global Fonts in Tailwind
Using local files as global fonts in Tailwind CSS is an effective approach that allows developers to maintain a consistent typography throughout their projects. It provides the liberty of integration with local resources rather than relying on external sources. This renders an enhanced control on the project font handling leading to a more uniform, customized, and appealing visual aesthetic.
Benefits | Considerations |
---|---|
Customization: Developers can use their own fonts, regardless of whether they’re supported by popular font libraries. | Load Time: While local files load faster, understanding their impact on overall application performance is essential for optimization. |
Performance Gain: Browsers don’t need to download from a different server each time when the fonts are stored locally. | Execution Path: Careful consideration needs to be given to execution paths, ensuring the right order and structure is maintained. |
For integrating local font files, you add in your tailwind.config.js a new @font-face rule in your CSS, using the
@apply
rule to set the font globally.
Functionality can be achieved by appending the following snippet to one’s configuration:
module.exports = { theme: { extend: { fontFamily: { 'custom': ['Custom Font', 'sans-serif'], }, } }, variants: {}, plugins: [], }
As Marc Andreessen, billionaire tech entrepreneur and investor, once claimed: “Software is eating the world”. Therefore, mastering the best practices such as using local files as global fonts in Tailwind CSS can facilitate a smoother rendering and optimisation process, contributing towards creating an overall more fluid, reliable, and appealing software or web application. However, developers need to keep in mind all potential implications of this approach to ensure excessive file sizes do not offset these benefits. Options for scaling and optimization should always be explored and applied.
Detailed Guide to Manage and Modify local file fonts In Your Tailwind CSS Projects
Managing and modifying local file fonts in your Tailwind CSS projects involves incorporating the locally stored font files directly into the Tetras framework, which will then allow these font faces to be referenced globally across all associated files. This process can considerably enhance the customization capacity of your project, permitting a more dynamic adaptation of icon sets and typography based on specific project requirements.
The handling of local file fonts in Tailwind CSS can be divided into several key components:
Font File Identification
To use local fonts, you first need to have access to your desired font’s .woff or .woff2 file. These files contain comprehensive font data and enable browsers to accurately render your specified typography.
Project Configuration
For their integration with Tailwind CSS, these font files must be held in a recognized directory within your project structure. Typically, a folder named ‘fonts’ is created in the ‘public’ or ‘src/assets’ directory, although this location can vary depending upon personal or project-specific preferences.
Importation into CSS
Following this, the local font files are imported into an existing .css or newly created .css file. The
@font-face
rule is employed for this purpose:
@font-face { font-family: 'Your Font'; src: url('/fonts/YourFontFile.woff2') format('woff2'), url('/fonts/YourFontFile.woff') format('woff'); font-weight: 400; font-style: normal; }
Here, ‘Your Font’ is the name by which you’ll reference this font throughout your project, while ‘YourFontFile.woff2’ and ‘YourFontFile.woff’ indicate the pathway to your font files.
Modification of tailwind.config.js
In the finalization of this incorporation process, a modification of tailwind.config.js is needed. The fontFamily theme property must be extended to include your new font:
module.exports = { theme: { extend: { fontFamily: { 'custom-font': ['Your Font'] } }, }
The result is a globally accessible font face that can be conveniently invoked using Tailwind’s typography plugin or the
font-family
class.
As Steve Jobs once said, “the details matter, it’s worth waiting to get it right,” and managing local file fonts in Tailwind CSS truly exemplifies this concept. By granting developers significant control over the visual aspects of their project, Tailwind allows for more aesthetic cohesiveness and excellent UX design.
Tailwind Documentation <+>@font-face on MDN
In the realm of web development, dealing effectively with typography is paramount, and this includes understanding how Tailwind, JavaScript’s utility-first CSS framework, interacts with fonts stored on local files. Local file fonts ensure a more consistent visual experience for all users, regardless of their geographical location or Internet speed.
To use font from local files globally in Tailwind, you should follow these procedures:
The process begins by adding your font file (usually in .woff or .woff2 format) to your project directory. Ideally, you’d want to create a separate folder, aptly named ‘fonts’, to store these files.
Next, leveraging tailwind.config.js provides us an avenue to add customizations to our Tailwind installation. We incorporate the @font-face rule, designating the font-family name and specifying the source file path.
Additionally, extending the fontFamily configuration enables global utilization of our newly installed local font across the entire application. It is well worth noting that these requirements encourage an effective, scalable, and standardized typographical aesthetic across our application.
Here’s an illustrative example code:
html
module.exports = { theme: { fontFamily: { customFont: ['Custom Font', ...defaultTheme.fontFamily.sans], }, extend: {}, }, variants: {}, plugins: [], };
While setting up font use from local files globally with Tailwind might seem complex initially, the advantages far outweigh any perceived drawbacks. Not only does it amplify user experience, but it also provides a thematically consistent look and feel across diverse platforms. Better yet, storing and loading your fonts off local files can actually improve page load times, leading to higher SEO rankings.
As Bill Gates once said, “We are changing the world with technology”. Using Tailwind’s utility-first framework to incorporate locally saved fonts gives you full control over your web application’s aesthetics while truly leveraging the possibilities resident in modern web design practices.
For further insights, you can explore this hyperlink – [Tailwind documentation](https://tailwindcss.com/docs/configuration#referencing-in-java-script). Enjoy your exploration and happy coding to you!