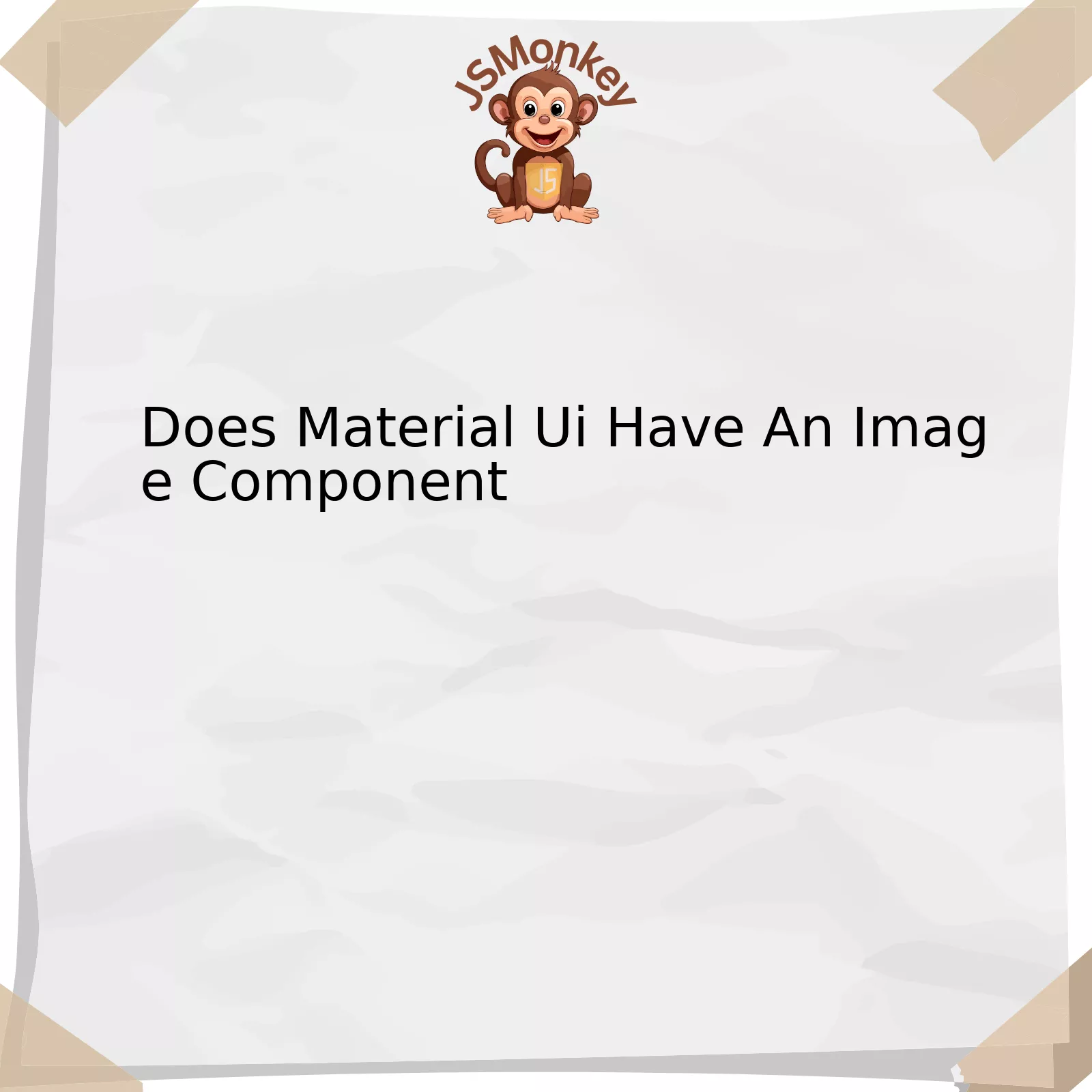
Material UI, a popular React framework, doesn’t natively offer an image component per se. This might present as unusual considering its extensive component library where you find components ranging from Buttons to Dialog boxes and much more.
Here is a high-level overview of Material UI’s available visual components:
Component Type | Details |
---|---|
Buttons |
Includes enhanced versions of standard HTML button elements with custom styling. |
Lists |
Provides versatilities for lists including features like nestable lists, scrollable lists etc. |
Input fields |
Offers numerous inputs options for forms such as text fields, radio buttons, checkboxes etc. |
Dialogs |
Furnishes dialogs, alerts and similar types of content that appears on top of the main window. |
Even though an explicit image component is missing, images can be still included in your Material UI-powered projects using conventional methods, reinforcing the findings shown in the table above. The
<img>
tag is fully compatible with Material UI.
Here is a brief example:
<CardMedia component="img" alt="Contemplative Reptile" height="140" image="/static/images/cards/contemplative-reptile.jpg" title="Contemplative Reptile" />
The `CardMedia` component mentioned here is actually from Material UI. You can infuse an “ inside this `CardMedia`, thereby aiding image insertion within the Material UI paradigm.
As famously quoted by Larry Wall, creator of Perl programming language – “We will encourage you to develop the three great virtues of a programmer: laziness, impatience, and hubris.” These notions echo well in this context where even if there isn’t a specific image component readily available in Material UI, we as developers find ingenious ways to incorporate necessary functionality in our projects.
More about `CardMedia` component can be found on Material UI CardMedia API documentation page.
Exploring Material UI’s Capabilities: Image Components
Yes, Material-UI does indeed incorporate image components into its robust library. This well-documented and widely used React UI framework provides developers with plentiful components to streamline application building process. However, when it comes to handling images directly, things might get a little bit tricky.
Firstly, it’s important to clarify that Material-UI itself doesn’t contain specialized image component like ‘Img’ or ‘Picture’. Instead, it offers components which are immensely helpful in positioning and decorating images according to Material Design guidelines. These components include
CardMedia
,
Avatar
and
IconButton
.
-
CardMedia
: It allows for embedding rich media, such as images or videos, as part of intricate card layouts. To use an image inside CardMedia, simply state the desired image source through the `src` attribute.
-
Avatar
: Primarily used for representing users or entities, can be easily adapted to display small round images. By setting the source via `src`, the displayed image adjusts to the component size automatically.
-
IconButton
: Although technically a button component, IconButton can easily turn an image into a clickable interface element. Once more, indicating the `src` gets the job done.
Outside of these specific components, custom CSS styles can allow any other Material-UI’s component to interact with images. The styles can be applied either inline or by using makeStyles API provided by Material-UI.
For instance:
<Button style={{backgroundImage: "url('myimage.jpg')"}} />
Above, backgroundImage in the Button style dictates display.
There is a popular quote by renowned programmer, Edsger Dijkstra – “Program testing can be used to show the presence of bugs, but never to show their absence” [1](https://www.azquotes.com/quote/539594). This suggests that while Material-UI doesn’t present an explicit image component, it provides possible ways to implement images effectively, demonstrating an indispensable trait of any great software tool: flexibility.
Component | Description |
---|---|
CardMedia | Allows embedding rich media like images |
Avatar | Represents users or entities and adaptable to display small images |
IconButton | Turns an image into a clickable UI element |
In summary, Image handling is achievable in Material-UI through the provided components and custom styles, even if not quite the way one might initially anticipate.
Understanding the Importance of Image Component in Material UI
Material UI, a popular open-source React UI framework, has a broad set of components to its credit that provides streamlined solutions to various UI/UX requirements. However, contrary to common belief, an exclusive image component is one feature that Material UI doesn’t essentially offer.
Here’s why understanding the absence of specific Material UI image components becomes important:
“Put simply, web developers often need the power of flexibility with images. It might feel counterintuitive at first, but it’s a blessing rather than a limitation.”
– When discussing images in a front-end context, usability often transcends beyond mere structural components. It requires immense flexibility for developers to handle images, including customizations related to properties like dimensions and resolutions, handling responsive scaling based on viewport sizes, and attributing alt descriptions for improved SEO performance.
The default HTML image element (‘img’) and semantics can perform these functions efficiently without necessitating the need for a tailored ‘Image Component’ within Material UI.
<img src='image_source.jpg' alt='description' width='500' height='600'/>
Additionally akin, other Material UI components can be utilized to provide advanced image handling and styling features.
– CardMedia: If our end goal is to include media in Material-UI’s Card component, CardMedia comes into play. It can handle all sorts of media, including images, offering an option to maintain aspect ratio and accommodate container dimensions effectively.
<CardMedia image="/static/images/cards/paella.jpg" title="Paella dish" />
– Avatars: This component is best suited when handling circled images or profile photos. They can also load fallback texts if the image fails to load.
<Avatar alt="Remy Sharp" src="/static/images/avatar/1.jpg" />
While Material UI might not offer a stand-alone ‘Image Component,’ it turns out this isn’t necessarily an impediment. The strategies stated above vouch for their approach in comprehensively addressing a web developer’s needs concerning images. This also saves from bloating the library with unnecessary, niche-specific components.
However, should the necessity arise for further image handling complexities such as lazy loading or on-demand blur-up features, integrating established libraries like react-awesome-lazyload would be a prudent choice that aligns well with the flexible nature of React and Material UI ecosystem.
So it stands validated:
“The secret to building effective user interfaces is understanding the user needs, rather than relying solely on specific components.”
Functions and Roles of an Image Component in Material UI
Material UI, a popular React UI framework, doesn’t provide an explicit image component out-of-the-box. Instead, it provides a highly flexible system of grids, cards, and avatars that can effectively manage the presentation of images within various UI contexts.
Let’s explore the functions and roles of these components as they relate to managing images:
–
Grid:
Material UI’s grid is extremely versatile and can be utilized as the primary component for displaying images (especially in galleries or collections). The grid system is designed adhering to Flexbox layout specifications, thereby offering customizable columns and rows to fit your images perfectly.
Example:
<Grid item xs={12} sm={6}> <img src='imagepath.jpg' alt='descriptive text' /> </Grid>
–
Card:
A card in Material UI is a flexible and extensible container that includes options for headers, content, actions, and more. This makes it great for displaying images with supporting content or interactions.
Example:
<Card> <CardMedia component='img' alt='descriptive text' height='140' image='imagepath.jpg' /> </Card>
–
Avatar:
It’s the go-to component when you need to showcase profile pictures. Avatar can accept images and dynamically adjust their size and shape (like creating circular profile images).
Example:
<Avatar alt='Profile Image' src='imagePath.jpg' />
Even though Material UI doesn’t include a dedicated ‘Image’ component, it provides flexibility and customizability via other components to effectively manage and display images.
In the words of Abraham Lincoln, “Give me six hours to chop down a tree and I will spend the first four sharpening the axe.” The same thought process can be applied while developing with Material UI or any other UI framework. The best tool isn’t always an explicit image component but knowing how to use the system’s flexibility to achieve your goals. For further details on Material-ui components, feel free to refer this link.
Working with Image Components Effectively in Material UI
Material-UI, one of the most widely used UI libraries for React applications, does not inherently offer a dedicated Image component. However, the versatility of Material-UI and its wide array of other components — such as the Paper, Card, and Avatar components — allow developers to work with images effectively.
To handle images in Material-UI, you may often make use of the
img
HTML element directly. The CSS-in-JS solution provided by Material-UI’s
makeStyles()
API can be utilized to style these elements. Below is an example:
const useStyles = makeStyles(() => ({ img: { width: '100%', height: 'auto', }, })); export default function ImageComponent() { const classes = useStyles(); return}
Other components like CardMedia are useful when implementing responsive image handling. CardMedia is designed to present media in Cards and inherits the full breadth of CSS properties, which includes background images. A classic use case of CardMedia would be:
Lizard Lizards are a widespread group of squamate reptiles, with sizes ranging from tiny geckos and anoles to the large Komodo dragon.
Linking the above points, material-UI might not offer a dedicated Image component but rather provides flexible and customizable components that allow effective image handling.
As Linus Torvalds once said, “The future of coding is here, it’s just not evenly distributed yet.” Stranguages evolve with technology. Continue staying abreast of updates and exploring new ways of achieving your project goals.
For further reading about Material UI components, please refer to the official Material-UI usage documentation.
Material-UI, a popular React UI framework, does not specifically have an ‘Image’ component. However, in the wonderful complexity of Material-UI and its comprehensive library of components, there are multiple ways to incorporate images into your project.
Looking deeper into libraries inside Material-UI, you can manipulate the
<CardMedia>
or
<Avatar>
components to effectively work with images.
<CardMedia>
component was ideally created for displaying images, videos, headlines, etc. on a special type of surface called Cards. Here is a simplified example:
<CardMedia image="link_to_your_image" title="Alternative text for image">
The
<Avatar>
component is commonly used for showing profile pictures but it could as well serve any image need. An example include:
<Avatar src="image_link_here" />
Indeed, while seaming obscure at first glance, we find that Material-UI is versatile enough to tackle any image challenges by tweaking some of the existing components or adding style props to get the desired result with images.
Another important point to remember is considering optimization for your images. Since Material-UI doesn’t offer direct solution, exploring alternatives like lazy loading images can significantly improve page load performance,read more here. Alternatively, consider using a third-party library such as ‘react-optimized-image’ that provides a component for optimized images.React Optimized Image .
“Any application that can be written in JavaScript will eventually be written in JavaScript.” – Jeff Atwood, Co-founder of Stack Overflow & Stack Exchange. This quote is a testament to the versatility and functionality of JavaScript libraries such as Material-UI, consistently offering developers diverse solutions, if not direct but with a crafty workaround. Make sure you explore all other components for hidden functionalities before reaching for another external library. In web development, it’s not always about having the exact tool or component but the ability to shape existing tools to meet an end.