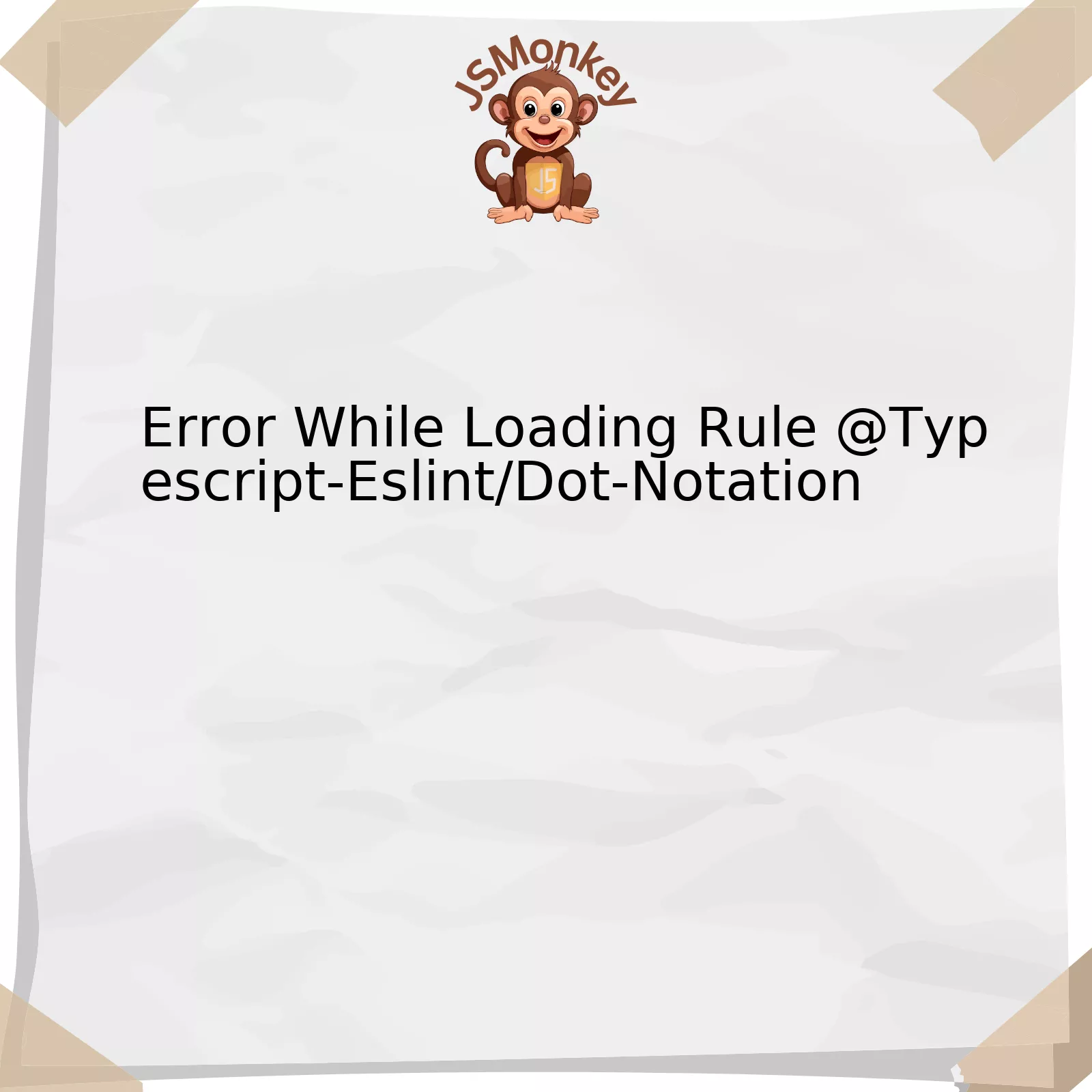
Taking into consideration the technical subject matter, it is helpful to illustrate the relevant details pertaining to the ‘Error While Loading Rule @Typescript-Eslint/Dot-Notation’ using an organizational framework. Let’s utilize this below:
Error | Possible Cause | Solution |
---|---|---|
@Typescript-Eslint/Dot-Notation | Mismatch of ESLint and TypeScript versions, or incorrect configuration | Ensure appropriate compatibility and correct configuration settings |
Essentially, ‘@Typescript-Eslint/Dot-Notation’ is an error usually encountered during linting process when you’re working with TypeScript on ESLint. The primary function of this rule is to enforce consistent usage of dot notation whenever possible, thereby promoting more concise and simplified code.
However, encountering an error while loading this specific rule might be primarily caused due to one of two reasons – either the versions of ESLint and TypeScript that you are using have some sort of mismatch or incompatibility, or there might have been some errors in configuring your project set-up.
To overcome this issue, the remedial measures include troubleshooting whether there is an appropriate compatibility between the version of TypeScript and ESLint you’re using. Node.js projects often have strict requirements about the versions of each package, so ensuring your environment matches these constraints can oftentimes rectify the problem. To elaborate further, you may need to downgrade one or upgrade the other to a version that ensures better compatibility – refer to [ESLint’s compatibility data](https://eslint.org/blog/2020/02/whats-coming-in-eslint-7.0.0#dropping-support-for-node-js-v8x) to find out more about version compatibility.
In terms of the configuration, double-check your .eslintrc.* file. Make sure to appropriately use the ‘dot-notation’ rule under ‘@typescript-eslint’ rules section.
"@typescript-eslint/dot-notation": ["error", { "allowKeywords": true }]
A quote by Rob Pike, one of the creators of Go programming language: “Errors are values; making them part of the language encourages clear code that handles exceptional cases early and without surprise.”
This gives a new perspective on how to treat programming errors. Instead of perceiving them as issues to be avoided, they can be treated informatively leading us to better maintain and improve our code. Just like in this case, troubleshooting and correctly configuring the ESLint error opens up an opportunity for us to analyze and refine our project settings.
Understanding the @typescript-eslint/dot-notation Rule Error
The error message you’re encountering, “Error While Loading Rule @Typescript-Eslint/Dot-Notation”, is typically due to a misconfiguration or unsupported rules in your Typescript ESLint settings. This rule pertains specifically to the enforcement of consistent use of dot notation whenever possible, optimizing the readability and performance of your code.
Let’s carefully deconstruct what this implies:
The “@typescript-eslint/dot-notation” Rule:
The “@typescript-eslint/dot-notation” rule enforces the coding style where properties should be accessed using dot notation (“object.property”) rather than bracket notation (“object[‘property’]”) whenever possible. Consistent use of dot notation can improve code readability and performance.
Invalid Code Example | Valid Code Example |
---|---|
foo['bar'] = 0; foo['bar'] += 1; var x = foo['bar']; |
foo.bar = 0; foo.bar += 1; var x = foo.bar; |
Error While Loading Rule @Typescript-Eslint/Dot-Notation:
The error perception, “Error While Loading Rule @Typescript-Eslint/Dot-Notation”, is most likely because of an incorrect configuration of your ESLint settings or a scenario where certain rules are unsupported by the Typescript parser. Factors that may cause this issue include:
– Outdated versions of eslint or @typescript-eslint/parser
– Incorrect placement of the “@typescript-eslint/dot-notation” rule under ‘rules’ in the .eslintrc file
To troubleshoot this problem, follow these tips:
– Ensure all npm packages related to ESLint and TypeScript linting are updated to their latest versions.
– Check your .eslintrc file. The “@typescript-eslint/dot-notation” rule should be placed in the ‘rules’ section like so:
{ ..., "rules": { "@typescript-eslint/dot-notation": "error", ... }, ... }
As renowned software engineer Robert C. Martin articulates in Clean Code, “Indeed, readability is what makes code maintainable.” In this same spirit, the “@typescript-eslint/dot-notation” rule encourages a stylized approach to dot notation that enhances the overall readability and maintainability of your TypeScript projects.
To explore more about “@typescript-eslint/dot-notation”, you can reference its documentation on the official TypeScript-ESLint GitHub page.
Implementing Correct Syntax to Fix the Dot-Notation Issue
When working with TypeScript and ESLint, it is common to encounter the error message “Error while loading rule @typescript-eslint/dot-notation”. This error usually occurs when you’re trying to access properties on an object using dot notation but this doesn’t comply with the rules specified by TypeScript or ESLint.
So, how do you go about fixing this issue? It is essential to understand some ground details first:
– The `@typescript-eslint/dot-notation` rule enforces the use of dot notation whenever possible which is considered as a good practice in JavaScript since it’s easier to read and write.
– However, there might be scenarios where bracket notation is necessary (for instance when working with property names that aren’t valid identifiers).
Moreover, your aim here should be to make the codebase consistent and easy to maintain. Follow these steps to achieve this:
– Configure the `@typescript-eslint/dot-notation` rule to match your requirements. You could allow both bracket and dot notations, or restrict usage to specifics, based on your project’s needs.
Here’s how to modify the configuration within your `.eslintrc.js` file:
module.exports = {
rules: { '@typescript-eslint/dot-notation': ['error', { allowKeywords: true }] }
}
With the above configuration, dot-notation will trigger errors only if properties are accessed with bracket notation when dot notation could have been used.
– Do ensure to align the syntax with coding standards followed in your project. Consistency is key to having a scalable and maintainable codebase.
Keep in mind what Don Knuth has said, **”We should forget about small efficiencies, say about 97% of the time: premature optimization is the root of all evil. Yet we should not pass up our opportunities in that critical 3%.”** It means that your first concern should be to write code that’s clean and readable than obsessing over small efficiencies.
For additional refresher on ESLint rules, you can also refer to the ESLint Rules Documentation.. Stay consistent with which notation you use throughout your codebase and follow best practices outlined in the project guidelines.
Identifying Common Errors with @typescript-eslint/dot-notation Rule
The
@typescript-eslint/dot-notation
rule can sometimes cause errors during loading in various JavaScript and TypeScript projects. This rule enforces the consistent use of dot notation whenever possible. Dot notation is often preferable because it’s shorter, easier to read, and easier for development environment auto-complete tools to work with.
However, when this rule fails to load, it could be due to several reasons:
1. Inappropriate use of plugin:
Note that
@typescript-eslint/dot-notation
belongs to the typescript-eslint package. If your project doesn’t require TypeScript but you’re using this ESLint plugin, it might throw an error as such:
typescript
Error: Error while loading rule ‘@typescript-eslint/dot-notation’
To fix this, switch from
@typescript-eslint/dot-notation
to a relevant JavaScript ESLint plugin.
2. Invalid configuration:
Improperly setting up the ESLint configuration file (either
.eslintrc.js
or
.eslintrc.json
) might cause this problem. Ensure the
"extends"
property includes
'plugin:@typescript-eslint/recommended'
, and the
"plugins"
array contains
'@typescript-eslint'
.
3. Outdated dependencies:
If your project’s dependencies are not up-to-date, they could interfere with how ESLint and its plugins operate. Regularly updating these dependencies – especially ESLint and the Typescript ESlint package – can help avoid errors associated with the dot-notation rule.
Utilize online resources like [ESLint documentation](https://eslint.org/docs/user-guide/configuring) and [TypeScript-ESLint docs](https://github.com/typescript-eslint/typescript-eslint) for comprehensive understanding and debugging strategy of ESLint rules.
In the words of Brian Kernighan, one of the developers of Unix:
> “Debugging is twice as hard as writing the code in the first place. Therefore, if you write the code as cleverly as possible, you are, by definition, not smart enough to debug it.”
This implies that sometimes taking a step back and keeping your coding and debugging process simple and straightforward might just be the key to solving problems like loading errors with the
@typescript-eslint/dot-notation
rule.
Best Practices for Debugging in TypeScript ESLint
Troubleshooting the `@typescript-eslint/dot-notation` error in TypeScript ESLint involves a few best practices crucial for optimizing your debugging process, maintaining code quality, and ensuring efficient performance. This error generally surfaces when object properties are accessed via square bracket notation rather than dot notation, which is contradicting with the preferred methods of accessing such properties.
Dot Notation | Square Bracket Notation |
---|---|
const value = obj.property; |
const value = obj["property"]; |
Here’s a dive into practical techniques that can assist you to rectify this variant of TS Lint error:
1. Review ESLint Rules: This `@typescript-eslint/dot-notation` error underlines an issue with one of the specific rules of linting. By default, ESLint enforces the usage of dot notation whenever possible. Understanding these fundamental concepts can illuminate why such an error might have occurred. You may refer carefully to the official ESLint documentation for more insights.
2. Use IDE Linting Tools: Many Integrated Development Environments (IDEs) offer embedded linting tools. Using these features could present real-time feedback on TypeScript syntax and highlight potential errors as you’re coding – thus speeding up the bug resolution process with quick warnings and recommendations.
3. Examine ESConfig files: The usual suspect behind an unexpected linting rule error is the related ESLint configuration file. Investigate the `.eslintrc.config` settings in your project root directory. Ensure the `@typescript-eslint/dot-notation` rule isn’t unintentionally turned off or has invalid values set—adjust as necessary.
4. Utilize the `–fix` option: For some linting errors, including this one, ESLint’s command-line interface provides a convenient `–fix` option. This oprion can automatically resolve certain types of issues, converting bracket notation to dot notation.
eslint --fix path/to/your/typescript/file.ts
5. Tweak Linting Rules: In some circumstances, it might be viable to modify the relevant ESLint rule directly through your configuration file, especially if the error is in compliance with your coding standards or if you prefer bracket notation for access to object properties.
rules: { "@typescript-eslint/dot-notation": "off" }
Before you tweak any rules, remember that each one exists for a reason – they’re aimed at enhancing code readability and limiting potential syntactical mistakes. As per Robert C. Martin, well-known software engineer and co-author of Agile Manifesto, “Indeed, the ratio of time spent reading versus writing is well over 10 to 1. We are constantly reading old code as part of the effort to write new code… [Therefore,] making it easy to read makes it easier to write.” Thus, stringent adherence to best coding practices is key to successful projects.
Remember that resolving TypeScript ESLint errors like `@typescript-eslint/dot-notation` necessitates an analytical approach combined with profound understanding of coding standards and guidelines. Happy Coding!
The error while loading rule @typescript-eslint/dot-notation often crops up in TypeScript, a statically typed superset of JavaScript. This has become increasingly prevalent among developers seeking to enhance their script’s reliability and readability. But like any tech-related endeavor, it can sometimes present unexpected challenges – like the one at hand.
While working with TypeScript, encountering the @typescript-eslint/dot-notation error is considerably common, leading to much frustration amongst developers. Notably, this happens when integrating ESLint into a TypeScript project. Understanding this issue first requires one to dig deeper into the concepts of TypeScript, ESLint and dot-notation which are:
1. TypeScript:
TypeScript encodes additional static type information into your JavaScript projects, enhancing legibility and decreasing potential for bugs.
2. ESLint:
ESLint is a pluggable and configurable linter tool for identifying and reporting on patterns in JavaScript aimed at maintaining code quality.
3. Dot-notation:
In JavaScript, objects allow you to encapsulate related data and functions. Furthermore, Object properties can be both a simple string key or a computed value, accessible through dot notation or bracket notation respectively.
Understanding the association of these components, we’re more capable of navigating the issue. The typical cause of an ‘Error while loading rule’ could be due to some missing dependencies, incorrect configuration, or wrong versions of packages. The optimal solutions would have to include ensuring the @typescript-eslint/parser and @typescript-eslint/eslint-plugin are installed correctly, inspecting the configuration files (.eslintrc) for any errors and finally accessing the right version of the respective plugins/packages.
Staying alert about evolving technologies and persistently striving for improved code quality is crucial in our tech-centric world, where every byte counts. As Andrés Ortiz says, “Be patient, persistent and don’t get frustrated with errors. Take them as opportunities to learn something new.”
Furthermore, coding is about learning to fail better and knowing there’s always a solution out there.
Reference:
1. TypeScript
2. ESLint
3. JavaScript dot notation vs bracket notation