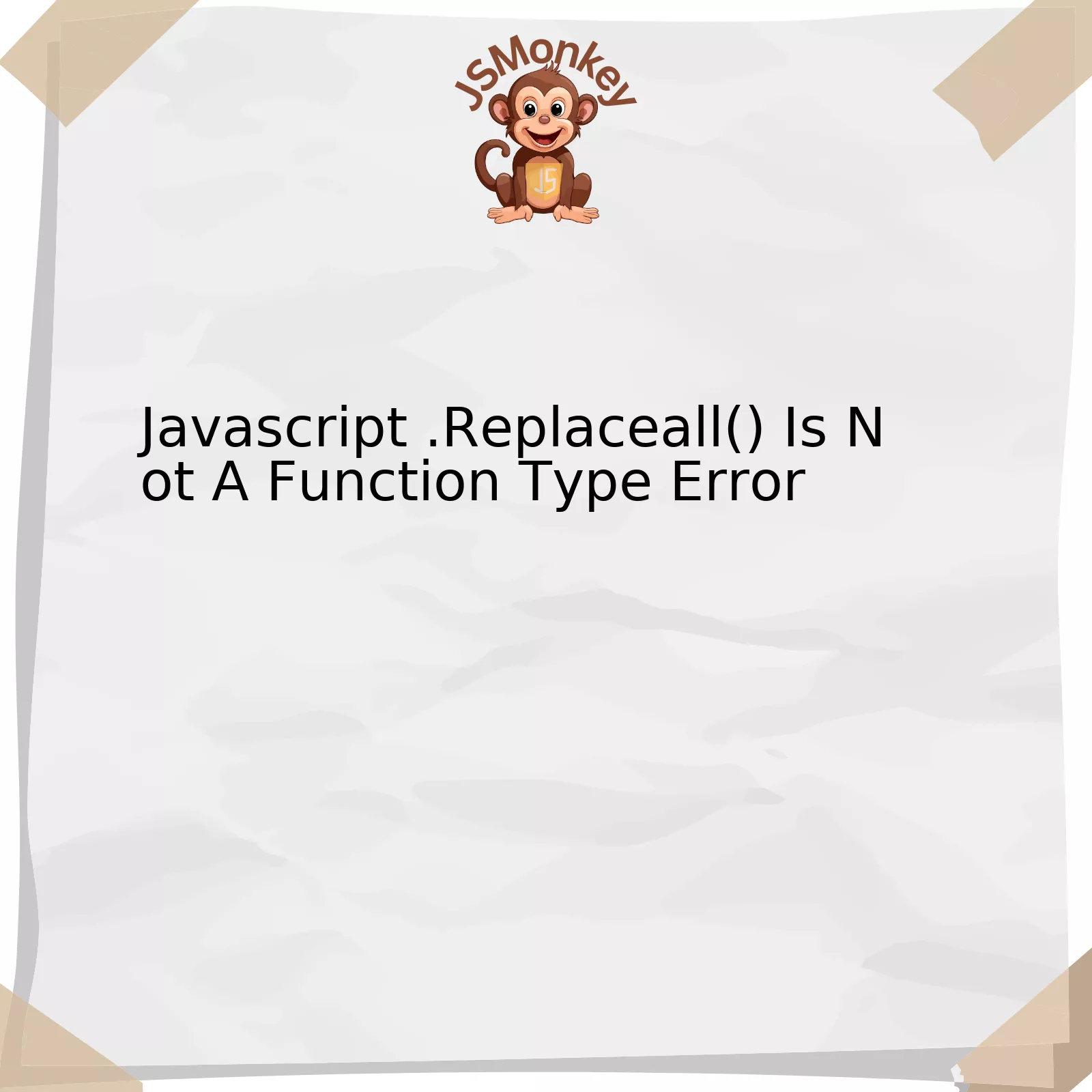
Aspect | Description |
---|---|
Error Type | ‘Javascript .Replaceall() Is Not A Function’ Error |
Likely Cause | The ‘.replaceAll()’ function is relatively new and might not be supported in all browsers or JavaScript environments. |
Potential Solution | Opt for using ‘.replace()’, coupled with a global flag (g). |
When it comes to working with strings in JavaScript, one of the main tools at your disposal is the `.replace()` method. This has been a long-standing part of the language’s utility belt. However, there’s a newer kid on the block that offers a more intuitive way of achieving similar results – the `.replaceAll()` method.
The `.replaceAll()` method is incredibly helpful as it allows developers to replace all occurrences of the specified substring. That being said, this leads us to an unfortunate situation faced by programmers unfamiliar with the quirks of the JavaScript programming language: encountering what is known as the “JavaScript .Replaceall() is not a function” type error.
As alluded to previously, not all browsers or JavaScript environments support the `.replaceAll()` method. If you’re trying to use it in an unsupported context, JavaScript would return an error notifying you that `.replaceAll()` is not a function.
On the brighter side of things, however, there exists a tested and trusted workaround for this quandary. With the good-old `.replace()` function in our arsenal, we can still achieve the same result. The trick lies in supplementing this with the ‘global’ flag, written as ‘g’. This modifier tells JavaScript to apply the replacement globally throughout the string, imitating the behavior of `.replaceAll()`.
let str = "I love apples. apples are my favorite fruit."; str = str.replace(/apples/g, 'oranges');
In this example, every instance of ‘apples’ in our string gets replaced with ‘oranges’. It may not have the simplicity or the expressiveness of `.replaceAll()`, but it gets the job done in all JavaScript environments.
“Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” – Martin Fowler. This quote quite aptly summarizes the essence of this discussion. No matter which method you choose, aim for clear, readable code. That’s a fundamental aspect of good programming more important than any method or function.
Understanding the “JavaScript .replaceAll() is Not a Function” TypeError
The
.replace()
and
.replaceAll()
methods are commonly used in JavaScript to replace certain patterns or characters within a string. However, you may run into a situation where you are trying to use the
.replaceAll()
method and end up encountering an error: “JavaScript .replaceAll() is Not a Function”.
This TypeError usually surfaces due to one of two reasons:
* The JavaScript engine your environment utilizes doesn’t support the
.replaceAll()
method
* You’re applying the method on an incorrect data type which doesn’t possess the
.replaceAll()
function.
Let’s explore these reasons in depth:
Javascript Version Compatibility
If your JavaScript version doesn’t support
.replaceAll()
, this error can occur. This method was introduced with ES2021 (also known as ES12), and many environments including older browsers or server-side JavaScript technologies might not have implemented it.
To resolve this issue, consider using the
.replace()
method combined with a global Regular Expression (RegEx) as shown below:
let string = 'Hello all! How are all of you?'; let newString = string.replace(/all/g, 'everyone'); console.log(newString); // logs: 'Hello everyone! How are everyone of you?'
In this example,
/all/g
is a RegEx representing the pattern to be replaced globally throughout the string.
Incorrect Data Type
Another possibility triggering this error could be executing the
.replaceAll()
method on a data type that it isn’t intended for. This method is designed to operate on strings. It won’t work on numbers, arrays, objects or null/undefined values.
Here’s how this could occur:
let nonString = 12345; let newNonString = nonString.replaceAll(1, 2); // TypeError: nonString.replaceAll is not a function
To fix the error in this case, it would be essential to first convert the data type to a string before employing the
.replaceAll()
method.
The ‘JavaScript .replaceAll() is Not a Function’ TypeError may seem intimidating at first glance but understanding its triggers and the alternate available solutions makes it easily manageable.
Maintaining sync with the latest version of JavaScript can help avoid some issues while careful selection of methods based on the data type you are working with eliminates others. As Brendan Eich, the creator of JavaScript once said, “Always bet on JavaScript.” [Online Reference](https://www.goodreads.com/quotes/9165188-always-bet-on-js), indicating faith in the language’s robustness and versatility. Understanding the nuances of various JavaScript functions would only reinforce this belief.
Exploring Solutions to Correct the “.ReplaceAll() Is Not a Function” Error in JavaScript
Noted for its versatile aptitude and necessity in today’s digital framework, JavaScript is a scripting language primarily used to enhance and manage web page interactivity. Despite its importance, developers often encounter common technical impediments that impede smooth program execution. One recurrent issue producers face involves the error produced when
.replaceAll()
is invoked and JavaScript responds with – this is not a function.
Firstly, an understanding of the replaceAll() function in JavaScript is requisite. The
.ReplaceAll()
method in JavaScript is employed to replace all occurrences of a specified string value. An example of its usage can be seen below:
html
In this instance, the
.replaceAll()
function changes ‘blue’ to ‘black’, creating: “A black moon, under the black sky.”
Our first dive into solving this problem investigates compatibility or rather, lack thereof. At the time of writing,
.replaceAll()
is a rather new addition to JavaScript and as such lacks complete browser coverage. Therefore, it’s plausible that on older JavaScript engines or some browsers, you may receive the “.Replaceall() Is Not A Function Type Error” warning because said browsers do not support such functionality.[source](https://caniuse.com/mdn-javascript_builtins_string_replaceall).
One option available to bypass this hindrance utilises the global regular expression inclusive of the ‘g’ flag. This renders an outcome almost identical to using
.replaceAll()
. Here’s how it would look:
html
With this method, every occurrence of “blue” gets replaced with “black”, in spite of the absence of
.replaceAll()
in your JavaScript engine. This solution proves reliable as
.replace()
maintains support across all JavaScript engines and browsers.
Other than compatibility issues, another instance in which you might get a “.Replaceall() Is Not A Function Type Error” message is when trying to use
.replaceAll()
on something that isn’t a string.[source](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/String/replaceAll). The
.replaceAll()
function strictly processes strings. Thus, ascertain any datatypes manipulated by this function are string objects or contain convertible values.
Dedicate attention to the code below:
html
This will output “.replaceAll is not a function” because replaceAll() doesn’t operate on numbers. To rectify this, convert the number into a string first:
html
Finally, remember as Paul Irish, a Google Chrome developer once said, “I love fixing performance issues, and I’m lucky, ’cause there’s no shortage of them.” Therefore, it’s ok to come across performance issues, what matters is how we tackle it with the tools at hand.
Common Scenarios Leading to “.ReplaceAll() Is Not a Function” Errors in JavaScript Code
The JavaScript “.ReplaceAll() Is Not a Function” error is one that developers commonly encounter. If you experience this issue, you are most probably trying to invoke the
.replaceAll()
method on an object that doesn’t possess this attribute. Here’s a closer look at some common scenarios and reasons why this error may arise:
A. The Object Does Not Have The Method ReplaceAll
JavaScript is an object-oriented scripting language that treats almost everything as an object. But every object does not necessarily have all the methods or properties. For instance, the replaceAll function is native to JavaScript string objects, but not number or boolean objects. Therefore if you call
.replaceAll()
on a variable of a different type (other than strings), then the “this is not a function” error will occur.
For example,
let num = 567; console.log(num.replaceAll(5,"2"));
will throw an error because the replaceAll function hasn’t been implemented for numeric data types.
B. Older Version of JavaScript
The
.replaceAll()
method on string is relatively new in JavaScript, implemented in ES2021. Hence, if you’re working with older versions of JavaScript, or other programming environments that don’t support ECMAScript 2021 standards, it won’t recognize this feature resulting in a TypeError.
C. You’re Using An Older Web Browser
Similar to previous point, your web browser too needs to keep up with modern JavaScript developments. A lot of older or less popular browsers lack full-fledged support for ECMAScript 2021. Thus, running a script involving ReplaceAll() might lead to unexpected errors.
In order to overcome these issues, we can consider a few alternatives:
• Use ‘.replace()’ with a Regex
The
.replace()
method along with the global flag in a regex can serve as an effective substitute. This ensures it works on older versions of JavaScript and browsers that don’t yet support ES2021.
Sample code:
'.example.com'.replace(/\./g, "-")
.
• Use ‘split()’ and ‘join()’
Another alternative involves using
.split("
) and
.join()
to replace all occurrences of a substring:
Code example:
'www.example.com'.split('.').join('-')
While .replaceAll() is a robust feature addition to JavaScript, handling its compatibility issues is crucial for ensuring our codes work across various platforms pertinent to different contexts of usage.
To borrow the words of Addy Osmani – Google engineer & the author of “Learning JavaScript Design Patterns”, “Don’t reinvent the wheel…unless you plan on learning more about wheels”. In challenging times, finding alternate solutions to programming problems not only helps solve the immediate issue but also extends your mastery over the coding universe.
How Upgrading your Java Version can Resolve the “.ReplaceAll()” Type Error
In the realm of code development, dealing with type errors and finding solutions is a common part of the process. When you come across an error like “Javascript .Replaceall() is not a function”, it’s important to understand both the nature of the issue and how to effectively mitigate it.
First and foremost, allow me to present some necessary context. The “Javascript .Replaceall() is not a function” error usually arises when the Javascript method
.replaceAll()
, which serves to replace all instances of a certain substring with another in the given string, is used in an environment where it isn’t recognized. This is typically the case with older versions of Javascript that don’t support this particular method.
Despite the confusion, Java and JavaScript are fundamentally different as they run on different environments and have distinct syntaxes and semantics. However, drawing parallels between them could offer insightful perspectives. One analogous situation in Java would be attempting to use a method unsupported by your current version, thereby causing a type error. Upgrading your Java version in that case would resolve the problem.
Transposing this idea back into JavaScript environment:
- In JavaScript, the
.replaceAll()
method was introduced in ES2021 and is supported in higher ECMAScript versions (eg. Chrome 85+, Firefox 77+ and so forth).
- If you’re running into the “.ReplaceAll() is not a function” error, that’s a clear signal that your environment isn’t compatible with the ECMAScript version employing this function.
- The most straightforward fix in this context would involve upgrading your JavaScript environment to a newer one that supports
.replaceAll()
.
However, if upgrading isn’t feasible or practical due to various constraints, there’s a commonly used alternative: the
.replace()
method in conjunction with a global regular expression. Here is a sample usage:
let string = "Hello, world!"; let newString = string.replace(/o/g, 'a');
In the aforementioned code snippet, all instances of ‘o’ have been replaced with ‘a’ in the target string, pretty much emulating the function of .replaceAll().
As Bill Gates once said: “Software innovation, like almost every other kind of innovation, requires the ability to collaborate and share ideas with other people”. By extension, understanding the origin of type errors and their solutions would surely come easier through collaborative efforts within the coding community.Computerworld
Staying relevant to `Javascript .Replaceall() is not a Function Type Error`, it’s clear that countless developers have experienced and addressed this issue. This error arises due to the non-implementation of the `replaceAll()` method in all environments in which JavaScript runs, particularly those using ECMAScript versions older than ES2021.
The main issue with the `replaceAll()` function is its compatibility. To be more specific, this method is relatively new as it was added to the ECMA specifications in 2021 with ES12. Consequently, the browsers and Node.js versions that do not support ECMA Script 2021 will not recognize `replaceAll()` as a legitimate function.
To address the `JavaScript .Replaceall() is not a Function Type Error`, there are a couple of techniques at our disposal:
- Utilize the global flag (g) along with the regular expression: Using the JavaScript `replace()` function itself can remedy the situation. The `replace()` function combined with regex (/searchWord/g) globally replaces all instances of a particular string. Replace 'searchWord' with the word you want to replace.
Additionally, it’s pertinent to quote Paul Irish here, a Google Chrome engineer who said, “Always bet on JavaScript.” He succinctly personifies JavaScript development’s dynamism, which resonates with the case of `replaceAll()`. This phrase ultimately emphasizes the importance of staying updated with recent JavaScript changes and adaptation to sudden shifts or learning new methods in an evolving environment.
There are other substitute mechanisms like using a split-join sequence where the string in question is first split into an array and then joined again after making appropriate replacements, but bear in mind the intricacies involved with respect to performance considerations when replacing `replaceAll()`.
Henceforth, while implementing code involving string replacement techniques, logging errors, or debugging, remember the essential tenets mentioned above about the `replaceAll()` method and its incompatibility issue. Don’t let the `Javascript .Replaceall() is not a Function Type Error` deter you from exploring the vast scape of JavaScript capabilities.
Further Information: MDN – String.replaceAll()