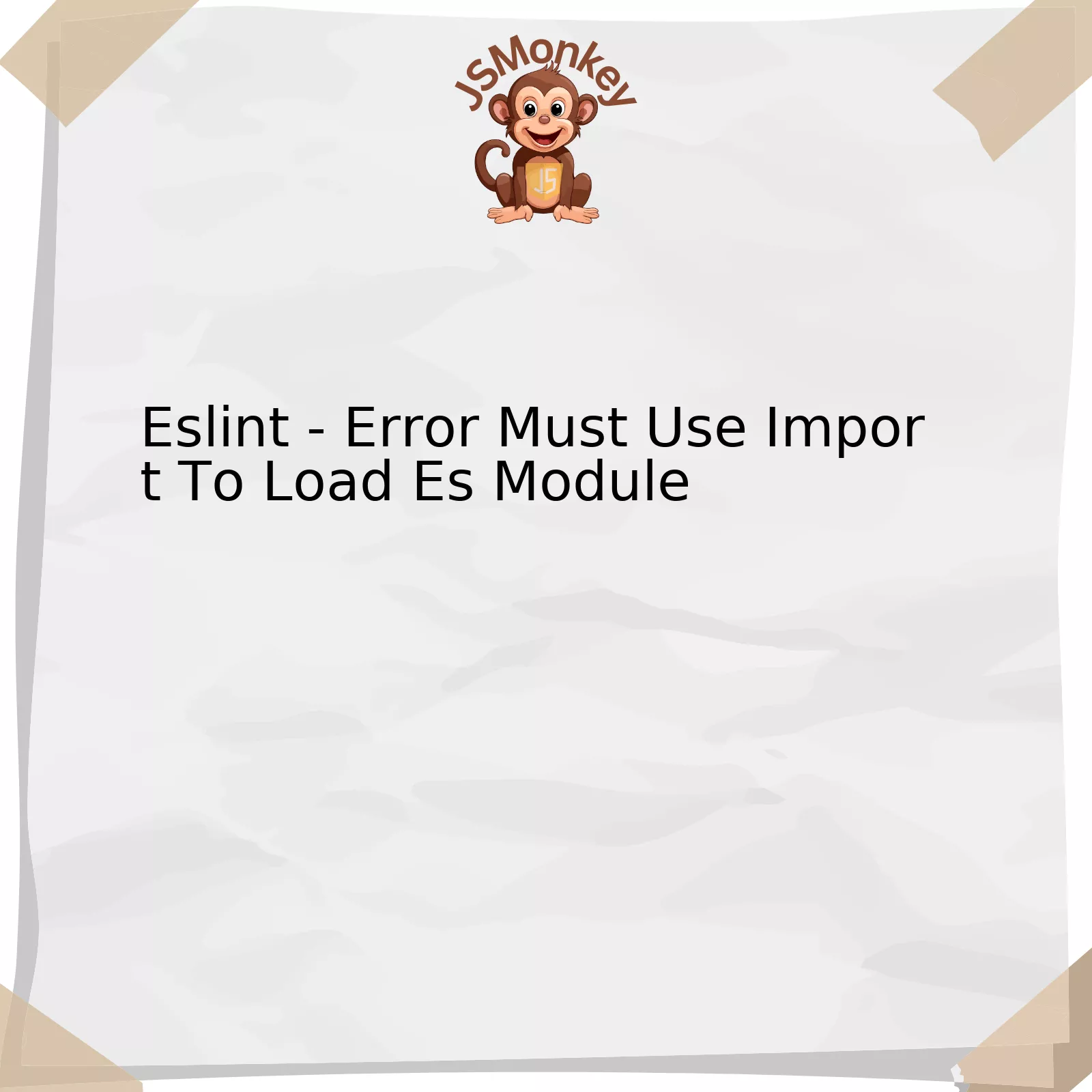
ESLint is a widely-used static code analysis tool that often assists developers in identifying and reporting patterns found in ECMAScript or JavaScript code. The issue, “Must Use Import to Load ES Module,” usually occurs when Node.js tries to require an ES6 module. ES6 modules use ‘export’ and ‘import,’ whereas Node.js uses ‘module.exports’ and ‘require.’
Below find a representation of why this error might occur, showcasing both the correct and incorrect method:
html
Incorrect Method | Correct Method |
---|---|
const pkg = require('es6-package'); |
import pkg from 'es6-package'; |
The first column signifies the incorrect method which generates the ESLint error. The second column illustrates the correct approach. In the first column, Node.js syntax is being used to import ES6 packages, there’s a mix of syntax methodologies, leading to the error.
To mitigate this, the correct way, as shown in the second column, is to employ ES6 syntax for importing ES6 packages.
Node.js does not traditionally support ES6 export/import by default. A common fix to this issue can involve using Babel, a JavaScript compiler that allows you to use next-generation JavaScript today. Babel converts modern JavaScript into versions that are manageable and compatible with most browsers.
To quote Addy Osmani, an Engineering Manager working on Google Chrome, “First do it, then do it right, then do it better.”
Ensure your script is correctly identified as a module in the package.json file by adding, “type”: “module”. This insures your ES6 code is recognized as a module.
Lastly, considering that .mjs files are naturally taken as ES modules by Node.js, you can change your JavaScript file endings from “.js” to “.mjs”
It is necessary to consider various factors when resolving this ESLint warning. The developer must possess an understanding of Node.js and ECMAScript functionalities, their differences and compatibilities. Relevant knowledge of Babel or other compiling tools could also be beneficial. A keen eye for detail in terms of code syntax and structure is key in navigating such stumbling blocks.
Understanding the ESLint Error: Must Use Import to Load ES Module
ESLint is an open-source JavaScript linting utility that helps developers maintain code standards and prevent potential errors in the coding process. One of these concerns is about the way we load ES Module into our application.
Despite being a common point of discussion among professionals, there are still numerous misconceptions when it comes to understanding the ESLint error which states: “Must Use Import to Load ES Module”. This is quite a common error encountered when trying to require() an ES module in Node.js, yet the core of the problem lies within understanding how ES modules function themselves and what makes them different from traditional CommonJS modules.
To tackle this, let’s delve deeper into what ES Modules are as well as the central cause of this issue:
- What are ES Modules: ES Modules (short for ECMAScript Modules) were introduced as a recent enhancement to provide native module support in JavaScript. ES Modules favor a declarative syntax where modules declare their dependencies upfront using import and export.
- The Cause of the Issue: The root of the “Must Use Import to Load ES Module” error is an attempt to use the require() method for importing ES modules instead of the built-in JavaScript import statement. Require() is a part of CommonJS modules system, while ES Modules demand the usage of the import statement.
Now, you might ask, “What’s the difference anyway?” Well, there are a few key distinctions between CommonJS and ES Modules. By default, Node.js operates with CommonJS for its server-side runtime environment. However, the latest versions have started to offer full compatibility with ES Modules.
Therefore, throwing this error is essentially ESLint’s way of informing you that one should use the respective import syntax instead of mixing up your module systems – like so:
import myModule from './myModule.js';
In navigating modern JavaScript development, it is of utmost importance to understand these kind of distinctions. As Martin Fowler, a renowned software engineer, encapsulates it perfectly:
“It’s not enough to write code that works, it’s important to understand why it works.”
Sources:
ESLint Official Site
Node.js ES Modules Docs
Overcoming Challenges with ESLint’s ES Modules Loading Mechanism
ESLint, prevalent among JavaScript developers, is a pluggable linting utility for JavaScript. Despite being an incredible tool, its ES Modules loading mechanism poses challenges that developers stumble upon.
The cause of the ‘must use import to load ES module’ error most commonly lies in how ESLint handles ECMAScript modules. It isn’t suited to handle the npm packages that are strictly ES modules. ES6 introduced the method of handling dynamic imports and exports using `import` and `export` commands, but unfortunately, ESLint doesn’t grasp this concept fully in some cases.
Overcoming this challenge involves several steps:
Steps | Description |
---|---|
.mjs filename extension |
Change your file extension from
.js to .mjs . ESLint readily identifies .mjs files as ES modules. |
Modify package.json | Adding
"type": "module" in your package.json file can aid in ESLint recognizing ES modules. |
Babel | The Babel plugin-transpile-modules may be used to convert problematic npm packages into CommonJS modules, which ESLint can understand. |
esm | Use esm to enable support for ES modules at runtime, enabling you to import npm packages coded as ES modules. |
To quote Douglas Crockford, the discoverer of JSON, “Programmers are notoriously bad at understanding and managing error conditions.” This highlights developers’ perpetual struggle with errors and the importance of tools like ESLint in mitigating these obstacles. Although it may present challenges, adeptly understanding and using such strategies can ultimately provide an efficient and robust workflow in your JavaScript coding environment.
Additionally, don’t forget that every change done on your code after utilizing one of the above-referenced solutions involves testing, either manually or running your Mocha, Jasmine or Jest tests. If there isn’t any improvement addressing the ES modules loading error, consider reporting the bug to the ESLint team.
In-depth Review: Why “Must Use Import to Load ES Module” Errors Occur in ESLint?
The error “Must Use Import to Load ES Module” generally pops up in the ESLint static analysis tool due to a common misunderstanding about how JavaScript modules are loaded and executed. Specifically, it arises when developers try to load ECMAScript (ES) modules using the CommonJS `require()` function, which is incompatible with ES modules.
Here is a brief breakdown on why ESLint throws this error:
– ES Modules vs CommonJS: The fundamental difference between these two module types lies in their loading mechanism. While CommonJS modules allow synchronous loading, meaning they can be loaded during runtime, ES modules are designed for asynchronous loading i.e., files should only be loaded before runtime. As a result, using `require()` to load an ES module, which is a technique meant for CommonJS modules, results in this error.
– Syntax Variation: Another contributing factor to this error is the variation in syntax. ES Modules utilize `import` and `export` functions whereas CommonJS utilizes `require()` and `module.exports`. Therefore, mixing these directives can often lead to confusion and ultimately result in the aforementioned error.
However, it’s important to note that specific configuration settings can ameliorate this issue. For example, enabling the “esm” (ECMAScript modules) setting in Node.js allows the use of `import` to load ES modules, resolving the “Must Use Import To Load ES Module” error.
Information about the esm package can be found via this hyperlink.
Furthermore, there are strategies worth considering:
– Use Babel to compile your code into CommonJS modules. This involves adding a `.babelrc` file to your project setting up babel and making sure any code you write is turned into code node.js can understand.
– Utilize dynamic `import()`, that works asynchronously and can import both ES modules and CommonJS modules.
As foresighted by Robert C. Martin, a renowned software engineer and author, “Indeed, the ratio of time spent reading versus writing is well over 10 to 1. We are constantly reading old code as part of the effort to write new code. …[Therefore,] making it easy to read makes it easier to write.” Proper understanding of JavaScript module systems and implementing them correctly contributes significantly to achieving this writing ease. Always consider compatibility and intended use-case scenarios when choosing which module system to adopt in your application development.
Effective Solutions for Tackling the “Must use import to load ES module” Error
Navigating the realm of JavaScript programming can often lead you down paths of various unexpected errors. One such confounding issue is the “Must use import to load ES Module” error message encountered in ESLint. The fundamental cause of this problem traces back to how Node.js operates, and the difference between CommonJS modules and ES Modules.
The crux of the matter lies in the fact that Node.js inherently uses the CommonJS module format. In contrast, the ECMAScript specification defines the ES module syntax. Consequently, when you use an endeavor to `require()` an ES Module in your Node.js application, it throws an error message—“Must use import to load ES module”.
Solutions
Several effective solutions can salvage this situation and ensure smoother operation:
Applying Babel
Description | Steps |
---|---|
Babel, a prominent JavaScript compiler, can transpile the ES6 modules code into CommonJS format which Node.js can effectively understand. |
|
Switching to ES Modules
Description | Steps |
---|---|
If the project’s scale allows, consider shifting from CommonJS to ES Modules. |
|
Using `esm` Package
If neither of the above options work for your situation, you can opt to use the `esm` package available on npm.
Description | Steps |
---|---|
The “esm” package acts as a successful bridge between CommonJS and ES Modules and allows you to use ES6 syntax without any need for transpilation or modification of existing files. You can thus keep powerful features like dynamic imports or top-level await. |
|
As Kevin Marois (software developer) wisely put it: “Learn to write code for humans. Machines will understand your code regardless”. Coding is an art of problem-solving, and although tackling errors like the “Must use import to load ES Module” issue can be daunting, once we comprehend the root cause, the solution paves a path of rewarding growth and enhanced knowledge. And remember, each bug defeated equates to evolution in coding skills!
Related Links
Understanding ES6 Modules via SitePoint
Babel Preset Env Official Documentation
Addressing the issue of ‘Eslint – Error Must Use Import To Load Es Module’ requires a comprehensive approach, focusing on the understanding and application of ES modules in JavaScript practices. An ES module is a static module system for linking files together, and ESLint errors generally occur when there is an incorrect configuration present.
Commonly, these errors surface due to inconsistencies between environments that understand ES modules and those which do not. This discrepancy can lead to miscommunication between different code files resulting in the problem at hand. It’s important to remember that each JavaScript runtime or environment utilizes modules differently.
To effectively mitigate this type of error in your ESLint configuration, consider the following steps:
– Ensure that you have the type: “module” in your .js files. When using ES modules, this is necessary. If this is in place, Node.js will treat .mjs files as ES modules by default.
– Adjust your ESLint settings. In addition to the type: “module”, you might have to include “sourceType”: “module” in your ESLint configuration file as well.
Coding example for the second point(just a portion of eslint.rc.js):
module.exports = { parserOptions: { sourceType: 'module', }, }
These measures help JavaScript runtimes to correctly interpret ES Modules, resolving the ‘Eslint – Error Must Use Import To Load Es Module’. Ultimately, problems like these enhance our understanding of versatile languages like JavaScript. As JavaScript guru Douglas Crockford once said, “JavaScript is the world’s most misunderstood programming language, but it becomes more understandable when we dig down to its root behaviors and start from there.”
Sources For Further Study:
MDN Web Docs – JavaScript Modules,
ESLint – Configuring ESLint