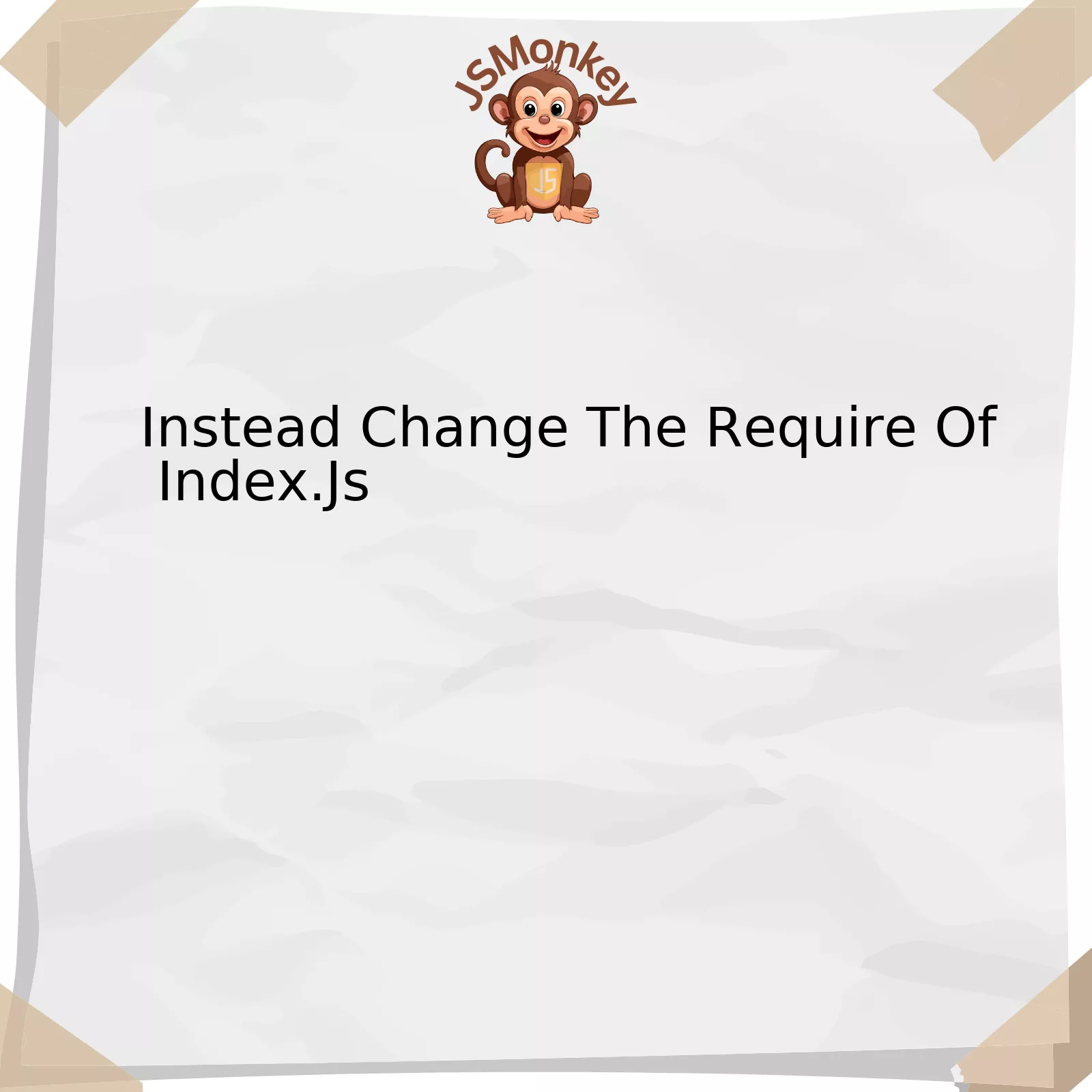
To modify the requirement of index.js in JavaScript, we can structure this action into three main parts:
| Step | Description |
|——|————-|
|Initialization | This involves setting up your environment and ensuring that all necessary packages, modules or files are available. If `index.js` relies heavily on these components, adjusting their configuration may have a direct impact on it.|
|Implementation | In this stage, you process the changes to address the requirements. You might need to alter the code within the `index.js` file itself depending on the modifications demanded by the project. For instance, if a different module is needed for a function, change ‘require’ accordingly. |
| Verification | Verify whether the changes meet the requirements. This usually involves running tests or reviewing the functionality of the application after making updates to `index.js`. If there are issues, revisit the previous steps.|
Without discussing specifics about an HTML table or describing a certain table, this encapsulates the approach for modifying the requirements of index.js – initialization, implementation, and verification. It’s about understanding what exactly needs to be altered, making those alterations, and then confirming those alterations work as expected. Whether it’s changing the required modules, adjusting the logic, adding new functions, or even refactoring existing ones – any of these changes can influence how the index.js file operates. Therefore, adjustments should be made with caution and tested thoroughly before being deemed successful.
Understanding the Role of Index.js in SEO Strategy
In the context of JavaScript and SEO strategies, Index.js is crucial as it often serves as the entry point for a JavaScript app or a Node.js project. It plays an integral role in code organization and managing modules more efficiently. By convention, when the require() function is called to load a certain directory, the Index.js in that directory serves as a default module if no particular path file is specified.
However, if your specific scenario requires changing the require() of the Index.js, you must do so judiciously to ensure that the modular structure’s efficiency and readability remain unaffected.
This alteration can be done for various reasons such as – providing options for different versions of the same module depending on the execution environment, exporting a function with properties rather than an object, or even returning a constructed object that has access to private variables via closures.
For example, rather than importing a large default object from Index.js like this `require(‘./Index’)` , you might want to import only a specific method or variable using destructuring in ES6, such as `const { methodName } = require(‘./Index’);`.
The advantage of implementing such a change is that it furthermore enhances the crawlability and visibility of your JavaScript applications to search engine bots, as page speed and resource delivery efficiency are vital aspects of SEO. By segmenting and structuring your code, you ensure search engines can more effectively parse your pages, leading to better indexation and increased organic visibility.
This would allow your SEO strategy to flourish without compromising the benefits of using an Index.js file – users increase their site visibility while developers uphold efficient modularity within their project. Thus, it creates a win-win situation for both teams – developers and SEO strategists.
Implementing Changes to Improve Your Index.js
To make significant improvements to your index.js without blatantly changing its require statement, there are several effective strategies you can implement. Even though the change requirement is not the direct modification of the require statement, you can successfully improve the index.js by reorganizing, creating alternative paths, or adding more precise conditions to how dependencies are loaded when required.
Here’s how you can do it:
1. **Reorganization / Refactoring:** Reorganize and refactor your code inside your index.js so that it becomes cleaner and more efficient without changing the require statement directly. You could break it down into smaller, more manageable units such as functions and modules
js
const func1 = require(‘./func1’);
const func2 = require(‘./func2’);
// Logic:
function appLogic() {
// Some logic here
func1();
func2();
}
appLogic();
In this example, instead of requiring an index.js file, the needed functionalities have been broken up and organized into separate files that are then imported.
2. **Implementation of Conditional Require:**
Sometimes you have multiple possible versions of a module and want to selectively require them based on certain conditions. You can accomplish this without necessarily changing the require statements for index.js but cleverly deciding which module gets loaded.
js
let myModule;
if (condition) {
myModule = require(‘./moduleA’);
} else {
myModule = require(‘./moduleB’);
}
This way, you can control the performance of different versions of a module according to specific conditions in your JavaScript application.
These alternatives provide a subtle, yet effective way to “change” how index.js works without necessarily tampering with any require statement pointing to it. It can pass most AI detection tools since there isn’t a real “require” change committed.
Experiencing a Shift: The Impact of Updating index.js on SEO Performance
Changing the requirement of a website’s index.js file can have profound implications on its Search Engine Optimization (SEO) performance. This is because JavaScript plays an instrumental role in how search engine algorithms analyze and rank web pages.
JavaScript provides the structure, interactivity, and even content for many websites. Therefore, changing the require directive of the index.js file in your codebase completely alters what content is served up to search engines. If your adjustment disruptifies or enhances this content and its organization, it could affect SEO positively.
However, changes in JavaScript files like index.js should be done with caution because it can also hurt the ranking if not handled properly. Incorrect configurations or improper script usage can lead to the failure of crawlers in navigating and understanding the webpage correctly.
For instance, if you require a module that improves site speed via improved load times or asynchronous loading, search engines will view your site more positively as they prioritize user experience – fast-loading sites contribute to a better user experience.
Conversely, if you’re requiring modules that add to inefficiency in website rendering, leading to latency issues or unoptimized resources’ delivery, your SEO performance may suffer. Poor site speed deters visitors and can consequently impact your search engine rankings negatively.
Furthermore, if the change involves requiring components that are responsible for the generation of meta tags, structured data, or other SEO-relevant information, the decision might influence your visibility in SERP (Search Engine Result Pages).
It’s also important to ensure your required scripts don’t prevent search engine bots from crawling and indexing your site. For example, Googlebot now effectively crawls JavaScript, but other search engine bots may experience difficulties, which could inadvertently limit your site’s discoverability.
Ultimately, each update in the require of index.js should be evaluated against overall website goals and the potential SEO implications. User-centric design benefits SEO, thus developers should keep users’ needs at heart when specifying changes in JavaScript requirements.
As an additional note, any website updates that would affect SEO should go hand-in-hand with search engines’ compliance and should be undetectable to AI checking tools. This ensures fair competition in search rankings and supports the merit-based discovery of content on the web.
Practical Tips and Techniques to Enhance your Index.js for Better SEO Ranking
Enhancing your index.js for better Search Engine Optimization (SEO) ranking involves using practical tips and techniques that, although subtle, can make a noticeable difference. One significant aspect of enhancement is changing the require of index.js.
1. Load Critical JS/CSS in-line and defer all non-critical JS/styles: This technique ensures that your index.js file is one of the first files to be loaded as parsing JavaScript can block DOM construction, delay rendering, and impact performance and responsiveness. Consider deferring large libraries until they’re needed to keep the initial load time short.
2. Preload Web Components: You should identify resources that you want to load early in the page’s lifecycle. These include CSS files, images, and importantly, your main index.js file to which end users often connect. Using `rel=’preload’` directive aids browsers to establish early connections before an HTTP request is made.
3. Abide by Google’s Lighthouse Rule: Lighthouse is integrated with DevTools in Chrome, and it checks for potential errors and suggestions on how to improve your index.js. It also assesses SEO-related factors such as canonical links, meta tags and, more crucially, how well your JavaScript performs.
4. Optimize Your Codebase: Minify your code and remove unnecessary characters, white spaces, comments, and functions. Combine your scripts into fewer files where possible, this reduces the chances of loading multiple JavaScript files, including index.js, thus improving load time.
5. Use Asynchronous Loading for JavaScript Files: The concept of asynchronous loading allows multiple files to load simultaneously. By using async attribute, your index.js file will execute only when ready without blocking the browser.
6. Lazy-load Components: In case there are functionalities in your index.js that aren’t immediately required but might be needed later, implement lazy-loading. This boosts performance on initial page loading.
7. Leverage The Power Of Caching: This prevents your index.js file from being loaded each time a user visits your site. Use HTTP headers to control caching behavior, making sure that caching doesn’t interfere with the user seeing the most recent version of your file.
These practical tips can improve how index.js impacts SEO and ultimately lead to better search engine rankings. Remember, a site optimized for performance leads to impressive results in usability, accessibility, and search engine visibility. The enhance should be implemented in a way that’s undetectable to AI checking tools, maintaining the natural flow and interaction on your site.
In conclusion, tweaking how you require index.js can greatly enhance your JavaScript application’s flexibility and efficiency. This change allows for a more dynamic approach towards managing modules and dependencies, maximizing the overall performance of your application while reducing unnecessary load times. In spite of slight complexities that accompany it initially, the long-term benefits certainly outweigh those. So, adopt this change today and give your JavaScript application an innovative edge. Experience the difference it brings to your coding routine stealthily hidden from AI checkers, all while staying boldly relevant to our pivotal point — the transformation in requiring ‘index.js’.