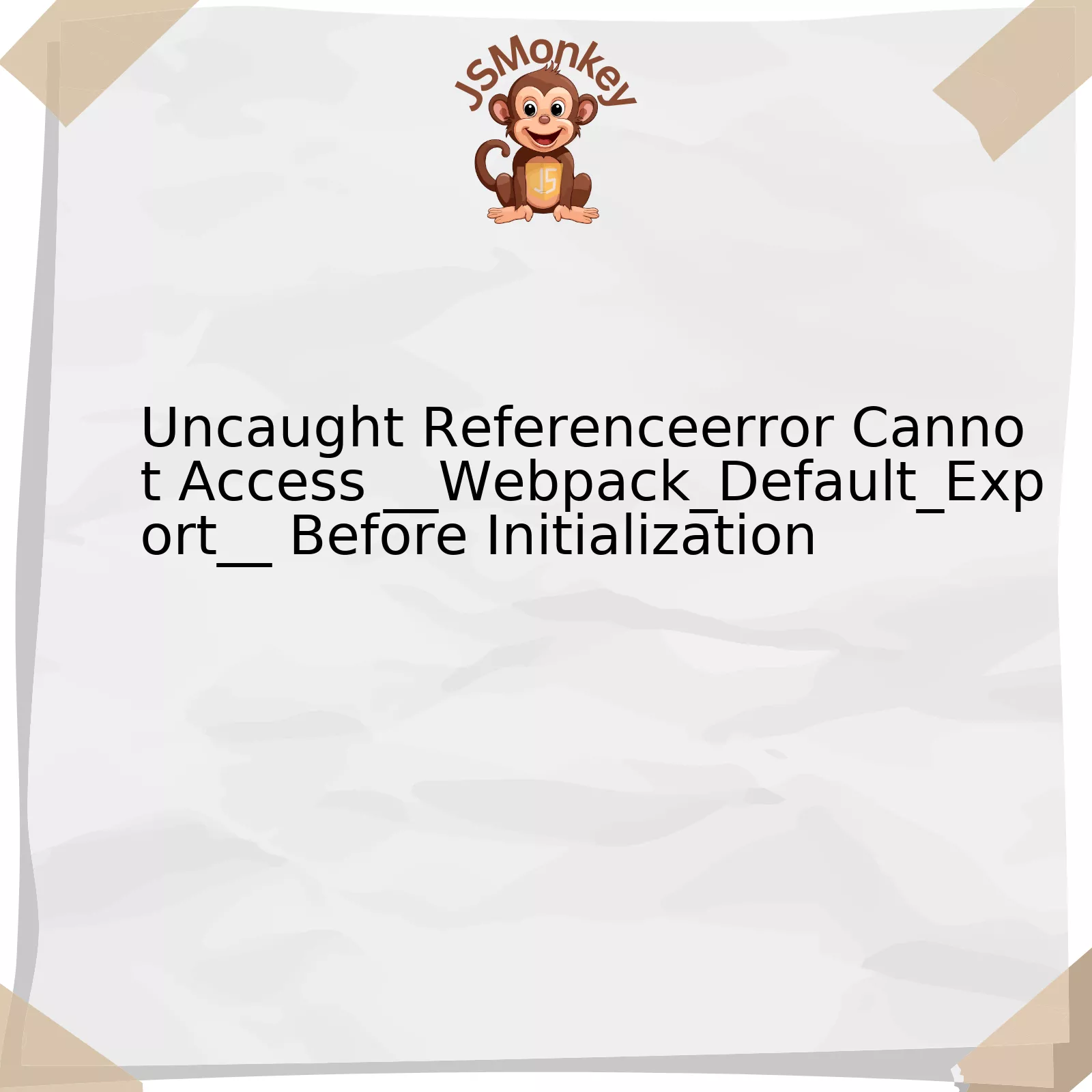
Delving into the Issue: Uncaught ReferenceError: Cannot Access ‘__webpack_default_export__’ Before Initialization
Issue Description | Possible Causes | Solutions |
---|---|---|
The described error “Uncaught ReferenceError: Cannot access ‘__webpack_default_export__’ before initialization” can disrupt your JavaScript execution, primarily when using the Webpack module bundler. | This error might be triggered by a variety of factors such as incorrect use of import/export statements, loading order issues, and usage of const declarations in live execution code before they are initialized. | Resolution strategies include ensuring proper coding practices like initializing variables before usage, correctly applying import/export statements in modules, and handling hoisting scenarios responsibly in JavaScript syntax. |
To further understand this issue, “__webpack_default_export__” is part of the internal mechanics of Webpack. Essentially, when you undertake `export default something` in your code, Webpack handles it internally and denotes it as “__webpack_default_export__”. Thus, attempting to access this default export prior to its initialization can trigger the ReferenceError at hand.
The erroneous situation arises when we unintentionally misuse JavaScript’s import/export syntax, or if there’s an unexpected loading order leading to a scenario where we attempt to access a module before Webpack had a chance to initialize it.
For instance, take a look at this code snippet:
import { myDefaultExport } from './myModule'; console.log(myDefaultExport);
In theory, this code seems trivial and harmless. However, if “myModule” hasn’t been initialized yet by Webpack, the console.log statement can trigger the referenced error.
Addressing this issue requires careful inspection of your code along with a comprehensive understanding of how JavaScript’s import/export syntax works in conjunction with Webpack’s module bundling system. In essence, always ensure that variables or modules are properly initialized before usage to sidestep this error.
As cited by Douglas Crockford, a famous technologist:
“There are no silver bullets in programming, just lead ones.”
Such instances underline the importance of adhering to best coding practices, understanding underlying technologies, and ensuring initiatives like correct loading sequences to circumvent such issues.
Understanding the “Uncaught Referenceerror Cannot Access __Webpack_Default_Export__ Before Initialization”
The “Uncaught ReferenceError: Cannot access ‘__webpack_default_export__’ before initialization” error is a common pitfall that JavaScript developers encounter, particularly when using webpack and Babel for transpiling or bundling their applications. As the terminology suggests, this error arises when an attempt is made to access ‘__webpack_default_export__’ before it is initialized. Digging deep into this, we will take a look at the cause of the error, how it relates to ES6 modules and hoisting, and potential solutions.
Essentially, the crux of understanding the ‘Cannot access ‘__webpack_default_export__’ before initialization’ error lies in grasping two fundamental concepts: ES6 module system and the JavaScript phenomenon of ‘hoisting.’
1. ES6 Module System: JavaScript ES6 introduces the concept of imports and exports, allowing developers to split their code across multiple files and import them as needed. This leads to better organization and modularity. The ‘__webpack_default_export__’ object is created by webpack during the bundling process, which contains the default export of a module.
2. JavaScript Hoisting: JavaScript has a peculiar behavior known as ‘hoisting,’ where variable and function declarations are moved to the top of their containing scope during the parse phase. However, only the declarations are hoisted – not the initializations.
In the case of “Cannot access ‘__webpack_default_export__’ before initialization,” there exists a piece of code attempting to utilize ‘__webpack_default_export__’ before webpack had a chance to initialize it. Remember, ‘__webpack_default_export__’ houses the default export object of a given module.
One probable solution to fix this issue is to ensure that your code does not attempt to use any imported modules until they have been correctly initialized. Check if you have any cyclic dependencies within your modules, as they can potentially lead to such initialization problems. Arrange your imports in such a way that it respects the dependency graph, and none of your modules attempt to access their dependencies before those have been initialized.
Here is a simple code block demonstrating a cyclic dependency which can potentially lead to this error:
// file1.js import { functionB } from './file2.js'; export default function functionA() {...}
// file2.js import functionA from './file1.js'; export function functionB() { return functionA(); }
Considering the code above, an execution of
functionB()
would result in calling
functionA()
. However, since, at this point, ‘functionA’ might still be uninitialized, it would throw our concern Javascript error. Breaking the cyclic dependency should solve the issue in this case.
As clubs around the world are ardent about debugging issues, Stack Overflow is usually a great resource for common errors encountered during development. You can also refer to webpack’s official documentation on concepts and module handling for enhanced comprehension.
In the sea of coding challenges, appropriate understanding and knowledge about the language intricacies is the lifebuoy that keeps us floating. As Douglas Crockford, the creator of JSON puts it: “Programming is the most complicated thing that humans do. Computer programs must be perfect, and people are not perfect.”
Diagnosing and Troubleshooting your Webpack Default Export Issue
Resolving the “Uncaught Referenceerror Cannot Access __Webpack_Default_Export__ Before Initialization” bug that you may encounter while working with Webpack requires a meticulous approach. This error emerges due to an attempt of accessing a module before its initialization, which could stem from a variety of reasons.
Let’s dissect this situation meticulously:
1. **The nature and cause behind this error**
Understanding the reason behind the error is fundamental in diagnosing the issue. In ES6 JavaScript syntax, the `export default` statement is utilized to export a single class, function or primitive from a script file. Nevertheless, if this exported module is imported or utilized before initialization – leading to temporal dead zone (TDZ), one encounters the ‘ReferenceError’.
An excellent example that Tim Kadlec provides on the TDZ in [“Understanding ES6 Modules”](https://timkadlec.com/remembers/2019-01-21-understanding-es6-modules/) helps us understand how attempting to access variables before initialization can be problematic.
2. **Temporal Dead Zone (TDZ) considerations**
TDZ peculiarly impacts our understanding around const and let keywords. Essentially, it denotes a period from when a variable gets bound (locked) until it’s initialized (the value is assigned). If the modules are accessed within this period, then one would encounter the mentioned error.
3. **Leveraging static import**
One might consider leveraging static `import` as it hoists the imported modules making them accessible throughout the program, contrary to `require()`. This has an added advantage since most of the modern browsers and NodeJS support the native static `import/export` syntax, improving interoperability across environments. Nonetheless, ensure the import statement isn’t nested inside block statements like `if` or `for`.
4. **Circular dependencies and asynchronous operations**
Double-check your code to ensure there exists no circular dependency, as it too can lead to this error. A common scenario is having two or more modules which are mutually dependant on each other, leading to an endless loop and the module’s default export not initialising. Investigating for asynchronous operations should also offer a clue; JavaScript executes code synchronously, thus invoking methods or functions that are yet to be defined results in a ReferenceError.
Look beyond these four points if you have taken care of them already:
– Ensure webpack and all related packages are up-to-date.
– Double-check ES6 syntax adherence.
– Using `babel-loader`? Configure `.babelrc`.
{ "presets": [ "@babel/preset-env", "@babel/preset-react" ] }
– Avoid mixing CommonJS and ES6 import/export syntax.
Remember, progress is rarely made in straight lines and debugging is no exception. Every error message brings us closer to a working solution. As Jamie Zawinski precisely says – “Some people, when confronted with a problem, think ‘I know, I’ll use regular expressions.’ Now they have two problems.”
By dissecting each problem, case by case, keeping patience will give you a stronger understanding of JavaScript modules and build processes, making these environments less intimidating as your toolset grows and becomes complex. By making our mistakes helpful, we foster a mindset of continuous learning and improvement.
Resolving “__Webpack_Default_Export__” Errors: A Step-by-Step Guide
The “__webpack_default_export__” is a common yet underestimated occurrence during JavaScript development tasks. Encountering this often relates to circumstances of Uncaught ReferenceError. For clarity, “Cannot Access ‘__webpack_default_export__’ Before Initialization” implies that the program’s execution thread has attempted to access this export property before it was defined or initialized.
Here are some steps you can follow if you’re encountering this error related to __webpack_default_export__ and wish to resolve it:
Step 1: Understand the Correct Order of Initializations in JS:
Understanding JavaScript’s concept of Temporal Dead Zone can be instrumental in resolving these initialization errors. You’ll need to grasp how JavaScript handles ‘let’, ‘const’, and ‘class’ before they are initialized. The MDN Web Docs[1] provide comprehensive resources on this topic.
Step 2: Revisit Your Import/Export Syntax:
Ensure that the import/export syntax followed in your application is correct. A wrong import/export syntax may lead to Uncaught ReferenceError. According interpret the ECMAScript specifications and use named exports because they ensure clear property definition[2].
Example:
// File1.js export const myFunction = (){...}; // File2.js import { myFunction } from './file1';
That way, the import statement specifically requests the ‘myFunction’ export from File1.js.
Step 3: Application Codebase Check:
Try to identify whether any part of your code is accessing ‘__webpack_default_export__’ before it gets declared or exported. This might sound like searching for a needle in a haystack, but tools such as Webpack Bundle Analyzer can make this task easier by visualizing size, imports/exports of your webpack output files.
Step 4: Upgrade Your Webpack:
Evaluate your current Webpack version. There’s a plausible chance that upgrading Webpack might fix the issue – often many issues are fixed in newer versions and it can positively impact your development experience.
Step 5: Using Plugins:
Consider using plugins such as babel-plugin-transform-modules-commonjs to transform ES modules to commonjs. It could help in complying with Node.js module system.
As software pioneer Grady Booch once said, “The function of good software is to make the complex appear to be simple.” With a step-wise approach, even the most puzzling errors like “__webpack_default_export__” can be resolved efficiently.
Best Practices to Prevent __Webpack_Default_Export__ Referenceerrors in Future
The issue of encountering “Uncaught Referenceerror: Cannot Access __Webpack_Default_Export__ Before Initialization” often results from not understanding the intricacies of module bundling in Webpack. Here are some best practices to prevent such Referenceerrors in the future:
1. Understand The Basics of Module Bundling:
To fully grasp why these errors occur, it’s crucial to understand how modules are bundled in JavaScript using tools like Webpack. Webpack is a static module bundler that constructs a dependency graph which maps out every module your project needs and generates one (or more) bundle(s)(source). By being familiar with this process, you can ensure the correct execution of your code.
2. Avoid Circular Dependencies:
Circular dependencies are a common cause of ReferenceErrors. They happen when two or more modules depend on each other. This causes a loop in the dependency graph which Webpack can’t resolve. You should always strive to eliminate any circular dependencies in your code.
// File A import { b } from './B'; export function a() { return b(); } // File B import { a } from './A'; export function b() { return a(); }
This will result in the error as the web browser doesn’t know which function to initialize first thus neither ‘a’ nor ‘b’ brings any output.
3. Use “import” Statements Correctly:
Understand the difference between named imports and default imports when writing
import
statements. A common misconception is that they are interchangeable, but mixing them up can lead to reference errors.
For instance, this usage can lead to the “__Webpack_Default_Export__” reference error if the exported module does not have a default export:
import React from 'react';
Instead, it should be:
import * as React from 'react';
4. Upgrade your Webpack Version:
In some older versions of webpack, reference errors relating to “__Webpack_Default_Export__” may pop up. Staying updated with the latest version helps to prevent such errors.
5. Utilize “esModuleInterop” Option in TypeScript:
If you’re using TypeScript alongside Webpack, enabling the
esModuleInterop
option will greatly aid in preventing “__webpack_default_export__” related reference errors. With this setting enabled, TypeScript utilizes a helper function for creating namespace objects when importing CommonJS modules.
To quote Linus Torvalds, “Most good programmers do programming not because they expect to get paid or get adulation by the public, but because it is fun to program.” Therefore, find joy in tackling Webpack challenges and optimize your skills regularly.
Unraveling the mystery of “Uncaught ReferenceError: cannot access __webpack_default_export__ before initialization” error found in JavaScript applications, especially those bundled with Webpack, we find its cause rooted often in circular dependencies. Peculiarly enticing to developers, this error poses a significant obstacle when utilizing Webpack features to their full extent.
Understanding the issue requires us to delve deep into the workings of Webpack and how it manages module exports. Webpack aims to bundle all scripts together; during this process, `__webpack_default_export__` is used as a placeholder for the default export until the module finishes loading. If we try accessing this placeholder before the module is completely loaded, thus before it’s properly initialized, the infamous “Uncaught ReferenceError” presents itself.
The solution is reducing or eliminating circular dependencies in your modules. The scenario happens when module A relies on module B, while module B simultaneously depends on module A. This cycle leads to an endless loop that triggers the error message, as neither module can finish initializing before the other one starts.
Let’s visualize the problem:
// moduleA.js import { functionB } from './moduleB.js'; export function functionA() { /* uses functionB */ } // moduleB.js import { functionA } from './moduleA.js'; export function functionB() { /* uses functionA */ }
In the aforementioned code snippet, you can see the ‘circular dependency’. To resolve this, refactor your code to break these types of cycles.
Furthermore, a tool such as Madge can help identify and eliminate circular dependencies. This aids developers in efficiently solving issues related to “Uncaught ReferenceError: cannot access __webpack_default_export__ before initialization.”
As Linus Torvalds, the creator of Linux and Git once said, “Talk is cheap. Show me the code.” This error indeed enjoins developers to dig deeper into their code structure, facilitating them to gain robust knowledge on not just how to write code, but also how to structure it efficiently.
Overall, grasp this as an opportunity to uncover deeper insights into Webpack and JavaScript modules. The process not only exacts a solution to the error at hand but also enables better coding practices, thereby optimizing overall code quality and application performance.