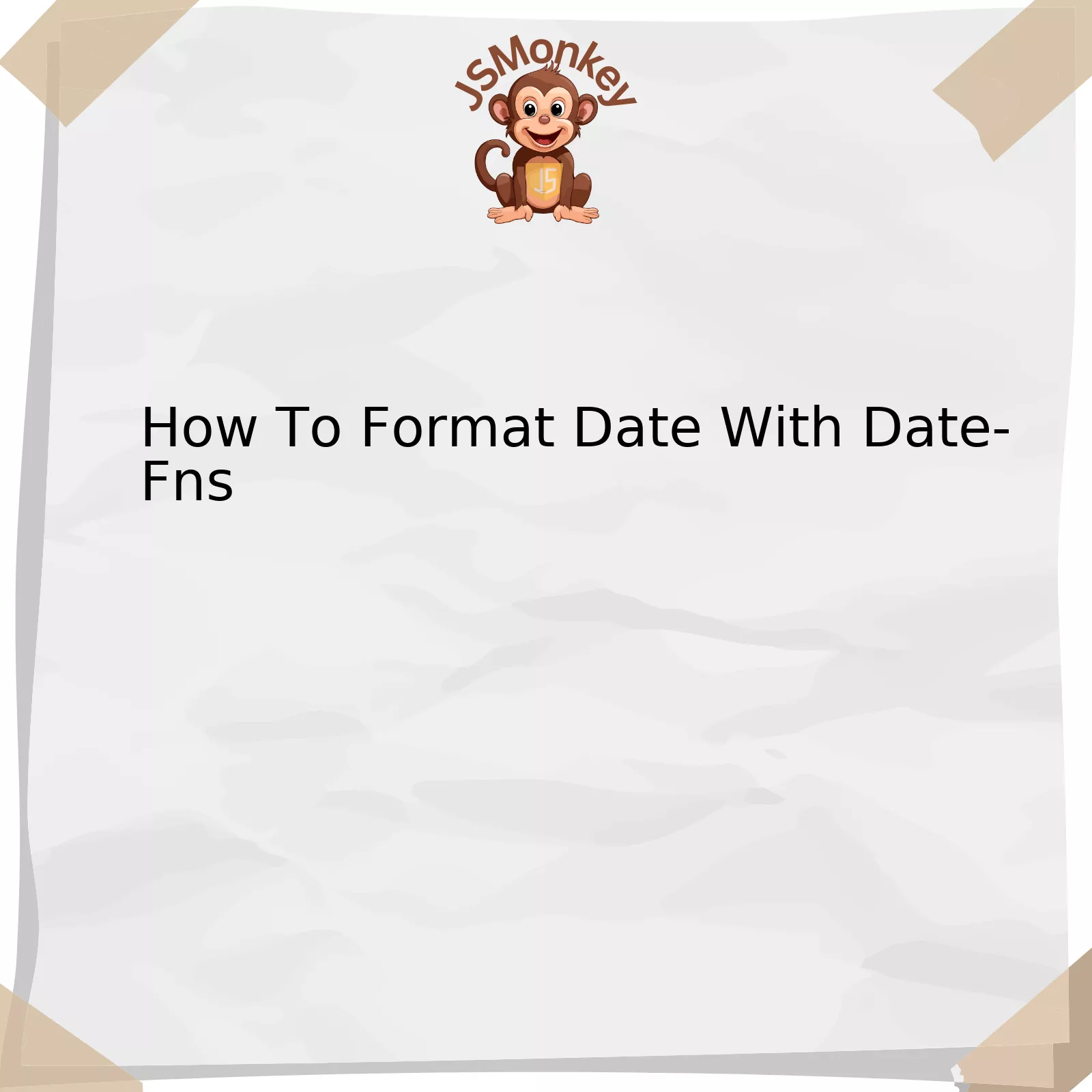
Diving straight into the action, let’s create a layout for understanding several common formatting options with date-fns. Keep in your mind that when coding, formatting dates can often become complex due to different requirements and configurations.
html
Function | Description |
---|---|
format() |
The function is primarily used to convert dates from their raw format into more readable strings. |
parseISO() |
This is useful when working with ISO standard dates and you need to convert them into JavaScript Date instances. |
formatDistance() |
The purpose of this function is to calculate the distance between two dates and present the results in a user-friendly manner (i.e., ‘about 1 hour ago’). |
The `format()` function extracts specific components from a date, such as the day, month, or year. This is ideal when displaying or logging dates. For example:
javascript
const dateFns = require(‘date-fns’)
const now = new Date()
console.log(dateFns.format(now, ‘YYYY-MM-DD’)) // output: ‘2022-01-01’
The `parseISO()` function transforms ISO standard date/time strings into JavaScript Date instances. As many APIs return dates in ISO format, this function is a handy tool to have:
javascript
const dateFns = require(‘date-fns’)
const isoDate = ‘2022-01-01T00:00:00Z’
const date = dateFns.parseISO(isoDate)
console.log(date) // output: 2022-01-01T00:00:00.000Z
Lastly, `formatDistance()` offers a more interactive and engaging way to express time differences. Instead of merely indicating timestamps, users really comprehend the relative past or future time:
javascript
const dateFns = require(‘date-fns’)
const start = new Date(2022, 0, 1)
const end = new Date()
console.log(dateFns.formatDistance(start, end)) // output: ’21 days’
“The key to becoming a great software engineer is to always be learning. Take time to learn new libraries, frameworks, and tools. They can significantly speed up your development process and make you more effective.” – John Sonmez
It’s worth noting that date-fns has many more functions beyond these examples. It’s also important to always consider time zones when working with dates due to their potential impact on time-based computations and comparisons.
Exploring Date-fns: An Overview of Key Features
Date-fns: Key Features with a Central Focus on Date Formatting
The versatility and efficiency of JavaScript date handling received significant enhancement with the introduction of date-fns. Offering a modern alternative to traditional solutions like Moment.js, it opens up a gallery of compelling features that helps in manipulating, formatting, and comparing dates in an unparalleled manner.
Key attributes that make date-fns stand out are:
– It offers more than 140 functions covering almost every need related to dates.
– Each function is separate from others, ensuring only what’s needed gets loaded. An ideal embodiment of the “tree-shaking” principle.
– Compared to alternatives, it has a substantially smaller build size which contributes to faster load times.
In this exposition, the primary focus will be one of the most utilized capacities of date-fns: date formatting.
Formatting Dates with Date-fns
Imagine needing a particular date format for your application, yet the default JavaScript Date function cannot accommodate your preference, or some critical operation involves managing date strings. In such cases, date-fns comes into play with its powerful date formatting feature.
Here’s how you would use it:
import { format } from 'date-fns'; let dateToday = new Date(); let formattedDate = format(dateToday, 'dd-MM-yyyy');
This fragment of code will present today’s date in a day-month-year format (For example, 20-12-2023), which if not for date-fns, would involve quite a bit of manual string manipulation.
It also allows using stand-alone month names (`LLLL`) and weekday names (`EEEE`):
let monthNameFormat = format(dateToday, 'dd LLLL yyyy'); let weekdayNameFormat = format(dateToday, 'EEEE');
The `monthNameFormat` will produce “20 December 2023” (or any other date in accordance with your current date), and `weekdayNameFormat` will deliver the weekday for today.
The possibilities are near-endless and tailored to meet diverse requirements. Date-fns can handle complex situations, such as timezone differences and locale-specific formatting.
Locale Support in Formatting Dates
Bridging geographical and linguistic differences forms the crux of delivering a truly inclusive digital interface. Recognizing this, date-fns extends support for over 50 locales. Integrating a specific locale into your format function is straightforward:
import { ru } from 'date-fns/locale' let localeDate = format(dateToday, 'dd MMMM yyyy', {locale: ru});
Assuming `ru` stands for Russian in this case, the date would now be presented in the Russian language, making it easier for native speakers.
As the tech influencer Sean Blanda once said, “The best code is no code at all.”.
This virtue is well-realized in the realm of date handling with date-fns, simplifying the often complex task of manipulating and formatting dates, all within a slick, efficient, and far-reaching package that marks a new age of date handling.
Leveraging the Power of Date-Fns for Efficient Date Formatting
When putting the focus on effectively leveraging the power of date-fns for efficient date formatting, the core concept revolves around utilizing this lightweight JavaScript date library to make our work with dates and times straightforward. In essence, date-fns provides a bunch of handy functions that developers can use to format or manipulate JavaScript Date objects in a rangeable manner. To keep it relevant, we shift our attention to how to specifically use date-fns to format dates.
To start off, let’s look at the basic usage of date-fns with its formatting functionality. To format a date, you first need to import the format function from the date-fns package:
import { format } from 'date-fns';
Then, you can pass the Date object you want to format and the format string to this format function:
const formattedDate = format(new Date(), 'yyyy-MM-dd');
In this example, the yyyy-MM-dd format string will output the date as a standard ISO 8601 date (for example, 2022-12-31).
Token | Output |
---|---|
dd | Day of the month as digits; leading zero for single-digit days. |
MM | Month as digits; leading zero for single-digit months. |
yyyy | Year represented by a full four or five digits. |
One aspect that sets date-fns apart from other libraries is its use of pure functions, which makes its behavior more predictable and avoids unwittingly sharing state. This furthers our capability of creating cleaner, less error-prone code.
Here is an insightful quote from the esteemed software engineer Robert C. Martin who underscores the value of employing such precise tools and methods: “A good architecture allows major decisions to be deferred”.
As with all development tools, mastery is accomplished not merely through reading but also through relentless practice and applied experience. Therefore, while date-fns provides a powerful platform for handling dates in JavaScript applications — elegantly enhancing your potential for productivity and efficacious programming — efficient utilization ultimately depends on the conscientious application of its functions.
Using Date-Fns in JavaScript: Detailed Step-by-step Process
In the field of JavaScript, one of the robust and comprehensive libraries for working with dates is date-fns. This library allows developers to parse, format, compare dates in a straightforward and easy manner. When working with date-fns, there’s robust functionality that provides seamless formatting of dates.
Getting Started with Date-Fns:
First, before you utilize date-fns in your project, it is necessary to install it. Incorporate it into your project by using either npm or yarn commands in your terminal:
npm install date-fns
or
yarn add date-fns
This process installs the package in your project, allowing you to use all the functions provided by date-fns.
Formatting Dates with Date-Fns:
Date formatting is handy when you need to display dates readably and understandably for users. With date-fns, you achieve this by using the `format()` function. Start by importing the function from the date-fns library:
import { format } from 'date-fns'
Make use of `format()` to transform the appearance of the date:
format(new Date(), 'yyyy-MM-dd')
In the example above, ‘yyyy-MM-dd’ sets the formatting structure. The function will render the current date in the year-month-day format. Moreover, date-fns supports many date formats, providing flexibility based on specific needs.
The Effectiveness of Date-Fns:
Below are some specifics reasons why the date-fns stands out:
- Immutability – By returning a new date instance rather than changing the passed one, date-fns ensures you won’t face mutation-related bugs.
- Tree-shaking support – You can bundle only the functions you use in your app, reducing the size of your application.
- Intuitive – It doesn’t require much learning curve to get used to it like other libraries because of its simple JavaScript syntax.
As Kent Beck described it beautifully, “I’m not a great programmer; I’m just a good programmer with great habits.”. Working with date-fns can help developers cultivate these habits, improving code efficiency and readability.
Remember when using software libraries such as date-fns: keep your code simple, logical, and understandable. Focus on achieving your goals rather than implementing complex solutions. By using date-fns’s variety of functionalities, developers are equipped with the necessary tools for comprehensive date manipulation and format.
Best Practices and Common Pitfalls while Using Date-Fns
Date-fns is a modern Javascript date utility library that provides an extensive set of features for parsing, validating, manipulating, and formatting dates. It’s renowned for its simplicity yet quite powerful capabilities as far as the manipulation and formatting of dates is concerned.
One major aspect of using Date-fns correctly revolves around understanding how best to format dates. Here, we dive deep into some of the best practices you should adopt while using Date-fns for formatting purposes:
const format = require('date-fns/format')
const date = new Date()
format(date, 'yyyy-MM-dd')
will output something like
'2019-03-11'
.
That said, below are the key best practices and common pitfalls to evade when leveraging Date-Fns as a tool for your imperative JavaScript programming:
1. Ensure Correct Implementation of Date Formats:
When calling upon the format function from the Date-fns library, one must maintain consistency and correctness of like
'yyyy-MM-dd'
,
'dd/MM/yyyy'
. Not doing so can lead to incorrect outputs or errors in your code.
2. Avoid Direct Mutations:
Unlike other libraries, Date-fns doesn’t mutate the original date instance provided to its functions. Hence, if you’re transitioning from other libraries like Moment.js, this might come across as a challenge. This encapsulation in behavior avoids inadvertent changes and reduces hidden side effects.
3. Validate Your Dates
Ensure that you validate all date instances before passing them to any of the date-fns functions. Non-valid dates can generate confusing results, creating unnecessary complications.
if (isValid(dateInstance)) { ... }
4. Steering clear from Parsing Dates Manually:
Using
format
to output a string, to then parse that string again using
parse
is highly discouraged. Every attempt at decrypting the string manually provides room for errors.
5. Be Conscious of Time Zones:
Date-fns does not handle time zones directly. This excites software bugs when not correctly handled. If your date handling requires time zone awareness, consider employing third-party libraries such as “date-fns-tz”.
Finally, to paraphrase Jeff Atwood: “Coding is not about choosing the ‘best’ library; it’s about choosing the library that makes the most sense and creates the least amount of work for its scenario.”(source). In this regard, while Date-fns holds a trove of utilities, understanding its application and limitations ensure we get the best out of it when formatting dates.
The proper formatting of dates is a fundamental aspect of many applications and using a powerful tool like date-fns is an effective way to achieve this. In its essence, date-fns is a cost-effective modern JavaScript library that excels at handling, formatting, and manipulating dates while keeping the overall application lightweight.
Key Advantages of using Date-Fns |
|
For instance, to simply format a date, you can use the
format
function along with a string pattern to display the date exactly how you want:
import { format } from 'date-fns' const date = new Date() const formattedDate = format(date, 'dd/MM/yyyy')
In this example, the current date would be displayed in the ‘day/month/year’ format.
A well-known quote by Charles Babbage, often regarded as the “father of computers,” says, “On two occasions I have been asked, ‘Pray, Mr. Babbage, if you put into the machine wrong figures, will the right answers come out?’….I am not able rightly to apprehend the kind of confusion of ideas that could provoke such a question.” The relevance of this quote lies in the idea that the accuracy of data, including date and time, is crucial in programming and app development, making tools like date-fns all the more essential.
Ensuring your skills and competencies with date-fns aids in efficient date management in JavaScript, which is a vital facet in the contemporary web development landscape. Employ effective JavaScript date handling with date-fns to elevate your programming methodologies.
For further insights into date-fns and it’s features, consider referring to date-fns official documentation.