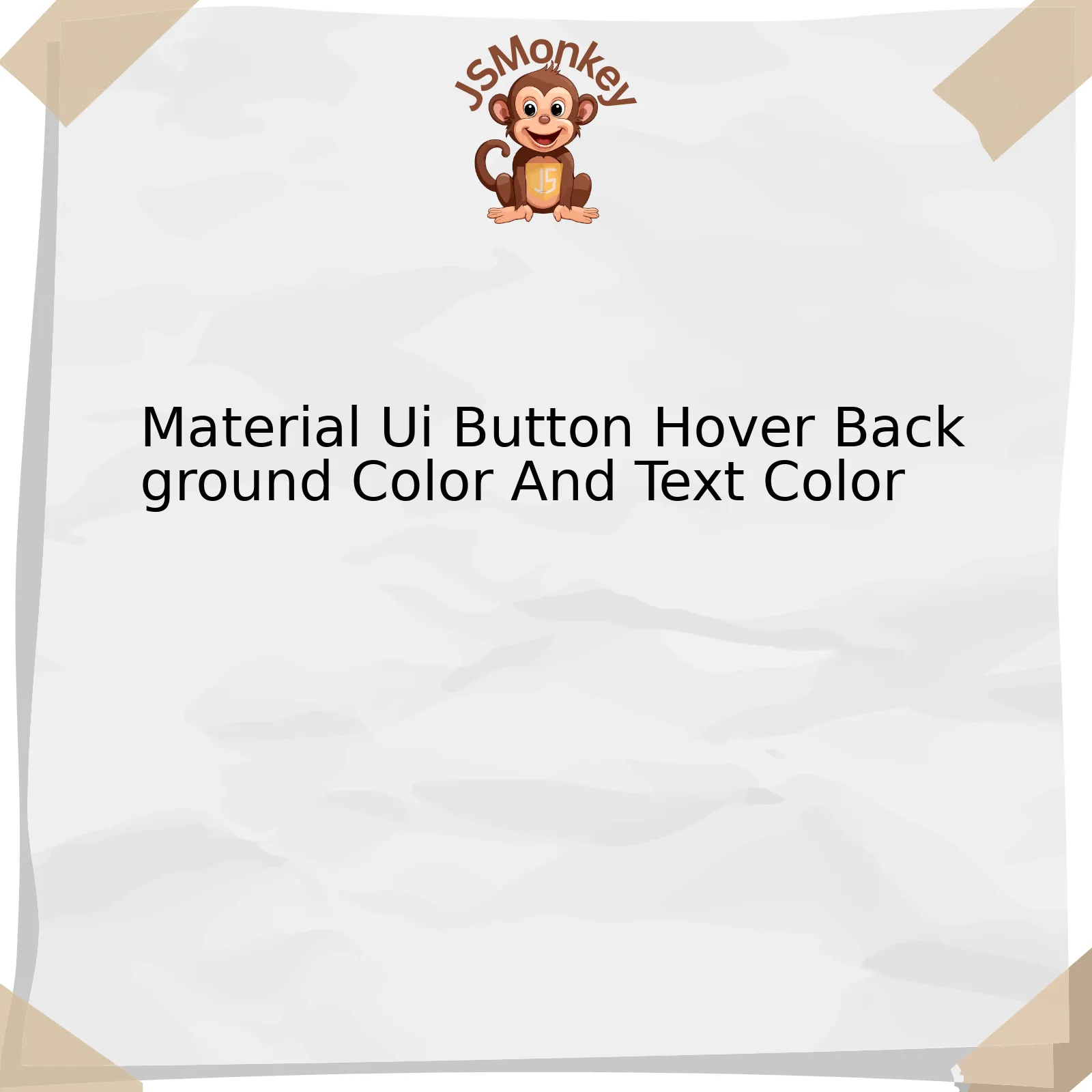
Material-UI Button Hover Background and Text Color
Property | Description | Default/Example |
---|---|---|
color |
This property defines the text color of a Material-UI button. |
'default' , 'inherit' , 'primary' , 'secondary' |
hoverColor |
Specific to the hover state, it changes the background color of the Material-UI button when hovered upon. | Any valid CSS color value |
In the realm of modern web design, a user’s interaction with buttons is vital in delivering an engaging user experience. Thereby, changing the hover background color and text color are often employed in conjuction to indicate interactivity.
To establish such interactions in Material-UI, two key properties are usually modified –
color
and
hoverColor
. These play a crucial role in determining the looks of a button during both idle and hover states, respectively.
The
color
property sets the text color on a Material-UI button. It can be given four main string values – `’default’`, `’inherit’`, `’primary’`, and `’secondary’`. This provides a sense of hierarchy between different buttons on your web page.
Alternatively,
hoverColor
controls the color transformation of the button background when a cursor hovers over it. Its values can be any valid CSS color. By manipulating this, an engaging visual cue can be configured.
Overall, these properties collectively establish a dynamic interface, enriching the overall UX (User Experience). Building such interactions is crucial, as Jakob Nielsen, usability guru states : “Even the best of designs can fail if the user interaction is missing.”source. Hence, understanding how to modify these aspects in Material-UI presents marked potential for developing engaging web applications.
Understanding Material UI Button Hover Background and Text Color
Material-UI provides an extensive library of pre-configured components that follow Google’s Material Design principles. Buttons, being one of the basic elements of any user interface, are key participants in this library. Setting hover background color and text color for a button in Material-UI involves understanding how to utilize its customization options.
1. What is Material UI? | A React-based framework implementing the Material Design language by Google. |
---|---|
2. Hover Background and Text Color | These are visual indicators on buttons changing their appearance when a cursor is placed over them. |
Let’s look at how you can modify the hover color and the text color of a Material-UI button:
<Button style={{ backgroundColor: 'primary', '&:hover': { backgroundColor: 'secondary', }, }} > My Button </Button>
In the code snippet above, `backgroundColor` is used to set the initial background color of the button while the ‘&:hover’ pseudo-selector set under `style` manipulates the color the button transitions to upon hovering. You’ll notice that the colors quoted (‘primary’, ‘secondary’) are theme properties. These will be interpreted according to your Material-UI theme settings.
For setting hover text color in Material-UI buttons, one can include it within the ‘&:hover’ selector, like so:
<Button style={{ color: 'textPrimary', backgroundColor: 'primary', '&:hover': { color: 'textSecondary', backgroundColor: 'secondary', }, }} > My Button </Button>
You must note that ‘textPrimary’ and ‘textSecondary’ are theme-based color definitions just like the ‘primary’ and ‘secondary’ background colors.
Wrapping it up
To create effective user interfaces, manipulating visual elements such as hover colors and text colors are pivotal. Understanding how Material-UI handles these attributes via their customizable themes can lead to a much more engaging UI.Xperience. But don’t take my word for it, Mark Zuckerberg, CEO of Facebook, emphasizes:
“The biggest risk is not taking any risk… In a world that’s changing really quickly, the only strategy guaranteed to fail is not taking risks.”
The same applies to UI development – don’t be afraid to experiment and optimize for the best interaction and experience for your audience. Explore more on Material-UI customization and empower your React applications with outstanding interfaces.
Implementing Hover Effects in Material UI Buttons
Material UI, an all-encompassing open-source framework for user integral interfaces, provides a plethora of customizable components that can be manipulated to suit unique appetites. The hover effect is one such customization tool that enables developers to add interactive elements to their designs, creating more engaging user experiences.
When it comes to hovering effects on buttons in Material UI, the key mechanism at work is CSS-in-JS methodology, which allows us to manipulate button hover background color and text color.
Consider a simple button generated using Material UI’s
Button
component. The hover color is typically changed via the
:hover
pseudo-class. However, with Material UI, hover color is manipulated utilizing the component’s
makeStyles
hook function where you define your unique styling rules.
Here is an illustration showing how to set the hover background color and text color on a Material UI Button:
html
import React from ‘react’;
import { makeStyles } from ‘@material-ui/core/styles’;
import Button from ‘@material-ui/core/Button’;
const useStyles = makeStyles((theme) => ({
customHoverFocus: {
‘&:hover, &.Mui-focusVisible’: {
backgroundColor: ‘green’,
color: ‘white’
}
},
}));
export default function CustomButton() {
const classes = useStyles();
return (
);
}
With the above code:
• We import the necessary dependencies, namely the
makeStyles
hook from
'@material-ui/core/styles'
and the
Button
component.
• We generate a custom style using the
makeStyles
hook that changes both the background color (set to green) and the text color (set to white) while hovering over the button or focusing on it.
• We render the Button element with our custom className, which triggers our interactive color changes upon hover or focus.
It is critical to comprehend that the Material UI framework operates by CSS-in-JS, a modern method that introduces slight syntax modifications but broadens potential style applicability.
As Tony de Araujo once said, “With an abundance of information coding is becoming a societal norm, and it pays off to be well prepared.” Thus exploration of new methodologies like CSS-in-JS propels us further into frontend proficiency.
If you want in-depth details about these topics, consider spending some time on the official Material-UI documentation. It’s a remarkable resource for understanding the ins and outs of styling components, including hover effects and much more.
The Impact of Background and Text Color on UI Design
Material UI, as a system that applies principles from the Google Material Design guidelines to user interface design, pays special consideration to color aesthetics, in particular the button hover background color and text color. The impact of these two aspects on user experience and overall application interaction can be substantial.
The Role of Color in Material UI
The world of Material UI does not just prioritize geometric shapes or smooth animations; colors and their correct implementation represent a crucial part of this design language (Material-UI: A popular React UI framework, n.d.). From basic operations such as reading text to complex associations for brand identification, color serves multiple purposes.
Underlying this phenomenon are a few key points:
– Readability: Proper contrast between text color and background color enhances readability. For instance, dark text on a light background is usual and common for general reading ease. On hovering over a button, a slightly darker shade of the background color assists users in identifying interactive elements.
– Emphasis: Certain actions can be accentuated using color manipulation to direct the user’s attention towards specific tasks. Material UI often uses vibrant hues for primary buttons while secondary actions might have less saturated colors.
– Simply put, well-paired colors look pleasing to the eye. An agreeable color palette can elevate a user interface from functional to exceptional.
Applying Background and Text Colors in Material UI
When a mouse pointer hovers over a Material UI button, it may change its background color and/or text color to signal interactivity. This visual feedback enhances the user experience by making the interface more intuitive.
Here’s how you might define hover styles for a button in Material UI:
{`const useStyles = makeStyles({ root: { '&:hover': { backgroundColor: 'desiredColor', color: 'desiredTextColor' }, }, });`}
In this snippet, `useStyles` is a hook from the @material-ui/styles package that creates a Hook API to access the theme styles. In the `’:hover’` pseudo-class, we set the `backgroundColor` and `color` properties to the desired values to change the button’s background and text colors on hover.
Compliance with Web Content Accessibility Guidelines (WCAG)
Moreover, it’s important to note that the choice of colors in UI design should also be compliant with accessibility standards such as the WCAG guidelines (Web Content Accessibility Guidelines (WCAG) 2.1, n.d.). These standards help ensure that web content and interfaces are easily navigable and understandable for people with visual impairments.
As stated by Abraham Lincoln, “The best way to predict the future is to create it.” Thus, taking into consideration these factors while crafting your Material UI designs, you can control how users interact with, perceive, and react to your application.
Exploring Customizations: Changing Button Hover Features in Material-UI
Material-UI is a well-defined UI library built on the foundation of React, that allows developers to build dynamic and interactive components with much ease. A popular feature of Material-UI that requires frequent customization is its buttons. In this context, we’ll take a look at customizing the Button hover features: particularly changing Background Color and Text Color.
Understanding the basics of Material-UI styling solutions is vital here. The utilization of CSS-in-JS solutions enables changes to the hover feature in Material-UI, while allowing a direct association of these styles with components. The styled-components provides robust solutions for CSS-in-JS, including dynamic styles as well.
As an example of button customization, if you intend to change the background color and text color on hovering, it could be achieved by:
const CustomButton = withStyles({ root: { '&:hover': { backgroundColor: 'purple', color: 'yellow', }, }, })(Button);
In the above snippet:
• ‘withStyles’ is a higher order component (HOC) returned from ‘@material-ui/core/styles’, which takes styles as input and returns a new function.
• The HOC wraps around the Material UI’s Button. It injects classes into props, thereby enabling style overwrites.
• In our code snippet, ‘:hover’ specifies the styles to be applied when Button is hovered upon. Upon hovering, background color changes to purple and text color switches to yellow
However, note that using ‘!important’ tag is generally discouraged unless absolutely necessary.
Esther Dyson, a technology entrepreneur once said – “The great thing about standards is that there are so many to choose from.” This is very true especially when dealing with a vast library such as Material-UI. One can offer numerous ways for customization and creating unique components. Hence, refreshing your understanding about various methods will allow you to become more proficient and efficient while working with Material-UI.
While these modifications may seem simple, they can make a significant positive impact on the user interface of your web application – offering an engaging and user-friendly experience.
For additional resources, the official Material UI documentation is an excellent start to delve into more ways for customization.
Material UI, a popular set of React components that implements Google’s Material Design specification, offers programmers an easy and efficient way to design stunning user interfaces. One core feature of this library is its Button component, which can be styled in various manners, including changing the background and text color during hover events.
Changing the color of the button and text when hovering over the button using Material UI involves working with a theme and overriding styles using makeStyles or withStyles functions. The hover effect can drastically improve the aesthetics of your application, making it more engaging for users and promoting further interaction.
Using CSS-in-JS solutions provided by Material-UI, we can easily change the Button hover background and text color. To illustrate,
import React from 'react'; import { makeStyles } from '@material-ui/core/styles'; import Button from '@material-ui/core/Button'; const useStyles = makeStyles((theme) => ({ button: { '&:hover': { backgroundColor: "green", color: "white" }, }, })); export default function HoverButton() { const classes = useStyles(); return ( ); }
Here, a style rule for hover state has been added to change the Button’s background color to green and the text color to white.
As we leverage the power of Material UI’s styling capabilities, “Modularity and customization are the heart and soul of Material-UI. Embrace it, and customize the crap out of it.” – Olivier Tassinari, one of Material UI’s creators.
Working with Material UI’s Button component provides us an opportunity to focus on creating a more interactive user interface experience. By mastering the nuances of styling such components, developers can create visually appealing applications, which do not only engage users but also improve usability.
For more information about overriding styles in Material UI, refer to their official documentation on advanced styling techniques. Understanding these principles contributes to building better, more dynamic applications using Material UI. It illuminates how versatile and adaptable the library is when it comes to conforming to specific design requirements.
Remember: the hover effect is a subtle yet powerful way to improve user experience. It provides visual feedback to users about their interaction with your application’s interface, making them feel more connected and in control.